What Is Caching in Python and How Can It Enhance Your Applications?
What is Caching in Python?
In the fast-paced world of software development, efficiency is key, and one powerful technique that developers often turn to is caching. Imagine a scenario where your application repeatedly fetches the same data from a database or performs the same complex computation over and over again. This redundancy not only consumes valuable resources but can also lead to sluggish performance. Enter caching: a strategy designed to store and quickly retrieve frequently accessed data, significantly enhancing the speed and responsiveness of applications. In the realm of Python, caching is not just a buzzword; it’s a vital tool that can transform how your programs operate.
At its core, caching in Python involves storing the results of expensive function calls or data retrieval operations so that subsequent requests for the same data can be served faster. By leveraging various caching mechanisms, developers can minimize the need for repeated calculations or database queries, ultimately leading to a more efficient use of resources. Python offers a range of libraries and frameworks that facilitate caching, making it accessible for developers of all skill levels.
Whether you’re building a web application, a data processing pipeline, or a machine learning model, understanding caching can help you optimize performance and improve user experience. In the following sections, we will delve deeper into the different types of caching available in Python,
Understanding Caching in Python
Caching is a technique used to store a subset of data in a temporary storage area, allowing for quick access to frequently requested information. In Python, caching can significantly enhance the performance of applications by reducing the time taken to retrieve data from slower storage systems, such as databases or remote APIs.
There are various ways to implement caching in Python, ranging from built-in decorators to third-party libraries. The most common methods include:
- Memoization: A specific form of caching used primarily in functions where the results of expensive function calls are stored, and subsequent calls with the same parameters return the cached result.
- File-Based Caching: Storing data in files on disk to persist cache data across program executions.
- In-Memory Caching: Utilizing data structures in memory, like dictionaries, to store cached data for fast access.
Python Caching Techniques
Python provides several options for implementing caching, each with its own use cases and benefits. Below are popular techniques:
Technique | Description | Use Cases |
---|---|---|
Memoization | Caching the results of function calls based on input parameters. | Recursive functions, expensive computations. |
LRU Cache | A built-in decorator that implements a Least Recently Used caching mechanism. | Limiting memory usage while still retaining frequently accessed items. |
Disk Caching | Storing cached data in files on disk. | Large datasets, data that does not change frequently. |
Third-Party Libraries | Using libraries such as `diskcache` or `Flask-Caching` for advanced caching features. | Web applications, complex data retrieval. |
Implementing Caching in Python
To illustrate caching in Python, consider the following examples:
- Using Memoization with `functools.lru_cache`:
“`python
from functools import lru_cache
@lru_cache(maxsize=32)
def fibonacci(n):
if n < 2:
return n
return fibonacci(n-1) + fibonacci(n-2)
```
- Implementing a Simple File-Based Cache:
“`python
import pickle
import os
cache_file = ‘cache.pkl’
def cache_data(data):
with open(cache_file, ‘wb’) as f:
pickle.dump(data, f)
def load_cache():
if os.path.exists(cache_file):
with open(cache_file, ‘rb’) as f:
return pickle.load(f)
return None
“`
- Using a Third-Party Library:
“`python
from diskcache import Cache
cache = Cache(‘/tmp/mycachedir’)
@cache.memoize()
def fetch_data(query):
Simulate an expensive database query
return expensive_database_query(query)
“`
By strategically implementing caching, developers can improve application responsiveness and efficiency, especially in environments where resource-intensive operations are common.
Understanding Caching in Python
Caching in Python refers to the technique of storing the results of expensive function calls and reusing them when the same inputs occur again. This can significantly improve performance by reducing the need to recompute results, particularly in applications where certain computations are repeated frequently.
Types of Caching
Caching can be broadly categorized into several types:
- Memory Caching: Stores data in RAM for quick access.
- Disk Caching: Writes cached data to disk, allowing for persistence beyond program execution but with slower access times compared to memory.
- Distributed Caching: Involves multiple servers to cache data, facilitating scalability and fault tolerance.
Implementing Caching in Python
Python provides several libraries for caching, the most notable being `functools.lru_cache`. This decorator enables caching of function results based on the arguments passed.
Example of using `lru_cache`:
“`python
from functools import lru_cache
@lru_cache(maxsize=128)
def expensive_function(x):
Simulate an expensive computation
return x * x
“`
In this example, results of `expensive_function` will be cached for up to 128 unique inputs.
Benefits of Caching
The advantages of implementing caching are numerous:
- Performance Improvement: Reduces the time taken for repeated calculations.
