What Does the Colon Do in Python? Unraveling Its Purpose and Importance
In the world of Python programming, the colon (:) is a small yet powerful character that plays a crucial role in defining structure and functionality within your code. While it may seem like a simple punctuation mark, the colon serves as a gateway to a range of programming constructs, from loops and conditionals to function definitions and class declarations. Understanding the significance of the colon is essential for both novice and experienced programmers alike, as it helps to establish clarity and organization in code, making it more readable and maintainable.
At its core, the colon acts as a signal that introduces a new block of code, indicating that what follows is a suite of statements that belong together. This is particularly important in Python, where indentation is used to define the scope of loops, functions, and conditionals. By mastering the use of the colon, programmers can effectively control the flow of their code, ensuring that each component interacts seamlessly with others.
Moreover, the colon is not just limited to control structures; it also plays a vital role in data structures such as dictionaries and slicing operations with lists and tuples. Its versatility makes it an indispensable tool in a Python programmer’s toolkit. As we delve deeper into the various contexts in which the colon is used, you’ll discover how this seemingly simple character can unlock a wealth
Understanding the Role of the Colon in Python
In Python, the colon (`:`) is a syntactical element that serves multiple purposes, primarily indicating the beginning of an indented block of code. This usage is critical for defining structures such as functions, loops, conditionals, and class definitions. Below are some key areas where the colon plays a significant role:
Defining Functions
When defining a function, the colon follows the function signature. It signifies that the subsequent lines will constitute the function’s body, which must be indented.
“`python
def my_function():
print(“Hello, World!”)
“`
Conditional Statements
In conditional statements, the colon is used after the `if`, `elif`, and `else` keywords to indicate the start of the block that will execute based on the condition’s truth value.
“`python
if x > 0:
print(“Positive”)
elif x < 0:
print("Negative")
else:
print("Zero")
```
Loops
The colon is also essential in loops, such as `for` and `while`, marking the beginning of the code block that will repeat.
“`python
for i in range(5):
print(i)
“`
“`python
while condition:
Code to execute while the condition is true
“`
Class Definitions
In class definitions, the colon indicates the start of the class body. All methods and properties of the class must be indented beneath the class definition.
“`python
class MyClass:
def my_method(self):
print(“Method of MyClass”)
“`
Data Structures
In data structures like dictionaries, the colon separates keys from values, establishing key-value pairs.
“`python
my_dict = {
“name”: “John”,
“age”: 30
}
“`
Summary of Colon Usage in Python
The colon in Python serves various critical functions, as summarized in the table below:
Context | Usage |
---|---|
Function Definition | Indicates the start of the function body |
Conditional Statements | Marks the beginning of the conditional block |
Loops | Denotes the start of the loop block |
Class Definition | Indicates the start of the class body |
Dictionaries | Separates keys from values |
Understanding the role of the colon is essential for mastering Python syntax and ensuring code clarity and functionality. Each instance where a colon is used marks a transition into a new block of code, emphasizing Python’s reliance on indentation and structure.
Function Definition
In Python, a colon (`:`) is used to denote the start of a block of code. This is particularly significant in defining functions. For example:
“`python
def my_function():
print(“Hello, World!”)
“`
Here, the colon indicates that the following indented lines belong to the `my_function` block.
Control Structures
Colons are also employed in control structures, such as `if`, `for`, `while`, and `try`. Each of these structures requires a colon to signify the beginning of the block of code that will execute based on the condition or iteration. For example:
“`python
if condition:
execute_code()
“`
In this snippet, the colon indicates that the subsequent indented code will execute if `condition` evaluates to `True`.
Classes and Methods
When defining classes and their methods, a colon is used similarly to indicate that the following lines constitute the class or method body. The syntax is as follows:
“`python
class MyClass:
def my_method(self):
print(“Method in MyClass”)
“`
The colons after `class MyClass` and `def my_method(self)` show where the class and method bodies begin.
