What Is a Circular Import in Python and How Can You Avoid It?
In the intricate world of Python programming, the concept of circular imports can often perplex even seasoned developers. Imagine a scenario where two or more modules depend on each other, creating a loop that can lead to unexpected behavior and errors. This phenomenon, known as a circular import, can disrupt the flow of your code and hinder the seamless execution of your applications. Understanding circular imports is crucial for any Python programmer aiming to write clean, efficient, and maintainable code. In this article, we will delve into the mechanics of circular imports, explore their implications, and provide strategies to avoid or resolve them.
Circular imports occur when two or more modules attempt to import each other directly or indirectly, leading to a situation where Python cannot resolve the dependencies correctly. This can result in an `ImportError`, as the interpreter struggles to load the modules in the correct order. The challenge lies not only in the error itself but also in the potential for hidden bugs and complicated debugging processes that can arise from such imports.
To navigate the complexities of circular imports, it is essential to grasp the underlying principles of Python’s import system. By understanding how Python handles module loading and the implications of interdependencies, developers can adopt best practices to prevent these issues from arising in the first place. Whether you are
Understanding Circular Imports
Circular imports occur when two or more modules attempt to import each other directly or indirectly, creating a loop in the import dependency. This situation can lead to issues such as `ImportError` or unexpected behavior in the code execution.
How Circular Imports Happen
Circular imports typically arise in scenarios where:
- Two modules are interdependent: Each module needs something from the other, resulting in an import cycle.
- Multiple layers of imports: A module A imports module B, and module B imports module A, either directly or through other modules.
For example, consider the following modules:
- Module A:
“`python
from module_b import function_b
def function_a():
function_b()
“`
- Module B:
“`python
from module_a import function_a
def function_b():
function_a()
“`
In this case, trying to import either module will lead to a circular import error.
Consequences of Circular Imports
The primary issues that arise from circular imports include:
- ImportError: Python raises an error indicating that a module cannot be imported due to a circular dependency.
- Partial Initialization: If a circular import is resolved, the imported module may not be fully initialized, leading to `AttributeError` when trying to access certain functions or classes.
Strategies to Avoid Circular Imports
To prevent circular imports, consider the following strategies:
- Refactor Code: Organize your code to minimize dependencies. For instance, separate shared functionality into a third module that both original modules can import without issues.
- Use Local Imports: Instead of importing at the top of a module, perform imports inside functions or methods. This delays the import until the function is called, potentially avoiding circular import issues.
“`python
Module A
def function_a():
from module_b import function_b Local import
function_b()
“`
- Design Patterns: Employ design patterns like dependency injection or service locators to reduce tight coupling between modules.
Example of Circular Import Resolution
Here is a table illustrating how to refactor modules to avoid circular imports:
Module | Before Refactor | After Refactor |
---|---|---|
Module A | from module_b import function_b | from shared_module import shared_function |
Module B | from module_a import function_a | from shared_module import shared_function |
Shared Module | — | def shared_function(): … |
By creating a `shared_module`, both `Module A` and `Module B` can import necessary functions without causing circular dependencies.
Understanding Circular Imports
Circular imports occur when two or more modules depend on each other directly or indirectly. This can lead to issues during the importing process, as Python may not fully load one of the modules before the other tries to access it. Such scenarios can result in `ImportError`, `AttributeError`, or unexpected behavior in the application.
How Circular Imports Occur
Circular imports typically arise from:
- Direct Circular Imports: Module A imports Module B, and Module B imports Module A.
- Indirect Circular Imports: Module A imports Module B, which in turn imports Module C, and Module C imports Module A.
The execution order of imports can lead to incomplete definitions of classes or functions being accessed in a module that is not fully initialized.
Identifying Circular Import Problems
Common symptoms of circular imports include:
- ImportError: Raised when a module is not found due to incomplete loading.
- AttributeError: Occurs when a function or class cannot be accessed because its definition is not yet available.
To identify circular imports, you can use:
- Static Code Analysis: Tools like `pylint` or `flake8` can detect circular dependencies.