- Reduced Load: Lowers the burden on the database or API calls by minimizing the frequency of requests.
- Resource Efficiency: Decreases CPU and memory usage by avoiding redundant computations.
Common Caching Libraries in Python
Several libraries are available to facilitate caching in Python, including:
Library | Description |
---|---|
`functools` | Built-in library that provides `lru_cache` decorator. |
`cachetools` | Offers various cache implementations like LRU, TTL, etc. |
`diskcache` | A persistent cache that stores data on disk. |
`Flask-Caching` | Provides caching support for Flask applications. |
Considerations When Using Caching
While caching offers significant benefits, it is essential to consider potential drawbacks:
- Staleness of Data: Cached data may become outdated, leading to inconsistencies.
- Memory Usage: Caching can consume a considerable amount of memory, especially with large datasets.
- Complexity: Introducing caching can complicate code, making it harder to maintain.
Best Practices for Caching
To maximize the effectiveness of caching in Python:
- Identify Expensive Operations: Focus on caching results from functions that are computationally intensive.
- Set Appropriate Expiration: Use time-to-live (TTL) policies to refresh cached data periodically.
- Monitor Cache Performance: Regularly evaluate cache hit rates and memory usage to optimize configurations.
- Use Unique Keys: Ensure that cached data is easily retrievable by employing unique keys for different inputs.
Caching in Python is a powerful technique that can enhance application performance. By understanding its types, benefits, and best practices, developers can effectively implement caching strategies tailored to their specific needs.
Understanding Caching Techniques in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Caching in Python is a powerful technique that significantly enhances application performance by storing frequently accessed data in memory. This reduces the need for repeated computations or database queries, leading to faster response times and improved user experience.
Michael Chen (Lead Data Scientist, Data Solutions Group). In Python, caching can be implemented using various libraries such as `functools.lru_cache` and external solutions like Redis. These tools allow developers to optimize resource usage and streamline data retrieval processes, which is crucial for data-intensive applications.
Sarah Thompson (Python Developer Advocate, Open Source Community). Effective caching strategies in Python not only enhance performance but also help in managing load during peak usage times. By leveraging caching, developers can ensure that their applications remain responsive and scalable, which is vital in today’s fast-paced digital landscape.
Frequently Asked Questions (FAQs)
What is caching in Python?
Caching in Python refers to the technique of storing frequently accessed data in a temporary storage area, known as a cache, to improve data retrieval speed and overall application performance.
How does caching improve performance in Python applications?
Caching reduces the need to repeatedly compute or fetch data from slower storage systems, such as databases or external APIs, thereby decreasing response times and resource consumption.
What are common caching strategies used in Python?
Common caching strategies include in-memory caching (using libraries like `functools.lru_cache`), file-based caching, and distributed caching systems such as Redis or Memcached.
What libraries can be used for caching in Python?
Popular libraries for caching in Python include `functools`, `cachetools`, `diskcache`, and `Flask-Caching`, each offering various features suited for different use cases.
How do you implement caching in a Python function?
You can implement caching in a Python function using decorators, such as `@lru_cache` from the `functools` module, which automatically caches the results of the function based on its input arguments.
What are the potential downsides of caching in Python?
Potential downsides of caching include increased memory usage, stale data if the cache is not invalidated properly, and added complexity in managing cache states and expiration policies.
Caching in Python is a technique that improves the efficiency of applications by temporarily storing the results of expensive function calls and reusing them when the same inputs occur again. This mechanism significantly reduces the time taken for computations, especially in scenarios where functions are called repeatedly with the same parameters. Python provides several built-in libraries and decorators, such as `functools.lru_cache`, which facilitate easy implementation of caching in programs.
One of the key advantages of caching is its ability to enhance performance, particularly in data-intensive applications. By minimizing redundant calculations, caching not only speeds up response times but also reduces the load on system resources. This is particularly beneficial in web applications, where response time is crucial for user experience. Furthermore, caching can be implemented at various levels, including in-memory caching for quick access or persistent caching for longer-term storage.
However, it is essential to manage cache effectively to avoid potential pitfalls such as stale data or excessive memory usage. Developers should consider cache eviction policies and the appropriate cache size based on the specific use case. Additionally, understanding when to cache and when not to cache is vital, as caching may not always yield performance improvements, particularly for functions with high variability in outputs.
In summary, caching
Author Profile
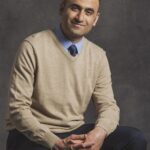
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?