What Is an EOF Error in Python and How Can You Fix It?
### Understanding EOF Errors in Python: A Deep Dive
In the world of programming, encountering errors is an inevitable part of the journey, and Python developers are no exception. Among the myriad of errors that can arise, the “EOFError” stands out as a particularly intriguing one. Short for “End Of File Error,” this exception occurs when a program attempts to read beyond the available data, often in contexts where user input or file reading is involved. For both novice and seasoned programmers, understanding EOFError is crucial for writing robust, error-resistant code.
When you’re working with input functions in Python, especially in interactive scripts or when reading from files, the EOFError can unexpectedly interrupt your flow. This error typically surfaces when the program reaches the end of a file or input stream without receiving the expected data. For instance, if a script anticipates user input but the user simply hits Enter without providing any data, an EOFError can be triggered, leading to confusion and potential disruptions in program execution.
Moreover, EOFErrors can arise in various scenarios, including reading from files that have been prematurely closed or when trying to parse input in a loop that doesn’t properly handle termination conditions. Understanding how and why these errors occur is essential for debugging and improving the resilience of your Python applications
Understanding EOFError in Python
EOFError, short for “End Of File Error,” is an exception raised in Python when a program attempts to read beyond the end of a file or input stream. This error typically occurs in scenarios involving file handling, user input, or network streams where the end of the data is reached unexpectedly.
The EOFError is particularly relevant when using functions that read input, such as `input()`, `read()`, or `readline()`. When these functions expect more data but encounter an end-of-file condition, Python raises this error to signal that there is no more data to read.
Common Causes of EOFError
Understanding the common scenarios that lead to EOFError can help developers implement better error handling and avoid unexpected crashes in their applications. Some of these scenarios include:
- Reading from an Empty File: Attempting to read data from a file that is empty will trigger EOFError.
- Unexpected End of Input: When using `input()` in a script, if the user presses Ctrl+D (on Unix) or Ctrl+Z (on Windows) to signal the end of input, an EOFError will be raised.
- Socket Connections: In network programming, reading from a socket that has been closed by the peer can result in an EOFError.
Handling EOFError
To gracefully handle EOFError, developers can use try-except blocks. This allows the program to continue running even when such errors occur. Below is an example of how to implement this:
python
try:
user_input = input(“Enter something: “)
except EOFError:
print(“No input was received. Please try again.”)
In this example, the program will not crash if the user provides no input; instead, it will print a friendly message.
Best Practices
To avoid EOFError and enhance the robustness of your code, consider the following best practices:
- Check File Existence: Always verify that the file exists and is not empty before attempting to read from it.
- Use a Loop for Input: When using `input()`, implement a loop that allows the user to retry until valid input is provided.
- Implement Error Logging: Log the occurrence of EOFError to diagnose issues more easily.
Example of EOFError in Context
To illustrate EOFError in a more practical context, consider the following table that summarizes common scenarios and their handling:
Scenario | Handling Method |
---|---|
Reading from an empty file | Check if the file is empty before reading. |
User input with Ctrl+D or Ctrl+Z | Use try-except to catch EOFError and prompt again. |
Network socket closed | Implement exception handling to manage unexpected closures. |
By following these guidelines and understanding the context in which EOFError arises, developers can create more resilient Python applications that handle unexpected situations gracefully.
Understanding EOFError in Python
EOFError, or End Of File Error, occurs in Python when the input function attempts to read beyond the end of a file or standard input stream. This error typically arises in contexts where the program expects more input than is available, leading to an unexpected termination of the input process.
Common Scenarios Leading to EOFError
Several situations can trigger an EOFError, including:
- Reading from Empty Input: When the input function is called but no data is provided.
- File Reading: Attempting to read from a file that has already reached its end.
- Input Stream Issues: Issues with standard input when using tools or environments that do not provide input as expected.
Example Cases of EOFError
To better understand EOFError, consider the following examples:
python
# Example 1: Missing Input
try:
user_input = input(“Enter some data: “)
except EOFError:
print(“No input was provided.”)
# Example 2: Reading from a file
with open(’empty_file.txt’, ‘r’) as f:
try:
data = f.read()
if not data:
raise EOFError(“Reached the end of the file.”)
except EOFError as e:
print(e)
In the first example, if the user fails to provide any input, an EOFError is raised. In the second, an attempt to read from an empty file leads to an EOFError.
