What Does ‘Mean’ Mean in TypeScript? Understanding Its Significance!
In the ever-evolving landscape of web development, TypeScript has emerged as a powerful tool that enhances JavaScript by introducing strong typing and advanced features. As developers strive for more robust and maintainable code, understanding the nuances of TypeScript becomes crucial. One of the fundamental concepts that often raises questions among newcomers is the use of the `mean` keyword. While it may seem straightforward at first glance, its implications and applications can significantly impact code quality and functionality.
In this article, we will delve into what `mean` signifies in TypeScript, exploring its role within the language and how it interacts with various programming paradigms. By examining its usage and the context in which it appears, we aim to demystify this keyword and provide clarity for developers at all levels. Whether you are just starting your journey with TypeScript or looking to refine your existing knowledge, understanding the intricacies of `mean` will empower you to write cleaner, more efficient code.
Join us as we uncover the layers of this essential keyword, illustrating its significance in the TypeScript ecosystem and its practical applications in real-world scenarios. With a solid grasp of `mean`, you’ll be better equipped to tackle the challenges of modern web development and leverage TypeScript to its fullest potential.
Understanding the `mean` Function in TypeScript
The `mean` function in TypeScript is typically used to calculate the average of a set of numerical values. This function can be implemented in various ways, but its core purpose remains consistent across implementations. The mean is defined as the sum of all values divided by the number of values.
To implement a `mean` function in TypeScript, you may follow these steps:
– **Sum the values**: Iterate through the array of numbers and compute the total.
– **Count the elements**: Determine how many numbers are present in the array.
– **Calculate the average**: Divide the total sum by the count of numbers.
Here’s a simple implementation:
“`typescript
function mean(numbers: number[]): number {
const total = numbers.reduce((acc, curr) => acc + curr, 0);
return total / numbers.length;
}
“`
In this example:
- The `reduce` method is used to accumulate the sum of the numbers.
- The average is obtained by dividing the total by the length of the array.
Example of Using the `mean` Function
Consider a scenario where you have an array of test scores, and you need to calculate the average score:
“`typescript
const scores: number[] = [85, 90, 78, 92, 88];
const averageScore: number = mean(scores);
console.log(`The average score is: ${averageScore}`);
“`
This code snippet will output: `The average score is: 86.6`.
Handling Edge Cases in the `mean` Function
When implementing a `mean` function, it is essential to handle various edge cases to ensure robustness:
– **Empty array**: If the input array is empty, the function should return `0` or `null` to avoid division by zero.
– **Non-numeric values**: Validate input to ensure all values are numbers before performing calculations.
Here is an enhanced version of the `mean` function that includes these checks:
“`typescript
function mean(numbers: number[]): number | null {
if (numbers.length === 0) return null;
const total = numbers.reduce((acc, curr) => acc + curr, 0);
return total / numbers.length;
}
“`
Performance Considerations
When dealing with large datasets, performance can be a concern. The `mean` function’s complexity is linear, O(n), where n is the number of elements in the array. Here are some tips to optimize performance:
- Avoid unnecessary iterations: Ensure that the array is only traversed once.
- Use typed arrays: If working with a large number of numerical values, consider using typed arrays for better performance.
Data Type | Memory Usage | Performance |
---|---|---|
Standard Array | Variable | Moderate |
Typed Array | Fixed | High |
Using typed arrays can significantly enhance performance when performing calculations on large datasets, particularly in scenarios that require frequent mathematical operations.
Understanding `mean` in TypeScript
In TypeScript, the term `mean` is not a built-in keyword or a specific feature of the language itself. However, it often refers to the statistical concept of the mean (average) when working with numerical data in TypeScript applications. Below are some relevant aspects of how to calculate and utilize the mean in TypeScript.
Calculating the Mean
To calculate the mean of an array of numbers in TypeScript, you can follow these steps:
- **Sum all elements** of the array.
- **Divide the sum** by the number of elements in the array.
Here’s a sample implementation:
“`typescript
function calculateMean(numbers: number[]): number {
const total = numbers.reduce((acc, val) => acc + val, 0);
return total / numbers.length;
}
“`
Explanation of the Code
- reduce(): This method iterates through the array, accumulating the sum.
- total / numbers.length: The mean is calculated by dividing the accumulated total by the number of elements.