- Code Review: Manually inspecting import statements can often reveal cycles.
Preventing Circular Imports
Several strategies can help avoid circular imports:
- Reorganizing Code: Refactor code to reduce interdependencies. Place shared functions or classes in a separate module.
- Importing Locally: Instead of importing at the top of a module, import within a function or method where the dependency is needed.
- Using Interfaces: Define interfaces or abstract classes to decouple modules.
Example of Circular Import
“`python
module_a.py
from module_b import func_b
def func_a():
print(“Function A”)
func_b()
module_b.py
from module_a import func_a
def func_b():
print(“Function B”)
func_a()
“`
In this example, attempting to run either function will lead to an ImportError, as both modules are waiting for the other to finish loading.
Resolving Circular Imports
To resolve existing circular imports, consider these approaches:
- Refactor Dependencies: Move shared logic to a new module.
- Use Lazy Imports: Import modules inside functions to delay their loading.
- Check for Initialization: Use `if __name__ == “__main__”:` to prevent certain code from running on import.
Conclusion on Circular Imports
While circular imports can lead to significant issues in Python, understanding their nature allows developers to implement effective strategies to avoid and resolve them, ensuring smoother module interactions and a more stable codebase.
Understanding Circular Imports in Python: Expert Perspectives
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Circular imports can lead to significant complications in Python applications. They occur when two or more modules attempt to import each other, creating a loop that can result in ImportError. It is crucial to design your modules carefully to avoid such situations, often by restructuring code or using import statements strategically.
Michael Chen (Python Developer Advocate, Open Source Community). Circular imports are a common pitfall for Python developers, especially in larger projects. They can hinder code readability and maintainability. To mitigate these issues, developers should consider using local imports within functions or classes instead of global imports at the module level, thus breaking the circular dependency.
Lisa Patel (Lead Python Instructor, Code Academy). Understanding circular imports is essential for any Python programmer. These imports can lead to runtime errors that are often difficult to debug. A good practice is to keep your modules focused and cohesive, ensuring that each module has a clear purpose, which can help in avoiding circular dependencies altogether.
Frequently Asked Questions (FAQs)
What is a circular import in Python?
A circular import occurs when two or more modules attempt to import each other directly or indirectly, creating a loop in the import dependency. This can lead to issues such as incomplete module initialization.
How does a circular import affect program execution?
Circular imports can result in ImportError or AttributeError, as Python may not fully initialize a module before it is accessed. This can cause unexpected behavior or crashes in the program.
What are common scenarios that lead to circular imports?
Common scenarios include tightly coupled modules that depend on each other for functionality, such as two classes in separate files that reference each other directly or indirectly.
How can circular imports be resolved in Python?
Circular imports can be resolved by restructuring the code, such as moving shared functionality to a separate module, using import statements within functions or methods, or employing dependency injection.
Are there any tools to detect circular imports in Python?
Yes, tools such as `pylint`, `flake8`, and `pydeps` can help detect circular imports by analyzing the import graph of the modules in a Python project.
Can circular imports be avoided entirely in Python?
While it may not be possible to eliminate all circular imports, they can be minimized through careful design, such as adhering to the principles of modularity and separation of concerns in code architecture.
A circular import in Python occurs when two or more modules attempt to import each other directly or indirectly, creating a loop in the import dependency. This situation can lead to complications, such as incomplete module initialization and runtime errors, as Python may not be able to resolve the dependencies correctly. Understanding the structure of your modules and their interdependencies is crucial in avoiding circular imports.
To prevent circular imports, developers can adopt several best practices. These include organizing code into smaller, more focused modules, using local imports within functions instead of global imports at the top of the module, and refactoring code to reduce interdependencies. By implementing these strategies, developers can create a more maintainable and error-free codebase.
In summary, circular imports can pose significant challenges in Python programming, but with careful planning and code organization, these issues can be effectively mitigated. Recognizing the signs of circular imports and applying best practices will enhance code clarity and functionality, ultimately leading to more robust applications.
Author Profile
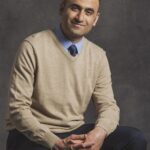
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?