Why Are Control Structures Essential in Python Programming?
In the world of programming, control structures are the backbone of logic and decision-making, allowing developers to dictate the flow of execution in their code. In Python, a language celebrated for its simplicity and readability, control structures play a pivotal role in transforming static lines of code into dynamic, responsive applications. Whether you’re building a robust web application, automating mundane tasks, or analyzing data, understanding how to effectively use control structures is essential for any aspiring Python programmer.
Control structures in Python come in various forms, each serving a distinct purpose in guiding the program’s execution. At their core, they enable programmers to make decisions, repeat actions, and manage the flow of control based on specific conditions or iterations. From the ubiquitous `if` statements that allow for conditional execution to loops that facilitate repetitive tasks, these structures empower developers to create more efficient and adaptable code.
Moreover, mastering control structures not only enhances the functionality of your programs but also improves their readability and maintainability. By learning how to implement these structures effectively, you can write code that is not only powerful but also easy for others (and your future self) to understand. As we delve deeper into the intricacies of Python’s control structures, you will discover their significance and the myriad ways they can
Understanding Control Structures in Python
Control structures are fundamental components in Python programming that dictate the flow of control within a program. They allow developers to manage the execution of code based on certain conditions, enabling the creation of dynamic and responsive applications. The primary types of control structures in Python include conditional statements, loops, and exception handling.
Conditional Statements
Conditional statements enable a program to execute specific blocks of code based on logical conditions. The primary conditional structures in Python are the `if`, `elif`, and `else` statements.
- if statement: Executes a block of code if a specified condition is true.
- elif statement: Allows for additional conditions to be checked if the previous conditions are .
- else statement: Executes a block of code when none of the preceding conditions are true.
Example syntax:
“`python
if condition1:
code block to execute if condition1 is true
elif condition2:
code block to execute if condition2 is true
else:
code block to execute if neither condition1 nor condition2 is true
“`
Loops
Loops are control structures that allow for repeated execution of a block of code. Python primarily supports two types of loops: `for` loops and `while` loops.
- for loop: Iterates over a sequence (like a list, tuple, or string) or other iterable objects.
- while loop: Repeats a block of code as long as a specified condition is true.
Example syntax for each:
“`python
for loop example
for item in iterable:
code block to execute for each item
while loop example
while condition:
code block to execute as long as condition is true
“`
Control Flow Statements
In addition to basic loop structures, Python provides control flow statements such as `break`, `continue`, and `pass` that enhance loop functionality.
- break: Terminates the loop immediately and transfers control to the statement following the loop.
- continue: Skips the current iteration and proceeds to the next iteration of the loop.
- pass: A null statement that is syntactically required but does not perform any action.
Exception Handling
Exception handling is crucial for managing errors and exceptional conditions in Python applications. It ensures that a program can handle unexpected events gracefully without crashing. The primary keywords used for exception handling are `try`, `except`, `finally`, and `else`.
Example structure:
“`python
try:
code that may raise an exception
except SomeException as e:
code that runs if an exception occurs
finally:
code that runs regardless of whether an exception occurred
“`
Control Structure | Purpose |
---|---|
if | Execute code based on a condition |
for | Iterate over a sequence |
while | Execute code as long as a condition is true |
try-except | Handle exceptions gracefully |
Utilizing control structures effectively allows developers to write more efficient, readable, and maintainable code. Understanding how to implement these structures is essential for any Python programmer aiming to create robust applications.
Control Structures in Python
Control structures are essential components of programming in Python, allowing developers to dictate the flow of execution based on certain conditions. They enable the creation of dynamic and responsive programs by facilitating decision-making processes, repetitive tasks, and the handling of multiple scenarios.
Types of Control Structures
Python primarily utilizes three types of control structures:
- Conditional Statements: Allow execution of code based on specific conditions.
- Loops: Facilitate the repetition of code until a certain condition is met.
- Control Flow Statements: Modify the flow of execution based on specific instructions.
Conditional Statements
Conditional statements evaluate expressions and execute different blocks of code accordingly. The primary forms include:
- if Statement: Executes a block of code if a condition is true.
- elif Statement: Checks additional conditions if the previous ones are .
- else Statement: Executes a block of code when none of the preceding conditions are true.
Example:
“`python
age = 18
if age < 18:
print("Minor")
elif age == 18:
print("Just an adult")
else:
print("Adult")
```
Loops
Loops are used for executing a block of code multiple times, either a fixed number of times or until a specific condition is met. The main types of loops in Python include:
- for Loop: Iterates over a sequence (like a list, tuple, or string).
