What Does a Colon Mean in Python? Understanding Its Role in Syntax
In the world of programming, every character has its significance, and in Python, the colon (`:`) is no exception. This seemingly simple punctuation mark plays a crucial role in defining the structure and flow of code, acting as a gateway to various programming constructs. Whether you’re a novice coder or a seasoned developer, understanding the function of the colon in Python can enhance your coding efficiency and clarity. Join us as we delve into the multifaceted uses of this essential symbol, unraveling its importance in creating readable and effective Python scripts.
The colon is primarily used in Python to introduce blocks of code, signaling that what follows is a new scope or a set of instructions that belong together. This is particularly evident in control structures such as loops and conditional statements, where the colon indicates the beginning of the block that will execute based on a certain condition. By establishing clear boundaries within the code, the colon helps maintain organization and readability, which are vital in collaborative programming environments.
Beyond control structures, the colon also plays a pivotal role in defining functions and classes, marking the start of their respective bodies. In addition, it is employed in slicing operations for lists and strings, allowing for concise data manipulation. As we explore the various contexts in which the colon appears, you’ll discover how this small but mighty character
Understanding the Role of Colons in Python
In Python, the colon (`:`) serves several critical roles that are essential for the syntax and structure of the language. It is primarily used to denote the start of an indented block of code, which is a fundamental aspect of Python’s design. This indentation signifies that the code following the colon is part of a control structure, function definition, or class definition.
Colons in Control Structures
In control structures such as `if`, `for`, and `while`, colons are used to indicate the beginning of the block of code that will execute based on the condition specified. For example:
“`python
if condition:
code block to execute if condition is True
do_something()
“`
In this example, the colon follows the `if` statement, and the indented code that follows is executed only if `condition` evaluates to `True`.
Colons in Function and Class Definitions
Colons are also utilized in defining functions and classes, marking the start of the respective code blocks:
“`python
def my_function():
code block for function
print(“Hello, World!”)
class MyClass:
code block for class
def method(self):
print(“Method in MyClass”)
“`
In both cases, the colon indicates that the subsequent lines are part of the function or class body.
Colons in Dictionary Key-Value Pairs
In dictionaries, colons play a crucial role in separating keys from their corresponding values. The syntax for creating a dictionary involves placing a colon between each key and value pair, as illustrated below:
“`python
my_dict = {
“key1”: “value1”,
“key2”: “value2”,
}
“`
Here, the colon indicates that `”key1″` corresponds to `”value1″` and `”key2″` corresponds to `”value2″`.
Table of Colons Usage
Context | Usage of Colon |
---|---|
Control Structures | Indicates the start of a code block following conditions |
Function Definitions | Denotes the beginning of the function’s body |
Class Definitions | Marks the start of the class body |
Dictionaries | Separates keys from values in key-value pairs |
Additional Uses of Colons
Colons can also be found in list comprehensions and slicing operations. In list comprehensions, they help in defining the expression that generates the list. For example:
“`python
squares = [x**2 for x in range(10)]
“`
In slicing, the colon is used to specify the start and end indices of the slice:
“`python
my_list = [0, 1, 2, 3, 4, 5]
subset = my_list[1:4] results in [1, 2, 3]
“`
This versatility makes the colon a powerful component of Python syntax, helping to define the structure and flow of the code effectively.
Colon Usage in Python
In Python, the colon (`:`) serves several important functions within the syntax of the language. It is primarily used to indicate the beginning of an indented block of code. Below are the main contexts in which the colon is utilized:
Control Structures
Colons are essential in defining control structures, such as conditionals and loops. When used in these contexts, the colon signals the start of a block of code that belongs to the control statement.
- If Statements:
“`python
if condition:
Block of code to execute if condition is True
“`
- For Loops:
“`python
for item in iterable:
Block of code to execute for each item
“`
- While Loops:
“`python
while condition:
Block of code to execute while condition is True
“`
Function Definitions
The colon is also employed in function definitions to denote the start of the function body. This follows the function signature.