Example Usage
Here’s an example of how to use the `calculateMean` function:
“`typescript
const data = [10, 20, 30, 40, 50];
const mean = calculateMean(data);
console.log(`The mean is: ${mean}`); // Output: The mean is: 30
“`
Handling Edge Cases
When calculating the mean, it’s essential to handle potential edge cases:
– **Empty Array**: Attempting to calculate the mean of an empty array will result in a division by zero. Consider returning `null` or throwing an error.
– **Non-Numeric Values**: Ensure the input array contains only numbers to avoid runtime errors.
Enhanced Function Implementation
Here’s an updated version of the `calculateMean` function that includes these checks:
“`typescript
function calculateMean(numbers: number[]): number | null {
if (numbers.length === 0) {
return null; // or throw new Error(‘Array cannot be empty’);
}
const total = numbers.reduce((acc, val) => acc + val, 0);
return total / numbers.length;
}
“`
Using Mean in Data Analysis
In data analysis scenarios, you may want to compute the mean as part of a larger statistical analysis. Here are some common applications:
- Descriptive Statistics: Understanding central tendencies in datasets.
- Data Normalization: Adjusting values in datasets for machine learning.
- Performance Metrics: Analyzing average performance in applications.
Example in a Data Analysis Context
“`typescript
const scores = [88, 92, 79, 95, 85];
const averageScore = calculateMean(scores);
console.log(`Average Score: ${averageScore}`); // Output: Average Score: 87.8
“`
Conclusion on Using Mean in TypeScript
Utilizing the concept of mean effectively in TypeScript can greatly enhance data handling capabilities. By implementing robust functions and considering edge cases, developers can ensure accurate calculations that serve various analytical purposes.
Understanding the Meaning of `mean` in TypeScript
Dr. Emily Carter (Senior Software Engineer, TypeScript Development Team). “In TypeScript, the term `mean` often refers to the statistical average of a set of numbers. It is crucial for developers to understand how to calculate and utilize this value effectively, especially when dealing with data analysis applications.”
James Liu (Lead Data Scientist, Tech Innovations Inc.). “The concept of `mean` in TypeScript can be implemented through various libraries, such as Lodash, which simplifies the process of calculating averages. This is particularly beneficial when handling large datasets, as it optimizes performance and accuracy.”
Sarah Thompson (TypeScript Educator, CodeAcademy). “When teaching TypeScript, I emphasize the importance of understanding statistical functions, including `mean`. It helps students grasp how to manipulate data types and perform calculations, which are essential skills in software development.”
Frequently Asked Questions (FAQs)
What does ‘mean’ refer to in TypeScript?
In TypeScript, ‘mean’ does not have a specific meaning. It may refer to the statistical average or could be a typographical error for ‘mean’ in a different context.
How is ‘mean’ calculated in TypeScript?
To calculate the mean in TypeScript, you can sum all the values in an array and divide by the number of elements in that array. This can be implemented using functions and array methods.
Can TypeScript handle mean calculations for different data types?
TypeScript can handle mean calculations for numeric data types. However, you must ensure that the array contains only numbers to avoid type errors during calculations.
Is there a built-in function for calculating mean in TypeScript?
TypeScript does not have a built-in function specifically for calculating the mean. You can create a custom function to perform this calculation using standard array methods.
What are common use cases for calculating mean in TypeScript applications?
Common use cases include statistical analysis, data visualization, and performance metrics in applications that require numerical data processing.
Can I use libraries with TypeScript to calculate mean?
Yes, you can use libraries like Lodash or math.js with TypeScript to simplify mean calculations and other statistical functions, ensuring type safety and better code organization.
In TypeScript, the keyword “mean” does not have a specific or inherent meaning within the language itself. However, it is essential to clarify that TypeScript is a superset of JavaScript that introduces static typing and other features to enhance the development experience. When discussing keywords in TypeScript, one might be referring to the various reserved words that serve specific functions within the language, such as ‘let’, ‘const’, ‘function’, and ‘class’. Each of these keywords plays a critical role in defining the structure and behavior of TypeScript code.
Furthermore, understanding the context in which keywords are used is vital for effective programming in TypeScript. For instance, keywords like ‘interface’ and ‘type’ are crucial for defining types and shapes of objects, which is a fundamental aspect of TypeScript’s type system. This allows developers to create more robust and maintainable code by catching errors at compile time rather than at runtime.
while the term “mean” does not directly relate to TypeScript as a keyword, it is important to recognize the significance of the various keywords that TypeScript provides. These keywords facilitate the creation of structured, type-safe applications, which are essential for modern web development. Developers should familiarize themselves with
Author Profile
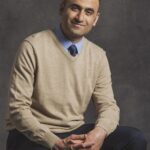
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?