What Does ‘while True’ Mean in Python: Understanding Infinite Loops?
In the world of programming, certain constructs can evoke both curiosity and caution, and one such construct in Python is the infamous `while True` loop. For many budding developers, the phrase might conjure images of endless cycles and infinite possibilities, but it also raises questions about control, efficiency, and the very nature of loops in programming. Understanding `while True` is essential for anyone looking to master Python, as it serves as a powerful tool for creating dynamic and responsive applications. In this article, we will delve into what `while True` means, how it operates within the Python environment, and the best practices for using it effectively.
At its core, the `while True` statement initiates an infinite loop that runs continuously until explicitly broken out of. This construct is particularly useful in scenarios where you want a program to keep running until a specific condition is met or a user decides to exit. However, with great power comes great responsibility; using `while True` without proper control mechanisms can lead to unresponsive programs or excessive resource consumption. Thus, understanding the implications of this loop is crucial for writing efficient and robust code.
As we explore the nuances of `while True`, we’ll also touch on practical applications, including user input handling and event-driven programming. By the end of this
Understanding the Structure of `while True`
The `while True` loop in Python is a fundamental construct that allows for the creation of an infinite loop. This loop will continue executing its block of code indefinitely until it is explicitly interrupted by a `break` statement or an external factor such as an exception or a system interrupt. The basic structure of a `while True` loop is as follows:
“`python
while True:
Code to execute repeatedly
“`
Within the loop, any code placed will be executed over and over again. The control flow only exits the loop when a `break` statement is encountered.
Common Use Cases for `while True`
`while True` loops are particularly useful in scenarios where the number of iterations is not predetermined. Some common use cases include:
- User Input: Continuously prompting users for input until valid data is received.
- Server Applications: Running services that need to listen for incoming requests continuously.
- Game Loops: Maintaining the game state and rendering frames until the user decides to exit.
These loops provide flexibility and control in various programming scenarios.
Breaking Out of the Loop
To exit a `while True` loop, the `break` statement is used. This statement halts the loop’s execution and transfers control to the statement immediately following the loop. Here is an example:
“`python
while True:
user_input = input(“Enter ‘exit’ to quit: “)
if user_input.lower() == ‘exit’:
break
“`
In the example above, the loop continues until the user types “exit”. This is a common pattern for handling user interactions.
Considerations and Best Practices
While `while True` loops are powerful, they should be used judiciously. Here are some best practices to consider:
- Ensure a Break Condition: Always ensure that there is a valid exit condition to prevent creating an unintentional infinite loop.
- Resource Management: Be cautious about resource usage. If the loop performs operations that consume memory or processing power, ensure they are efficient or limited.
- Avoid Blocking Calls: Avoid using blocking calls (like `input()`) inside the loop if responsiveness is critical, as it can halt execution.
Example of `while True` with Multiple Break Conditions
“`python
while True:
command = input(“Enter command (start/stop/exit): “)
if command == ‘start’:
print(“Starting process…”)
elif command == ‘stop’:
print(“Stopping process…”)
elif command == ‘exit’:
print(“Exiting program.”)
break
else:
print(“Unknown command, please try again.”)
“`
In this example, the loop processes multiple commands and exits gracefully when the user types “exit”.
Performance Considerations
When implementing a `while True` loop, performance can be affected by how the loop is structured. Consider the following points:
Factor | Impact |
---|---|
Loop Complexity | Higher complexity increases CPU usage and can lead to performance degradation. |
Delay Mechanism | Including sleep intervals (e.g., `time.sleep()`) can reduce CPU load during idle waiting periods. |
Error Handling | Implementing try-except blocks can prevent crashes due to unexpected input or exceptions. |
By following these guidelines, you can effectively implement `while True` loops in your Python applications while maintaining good performance and code readability.
Understanding `while True` in Python
The `while True` statement in Python creates an infinite loop that continues to execute as long as the condition `True` remains valid. This construct is commonly used in scenarios where a program needs to repeatedly execute a block of code until a specific condition is met to break the loop.
How `while True` Works
- The loop starts with the `while` keyword followed by the condition `True`.
- The code block within the loop will execute indefinitely unless interrupted by a `break` statement or an external factor (such as an error or user interrupt).
Example:
“`python
while True:
print(“This will print endlessly until interrupted.”)
“`
Common Use Cases
The `while True` loop is particularly useful in various programming scenarios:
- User Input Handling: Continuously prompt users for input until valid data is provided.
