What Does the Colon Mean in Python? Understanding Its Role and Usage
In the world of programming, every symbol carries its own significance, and Python is no exception. Among these symbols, the colon (`:`) stands out as a powerful and versatile character that plays a crucial role in the structure and readability of Python code. Whether you’re a seasoned developer or a newcomer to the language, understanding the function of the colon is essential for mastering Python’s syntax and flow control. This article will delve into the various contexts in which the colon is utilized, shedding light on its importance in defining blocks of code, creating functions, and more.
The colon serves as a pivotal marker in Python, indicating the start of an indented block of code that follows a statement. This simple yet effective use of the colon enhances code clarity and organization, making it easier for developers to read and maintain their programs. From defining functions and classes to controlling the flow of loops and conditionals, the colon is integral to establishing the structure that Python programmers rely on. By grasping its role, you can unlock the full potential of Python’s syntax and improve your coding proficiency.
As we explore the multifaceted uses of the colon in Python, we will uncover how this seemingly modest symbol can impact the way you write and understand code. Whether you’re looking to refine your skills or simply curious
Understanding the Role of the Colon in Python
In Python, the colon (`:`) is a critical syntactical element that serves various purposes, primarily in defining code blocks. Its placement indicates that a block of code follows, which is essential for the language’s indentation-based structure. Here are the primary uses of the colon in Python:
- Defining Functions: When creating a function, the colon follows the function declaration line, signaling the start of the function body.
“`python
def my_function():
print(“Hello, World!”)
“`
- Control Flow Statements: The colon is used in control flow statements such as `if`, `for`, `while`, and `try`. It indicates that a block of code will execute based on a condition or iteration.
“`python
if condition:
print(“Condition is True”)
“`
- Class Definitions: Similar to functions, class definitions also utilize a colon to denote the beginning of the class body.
“`python
class MyClass:
def method(self):
return “This is a method”
“`
- Lambda Functions: In lambda functions, the colon separates the parameters from the expression.
“`python
my_lambda = lambda x: x + 1
“`
Examples of Colon Usage
The following table summarizes various constructs in Python that use the colon:
Construct | Example | Description |
---|---|---|
Function Definition | def func_name(): |
Indicates the start of a function body. |
If Statement | if condition: |
Starts the block of code that executes if the condition is true. |
For Loop | for i in range(5): |
Begins the loop’s body. |
Class Definition | class MyClass: |
Marks the beginning of the class body. |
Try Statement | try: |
Indicates the start of a block that handles exceptions. |
Indentation Following the Colon
The use of the colon is closely tied to Python’s indentation rules. After a colon, the subsequent lines of code must be indented consistently to form a block. This indentation is not merely a stylistic choice; it is a syntactic requirement that Python enforces to define the scope of loops, functions, and conditionals.
- Indentation: The standard practice is to use four spaces for each indentation level. Mixing tabs and spaces can lead to errors.
“`python
if True:
print(“This is indented under the if statement”)
“`
the colon is a fundamental aspect of Python syntax that enables the definition of blocks of code for functions, classes, and control structures. Understanding its use is essential for writing clear and functional Python code.
Colon in Python: Syntax and Usage
In Python, the colon (`:`) is a crucial syntactical element that serves multiple functions. Its primary role is to indicate the start of an indented block of code, which is essential for defining the scope of control structures, functions, and classes.
Control Structures
The colon is used in control structures such as `if`, `for`, `while`, and `try`. It signifies that the following indented lines are part of the block controlled by the statement.
Example: Conditional Statements
“`python
if condition:
Code block executed if condition is true
print(“Condition is true”)
“`
Example: Loops
“`python
for i in range(5):
Code block executed in each iteration
print(i)
“`
Function and Class Definitions
In function and class definitions, the colon indicates the beginning of the function or class body.
Example: Function Definition
“`python
def my_function(param1, param2):
Code block for the function
return param1 + param2
“`
Example: Class Definition
“`python
class MyClass:
Code block for the class
def method(self):
print(“This is a method.”)
“`
Slice Notation
Colons are also utilized in slicing operations to extract portions of sequences, such as lists or strings. This allows for specifying a start index, end index, and step size.