Handling EOFError
Properly handling EOFError can enhance the robustness of your code. Consider the following strategies:
- Try-Except Blocks: Surround input or file reading operations with try-except blocks to catch EOFError.
- Input Validation: Before processing input, validate that it exists to prevent premature termination.
- Use Default Values: Provide default values when input is not available to maintain program flow.
Example of handling EOFError:
python
try:
data = input(“Please enter data: “)
except EOFError:
data = “Default Data”
print(data)
EOFError in Different Environments
The behavior of EOFError may vary depending on the environment in which the Python code is executed:
Environment | Behavior |
---|---|
Interactive Shell | EOFError can occur if Ctrl+D (Linux/Mac) or Ctrl+Z (Windows) is pressed. |
Scripts executed from Command Line | EOFError occurs if no input is provided when the script expects it. |
Integrated Development Environments (IDEs) | Some IDEs handle standard input differently, potentially masking EOFError. |
Understanding these variations can help in anticipating and managing EOFError across diverse environments.
Debugging EOFError
When encountering an EOFError, consider the following debugging steps:
- Check Input Source: Ensure that the source of input is correctly configured and providing data.
- Review File Operations: Confirm that file operations are correctly implemented, and the file is opened in the appropriate mode (e.g., read mode).
- Examine Code Logic: Verify that the logical flow of the program does not lead to unexpected calls for input.
By methodically approaching EOFError, developers can effectively troubleshoot and resolve issues related to input handling in their Python applications.
Understanding EOF Errors in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “An EOFError in Python occurs when the input function reaches the end of a file or stream without reading any data. This typically happens when the program expects more input than is provided, signaling that the end of the data has been reached unexpectedly.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “EOFError is particularly common in scenarios involving file handling or user input. Developers should implement error handling techniques to gracefully manage these situations, ensuring that the program does not crash when it encounters an unexpected end of input.”
Sarah Johnson (Data Scientist, Analytics Hub). “In data processing applications, EOFError can indicate that a data stream has been prematurely terminated. Understanding the context in which this error arises is crucial for debugging and can help maintain the integrity of data pipelines.”
Frequently Asked Questions (FAQs)
What is an EOF error in Python?
An EOF (End Of File) error in Python occurs when the interpreter reaches the end of a file or input stream unexpectedly while trying to read data. This often happens in situations where the program expects more input than is provided.
What causes an EOF error in Python?
EOF errors can be caused by various factors, including attempting to read from an empty file, not providing sufficient input data in interactive mode, or improperly handling file operations such as reading beyond the available data.
How can I handle an EOF error in Python?
To handle an EOF error, you can use exception handling with a try-except block. Specifically, catch the EOFError exception to gracefully manage situations where the end of the input is reached unexpectedly.
Can EOF errors occur with file operations in Python?
Yes, EOF errors can occur during file operations if you attempt to read more data than is present in the file. Using methods like `read()`, `readline()`, or `readlines()` without checking the file’s end can lead to such errors.
Is an EOF error the same as an IOError in Python?
No, an EOF error is specifically related to the unexpected end of input or file, while an IOError (or OSError in Python 3) pertains to issues with file operations, such as failing to open a file or reading from a non-existent file.
How can I prevent EOF errors in my Python code?
To prevent EOF errors, ensure that your input sources are correctly populated before reading. Additionally, implement checks to verify the availability of data and utilize exception handling to manage potential EOF scenarios effectively.
An EOF (End Of File) error in Python typically occurs when the interpreter reaches the end of a file while trying to read input but finds that there is no more data available. This error can arise in various contexts, such as when using the `input()` function or reading from files. It is an indication that the program expected more data than was provided, which can lead to unexpected behavior or crashes if not handled properly.
Understanding the EOF error is crucial for effective error handling in Python programming. Developers should anticipate scenarios where input may be incomplete or files may not contain the expected amount of data. Implementing proper checks and exception handling can mitigate the impact of EOF errors, ensuring that programs can gracefully handle such situations without terminating abruptly.
In summary, EOF errors serve as a reminder of the importance of robust input validation and error management in programming. By being aware of the potential for these errors and proactively addressing them, developers can create more resilient applications that provide a better user experience and maintain stability even in the face of unexpected input conditions.
Author Profile
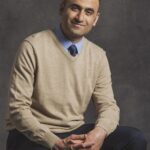
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?