What Does the abs Function Do in Python? Exploring Its Purpose and Usage
In the world of programming, Python stands out for its simplicity and versatility, making it a favorite among both beginners and seasoned developers. One of the many built-in functions that contribute to Python’s ease of use is the `abs()` function. While it may seem straightforward at first glance, understanding what `abs()` does and how it can be effectively utilized can enhance your coding skills and problem-solving abilities. Whether you’re performing mathematical calculations or manipulating data, the `abs()` function offers a straightforward way to work with numerical values, paving the way for cleaner and more efficient code.
The `abs()` function in Python serves a specific purpose: it returns the absolute value of a given number. This means that regardless of whether the input is positive or negative, the output will always be a non-negative number. This fundamental concept is crucial in various programming scenarios, from mathematical computations to data analysis, where the sign of a number may not be relevant to the task at hand. By leveraging the `abs()` function, programmers can streamline their code and focus on the underlying logic without getting bogged down by unnecessary complications.
In addition to its primary function, `abs()` is often used in conjunction with other operations, making it a powerful tool in a programmer’s arsenal. Understanding how to
Understanding the `abs()` Function in Python
The `abs()` function in Python is a built-in function that returns the absolute value of a number. The absolute value of a number is its distance from zero on the number line, regardless of direction. This means that `abs()` converts negative values to positive values while leaving positive values unchanged.
The syntax for the `abs()` function is straightforward:
“`python
abs(x)
“`
Where `x` can be an integer, a floating-point number, or a complex number. The return type of `abs()` corresponds to the type of the argument provided.
Key Characteristics of `abs()`
- It accepts different numeric types: integers, floats, and complex numbers.
- The return value is always non-negative.
- For complex numbers, it computes the magnitude.
Usage Examples
To illustrate the use of the `abs()` function, consider the following examples:
“`python
For integers
print(abs(-5)) Output: 5
print(abs(3)) Output: 3
For floats
print(abs(-3.14)) Output: 3.14
print(abs(2.71)) Output: 2.71
For complex numbers
print(abs(3 + 4j)) Output: 5.0
“`
Return Types
The return type of the `abs()` function is determined by the type of the argument passed:
Input Type | Return Type |
---|---|
Integer | Integer |
Float | Float |
Complex Number | Float |
Practical Applications
The `abs()` function can be utilized in various scenarios:
- Mathematical computations: When performing calculations that require non-negative results.
- Distance calculations: Useful in geometric contexts where distance must be calculated without regard to direction.
- Error calculations: Often applied in statistics or data analysis to assess deviations or errors, such as calculating the absolute error between predicted and actual values.
Conclusion
The `abs()` function is a fundamental tool in Python for anyone needing to manipulate or analyze numeric data. Its simplicity and effectiveness make it a go-to function for ensuring values are non-negative, which is essential in many mathematical and computational contexts.
Understanding the abs() Function in Python
The `abs()` function in Python is a built-in function that returns the absolute value of a given number. Absolute value is the non-negative value of a number without regard to its sign. The function can handle integers, floating-point numbers, and even complex numbers.
Function Signature
The signature of the `abs()` function is as follows:
“`python
abs(x)
“`
- Parameter:
- `x`: This can be an integer, a float, or a complex number.
- Return Value:
- The function returns the absolute value of `x`.
Usage Examples
The `abs()` function can be utilized in various scenarios, including mathematical calculations and data processing. Here are some examples:
“`python
Example with integer
result1 = abs(-10) Output: 10
Example with float
result2 = abs(-3.14) Output: 3.14
Example with complex number
result3 = abs(3 + 4j) Output: 5.0
“`
Detailed Explanation of Behavior
- For Integers:
- It converts negative integers to positive and leaves positive integers unchanged.
- For Floats:
- Similar to integers, it removes the negative sign of negative floating-point numbers.
