What Exactly is an Instance in Python and Why Does It Matter?
In the world of Python programming, the term “instance” often surfaces in discussions about object-oriented programming (OOP). For both novice and seasoned developers, understanding what an instance is can significantly enhance their ability to harness the full power of Python’s capabilities. An instance is not just a technical term; it embodies the essence of how we create and manipulate objects in our code, allowing us to model real-world entities and behaviors seamlessly. Whether you’re building a simple script or a complex application, grasping the concept of instances will empower you to write cleaner, more efficient, and more organized code.
At its core, an instance in Python refers to a specific realization of a class. When you define a class, you are essentially creating a blueprint for an object, encapsulating its properties and behaviors. An instance is created when you instantiate this class, resulting in a unique object that possesses its own state and can perform actions defined by the class. This distinction between class and instance is fundamental to OOP, enabling developers to create multiple objects from the same class, each with its own unique attributes.
Understanding instances is crucial for leveraging Python’s powerful OOP features, such as inheritance and polymorphism. Instances allow programmers to work with data in a structured way, promoting code reusability and modular
Understanding Instances in Python
In Python, an instance refers to a specific object created from a class. A class serves as a blueprint for creating instances, encapsulating data and functionalities that are common to all objects of that type. Each instance can have its own unique attributes and can utilize the methods defined in its class.
When you instantiate a class, Python allocates memory for the new object and initializes it, allowing you to interact with it. This process is performed using the class name followed by parentheses. For example:
“`python
class Dog:
def bark(self):
return “Woof!”
my_dog = Dog() my_dog is an instance of the Dog class
“`
In this example, `my_dog` is an instance of the `Dog` class. Each instance can have its own state (attributes) and behavior (methods).
Attributes and Methods of Instances
Instances can have attributes and methods, which can be used to store data and define behaviors respectively.
- Attributes: These are variables that hold data specific to an instance. For example, a `Dog` instance could have attributes like `name` and `age`.
- Methods: These are functions defined within a class that describe the behaviors of an instance. They can manipulate instance attributes or perform actions.
Here’s how you might define attributes and methods within a class:
“`python
class Dog:
def __init__(self, name, age):
self.name = name Instance attribute
self.age = age Instance attribute
def bark(self): Instance method
return f”{self.name} says Woof!”
“`
In this example, the `__init__` method is a special method called a constructor, which initializes the attributes of an instance.
Comparison of Instances
Instances can be compared using the `is` and `==` operators. The `is` operator checks for identity (whether two references point to the same object), while the `==` operator checks for equality (whether the values of the instances are the same).
Operator | Description |
---|---|
`is` | Checks if two instances are the same object in memory. |
`==` | Checks if the values of two instances are equal. |
Example:
“`python
dog1 = Dog(“Buddy”, 3)
dog2 = Dog(“Buddy”, 3)
print(dog1 is dog2) , as they are different instances
print(dog1 == dog2) True if the __eq__ method is implemented to compare attributes
“`
Instance Methods vs Class Methods
In Python, there are two primary types of methods: instance methods and class methods.
- Instance Methods: These operate on an instance of the class and can access its attributes and other methods.
- Class Methods: Defined with a decorator `@classmethod`, these operate on the class itself rather than instances. They take `cls` as a parameter instead of `self`.
Example of a class method:
“`python
class Dog:
species = “Canis familiaris” Class attribute
@classmethod
def get_species(cls):
return cls.species
“`
This distinction is crucial for understanding how to effectively utilize classes and instances in Python.
Understanding Instances in Python
In Python, an instance refers to a specific object created from a class. A class serves as a blueprint for creating objects, encapsulating data attributes and methods that define the behavior of those objects. When a class is instantiated, it generates a unique instance that can maintain its own state.
Creating Instances
To create an instance of a class, you typically follow these steps:
- Define a class using the `class` keyword.
- Use the class name followed by parentheses to create an instance.
Example:
“`python
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
my_dog = Dog(“Buddy”, 5)
“`
In the example above, `my_dog` is an instance of the `Dog` class. It has its own `name` and `age` attributes.
Attributes and Methods of Instances
Instances can have their own unique attributes and methods, defined within the class. The `self` parameter in methods refers to the instance itself.
- Attributes: Variables that hold data specific to an instance.
- Methods: Functions defined within a class that can manipulate instance attributes.