- Defining a Function:
“`python
def my_function(parameters):
Code to execute when the function is called
“`
Class Definitions
When defining a class, the colon indicates the beginning of the class body, similar to functions.
- Class Definition Example:
“`python
class MyClass:
Code defining the class attributes and methods
“`
Data Structures
In dictionaries, the colon separates keys from their corresponding values, forming key-value pairs.
- Dictionary Example:
“`python
my_dict = {
‘key1’: ‘value1’,
‘key2’: ‘value2’
}
“`
List Comprehensions
Colons appear in more complex constructs like list comprehensions, particularly when using the `if` statement to filter items.
- List Comprehension Example:
“`python
squares = [x**2 for x in range(10) if x % 2 == 0]
“`
Lambda Functions
In lambda functions, the colon separates the parameters from the expression being evaluated.
- Lambda Function Example:
“`python
square = lambda x: x ** 2
“`
Type Hints
Colons are also used in function annotations to specify the expected types of parameters and return values.
– **Type Hint Example**:
“`python
def add(a: int, b: int) -> int:
return a + b
“`
Understanding the usage of the colon in Python is crucial for writing syntactically correct and effective code. Its roles in defining structures, separating elements, and indicating blocks of code are foundational to mastering Python programming.
Understanding the Role of Colons in Python Programming
Dr. Emily Carter (Senior Python Developer, CodeCraft Solutions). The colon in Python serves as a crucial syntactical marker that indicates the start of an indented block of code. This is particularly important in defining structures such as functions, loops, and conditional statements, where the subsequent indented lines are executed as part of the defined block.
Michael Chen (Lead Software Engineer, Tech Innovations Inc.). In Python, the colon is not just a stylistic choice; it is a requirement for the language’s syntax. It helps to delineate the header of control structures, making the code more readable and organized. The use of colons contributes to Python’s emphasis on clean and understandable code.
Sarah Patel (Python Educator and Author, LearnPython.org). Understanding the function of the colon is essential for beginners. It signals to the interpreter that what follows will be a block of code that is logically grouped together. This feature of Python enhances its readability and encourages best practices in coding.
Frequently Asked Questions (FAQs)
What does a colon mean in Python?
A colon in Python indicates the start of an indented block of code, commonly used in control structures such as loops, conditionals, and function definitions.
Where is a colon used in Python syntax?
Colons are used after keywords like `if`, `for`, `while`, `def`, and `class` to signify that a block of code follows, which must be indented.
Why is indentation important after a colon in Python?
Indentation is crucial in Python as it defines the scope and structure of the code blocks, replacing the need for braces used in other programming languages.
Can a colon be used in other contexts in Python?
Yes, colons are also used in slicing lists and strings, as well as in dictionary key-value pairs, where they separate keys from their corresponding values.
What happens if I forget to include a colon in a control structure?
Omitting a colon will result in a `SyntaxError`, as Python expects the colon to indicate the start of a new block of code.
Is it possible to use a colon in variable names in Python?
No, colons cannot be used in variable names in Python. They are reserved for specific syntactical purposes within the language.
In Python, the colon (:) serves as a critical syntactical element that indicates the beginning of an indented block of code. It is commonly used in various constructs such as function definitions, conditional statements, loops, and class definitions. The presence of a colon signals to the interpreter that the subsequent lines will be part of a new code block that is logically associated with the preceding statement.
Moreover, the colon is essential for defining the structure of the code, as it helps delineate where a block starts. For instance, in an if statement, the colon indicates that the subsequent indented lines are to be executed if the condition evaluates to true. This mechanism is central to Python’s readability and its emphasis on clear, concise code formatting.
In summary, the colon in Python is not merely a punctuation mark; it plays a fundamental role in the language’s syntax. Understanding its function is crucial for writing effective Python code, as it directly impacts how code is organized and executed. Mastery of the colon’s use can enhance both the clarity and functionality of programming in Python.
Author Profile
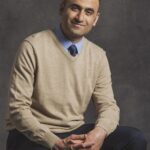
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?