What Is a Python Algorithm? Unraveling the Basics and Their Importance
In the realm of computer programming, algorithms serve as the backbone of problem-solving and data processing. Among the myriad of programming languages, Python stands out for its simplicity and versatility, making it a favorite among both beginners and seasoned developers. But what exactly is a Python algorithm? This question opens the door to a fascinating exploration of how Python can be employed to create efficient solutions to complex problems. Whether you’re looking to optimize a process, analyze data, or automate tasks, understanding Python algorithms is crucial to harnessing the full potential of this powerful language.
At its core, a Python algorithm is a step-by-step procedure or formula for solving a specific problem using the Python programming language. These algorithms can range from simple tasks, like sorting a list of numbers, to more complex operations, such as implementing machine learning models or processing large datasets. What makes Python particularly appealing is its rich ecosystem of libraries and frameworks that simplify the implementation of these algorithms, allowing developers to focus on logic rather than syntax.
Delving deeper into Python algorithms reveals a world of concepts such as efficiency, complexity, and optimization. Each algorithm has its own strengths and weaknesses, often determined by the nature of the problem it aims to solve. By understanding these principles, programmers can make informed decisions about which algorithms to use
Understanding Python Algorithms
An algorithm in Python is essentially a step-by-step procedure or formula for solving a problem. It serves as the foundation for writing programs and implementing logic, enabling developers to perform computations, data processing, and automated reasoning. Python algorithms can be expressed in various forms, including flowcharts, pseudocode, and actual code, allowing for flexibility in understanding and implementation.
Types of Algorithms in Python
Python supports several types of algorithms, each tailored for specific tasks. These can be categorized as follows:
- Sorting Algorithms: Used to arrange data in a specific order (ascending or descending). Common examples include:
- Quick Sort
- Merge Sort
- Bubble Sort
- Searching Algorithms: Designed to retrieve information stored within a data structure. Key types include:
- Linear Search
- Binary Search
- Graph Algorithms: Used for processing graphs and networks, including:
- Dijkstra’s Algorithm
- Depth-First Search (DFS)
- Breadth-First Search (BFS)
- Dynamic Programming Algorithms: Optimize complex problems by breaking them down into simpler subproblems, such as:
- Fibonacci Sequence
- Knapsack Problem
Implementing a Simple Algorithm
To illustrate how algorithms function in Python, consider the implementation of a linear search algorithm. This algorithm searches for a specific value within a list by checking each element sequentially.
“`python
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i Return the index of the target
return -1 Return -1 if the target is not found
“`
This function takes a list `arr` and a `target` value as inputs, iterating through the list to find the target’s index.
Algorithm Complexity
Understanding algorithm complexity is crucial for evaluating the efficiency of an algorithm. Two primary metrics are used to describe complexity:
- Time Complexity: Indicates the amount of time an algorithm takes to complete as a function of the input size.
- Space Complexity: Represents the amount of memory an algorithm uses relative to the input size.
The complexity can be expressed in Big O notation, which classifies algorithms according to their worst-case scenarios. Here’s a brief comparison:
Algorithm Type | Time Complexity | Space Complexity |
---|---|---|
Linear Search | O(n) | O(1) |
Binary Search | O(log n) | O(1) |
Merge Sort | O(n log n) | O(n) |
By analyzing both time and space complexity, developers can make informed decisions about which algorithm to use based on the constraints of their specific application.
Python algorithms are a fundamental aspect of programming, enabling efficient problem-solving and data manipulation. Understanding the various types of algorithms, their implementation, and their complexity is essential for any Python developer seeking to enhance their coding skills.
Definition of a Python Algorithm
A Python algorithm is a well-defined sequence of steps or rules that are designed to solve a specific problem or perform a task using the Python programming language. Algorithms can be implemented in various ways, but in the context of Python, they typically involve a combination of data structures, control flow statements, and functions.
Characteristics of Python Algorithms
Python algorithms possess several key characteristics that define their functionality and efficiency:
- Unambiguous: Each step must be clear and precise to avoid confusion.
- Finiteness: An algorithm must terminate after a finite number of steps.
- Input: An algorithm can have zero or more inputs, which are the data it processes.
- Output: An algorithm must produce at least one output, which is the result of the computation.
- Effectiveness: Each operation must be feasible and able to be performed using basic operations.
Types of Python Algorithms
Python algorithms can be categorized based on their functionality and the problems they solve. Some common types include:
- Sorting Algorithms: Organize data in a particular order (e.g., bubble sort, quicksort).
- Searching Algorithms: Retrieve information from data structures (e.g., binary search).