Example:
“`python
class Cat:
def __init__(self, name, color):
self.name = name
self.color = color
def meow(self):
return f”{self.name} says meow!”
my_cat = Cat(“Whiskers”, “black”)
print(my_cat.meow()) Output: Whiskers says meow!
“`
Instance vs. Class Attributes
It’s crucial to distinguish between instance attributes and class attributes:
Type | Definition | Example |
---|---|---|
Instance Attribute | Specific to each instance of the class. | `self.name` in `Dog` class |
Class Attribute | Shared across all instances of the class. | `species = “Canine”` in `Dog` class |
Example:
“`python
class Dog:
species = “Canine” Class attribute
def __init__(self, name):
self.name = name Instance attribute
my_dog = Dog(“Buddy”)
print(my_dog.species) Output: Canine
“`
Accessing Instance Attributes
Instance attributes can be accessed using the dot notation. This allows for both retrieving and modifying the attributes.
Example:
“`python
my_dog.age = 6 Modifying instance attribute
print(my_dog.age) Output: 6
“`
Instance Methods
Instance methods allow operations on the instance data. They can take parameters and often manipulate instance attributes.
Example:
“`python
class Car:
def __init__(self, model):
self.model = model
def start_engine(self):
return f”The engine of {self.model} is now running.”
my_car = Car(“Toyota”)
print(my_car.start_engine()) Output: The engine of Toyota is now running.
“`
Conclusion on Instances
Instances are fundamental to object-oriented programming in Python. Understanding how to create and manipulate instances enables developers to build complex applications that model real-world entities effectively.
Understanding Instances in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, an instance refers to a specific object created from a class. Each instance can hold different values for its attributes, allowing for the creation of multiple objects with similar properties but distinct behaviors.”
Michael Thompson (Python Developer Advocate, CodeCraft). “When we talk about instances in Python, we are essentially discussing the tangible manifestations of classes. Each instance is a unique entity that can interact with other instances and methods defined within its class, making object-oriented programming powerful and flexible.”
Sarah Patel (Lead Data Scientist, Data Insights Group). “Understanding instances is crucial for mastering Python’s object-oriented programming paradigm. Instances allow developers to encapsulate data and functionality, fostering code reuse and modularity, which are essential for building scalable applications.”
Frequently Asked Questions (FAQs)
What is an instance in Python?
An instance in Python refers to a specific object created from a class. It represents a concrete occurrence of the class, encapsulating data and behavior defined by that class.
How do you create an instance in Python?
To create an instance in Python, you call the class as if it were a function, using the class name followed by parentheses. For example, `my_instance = MyClass()` creates an instance of `MyClass`.
What is the difference between a class and an instance?
A class is a blueprint for creating objects, defining attributes and methods. An instance is a unique object created from that class, containing its own data and state.
Can you have multiple instances of the same class in Python?
Yes, you can create multiple instances of the same class in Python. Each instance will have its own separate attributes and state, even though they share the same class definition.
What is the purpose of using instances in Python?
Instances allow for the encapsulation of data and functionality, enabling object-oriented programming principles such as inheritance and polymorphism, which facilitate code reusability and organization.
How do you access attributes and methods of an instance in Python?
You access attributes and methods of an instance using the dot notation. For example, `my_instance.attribute` retrieves an attribute, while `my_instance.method()` calls a method defined in the class.
An instance in Python refers to a specific object created from a class. A class serves as a blueprint that defines the properties and behaviors of the objects it can create. When an instance is generated, it inherits the attributes and methods defined in its class, allowing for the encapsulation of data and functionality. This object-oriented programming approach enables developers to create modular, reusable code that can represent real-world entities effectively.
Instances are crucial for managing state and behavior in Python applications. Each instance can maintain its own state through instance variables, which are unique to that specific object. This allows for multiple instances of the same class to exist independently, each with its own set of data. Furthermore, instances can interact with one another through methods, promoting a dynamic and cohesive structure within the code.
In summary, understanding instances in Python is fundamental for leveraging the power of object-oriented programming. By creating instances from classes, developers can encapsulate data and functionality, fostering code reusability and organization. This concept is pivotal for building complex applications, as it allows for the representation of diverse entities and their interactions within a program.
Author Profile
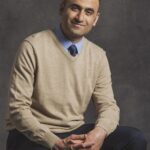
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?