Data Structures
Colons are also used in data structures such as dictionaries, where they separate keys from values. For instance:
“`python
my_dict = {
“key1”: “value1”,
“key2”: “value2”
}
“`
In this dictionary, the colon separates each key from its corresponding value.
List Comprehensions and Slicing
In list comprehensions and slicing, colons play a role in defining ranges and conditions. For instance:
“`python
squared_numbers = [x**2 for x in range(10)]
sliced_list = my_list[start:end]
“`
In slicing, the colon indicates the start and end indices of the list.
Lambda Functions
The colon is also used in lambda functions to separate parameters from the expression. For example:
“`python
add = lambda x, y: x + y
“`
Here, the colon separates the parameters `x` and `y` from the expression `x + y` that defines the function’s behavior.
Summary of Usage
The colon in Python serves multiple purposes, including:
- Function Definitions: Marks the start of a function block.
- Control Structures: Indicates the beginning of conditional or iterative blocks.
- Class and Method Definitions: Denotes the body of classes and methods.
- Dictionaries: Separates keys and values.
- List Comprehensions and Slicing: Defines ranges and conditions.
- Lambda Functions: Distinguishes parameters from expressions.
The colon is a fundamental syntax element in Python, essential for structuring code clearly and functionally.
Understanding the Role of the Colon in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). The colon in Python serves as a critical syntactical element that indicates the start of an indented block of code. It is essential in defining structures such as functions, loops, and conditional statements, thereby enhancing code readability and organization.
Michael Thompson (Lead Python Developer, CodeCraft Solutions). In Python, the colon acts as a delimiter that separates the header of a control structure from its body. This distinction is vital for the interpreter to understand the scope of the code that follows, ensuring that the program executes as intended.
Sarah Lee (Python Educator, LearnPython.org). The colon is not just a syntactical requirement; it plays a significant role in Python’s design philosophy, which emphasizes clarity and simplicity. By using colons to denote blocks of code, Python allows developers to write clean and maintainable code, which is especially beneficial for beginners.
Frequently Asked Questions (FAQs)
What does a colon do in Python?
A colon in Python is used to indicate the start of an indented block of code. It is commonly found in control flow statements, such as `if`, `for`, `while`, and function definitions.
How is a colon used in function definitions?
In function definitions, a colon follows the function header to signify the beginning of the function body, which contains the code that will be executed when the function is called.
What is the significance of a colon in conditional statements?
In conditional statements, a colon is used after the condition to indicate that the subsequent indented lines are the block of code that will execute if the condition evaluates to true.
Can you provide an example of a colon in a loop?
Certainly. In a `for` loop, a colon is placed after the loop declaration, such as `for i in range(5):`, followed by an indented block that specifies the actions to be performed for each iteration.
Are there other contexts where colons are used in Python?
Yes, colons are also used in dictionary definitions to separate keys from values, as well as in slicing syntax to specify start and end indices in lists and other sequences.
What happens if a colon is omitted in Python?
If a colon is omitted where it is required, Python will raise a `SyntaxError`, indicating that the expected block of code is not properly defined.
The colon in Python serves several critical functions that are essential for the structure and readability of the code. Primarily, it is used to denote the beginning of an indented block of code following statements such as function definitions, class definitions, conditional statements, and loops. This syntactical requirement helps Python maintain a clear and organized flow of control, distinguishing between the header of a statement and its subsequent body.
In addition to its role in defining code blocks, the colon is also employed in slicing operations, particularly with lists and strings. In this context, it separates the start and end indices, allowing for the extraction of specific segments of data. This versatility of the colon enhances Python’s expressiveness and efficiency, enabling developers to write concise and readable code.
Overall, understanding the functions of the colon in Python is fundamental for anyone looking to master the language. Its use in defining code structure and facilitating data manipulation underscores the importance of proper syntax in programming. As such, developers should pay close attention to the placement and context of colons in their code to ensure clarity and functionality.
Author Profile
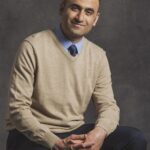
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?