What Do Values Do in Python? Understanding Their Role and Importance
In the world of Python programming, understanding the concept of values is crucial for both novice and seasoned developers alike. Values serve as the building blocks of data manipulation, influencing how we store, process, and interact with information in our code. Whether you’re crafting a simple script or developing complex applications, grasping the role of values can significantly enhance your programming prowess. Join us as we delve into the intricate landscape of values in Python, exploring their types, behaviors, and the pivotal role they play in effective coding practices.
At its core, a value in Python represents a piece of data that can be assigned to variables, passed to functions, and manipulated through various operations. These values come in different forms, including integers, strings, floats, and more, each with its unique characteristics and use cases. Understanding how these values interact with one another is essential for writing efficient and error-free code. Moreover, Python’s dynamic typing system allows for a flexible approach to handling values, enabling developers to write more adaptable and robust programs.
As we navigate through the nuances of values in Python, we will uncover how they influence variable assignment, control flow, and data structures. This exploration will not only clarify the foundational aspects of values but also highlight their significance in the broader context of Python programming. Whether you’re looking
Understanding Values in Python
In Python, a value is a fundamental piece of data that can be assigned to a variable. Values can take various forms, depending on their types, which include integers, floats, strings, lists, tuples, dictionaries, sets, and more. Each type of value has distinct characteristics and behaviors that dictate how they can be utilized within a program.
Types of Values
The most common data types in Python and their associated values include:
- Integers: Whole numbers, e.g., `42`, `-7`.
- Floats: Decimal numbers, e.g., `3.14`, `-0.001`.
- Strings: Sequences of characters, e.g., `”Hello, world!”`, `’Python’`.
- Booleans: Represent truth values, either `True` or “.
- Lists: Ordered collections of items, e.g., `[1, 2, 3]`, `[“apple”, “banana”]`.
- Tuples: Immutable ordered collections, e.g., `(1, 2, 3)`, `(“a”, “b”)`.
- Dictionaries: Key-value pairs, e.g., `{“name”: “Alice”, “age”: 25}`.
- Sets: Unordered collections of unique items, e.g., `{1, 2, 3}`.
Creating and Using Values
Values in Python can be assigned to variables, which act as references to those values. For instance:
“`python
number = 10 An integer value
pi = 3.14 A float value
greeting = “Hello” A string value
“`
These variables can then be used in expressions, passed to functions, or manipulated in various ways.
Immutability and Mutability
Values also differ in their mutability:
- Immutable Values: These cannot be changed once they are created. Examples include integers, floats, strings, and tuples.
- Mutable Values: These can be modified after creation, such as lists, dictionaries, and sets.
This distinction is crucial for understanding how data is managed in Python.
Data Type | Mutability | Example |
---|---|---|
Integer | Immutable | 42 |
Float | Immutable | 3.14 |
String | Immutable | “Hello” |
List | Mutable | [1, 2, 3] |
Dictionary | Mutable | {“key”: “value”} |
Value Comparisons
In Python, values can also be compared using various operators, which return boolean values based on the comparison:
– **Equality (`==`)**: Checks if two values are equal.
– **Inequality (`!=`)**: Checks if two values are not equal.
– **Greater than (`>`)**: Checks if one value is greater than another.
- Less than (`<`): Checks if one value is less than another.
These comparisons are essential for control flow statements, such as `if` conditions and loops, allowing for dynamic decision-making in code.
Values form the backbone of data manipulation in Python. Understanding their types, mutability, and how to effectively compare and use them is crucial for any Python programmer. By mastering these concepts, one can enhance their coding capabilities and create more efficient and effective programs.
Understanding Values in Python
In Python, the term “values” refers to the data represented in the program. These values can take various forms, such as numbers, strings, lists, tuples, and more. Each value has a specific data type that determines how it can be used and manipulated.
Types of Values in Python
Python supports several built-in data types, which can be categorized into the following groups:
- Numeric Types:
- int: Represents integer values (e.g., `5`, `-3`)
- float: Represents floating-point values (e.g., `3.14`, `-0.001`)
- complex: Represents complex numbers (e.g., `3 + 4j`)
- Sequence Types:
- str: Represents a string of characters (e.g., `”Hello, World!”`)
- list: An ordered, mutable collection of values (e.g., `[1, 2, 3]`)
- tuple: An ordered, immutable collection of values (e.g., `(1, 2, 3)`)
- Mapping Type:
- dict: A collection of key-value pairs (e.g., `{‘name’: ‘Alice’, ‘age’: 30}`)
- Set Types:
- set: An unordered collection of unique values (e.g., `{1, 2, 3}`)
- frozenset: An immutable version of a set (e.g., `frozenset([1, 2, 3])`)
- Boolean Type:
- bool: Represents truth values (e.g., `True`, “)
How Values are Used in Python
Values are used in various contexts within Python programming, including:
- Variable Assignment: Values can be assigned to variables, allowing for the storage and manipulation of data.
