What Does ‘t’ Do in Python? Understanding Its Role and Usage
### Introduction
In the world of Python programming, the language’s simplicity and versatility have made it a favorite among developers, data scientists, and hobbyists alike. As you delve deeper into Python, you may encounter various symbols, keywords, and functions that can significantly enhance your coding experience. One such intriguing element is the letter “t.” While it may seem innocuous at first glance, understanding what “t” represents in the context of Python can unlock a new level of clarity and efficiency in your coding endeavors. Whether you’re a seasoned programmer or just starting your journey, grasping the nuances of this seemingly simple character can elevate your Python skills to new heights.
At its core, “t” can serve multiple purposes in Python, depending on the context in which it is used. It may represent a variable, a parameter, or even a part of a function name. This versatility allows programmers to utilize “t” in various scenarios, making it a valuable component of their coding toolkit. As you explore the intricacies of Python, you’ll discover how this unassuming letter can play a pivotal role in enhancing code readability and functionality.
Moreover, the significance of “t” extends beyond mere representation; it often embodies the principles of Pythonic programming. By understanding how to effectively use “t,”
Understanding the Role of `t` in Python
In Python, the letter `t` can serve various purposes depending on the context in which it is used. It is not a reserved keyword or function but can represent different entities such as variables, function parameters, or even components in data structures. Below are some common uses of `t` in Python programming.
Common Uses of `t`
- Variable Name: `t` is often utilized as a variable name in loops or functions, particularly in mathematical or scientific computations. For example:
python
for t in range(10):
print(t)
- Time Representation: In time-related functions or libraries, `t` frequently denotes time. For instance, in simulations or animations, `t` might represent the elapsed time.
- Tuple: In some cases, `t` could be shorthand for a tuple. When unpacking values from a tuple, `t` might be used to temporarily hold the tuple:
python
t = (1, 2, 3)
a, b, c = t
Contextual Examples of `t`
The context where `t` is employed significantly influences its meaning. Below are examples showcasing how `t` can be utilized in various scenarios:
Context | Example | Description |
---|---|---|
Loop Variable | for t in range(5): |
Iterates through the numbers 0 to 4. |
Time in Simulations | t += delta_time |
Increments `t` by a small time step. |
Tuple Unpacking | t = (x, y); a, b = t |
Unpacks the values from the tuple `t` into variables `a` and `b`. |
Best Practices When Using `t`
When using `t` as a variable name or in any context, it is essential to consider the following best practices:
- Clarity: While using single-letter variables like `t` is acceptable in short functions or loops, ensure that their purpose is clear to anyone reading the code. Descriptive names are often preferred for better readability.
- Scope Awareness: Be mindful of the scope of `t`. Using `t` in nested functions or loops can lead to confusion if it is reused in a different context.
- Consistent Usage: If `t` represents time in your code, maintain consistency in its usage throughout the program to avoid misunderstandings.
By adhering to these practices, the use of `t` can contribute positively to the clarity and functionality of your Python code.
Understanding the `t` Function in Python
In Python, the term `t` does not refer to a specific built-in function or keyword. However, it can represent various contexts depending on how it is utilized within a script or framework. Below are several interpretations of `t` in Python programming:
Common Interpretations of `t` in Python
- Variable Naming:
- `t` is often used as a variable name, particularly in mathematical computations, timing functions, or algorithms.
- Example:
python
t = 10 # Represents a time variable
- Library Functions:
- In certain libraries, `t` may be a shorthand for specific functions. For instance, in data manipulation libraries or frameworks, `t` could be a method or an object.
- String Manipulation:
- In string formatting, `t` could be part of a formatted string where it stands for a placeholder.
- Example:
python
name = “Alice”
greeting = f”Hello, {name}. Welcome to {t}!” # Assuming t is defined earlier
Examples in Context
Context | Example Usage |
---|---|
Variable | `t = 5` |
Time Calculation | `from time import time; t = time()` |
Placeholder in Format | `print(f”Current time: {t}”)` |
Using `t` in Libraries
In various libraries, `t` can assume different roles:
- Matplotlib: In plotting functions, `t` can represent time in time series data.
