What Does the Double Equal Sign Mean in Python? Understanding Equality in Code
In the world of programming, understanding the nuances of syntax is crucial for writing effective and error-free code. One such nuance that often puzzles beginners is the double equal sign (==) in Python. This seemingly simple operator holds significant importance in the realm of comparisons and logical operations, serving as a fundamental building block in decision-making processes within your code. Whether you’re crafting a complex algorithm or just dabbling in a few lines of Python, grasping what the double equal sign represents can elevate your coding skills and enhance your problem-solving abilities.
At its core, the double equal sign is a comparison operator that checks for equality between two values. Unlike a single equal sign, which is used for assignment, the double equal sign allows programmers to determine if two expressions yield the same result. This distinction is vital, as it helps prevent common pitfalls that can lead to unexpected behaviors in your programs. Understanding how and when to use this operator is essential for anyone looking to write clean, efficient code.
Moreover, the double equal sign plays a pivotal role in control flow statements such as if-else conditions and loops. By leveraging this operator, you can create dynamic and responsive programs that react to user input or changing data conditions. As you delve deeper into Python, you’ll discover the power of the double equal sign
Understanding the Double Equal Sign
In Python, the double equal sign (`==`) is an operator used for comparison. It determines whether two values are equal. This operator plays a crucial role in conditional statements, loops, and any situation where a comparison between values is necessary.
When using the double equal sign, Python evaluates the expressions on either side and returns a Boolean result: `True` if the values are equal, and “ if they are not. This is fundamental for decision-making in programming.
Comparison with Other Operators
To better understand the functionality of the double equal sign, it is helpful to compare it with other related operators:
Operator | Description | Example |
---|---|---|
== | Equality operator, checks if two values are equal | 5 == 5 True |
!= | Inequality operator, checks if two values are not equal | 5 != 3 True |
> | Greater than operator, checks if the left value is greater than the right value | 7 > 5 True |
< | Less than operator, checks if the left value is less than the right value | 3 < 5 True |
>= | Greater than or equal to operator | 5 >= 5 True |
<= | Less than or equal to operator | 3 <= 5 True |
Common Use Cases
The double equal sign is frequently used in various programming constructs. Here are some common scenarios:
- Conditional Statements: It is often used in `if` statements to execute code based on whether a condition is met.
“`python
if x == 10:
print(“x is equal to 10”)
“`
- Loops: In loop conditions, the double equal sign is essential for determining when to continue or stop iterating.
“`python
while user_input == “yes”:
perform an action
“`
- Function Arguments: It can also be utilized to check if function arguments match expected values.
“`python
def check_value(val):
if val == 5:
return “Value is five”
“`
Data Types and Equality
When comparing values using `==`, Python checks for equality in terms of value rather than type. This means that different data types can be compared, but the result will depend on their actual values.
- Example: Comparing an integer and a float:
“`python
print(5 == 5.0) True
“`
However, it is important to note that while `==` checks for value equality, the `is` operator checks for identity, determining if two references point to the same object in memory.
Understanding the double equal sign is critical for writing effective Python code, as it forms the basis of comparison and logic in programming.
Understanding the Double Equal Sign in Python
In Python, the double equal sign `==` is an equality operator used to compare two values. It checks whether the values on either side of the operator are equivalent. The result of this operation is a Boolean value: either `True` or “.
How the Double Equal Sign Works
When using the double equal sign, Python evaluates the data types and values of the operands being compared. The comparison is made based on the following criteria:
- Value Comparison: The operator checks if the values are the same.
- Type Conversion: If the operands are of different types, Python may attempt to convert one or both types for the comparison. For example, comparing an integer and a float may yield a valid comparison.
Here are some examples to illustrate how the double equal sign functions:
“`python
Example 1: Comparing integers
a = 5
b = 5
print(a == b) Output: True
Example 2: Comparing strings
str1 = “hello”
str2 = “hello”
print(str1 == str2) Output: True
Example 3: Comparing different data types
num = 10
text = “10”
print(num == text) Output:
Example 4: Implicit type conversion
num_float = 10.0
print(num == num_float) Output: True
“`
Common Use Cases for the Double Equal Sign
The double equal sign is frequently employed in conditional statements and loops. Here are some typical scenarios:
- If Statements: To execute code based on a condition.
