What Does ‘t’ Mean in Python? Unraveling the Mystery Behind the Letter!
In the realm of Python programming, every character and symbol carries significance, often unlocking new functionalities or enhancing code readability. Among these characters, the letter “t” can appear in various contexts, each with its own implications and meanings. Whether you’re a seasoned developer or a newcomer to the world of Python, understanding these nuances can elevate your coding skills and deepen your comprehension of the language’s syntax and semantics.
At first glance, “t” might seem like just another letter, but it plays a pivotal role in different scenarios, from variable naming conventions to specific library functions. For instance, in the context of data structures, “t” could represent a tuple, a fundamental building block for storing collections of items. Alternatively, in certain libraries or frameworks, “t” may denote a specific type or function that is integral to the operation of the code.
As you delve deeper into the intricacies of Python, you’ll discover that the meaning of “t” is often context-dependent, shaped by the surrounding code and the conventions of the Python community. This exploration will not only clarify the role of “t” but also enhance your overall understanding of Python’s versatile nature, empowering you to write more efficient and effective code. So, let’s embark on this journey to uncover the many dimensions
Understanding the `t` Variable in Python
In Python, the variable `t` can represent various concepts depending on the context in which it is used. It is not a reserved keyword or a built-in function but rather a common variable name that developers might employ for different purposes. Below are some common usages of the `t` variable in Python programming.
Common Usages of `t`
- Time Representation: Often, `t` is used to denote time in simulations, animations, or calculations involving time intervals. For example, in physics simulations, `t` might represent time in seconds.
- Loop Iterations: In loops, `t` may serve as a counter or an index, especially in small scripts or examples. Developers might use `t` as a shorthand for “time step” or as a temporary variable.
- Temporary Variable: In functions or algorithms, `t` is frequently used as a temporary placeholder for computations. For instance, when performing calculations involving multiple steps, `t` can hold intermediate results.
Example Usages
Here are some examples illustrating different contexts in which `t` may be utilized:
“`python
Example 1: Using `t` as a time variable
import time
start_time = time.time()
for t in range(5):
time.sleep(1) Simulating a task that takes time
print(f”Elapsed time: {time.time() – start_time:.2f} seconds”)
Example 2: Using `t` in a mathematical function
def calculate_area(radius):
t = 3.14 Approximation of π
return t * radius ** 2
area = calculate_area(5)
print(f”Area of the circle: {area}”)
“`
Best Practices for Variable Naming
While using `t` as a variable name can be convenient, it is essential to follow best practices in variable naming to maintain code readability. Here are some guidelines:
- Descriptive Names: Instead of using single letters like `t`, opt for more descriptive names that reflect the variable’s purpose, such as `time_elapsed` or `iteration_count`.
- Consistency: Maintain consistent naming conventions throughout your codebase. If you use `t` in one function, consider whether it makes sense to use it elsewhere.
- Avoid Overuse: Reserve single-letter variable names for short-lived variables within small scopes, such as loop counters or temporary calculations.
Variable Name | Description |
---|---|
t | Often used for time or as a temporary placeholder |
time_elapsed | Descriptive variable for tracking time |
counter | Commonly used for loop iterations |
By understanding the context and application of `t` in Python, developers can make informed decisions about variable naming and usage, ultimately improving code clarity and maintainability.
Understanding the `t` Variable in Python
In Python, the term `t` can refer to different contexts depending on its usage within the code. Here are the primary interpretations:
Common Contexts for `t` in Python
– **Variable Names**:
- `t` is frequently used as a variable name. As a convention, it often represents time in simulations or iterations, particularly in physics or mathematical computations.
