What Does ‘wb’ Mean in Python: Understanding Write Binary Mode?
In the world of Python programming, understanding the nuances of file handling can significantly enhance your coding efficiency and effectiveness. Among the various modes available for opening files, the term ‘wb’ often surfaces, especially when dealing with binary data. But what exactly does ‘wb’ mean, and why is it crucial for developers working with files? This article will unravel the significance of ‘wb’ in Python, shedding light on its applications and best practices, ensuring that you can harness its power in your projects.
When you open a file in Python, you have the option to specify various modes that dictate how the file will be accessed. The ‘wb’ mode is a combination of two key elements: ‘w’ for write and ‘b’ for binary. This means that when you open a file using ‘wb’, you are preparing to write binary data to that file, which is essential for tasks such as saving images, audio files, or any other non-text data. Understanding this mode is vital for developers who need to manipulate files that don’t conform to standard text encoding, as it ensures that the data is handled correctly and efficiently.
Moreover, using ‘wb’ comes with specific implications for how data is written and managed within your Python applications. Unlike text files, where encoding can introduce complications,
Understanding ‘wb’ Mode in Python
When dealing with file operations in Python, the mode in which a file is opened plays a crucial role in determining how data is read from or written to that file. The ‘wb’ mode specifically refers to writing binary data to a file.
In this context, ‘w’ stands for write mode, which allows for writing data to a file. If the file already exists, it will be truncated (emptied) before writing new data. The ‘b’ indicates that the file is opened in binary mode. This is essential for handling non-text files, such as images, audio files, or any other type of binary data.
Key distinctions when using ‘wb’ mode include:
- Binary Format: Data is written in a format that is not encoded into a text representation. This is particularly important for binary files where the integrity of the data must be preserved.
- No Character Encoding: Unlike text mode, where data may undergo encoding transformations (like UTF-8), binary mode writes data exactly as it is in memory.
When to Use ‘wb’ Mode
Using ‘wb’ mode is appropriate in several scenarios:
- Saving images or audio files that must maintain their original binary format.
- Writing serialized data from structures like bytes or byte arrays.
- Handling proprietary file formats that require specific binary layouts.
For example, when dealing with image processing in Python, libraries like Pillow often require you to open a file in ‘wb’ mode to save images correctly without data loss.
Example of Using ‘wb’ Mode
The following code snippet illustrates how to use the ‘wb’ mode in Python for writing binary data to a file:
python
# Example of writing binary data to a file
data = bytes([120, 3, 255, 0, 100]) # Example binary data
with open(‘binary_file.bin’, ‘wb’) as file:
file.write(data)
In this example, a byte array is created and written to ‘binary_file.bin’ using ‘wb’ mode, ensuring that the data is written as raw bytes.
Comparative Table of File Modes
Mode | Description | Text/Binary |
---|---|---|
‘r’ | Open for reading | Text |
‘w’ | Open for writing (truncates) | Text |
‘rb’ | Open for reading (binary) | Binary |
‘wb’ | Open for writing (binary, truncates) | Binary |
This table outlines some common file modes in Python, highlighting the differences between text and binary modes, including their intended operations. Understanding these distinctions is essential for effective file handling in Python programming.
Understanding ‘wb’ Mode in Python
In Python, the `’wb’` mode is commonly used when opening files for writing in binary format. This mode is essential for handling non-text files, such as images or executable files, where data integrity is crucial.
File Modes in Python
When working with files in Python, various modes dictate how files are accessed. The most relevant modes include:
- ‘r’: Read (default mode). Opens a file for reading.
- ‘w’: Write. Opens a file for writing, truncating the file first.
- ‘a’: Append. Opens a file for writing, appending new data without truncating.
- ‘b’: Binary. Used in conjunction with other modes to handle binary data.
The `’wb’` mode combines the write and binary modes, allowing you to write binary data to a file.
When to Use ‘wb’
The `’wb’` mode is particularly useful in scenarios such as:
- Writing images, audio files, or video files.
- Handling data serialization formats like Protocol Buffers or MessagePack.
- Writing binary logs or dumps from applications.
Using `’wb’` ensures that the data is written exactly as it is, without any encoding transformations that could corrupt the data.