“`python
x = 10 Assigning an integer value
name = “Alice” Assigning a string value
“`
- Expressions and Operations: Values can be combined using operators to create expressions.
“`python
result = x + 5 Adds 5 to the integer value of x
greeting = name + ” is here.” Concatenates strings
“`
- Function Arguments: Functions can take values as input parameters.
“`python
def greet(person):
return “Hello, ” + person
message = greet(name) Passing the value of name
“`
Value Immutability and Mutability
In Python, values can be classified based on their mutability:
- Immutable Values: These values cannot be changed after their creation. Examples include:
- `int`
- `float`
- `str`
- `tuple`
- Mutable Values: These values can be changed after their creation. Examples include:
- `list`
- `dict`
- `set`
This distinction is crucial when working with data structures, as mutable objects can lead to unintended side effects if not handled properly.
Checking Value Types
To determine the type of a value, the built-in `type()` function can be used:
“`python
print(type(10))
print(type(“Hello”))
print(type([1, 2, 3]))
“`
This functionality helps in understanding how to manipulate values correctly based on their type.
Conclusion on Values in Python
The concept of values in Python is fundamental to its functionality. Understanding the types, uses, and behaviors of these values is essential for effective programming.
Understanding the Role of Values in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, values are fundamental as they represent the data stored in variables. Understanding how values are assigned, manipulated, and compared is crucial for effective programming and debugging.”
Michael Thompson (Python Developer Advocate, CodeMaster Academy). “Values in Python serve as the building blocks of data structures. They can be integers, strings, lists, or even custom objects, and knowing how to work with them efficiently can greatly enhance a developer’s ability to write clean and maintainable code.”
Linda Zhang (Data Scientist, AI Analytics Group). “The concept of values in Python extends beyond mere data types; it encompasses how these values interact with functions and methods. Mastery of value handling is essential for anyone looking to leverage Python for data analysis or machine learning.”
Frequently Asked Questions (FAQs)
What does the `values()` method do in Python dictionaries?
The `values()` method returns a view object that displays a list of all the values in the dictionary. This view is dynamic, reflecting changes made to the dictionary.
How can I convert the output of `values()` to a list?
You can convert the output of the `values()` method to a list by passing it to the `list()` constructor, like so: `list(my_dict.values())`. This creates a static list of the dictionary’s values.
Can I iterate over the values returned by `values()`?
Yes, you can iterate over the values returned by the `values()` method using a for loop. For example: `for value in my_dict.values():` allows you to process each value in the dictionary.
Does the `values()` method return unique values only?
No, the `values()` method returns all values in the dictionary, including duplicates. If you need unique values, you can convert the output to a set: `set(my_dict.values())`.
Is the order of values returned by `values()` guaranteed in Python?
Yes, starting from Python 3.7, the `values()` method returns values in the order they were added to the dictionary, maintaining insertion order.
Can I use `values()` with other data structures in Python?
No, the `values()` method is specific to dictionaries. Other data structures, such as lists or sets, do not have a `values()` method. For those, you would use different approaches to access their elements.
In Python, the term “values” refers to the data that variables can hold. These values can take various forms, including integers, floating-point numbers, strings, lists, tuples, dictionaries, and more. Each of these data types has its own characteristics and behaviors, allowing for a wide range of programming possibilities. Understanding how to work with different values is fundamental for effective coding in Python, as it lays the groundwork for data manipulation and algorithm development.
Moreover, values in Python are associated with the concept of immutability and mutability. Immutable types, such as strings and tuples, cannot be changed after their creation, while mutable types, like lists and dictionaries, can be modified. This distinction is crucial when designing data structures and algorithms, as it affects performance and memory management. Additionally, Python’s dynamic typing allows for variables to hold values of different types over their lifecycle, providing flexibility in coding.
Another important aspect of values in Python is their role in expressions and operations. Python supports various operators that can be applied to values, enabling arithmetic calculations, logical comparisons, and more. Understanding how to effectively utilize these operators with different value types is essential for creating efficient and functional code. Furthermore, the concept of value equality and identity plays
Author Profile
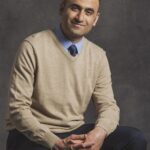
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?