- NumPy: It may denote an array or a specific dimension in matrix operations.
For instance, when plotting with Matplotlib:
python
import matplotlib.pyplot as plt
import numpy as np
t = np.linspace(0, 10, 100) # Creating a time variable
y = np.sin(t) # Sine function of time
plt.plot(t, y)
plt.show()
Best Practices for Using `t`
- Descriptive Naming: Use descriptive variable names instead of single letters unless used in a small scope, such as loops or short functions.
- Contextual Clarity: Ensure that the context of `t` is clear, especially in collaborative projects or scripts that may be revisited later.
the Usage of `t`
While `t` may not be a specific Python function, its usage as a variable or shorthand in various contexts is common in coding practices. Understanding its application can enhance clarity and efficiency in Python programming.
Understanding the Role of ‘t’ in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, the variable ‘t’ is often used as a shorthand for ‘time’ or ‘test’, especially in functions or loops where brevity enhances readability. It serves as a placeholder that can represent various data types, depending on the context of its use.”
Michael Chen (Lead Software Engineer, CodeCrafters). “The letter ‘t’ is frequently employed in Python scripts to denote temporary variables or iterators. Its simplicity allows developers to focus on the logic of their code without unnecessary complexity, making it a common convention in many coding styles.”
Sarah Patel (Python Educator and Author). “When teaching Python, I often highlight the use of ‘t’ in examples to illustrate how variable naming can impact code clarity. While ‘t’ is convenient, it’s essential to balance brevity with descriptive naming to ensure that code remains understandable for others.”
Frequently Asked Questions (FAQs)
What does the ‘t’ variable represent in Python?
The ‘t’ variable in Python does not have a specific meaning; it is often used as a generic variable name in examples or code snippets. Its purpose depends on the context in which it is used, such as representing time, a temporary value, or an element in a loop.
What is the significance of ‘t’ in Python threading?
In Python’s threading module, ‘t’ is commonly used as a shorthand for a thread object. Developers often name thread instances with ‘t’ to signify that the variable represents a thread, making the code easier to read and understand.
How is ‘t’ used in time-related functions in Python?
In time-related functions, ‘t’ can denote time intervals or timestamps. For instance, ‘t’ might be used to hold the current time returned by functions like `time.time()` or `time.perf_counter()` for performance measurements.
What does ‘t’ signify in Python’s mathematical operations?
In mathematical operations, ‘t’ can serve as a placeholder for a variable, particularly in equations or functions. It is often used in mathematical modeling or simulations to represent time or another independent variable.
Is ‘t’ a built-in function or keyword in Python?
No, ‘t’ is neither a built-in function nor a reserved keyword in Python. It is simply a variable name that can be used freely by programmers, and its meaning is determined by its usage in the code.
Can ‘t’ be used as an identifier for functions or classes in Python?
Yes, ‘t’ can be used as an identifier for functions or classes in Python. However, it is advisable to use more descriptive names to improve code readability and maintainability.
The keyword ‘t’ in Python does not have a specific, predefined function or meaning within the language itself. Instead, it is often used as a variable name or identifier in various contexts, such as in loops, functions, or mathematical expressions. The choice of ‘t’ as a variable name is typically arbitrary and may be chosen for its brevity, especially in mathematical or algorithmic implementations where the context is clear. However, it is essential to ensure that variable names are meaningful and descriptive to enhance code readability and maintainability.
In the realm of programming, the use of short variable names like ‘t’ can be appropriate in certain situations, such as when dealing with time-related calculations, iterations, or temporary storage. For instance, ‘t’ is commonly used to represent time in physics simulations or algorithms that require tracking time intervals. Nonetheless, developers should exercise caution and avoid overusing such abbreviations to prevent confusion, especially in larger codebases where clarity is paramount.
In summary, while ‘t’ does not hold a specific function in Python, its usage as a variable name can be contextually relevant. Developers should prioritize meaningful naming conventions to improve code clarity. Ultimately, the effective use of variable names, including ‘t’, contributes
Author Profile
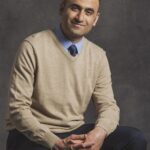
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?