What Does ‘Invalid Syntax’ Mean in Python and How Can You Fix It?
### Introduction
If you’ve ever ventured into the world of Python programming, you may have encountered the dreaded “invalid syntax” error. This seemingly cryptic message can be a source of frustration for beginners and seasoned developers alike, often appearing unexpectedly and halting your code execution. But what does it really mean, and how can you effectively troubleshoot it? Understanding the nuances of this error is crucial for anyone looking to refine their coding skills and enhance their problem-solving abilities in Python. In this article, we’ll demystify the “invalid syntax” error, exploring its common causes and offering practical tips to help you overcome it with confidence.
### Overview
At its core, an “invalid syntax” error in Python indicates that the interpreter has encountered a line of code that it cannot parse. This can stem from a variety of issues, such as missing punctuation, incorrect indentation, or misplaced keywords. Even a small typo can send your code into a tailspin, making it essential to pay close attention to the structure and format of your statements. As you delve deeper into Python, recognizing these pitfalls will not only save you time but also enhance your overall coding proficiency.
Moreover, understanding the context in which this error arises can significantly improve your debugging skills. By familiarizing yourself with common scenarios that lead
Understanding Invalid Syntax in Python
Invalid syntax in Python refers to an error that occurs when the interpreter encounters a line of code that does not conform to the language’s grammatical rules. This can arise from various issues, including typographical errors, incorrect indentation, or the misuse of keywords.
Common causes of invalid syntax errors include:
- Missing punctuation: Forgetting commas, colons, or parentheses can lead to syntax errors.
- Incorrectly formed expressions: Using operators inappropriately or mixing data types incorrectly can trigger this error.
- Mismatched parentheses or quotes: Every opening parenthesis or quote must have a corresponding closing counterpart.
- Improper indentation: Python relies on indentation to define the structure of the code. Inconsistent use can result in syntax errors.
When Python encounters an invalid syntax, it raises a `SyntaxError`, which provides feedback on where the error occurred. The message often includes a description of the problem and a caret (^) indicating the position of the error in the code.
Example Scenarios of Invalid Syntax
To illustrate some common syntax errors, consider the following examples:
Code Snippet | Error Type | Explanation |
---|---|---|
`print(“Hello World”` | Missing parenthesis | The closing parenthesis is missing. |
`if x > 10 print(x)` | Missing colon | A colon is required at the end of the if statement. |
`for i in range(5): | Improper indentation | The code block following the for loop is not indented. |
`def my_function(x) | Missing colon | The colon at the end of the function definition is missing. |
In these examples, correcting the errors involves ensuring that the syntax matches Python’s rules.
Debugging Syntax Errors
To debug syntax errors effectively, follow these strategies:
- Read the error message: Python provides specific details about the error, including the line number and type of syntax issue.
- Check for common mistakes: Review the common causes listed earlier to identify potential issues in your code.
- Use an Integrated Development Environment (IDE): Many IDEs offer syntax highlighting and error checking, which can help you spot syntax errors before running the code.
- Break down complex statements: If a line of code is particularly complex, break it into smaller parts to isolate the error.
By adhering to these practices, you can minimize the occurrence of syntax errors and enhance your coding efficiency.
Understanding Invalid Syntax in Python
Invalid syntax in Python refers to a situation where the code you have written does not conform to the grammatical rules of the Python programming language. This results in a `SyntaxError`, which is Python’s way of indicating that it cannot interpret the code because it is incorrectly structured.
Common Causes of Invalid Syntax
Several common issues can lead to invalid syntax errors:
- Missing Colons: In Python, colons are required at the end of control flow statements (e.g., `if`, `for`, `while`, `def`, and `class`).
python
if condition # Missing colon
print(“Hello”)
- Mismatched Parentheses or Brackets: Every opening parenthesis or bracket must have a corresponding closing one.
python
print(“Hello” # Missing closing parenthesis
- Incorrect Indentation: Python relies on indentation to define code blocks. Misaligned indentation can cause syntax errors.
python
def my_function():
print(“Hello”) # IndentationError due to lack of indentation
- Invalid Variable Names: Variable names must start with a letter or an underscore and cannot contain spaces or special characters (except for underscores).
python
1variable = 5 # SyntaxError due to invalid variable name
- Improperly Closed Strings: Strings must be enclosed in quotes (single or double). Forgetting to close a string can lead to syntax errors.
python
my_string = “Hello # Missing closing quote
How to Identify Syntax Errors
When Python encounters a syntax error, it provides feedback in the form of an error message. Understanding this message is crucial for debugging:
- Error Message: The message typically includes the line number where the error occurred and a description of the issue.