- For Complex Numbers:
- The absolute value is computed using the formula:
\[ |a + bi| = \sqrt{a^2 + b^2} \]
- For example, the absolute value of \(3 + 4j\) is computed as follows:
\[
3 + 4j | = \sqrt{3^2 + 4^2} = \sqrt{9 + 16} = \sqrt{25} = 5.0 |
---|
\]
Common Use Cases
The `abs()` function is commonly employed in various programming scenarios, such as:
- Mathematical Calculations: To ensure values are non-negative when performing arithmetic operations.
- Data Analysis: To analyze deviations from a mean or expected value.
- Distance Calculations: To compute distances in various algorithms, such as in machine learning.
Performance Considerations
The `abs()` function is optimized for performance in Python, operating in constant time regardless of the input size. However, developers should be aware of:
- Type Coercion: Ensure that the input type is compatible to avoid unexpected errors.
- Complex Numbers: The operation might be slightly more computationally expensive due to the square root calculation involved.
Alternative Methods
While `abs()` is the standard way to obtain absolute values, there are alternative methods, such as using conditional statements. However, these methods are less efficient and less readable than the built-in function.
Method | Example | Efficiency |
---|---|---|
Built-in abs() | `abs(-5)` | O(1) |
Conditional | `-x if x < 0 else x` | O(1) |
Utilizing `abs()` is recommended for clarity and performance in Python programming.
Understanding the Role of the abs() Function in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The abs() function in Python is essential for obtaining the absolute value of a number. It effectively eliminates any negative sign, ensuring that the output is always non-negative, which is particularly useful in mathematical computations and data analysis.”
Michael Chen (Data Scientist, Big Data Analytics Group). “In data processing, the abs() function plays a crucial role in transforming datasets. By converting negative values to their positive counterparts, it allows for more accurate statistical analyses and visualizations, especially when dealing with metrics like distances or deviations.”
Sarah Thompson (Python Developer, CodeCraft Solutions). “Using the abs() function is straightforward in Python. It can be applied to integers, floats, and even complex numbers, making it a versatile tool in a developer’s toolkit for ensuring that calculations remain within expected bounds.”
Frequently Asked Questions (FAQs)
What does the abs function do in Python?
The `abs` function in Python returns the absolute value of a number, which is the non-negative value of that number without regard to its sign.
What types of arguments can be passed to the abs function?
The `abs` function can accept integers, floating-point numbers, and complex numbers. For complex numbers, it returns the magnitude.
How is the abs function used in Python code?
The `abs` function is used by calling it with a number as an argument, such as `abs(-5)` which returns `5`, or `abs(-3.14)` which returns `3.14`.
Can the abs function be used with variables?
Yes, the `abs` function can be used with variables. For example, if `x = -10`, then `abs(x)` will return `10`.
Is the abs function a built-in function in Python?
Yes, `abs` is a built-in function in Python, meaning it is readily available without the need for any imports.
Are there any performance considerations when using the abs function?
The `abs` function is highly optimized for performance in Python, and its execution time is negligible for typical use cases. However, unnecessary calls in large loops should be avoided for efficiency.
The `abs()` function in Python is a built-in function that returns the absolute value of a number. It can be applied to integers, floating-point numbers, and complex numbers. The primary purpose of this function is to eliminate any negative sign from the number, thereby providing a non-negative value. This functionality is particularly useful in mathematical computations where the magnitude of a number is more significant than its sign.
In addition to its basic usage, the `abs()` function is versatile and can be employed in various contexts, such as data analysis, algorithm development, and mathematical modeling. Its ability to handle different data types makes it a valuable tool for developers and data scientists alike. For instance, when working with distances or differences, the absolute value provides clarity and ensures that calculations yield meaningful results.
Furthermore, understanding the `abs()` function is essential for anyone looking to write efficient and effective Python code. By incorporating this function into their programming practices, developers can simplify their logic and improve the readability of their code. Overall, the `abs()` function is a fundamental aspect of Python that enhances mathematical operations and contributes to cleaner, more efficient programming.
Author Profile
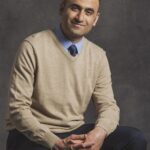
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?