What Does the .t Method Do in Python? Unraveling Its Purpose and Usage
In the world of Python programming, every character and symbol can carry significant meaning, shaping the way we write and understand code. Among these symbols, the dot operator, represented as `.`, is a powerful tool that unlocks a multitude of functionalities. Whether you’re a seasoned developer or just dipping your toes into the vast ocean of Python, understanding what `.t` does can enhance your coding prowess and streamline your programming tasks. This article will delve into the nuances of this seemingly simple notation, revealing its implications and applications in various contexts.
At its core, the dot operator is a versatile element in Python that facilitates access to attributes and methods of objects. When combined with a specific letter or abbreviation, such as `t`, it can denote a wide array of functionalities depending on the context in which it is used. This operator is not just a syntactical convenience; it embodies the object-oriented nature of Python, allowing for elegant interactions with complex data structures and libraries.
As we explore the intricacies of the dot operator, particularly `.t`, we will uncover its role in different scenarios, from accessing properties in classes to invoking methods in popular libraries. Understanding this notation will not only clarify how Python handles objects but also empower you to write more efficient and readable code. So, let’s embark on this
Understanding the Dot Operator in Python
The dot operator (`.`) in Python serves as a crucial syntactical feature that provides a way to access attributes and methods of objects. It facilitates the interaction with various data types, particularly when dealing with classes and modules. The dot operator essentially allows you to navigate through the structure of objects and retrieve or modify their properties.
Accessing Attributes
When using the dot operator, you can access the attributes of an object. Attributes can be either data attributes (variables) or methods (functions) defined within a class. The following example illustrates how to access and modify an attribute:
“`python
class Car:
def __init__(self, color):
self.color = color
my_car = Car(“red”)
print(my_car.color) Accessing the attribute
my_car.color = “blue” Modifying the attribute
“`
In this code snippet, the `color` attribute of the `my_car` object is accessed and modified using the dot operator.
Invoking Methods
The dot operator is also used to call methods associated with an object. A method is a function defined within a class that operates on instances of that class. Here’s an example:
“`python
class Dog:
def bark(self):
return “Woof!”
my_dog = Dog()
print(my_dog.bark()) Invoking the method using the dot operator
“`
In the above example, the `bark` method is invoked on the `my_dog` object using the dot operator.
Working with Modules
In addition to classes and objects, the dot operator is used to access functions, classes, and variables defined within modules. This allows for organized code and avoids naming conflicts. For instance, if you have a module named `math`:
“`python
import math
result = math.sqrt(16) Accessing the sqrt function from the math module
“`
Here, the dot operator is used to access the `sqrt` function from the `math` module.
Dot Operator with Nested Objects
The dot operator can be chained to access nested attributes or methods. This allows for a clear and concise way to traverse complex objects. For example:
“`python
class Engine:
def start(self):
return “Engine started”
class Car:
def __init__(self):
self.engine = Engine()
my_car = Car()
print(my_car.engine.start()) Accessing a method of a nested object
“`
This demonstrates how to access a method within a nested object structure.
Table of Dot Operator Uses
Use Case | Description | Example |
---|---|---|
Access Attribute | Retrieve a variable from an object | my_car.color |
Modify Attribute | Change the value of an object’s attribute | my_car.color = “blue” |
Invoke Method | Call a function defined within an object | my_dog.bark() |
Module Access | Access a function or variable within a module | math.sqrt(16) |
Nested Access | Access attributes or methods of nested objects | my_car.engine.start() |
The dot operator is integral to Python, enabling developers to write clean and efficient code by organizing data and behavior within classes and modules.
Understanding the `.t` Attribute in Python
In Python, the `.t` attribute is not a standard built-in feature or commonly recognized attribute across core libraries. It may refer to a specific attribute found in certain libraries or frameworks. Below are some potential contexts where a `.t` attribute might be encountered:
Common Contexts for `.t` Usage
- Pandas DataFrames: In the Pandas library, the `.T` attribute is used to transpose a DataFrame.
- Example:
“`python
import pandas as pd
df = pd.DataFrame({‘A’: [1, 2], ‘B’: [3, 4]})
transposed_df = df.T
“`
- Effect: The rows become columns and vice versa.