- Graph Algorithms: Solve problems related to graph theory (e.g., Dijkstra’s algorithm).
- Dynamic Programming: Optimize problems by breaking them down into simpler subproblems (e.g., Fibonacci sequence).
- Machine Learning Algorithms: Learn patterns from data (e.g., linear regression, decision trees).
Common Python Algorithm Examples
Here are a few examples of algorithms implemented in Python:
Bubble Sort
“`python
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
“`
Binary Search
“`python
def binary_search(arr, target):
left, right = 0, len(arr) – 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
```
Complexity Analysis of Algorithms
Understanding the efficiency of algorithms is critical. The complexity of algorithms can be analyzed in terms of time and space:
Algorithm Type | Time Complexity | Space Complexity |
---|---|---|
Bubble Sort | O(n^2) | O(1) |
Quick Sort | O(n log n) | O(log n) |
Binary Search | O(log n) | O(1) |
Dijkstra’s Algorithm | O(V^2) or O(E log V) | O(V) |
Implementing Algorithms in Python
When implementing algorithms in Python, consider the following best practices:
- Modularity: Write functions to encapsulate logic for reusability and clarity.
- Readability: Use meaningful variable names and comments to enhance understanding.
- Testing: Validate algorithms with various test cases to ensure correctness and robustness.
- Optimization: Analyze and refine algorithms to improve performance based on specific use cases.
Conclusion on Python Algorithms
Python algorithms are foundational to programming in Python, serving as the building blocks for more complex applications. By understanding and implementing various algorithms, developers can effectively solve a wide range of computational problems.
Understanding Python Algorithms Through Expert Insights
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “A Python algorithm is essentially a step-by-step procedure or formula for solving a problem using the Python programming language. It is crucial for data analysis and machine learning, as it allows developers to implement efficient and effective solutions.”
Michael Thompson (Software Engineer, CodeCraft Solutions). “In the realm of software development, a Python algorithm is a structured approach to problem-solving. It leverages Python’s syntax and libraries to create solutions that can range from simple calculations to complex data manipulations.”
Sarah Patel (Computer Science Professor, University of Technology). “Understanding what a Python algorithm is involves recognizing its role in computational thinking. It is not just about coding; it is about designing a logical sequence of operations that can be implemented in Python to achieve a desired outcome.”
Frequently Asked Questions (FAQs)
What is a Python algorithm?
A Python algorithm is a step-by-step procedure or formula for solving a problem or performing a task using the Python programming language. It consists of a sequence of instructions that can be executed to achieve a specific outcome.
How do algorithms work in Python?
Algorithms in Python work by defining a clear set of rules or operations that the program follows to process input data and produce output. They can be implemented using functions, loops, and conditional statements to manipulate data effectively.
What are common types of algorithms used in Python?
Common types of algorithms used in Python include sorting algorithms (like quicksort and mergesort), searching algorithms (such as binary search), and algorithms for data manipulation (like graph algorithms and dynamic programming).
Can you give an example of a simple Python algorithm?
A simple example of a Python algorithm is a function that calculates the factorial of a number. The algorithm recursively multiplies the number by the factorial of the number minus one until it reaches one.
Why are algorithms important in programming?
Algorithms are crucial in programming because they provide a clear and efficient method for solving problems. They help optimize performance, reduce complexity, and ensure that programs run effectively and efficiently.
How can I learn more about algorithms in Python?
To learn more about algorithms in Python, consider studying algorithm design and analysis through online courses, textbooks, and coding practice platforms. Engaging in projects and challenges can also enhance your understanding and application of algorithms.
A Python algorithm refers to a step-by-step procedure or formula for solving a specific problem using the Python programming language. Algorithms can be expressed in various forms, including natural language, pseudocode, or programming code. In Python, algorithms are implemented through functions, classes, and other programming constructs, enabling developers to create efficient and effective solutions to a wide range of computational problems.
Understanding Python algorithms is crucial for programmers as they form the foundation of problem-solving in computer science. Algorithms can be categorized into various types, such as sorting algorithms, searching algorithms, and optimization algorithms, each serving distinct purposes. Mastery of these algorithms allows developers to write code that not only functions correctly but also performs optimally in terms of time and space complexity.
Moreover, the ability to design and implement algorithms in Python enhances a programmer’s skill set, making them more versatile and capable of tackling complex challenges. As Python is widely used in fields such as data science, machine learning, and web development, proficiency in algorithm design is essential for leveraging the full potential of the language. Overall, a solid grasp of Python algorithms is integral to achieving success in programming and software development.
Author Profile
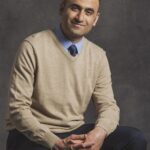
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?