- while Loop: Continues executing as long as a condition remains true.
Example:
“`python
For loop
for i in range(5):
print(i)
While loop
count = 0
while count < 5:
print(count)
count += 1
```
Control Flow Statements
Control flow statements provide advanced control over loop and conditional execution. Key statements include:
- break: Exits the nearest enclosing loop.
- continue: Skips the rest of the current loop iteration and moves to the next iteration.
- pass: A null operation placeholder, often used when a statement is syntactically required but no action is necessary.
Example:
“`python
for i in range(5):
if i == 3:
break Exit the loop when i is 3
print(i)
for i in range(5):
if i == 2:
continue Skip the iteration when i is 2
print(i)
“`
Using Control Structures Effectively
To maximize the efficiency of control structures in Python, consider the following best practices:
- Keep conditions simple: Complex conditions can lead to confusion and errors.
- Avoid deep nesting: Too many nested structures can make the code difficult to read.
- Utilize functions: Abstracting repetitive logic into functions can reduce redundancy and improve clarity.
By mastering control structures, developers enhance their ability to create robust, maintainable, and efficient Python applications.
Understanding the Role of Control Structures in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Control structures are fundamental in Python as they allow developers to dictate the flow of execution in a program. By utilizing conditionals and loops, programmers can create dynamic applications that respond to user input and external data, making the software more interactive and efficient.
James Liu (Lead Data Scientist, Data Insights Group). In Python, control structures are essential for managing complex data processing tasks. They enable the implementation of algorithms that can iterate over datasets, apply conditional logic, and optimize performance by avoiding unnecessary computations. This is particularly crucial in data analysis and machine learning applications.
Sarah Thompson (Computer Science Educator, Future Coders Academy). Teaching control structures in Python is vital for students as it lays the groundwork for understanding programming logic. By mastering if statements, loops, and function calls, learners can build a strong foundation that prepares them for more advanced concepts in software development and problem-solving.
Frequently Asked Questions (FAQs)
What do we use control structures for in Python?
Control structures in Python are used to dictate the flow of execution in a program. They allow developers to implement decision-making, looping, and branching logic, enabling the program to respond dynamically to different conditions.
What are the main types of control structures in Python?
The main types of control structures in Python include conditional statements (if, elif, else), loops (for and while), and control flow statements (break, continue, and pass). Each serves a specific purpose in managing how code is executed.
How do conditional statements work in Python?
Conditional statements evaluate expressions and execute specific blocks of code based on whether the expressions evaluate to true or . This allows for branching logic in programs, enabling different outcomes based on varying conditions.
What is the purpose of loops in Python?
Loops in Python are used to repeatedly execute a block of code as long as a specified condition is true. This is useful for tasks that require iteration over collections or when a task needs to be performed multiple times without manually repeating the code.
Can you explain the difference between ‘break’ and ‘continue’ in loops?
The ‘break’ statement terminates the loop entirely, exiting the loop when a certain condition is met. In contrast, the ‘continue’ statement skips the current iteration and proceeds to the next iteration of the loop, allowing for selective execution of loop cycles.
Why are control structures important in programming?
Control structures are crucial in programming as they enhance the flexibility and efficiency of code. They enable developers to create dynamic applications that can handle various scenarios, improving both user experience and functionality.
Control structures in Python are fundamental components that allow developers to dictate the flow of execution within a program. They enable the implementation of decision-making processes, iterations, and branching logic, which are essential for creating dynamic and responsive applications. By utilizing control structures such as conditional statements (if, elif, else) and loops (for, while), programmers can manage how data is processed and how the program behaves under various conditions.
These structures enhance the ability to write efficient and readable code. For instance, conditional statements facilitate the execution of specific code blocks based on certain criteria, allowing for tailored responses to user inputs or other variables. Similarly, loops enable repetitive tasks to be performed without the need for redundant code, thus promoting code reusability and maintainability. Overall, control structures are pivotal in transforming static code into interactive and functional programs.
In summary, control structures are indispensable tools in Python programming that empower developers to create complex logic and workflows. Their proper use not only improves the functionality of applications but also contributes to cleaner and more organized code. Understanding and effectively implementing these structures is crucial for anyone looking to excel in Python programming and software development.
Author Profile
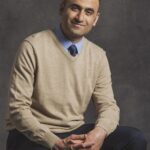
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?