“`python
if a == b:
print(“a is equal to b”)
“`
- While Loops: To continue iterating until a condition is met.
“`python
while user_input == “yes”:
print(“Continuing…”)
“`
- List Comprehensions: To filter items based on equality.
“`python
filtered_list = [x for x in original_list if x == target_value]
“`
Comparison with Other Operators
The double equal sign is often confused with the single equal sign `=`. Here’s a comparative overview:
Operator | Description | Usage Example |
---|---|---|
`==` | Equality operator. Checks for value equality. | `a == b` |
`=` | Assignment operator. Assigns a value to a variable. | `a = 5` |
Best Practices
When using the double equal sign for comparisons, consider the following best practices:
- Use Triple Equals for Identity Checks: In Python, the `is` operator can be used when you want to check if two variables point to the same object in memory.
- Avoid Type Mismatches: Ensure you are comparing similar types unless intentional type conversion is part of your logic.
- Readability: Write clear and understandable comparisons to improve code readability and maintainability.
Employing the double equal sign correctly is crucial for effective conditional logic in Python programming.
Understanding the Double Equal Sign in Python
Dr. Emily Carter (Senior Software Engineer, CodeTech Solutions). The double equal sign in Python, denoted as `==`, is a comparison operator that checks for equality between two values. It evaluates to True if the values are equivalent and otherwise, making it essential for conditional statements and loops in programming.
Michael Thompson (Lead Python Developer, Tech Innovations Inc.). In Python, using `==` allows developers to compare not just primitive data types but also complex objects. This operator is crucial for ensuring that the logic in your code behaves as expected, particularly when dealing with user input or data structures.
Sarah Lee (Computer Science Educator, Future Coders Academy). Understanding the double equal sign is fundamental for anyone learning Python. It is important to distinguish it from the single equal sign `=`, which is used for assignment. Misusing these operators can lead to logical errors that are often difficult to debug.
Frequently Asked Questions (FAQs)
What does double equal sign mean in Python?
The double equal sign (`==`) in Python is a comparison operator used to check if two values are equal. It evaluates to `True` if the values are the same and “ if they are not.
How is the double equal sign different from a single equal sign?
The single equal sign (`=`) is an assignment operator used to assign a value to a variable, while the double equal sign (`==`) is used for comparison to check equality between two values.
Can the double equal sign be used with different data types?
Yes, the double equal sign can compare values of different data types, but the result will depend on the context. Python attempts to convert types if possible, but comparing incompatible types will yield “.
What happens if I use the double equal sign with mutable objects?
Using the double equal sign with mutable objects, such as lists or dictionaries, checks for value equality, meaning it will return `True` if the contents are the same, not necessarily if they are the same object in memory.
Is it possible to override the behavior of the double equal sign in custom classes?
Yes, you can override the behavior of the double equal sign in custom classes by defining the `__eq__` method. This allows you to specify how instances of your class should be compared for equality.
What should I use if I want to check if two variables point to the same object?
To check if two variables point to the same object in memory, you should use the `is` operator. This operator evaluates to `True` if both variables refer to the same object, rather than just checking for value equality.
The double equal sign (==) in Python is a comparison operator used to evaluate whether two values are equal. Unlike the single equal sign (=), which is used for assignment, the double equal sign checks for equality and returns a Boolean value: True if the values are equal and if they are not. This operator is fundamental in control flow statements, such as if conditions, where the outcome of the comparison determines the execution of subsequent code blocks.
It is important to recognize that the double equal sign performs a value comparison, which means it checks if the values on both sides are the same, regardless of their data types. Python also includes type coercion in some cases, allowing comparisons between different data types, such as integers and floats, to be evaluated correctly. However, when comparing complex data types, such as lists or objects, the equality check may involve deeper comparisons based on the contents or attributes of the objects.
In summary, the double equal sign is a crucial operator in Python programming that facilitates equality checks between variables and values. Understanding its function and proper usage is essential for writing effective conditional statements and ensuring logical correctness in code. Developers should also be aware of the nuances of type comparison to avoid unexpected results in their programs.
Author Profile
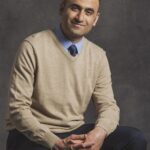
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?