Example of Using ‘wb’
Here is a simple example demonstrating how to use `’wb’`:
python
# Writing binary data to a file
data = bytearray([120, 3, 255, 0, 100]) # Example binary data
with open(‘output.bin’, ‘wb’) as file:
file.write(data)
In this example, a `bytearray` is created and written to a file named `output.bin`. The use of `’wb’` ensures that the binary data is written without alteration.
Considerations When Using ‘wb’
When using the `’wb’` mode, keep the following points in mind:
- Data Loss: Opening a file in `’wb’` mode truncates the file if it already exists. Ensure that you do not overwrite important data inadvertently.
- Binary vs Text: Always use `’wb’` for binary files. For text files, use `’w’` or `’wt’` to ensure proper encoding handling.
- File Size: Writing large binary files may require careful consideration of memory usage and file handling practices.
Common Errors
When working with the `’wb’` mode, you may encounter specific errors, including:
Error | Description |
---|---|
`FileNotFoundError` | The specified path does not exist or is incorrect. |
`PermissionError` | Insufficient permissions to write to the specified location. |
Ensuring the correct file path and permissions can help mitigate these issues.
Understanding the Significance of ‘wb’ in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, the ‘wb’ mode stands for ‘write binary.’ This mode is crucial when dealing with binary files, such as images or executable files, ensuring that the data is written correctly without any unintended transformations that could occur in text mode.”
Michael Chen (Software Engineer, Data Solutions Corp.). “Using ‘wb’ in Python is essential for preserving the integrity of binary data. When you open a file with this mode, you are explicitly telling Python to handle the data as raw bytes, which is particularly important for applications that require precise data manipulation, like file compression or encryption.”
Laura Patel (Python Instructor, Code Academy). “Many beginners overlook the importance of file modes in Python. Understanding ‘wb’ is fundamental for anyone working with file I/O operations, especially when the goal is to save images or other non-text data. It prevents data corruption and ensures that the file can be read back accurately later.”
Frequently Asked Questions (FAQs)
What does ‘wb’ mean in Python file handling?
‘wb’ stands for “write binary.” It is a mode used when opening files in Python, indicating that the file is opened for writing in binary format.
Why would I use ‘wb’ instead of ‘w’?
Using ‘wb’ is essential when dealing with non-text files, such as images or audio files, where binary data needs to be written without any encoding alterations.
Can I read a file opened in ‘wb’ mode?
No, a file opened in ‘wb’ mode cannot be read. It is exclusively for writing binary data. To read, you must open the file in a mode such as ‘rb’ (read binary).
What happens if I try to write text data using ‘wb’?
If you attempt to write text data using ‘wb’, Python will raise a `TypeError` because binary mode expects bytes, not strings. You must encode the string to bytes first.
Is it necessary to close a file opened in ‘wb’ mode?
Yes, it is crucial to close a file opened in ‘wb’ mode to ensure that all data is properly written and resources are released. This can be done using the `close()` method or by utilizing a `with` statement.
How do I convert a string to bytes before writing in ‘wb’ mode?
You can convert a string to bytes using the `encode()` method. For example, `my_string.encode(‘utf-8’)` converts the string to a byte representation suitable for writing in ‘wb’ mode.
The term ‘wb’ in Python is a mode used when opening files, specifically indicating that the file is to be opened in ‘write binary’ mode. This is crucial when dealing with binary files, such as images or executable files, where data integrity must be maintained. In this mode, any data written to the file is treated as binary data, which prevents any unintended alterations that might occur if the file were opened in text mode.
Using ‘wb’ ensures that the data is written exactly as it is, byte for byte, without any conversion to a string format. This is particularly important for applications that require precise control over the data being written, such as when saving images or other non-text files. It is also essential to remember that when using ‘wb’, the file will be created if it does not already exist, and if it does exist, it will be truncated, meaning any existing content will be lost.
In summary, the ‘wb’ mode is a fundamental aspect of file handling in Python that facilitates the correct writing of binary data. Understanding its implications helps developers avoid common pitfalls associated with file I/O operations, ensuring that data is preserved accurately and efficiently. This knowledge is essential for anyone working with files in Python, particularly
Author Profile
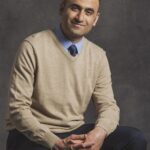
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?