– **Type Hinting**:
- In type hinting, `t` may refer to a generic type variable. For instance, in the following code, `T` is a common convention, but `t` could be used similarly:
“`python
from typing import TypeVar
T = TypeVar(‘T’)
def process_item(item: T) -> T:
return item
“`
- Tuple Unpacking:
- In tuple unpacking, `t` may represent a tuple:
“`python
t = (1, 2, 3)
a, b, c = t
“`
Example Scenarios Using `t`
– **Using `t` in Time Calculations**:
“`python
for t in range(10):
print(f”Current time step: {t}”)
“`
– **As a Generic Type**:
“`python
from typing import List, TypeVar
T = TypeVar(‘T’)
def get_first_item(items: List[T]) -> T:
return items[0]
“`
- Tuple Manipulation:
“`python
t = (10, 20, 30)
x, y, z = t
print(f”x: {x}, y: {y}, z: {z}”)
“`
Best Practices for Using `t`
- Descriptive Naming: While `t` is concise, more descriptive names (e.g., `time_step`, `item`, `data_tuple`) improve code readability.
- Context Matters: Ensure that the use of `t` fits the context of the code, preventing confusion for anyone reading or maintaining it later.
Conclusion of Usage
The variable `t` in Python is versatile, functioning as a simple name for variables, a placeholder for generic types, or part of tuple operations. When employing `t`, consider clarity and context for effective communication within your code.
Understanding the Significance of ‘t’ in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, the letter ‘t’ can represent various elements depending on the context. For instance, in data structures, ‘t’ might denote a tuple or a variable name, especially in algorithms where clarity is paramount.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “When discussing Python, ‘t’ often refers to a time-related variable in programming scenarios, particularly in simulations or time series analysis. Understanding its context is crucial for accurate data manipulation.”
Laura Chen (Python Educator, Digital Learning Academy). “In educational settings, ‘t’ frequently symbolizes a temporary variable in examples, especially in teaching loops or functions. It is essential for learners to grasp the flexibility of variable naming in Python.”
Frequently Asked Questions (FAQs)
What does the ‘t’ represent in Python type hints?
The ‘t’ in Python type hints typically represents a type variable. It is often used in generic programming to indicate that a function or class can operate on different types while maintaining type safety.
What is the significance of ‘t’ in Python’s typing module?
In the context of the typing module, ‘t’ is commonly used as a placeholder for a specific type. This allows for the creation of generic classes and functions that can accept or return different types, enhancing code reusability.
How is ‘t’ used in function annotations?
The ‘t’ can be used in function annotations to specify that the function can accept parameters of various types or return a value of a certain type. This improves code clarity and helps with static type checking.
Can ‘t’ be used to create custom types in Python?
Yes, ‘t’ can be used in conjunction with the TypeVar function from the typing module to create custom types. This allows developers to define generic types that can be reused across different functions and classes.
Is ‘t’ a built-in keyword in Python?
No, ‘t’ is not a built-in keyword in Python. It is simply a convention used by developers to denote type variables, but it can be named anything as long as it follows Python’s variable naming rules.
Are there any best practices for using ‘t’ in Python?
Best practices include using descriptive names for type variables instead of single letters when clarity is needed, and ensuring that type hints are consistent throughout the codebase to facilitate better understanding and maintenance.
The letter ‘t’ in Python can refer to various concepts depending on the context in which it is used. It is not a standalone keyword in the Python programming language but may appear in variable names, function names, or even as part of specific libraries or frameworks. For instance, in the context of data manipulation, ‘t’ might represent a variable used to store a temporary value or a transformation of data. Understanding its meaning requires examining the surrounding code and the specific application being discussed.
Additionally, ‘t’ can also be associated with certain libraries or functions where it may serve as an abbreviation for terms such as ‘transpose’ in matrix operations or ‘type’ in type-checking scenarios. In some cases, it may be used as a shorthand notation in mathematical expressions or algorithms implemented in Python. Therefore, its significance is highly contextual and varies across different programming tasks.
In summary, while ‘t’ is not a defined keyword in Python, its usage is prevalent in various programming scenarios. Developers must pay attention to the context to accurately interpret its meaning. This highlights the importance of clear naming conventions and documentation in programming to enhance code readability and maintainability.
Author Profile
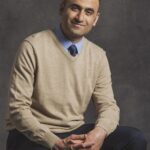
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?