Syntax:
“`python
sequence[start:end:step]
“`
Example: List Slicing
“`python
my_list = [0, 1, 2, 3, 4, 5]
sliced_list = my_list[1:4] Returns [1, 2, 3]
“`
Dictionary Comprehensions and Lambda Functions
In dictionary comprehensions, colons separate keys from values. Similarly, in lambda functions, colons help define the parameters and their corresponding expressions.
Example: Dictionary Comprehension
“`python
squared = {x: x**2 for x in range(5)} Creates {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
“`
Example: Lambda Function
“`python
add = lambda x, y: x + y Returns the sum of x and y
“`
Conditional Expressions
In Python, colons are used in conditional expressions (ternary operator) to define the outcome based on a condition.
Syntax:
“`python
value_if_true if condition else value_if_
“`
Example:
“`python
result = “Even” if x % 2 == 0 else “Odd”
“`
Summary of Colon Usage
Context | Description |
---|---|
Control Structures | Indicates the beginning of an indented block. |
Function Definitions | Marks the start of the function body. |
Class Definitions | Marks the start of the class body. |
Slicing | Used to specify start, end, and step in sequences. |
Dictionary Comprehensions | Separates keys from values. |
Lambda Functions | Defines parameters and expressions. |
Conditional Expressions | Separates true and outcomes. |
The colon is integral to Python’s readability and structure, guiding the indentation that defines the flow of control in programs.
Understanding the Role of Colons in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). The colon in Python serves as a critical syntactical element that indicates the start of an indented block of code. This is essential for defining structures such as functions, loops, and conditional statements, ensuring that the code’s logical flow is maintained.
Michael Chen (Python Instructor, Code Academy). In Python, the colon is not just a punctuation mark; it signifies the beginning of a new code block. This feature differentiates Python from many other programming languages, as it uses indentation rather than braces or keywords to define scope, making the code more readable and organized.
Sarah Patel (Lead Developer, Open Source Projects). The use of colons in Python is fundamental for creating clear and concise code. For example, when defining a function or initiating a loop, the colon indicates that the subsequent lines will be part of that structure. This design choice enhances the language’s simplicity and elegance.
Frequently Asked Questions (FAQs)
What does the colon mean in Python?
The colon in Python is used to indicate the start of an indented block of code. It typically follows control flow statements such as `if`, `for`, `while`, and function definitions.
How is the colon used in function definitions?
In function definitions, the colon is placed after the function header to signify the beginning of the function body. For example, `def my_function():` indicates that the subsequent indented lines form the function’s implementation.
What role does the colon play in dictionary definitions?
In Python dictionaries, the colon separates keys from their corresponding values. For instance, in `my_dict = {‘key’: ‘value’}`, the colon distinguishes `’key’` from `’value’`.
Can the colon be used in list comprehensions?
No, the colon is not used in list comprehensions. Instead, list comprehensions utilize a syntax that includes brackets and an expression followed by a `for` clause, such as `[x for x in iterable]`.
Is the colon necessary in control flow statements?
Yes, the colon is mandatory in control flow statements like `if`, `for`, and `while`. Omitting the colon will result in a syntax error, as Python requires it to define the block of code that follows.
What happens if I forget to indent after a colon?
If you forget to indent after a colon, Python will raise an `IndentationError`, indicating that it expects an indented block of code following the statement that ends with a colon.
The colon (:) in Python serves several important purposes that are fundamental to the language’s syntax and structure. Primarily, it is used to indicate the start of an indented block of code that follows certain statements, such as defining functions, classes, or control flow structures like if statements, for loops, and while loops. This use of the colon is crucial for defining the scope and organization of code, allowing Python to maintain readability and clarity, which are core principles of the language.
Additionally, the colon is employed in slicing operations, particularly with lists, tuples, and strings. In this context, it allows for the selection of a subset of elements from a sequence by specifying a start and end index. This functionality enhances Python’s capabilities for data manipulation and retrieval, making it a powerful tool for developers working with various data types.
In summary, the colon is a vital component of Python’s syntax that signifies the beginning of a new code block and facilitates slicing operations. Understanding its usage is essential for writing effective and efficient Python code. Mastery of the colon’s functions contributes to better coding practices and a deeper comprehension of Python’s structure and capabilities.
Author Profile
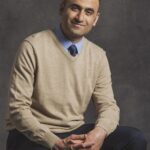
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?