- Event Listening: Keep a program running to listen for events or messages, such as in server applications.
- Game Loops: Maintain the state of a game until the game is over or the player chooses to exit.
Breaking Out of the Loop
To exit a `while True` loop, a `break` statement is typically employed. This allows for a controlled exit from the loop based on specific conditions.
Example:
“`python
while True:
user_input = input(“Enter ‘exit’ to stop: “)
if user_input == ‘exit’:
break
“`
In this example, the loop continues until the user types “exit”, at which point the `break` statement terminates the loop.
Potential Risks and Considerations
While using `while True` can be effective, it also carries risks:
- Infinite Loops: If not properly managed, a `while True` loop can lead to infinite loops, causing the program to hang or consume excessive resources.
- Resource Management: Ensure that resources (like file handles or network connections) are properly managed within the loop to prevent leaks.
Best Practices
To effectively use `while True`, consider the following best practices:
- Always include a clear exit condition using `break`.
- Utilize `try-except` blocks to handle exceptions gracefully.
- Keep the loop body efficient to minimize performance impacts.
Example of a safer implementation:
“`python
while True:
try:
user_input = input(“Type something (or ‘quit’ to exit): “)
if user_input.lower() == ‘quit’:
break
print(f”You typed: {user_input}”)
except KeyboardInterrupt:
print(“\nExiting…”)
break
“`
By following these practices, developers can harness the power of `while True` while minimizing potential pitfalls associated with infinite loops.
Understanding the Significance of `while True` in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The `while True` construct in Python is a fundamental loop that continues indefinitely until explicitly broken. It is particularly useful in scenarios where a program needs to wait for an event or condition to change, such as in server applications or interactive scripts.”
Michael Chen (Python Developer and Educator, Code Academy). “Using `while True` allows developers to create robust and flexible loops. However, it is essential to implement a proper exit strategy within the loop to avoid infinite execution, which can lead to resource exhaustion or application crashes.”
Sarah Thompson (Lead Data Scientist, Data Insights Group). “In data processing tasks, `while True` can be particularly advantageous for continuously reading data streams until a termination condition is met. This pattern is often seen in real-time data analysis applications where constant monitoring is required.”
Frequently Asked Questions (FAQs)
What does while true mean in Python?
The statement `while True:` initiates an infinite loop in Python, meaning the loop will continue to execute indefinitely until a break condition is met or the program is terminated.
How can I exit a while true loop in Python?
You can exit a `while True` loop by using the `break` statement within the loop when a specific condition is satisfied, or by raising an exception or terminating the program.
Are there any risks associated with using while true?
Yes, using `while True` without a proper exit condition can lead to unresponsive programs or high CPU usage, as the loop will run endlessly unless explicitly stopped.
Can I use while true with other control statements?
Yes, `while True` can be combined with control statements such as `if`, `else`, and `try-except` to manage the flow of the program and handle exceptions effectively.
What is a common use case for while true in Python?
A common use case for `while True` is in server applications, where the server continuously listens for incoming requests until it is instructed to shut down.
Is there a performance impact of using while true in Python?
The performance impact depends on what is executed within the loop. If the loop contains resource-intensive operations without delays, it may lead to performance degradation. Using `time.sleep()` can mitigate this issue.
The phrase “while True” in Python is commonly used to create an infinite loop. This construct allows a block of code to execute repeatedly without a predetermined end condition. The loop will continue to run indefinitely until it is explicitly interrupted, typically by a break statement or an external interruption such as a keyboard interrupt. This feature is particularly useful in scenarios where continuous monitoring or repeated execution of a task is required, such as in server applications or real-time data processing.
One of the key aspects of using “while True” is the necessity for careful management of the loop’s execution. Developers must ensure that there are appropriate exit conditions to prevent the program from running endlessly and consuming system resources. This can be achieved through conditional statements that check for specific criteria to break the loop when necessary. Additionally, implementing sleep intervals or other control mechanisms can help mitigate resource usage during the loop’s execution.
In summary, “while True” serves as a powerful tool in Python for creating infinite loops, but it requires responsible usage and control mechanisms to avoid potential pitfalls. Understanding when and how to use this construct effectively can enhance the functionality of Python applications while ensuring they remain efficient and manageable.
Author Profile
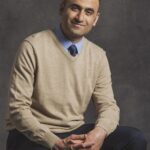
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?