- Caret (^) Symbol: Python often indicates the position of the error using a caret symbol under the problematic part of the code.
Example error message:
File “example.py”, line 2
print(“Hello”
^
SyntaxError: unexpected EOF while parsing
Best Practices to Avoid Syntax Errors
To minimize the risk of encountering syntax errors, consider the following best practices:
- Use an IDE or Code Editor: Integrated Development Environments (IDEs) and code editors often provide syntax highlighting and error detection features.
- Consistent Formatting: Maintain consistent indentation and spacing in your code. Use four spaces per indentation level as per PEP 8 guidelines.
- Readability: Write clear and readable code. Avoid overly complex statements that can lead to confusion.
- Debugging Tools: Use debugging tools and linters to catch errors before running your code. Tools like Pylint or flake8 can highlight syntax issues.
- Test Incrementally: Write and test your code in small increments to catch syntax errors early in the development process.
By adhering to these practices, programmers can significantly reduce the likelihood of encountering invalid syntax errors in their Python code.
Understanding Invalid Syntax in Python: Expert Insights
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Invalid syntax in Python indicates that the code structure does not conform to the language’s grammatical rules. This can occur due to missing punctuation, incorrect indentation, or misuse of keywords, leading to runtime errors that prevent the execution of the script.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When a Python interpreter encounters invalid syntax, it halts execution and raises a SyntaxError. Understanding the specific location and context of the error is crucial for debugging, as it often points to the line where the mistake originated, even if the actual issue lies earlier in the code.”
Sarah Johnson (Python Educator, LearnPython Academy). “For beginners, invalid syntax can be particularly frustrating. It is essential to adopt good coding practices, such as consistently using proper indentation and commenting code, to minimize syntax errors. Tools like linters can also help identify potential issues before running the code.”
Frequently Asked Questions (FAQs)
What does invalid syntax mean in Python?
Invalid syntax in Python indicates that the code contains an error that prevents it from being parsed correctly. This often occurs due to typos, incorrect indentation, or misuse of Python’s syntax rules.
What are common causes of invalid syntax errors?
Common causes include missing colons, unmatched parentheses or brackets, incorrect indentation, and using reserved keywords incorrectly. These mistakes disrupt the expected structure of Python code.
How can I identify where the invalid syntax error occurs?
Python provides an error message that typically includes the line number where the syntax error was detected. Reviewing the code around that line can help identify the specific issue.
Can invalid syntax errors occur in multi-line statements?
Yes, invalid syntax errors can occur in multi-line statements if the continuation character (`\`) is missing or if the parentheses, brackets, or braces are not properly closed.
Is there a way to prevent invalid syntax errors in Python?
To prevent invalid syntax errors, consistently follow Python’s syntax rules, use an integrated development environment (IDE) with syntax highlighting, and regularly run code to catch errors early.
What should I do if I encounter an invalid syntax error?
If you encounter an invalid syntax error, carefully read the error message, check the indicated line for common mistakes, and consult the Python documentation for clarification on syntax rules.
In Python, the term “invalid syntax” refers to errors that occur when the interpreter encounters code that does not conform to the language’s grammatical rules. This error message indicates that there is a mistake in the structure of the code, which prevents the interpreter from executing it. Common causes of invalid syntax include missing colons, incorrect indentation, unbalanced parentheses, and misuse of keywords or operators.
Understanding the implications of an invalid syntax error is crucial for effective debugging and code development. When a programmer receives this error, it serves as a prompt to review the specific line of code indicated in the error message. By carefully examining the syntax, developers can identify and correct the issue, ensuring that their code adheres to Python’s syntax rules.
Key takeaways include the importance of adhering to proper syntax conventions in Python to avoid such errors. Utilizing tools like linters and code formatters can significantly reduce the likelihood of syntax errors. Additionally, practicing good coding habits, such as consistent indentation and thorough code reviews, can help maintain code quality and prevent invalid syntax issues from arising in the first place.
Author Profile
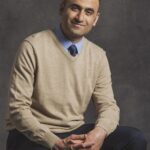
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?