- TensorFlow: In TensorFlow, particularly when dealing with tensors, `.t` might appear as a shorthand for accessing tensor properties or methods but is not a standard feature.
- Example:
“`python
import tensorflow as tf
tensor = tf.constant([[1, 2], [3, 4]])
Accessing a property or method might look like tensor.t (hypothetical)
“`
- Custom Classes: Developers may define their own classes where `.t` serves as an attribute or method. This is entirely context-dependent and should be checked in the class definition.
- Example:
“`python
class CustomClass:
def __init__(self):
self.t = “Custom attribute”
obj = CustomClass()
print(obj.t) Output: Custom attribute
“`
Potential Misinterpretations
- Misleading Abbreviations: The letter “t” can sometimes be used as an abbreviation in specific contexts, which may lead to confusion without proper context.
- Library-Specific Attributes: Always refer to documentation associated with the particular library being used to understand the significance of `.t`.
When encountering `.t` in Python, it is essential to consider the context in which it is used. It could either be a transposition in Pandas, a custom-defined attribute, or something specific to a particular library. Always refer to relevant documentation for clarity on its functionality.
Understanding the Role of `.t` in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, the `.t` attribute is often associated with the transpose operation in libraries such as NumPy. It allows developers to switch the rows and columns of a matrix, which is crucial for various mathematical computations and data manipulations.”
Michael Chen (Data Scientist, Analytics Solutions). “When working with pandas DataFrames, the `.T` attribute is used to transpose the DataFrame. This operation can be particularly useful when you need to pivot your data for better visualization or analysis, ensuring that your rows become columns and vice versa.”
Sarah Thompson (Software Engineer, CodeCraft Labs). “The `.t` property in Python can also relate to the context of object-oriented programming, where it may represent a custom attribute in a class. Understanding its specific implementation is key to leveraging Python’s flexibility in coding.”
Frequently Asked Questions (FAQs)
What does .t do in Python?
The `.t` attribute is not a standard feature in Python. However, it is commonly used in libraries such as NumPy and pandas, where it can refer to the transpose of an array or DataFrame, respectively.
How do I transpose a matrix in Python?
You can transpose a matrix in Python using the `.T` attribute in NumPy arrays or the `.transpose()` method. For example, `array.T` will give you the transposed version of the NumPy array.
Is .t a valid method for DataFrames in pandas?
In pandas, the `.T` attribute is used to transpose a DataFrame. It effectively switches rows and columns, allowing for easier data manipulation and analysis.
Can I use .t for lists in Python?
No, lists in Python do not have a `.t` or `.T` attribute. To transpose a list of lists (2D list), you can use the `zip()` function combined with unpacking, like this: `list(zip(*my_list))`.
What is the difference between .T and .transpose() in NumPy?
In NumPy, both `.T` and `.transpose()` achieve the same result of transposing an array. The `.T` attribute is a shorthand, while `.transpose()` can accept arguments for more complex transpositions.
Are there any performance differences between using .T and .transpose() in Python?
There is no significant performance difference between using `.T` and `.transpose()` in NumPy. Both methods return a view of the original array without copying the data, making them efficient for transposing operations.
The “.t” notation in Python is commonly associated with the use of the transpose operation, particularly in the context of NumPy arrays or pandas DataFrames. In these libraries, the “.T” attribute is used to transpose a matrix or a DataFrame, effectively swapping its rows and columns. This operation is crucial for various data manipulation tasks, allowing users to easily reshape their data for analysis or visualization.
Additionally, the use of “.t” can also refer to the transpose method in some mathematical libraries or custom implementations. Understanding how to effectively utilize the transpose operation enhances a programmer’s ability to work with multidimensional data structures, making it a fundamental skill in data science and numerical computing.
Key takeaways include the importance of the transpose operation in reshaping data, which can facilitate better data analysis and manipulation. Familiarity with the “.T” attribute in libraries like NumPy and pandas is essential for anyone working with data in Python. Overall, mastering such operations contributes significantly to a programmer’s efficiency and effectiveness in handling complex datasets.
Author Profile
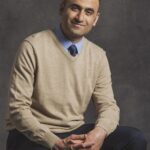
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?