What Is Case in Python? Understanding Its Importance and Usage
In the world of programming, understanding the nuances of language syntax is essential for writing clean, efficient code. One such nuance that often puzzles beginners and even seasoned developers alike is the concept of “case” in Python. Whether you’re crafting variable names, function definitions, or class names, the way you utilize case can significantly impact the readability and maintainability of your code. In this article, we will delve into the intricacies of case in Python, exploring its various forms and the conventions that govern its use.
At its core, case refers to the distinction between uppercase and lowercase letters in identifiers—names given to variables, functions, and classes. Python, like many programming languages, is case-sensitive, meaning that `Variable`, `variable`, and `VARIABLE` are interpreted as three distinct entities. This characteristic underscores the importance of adhering to established naming conventions, which not only enhance code clarity but also foster collaboration among developers.
Moreover, Python embraces several case styles that serve specific purposes and contexts. From the widely recognized snake_case for variable names to the CamelCase convention for class names, each style carries its own significance in the realm of Python programming. As we explore these conventions further, you’ll discover how mastering case can elevate your coding practices and contribute to more professional, readable code. Join
Understanding Case Sensitivity in Python
In Python, case sensitivity refers to the distinction between uppercase and lowercase letters in identifiers such as variable names, function names, and class names. This characteristic is crucial for maintaining clarity and preventing conflicts within the code.
For instance, the following identifiers are considered distinct due to their differing cases:
- `variable`
- `Variable`
- `VARIABLE`
This means that using the same name with different cases can lead to confusion and bugs if not handled carefully. The case sensitivity in Python promotes better coding practices by encouraging the use of clear and consistent naming conventions.
Importance of Naming Conventions
Adhering to naming conventions is essential in Python programming, as it enhances code readability and maintainability. Common conventions include:
- Snake Case: Using lowercase letters and underscores (e.g., `my_variable`).
- Camel Case: Starting with a lowercase letter and capitalizing subsequent words (e.g., `myVariable`).
- Pascal Case: Capitalizing the first letter of each word (e.g., `MyClass`).
Here is a table summarizing these conventions:
Convention | Example | Usage |
---|---|---|
Snake Case | my_variable | Variable names and function names |
Camel Case | myVariable | Variable names (less common in Python) |
Pascal Case | MyClass | Class names |
Following these conventions helps avoid errors that might arise from case sensitivity. For example, if a function is defined using snake case, calling it with camel case will result in a `NameError`.
Case Sensitivity in Built-in Functions and Libraries
Python’s standard library and built-in functions are also case-sensitive. This means that function names must be called with the exact casing defined in the documentation. For example:
- `print()` is valid, while `Print()` will raise an error.
- `len()` works, but `Len()` will not be recognized.
It is important to familiarize oneself with the correct casing of built-in functions to avoid runtime errors.
Best Practices for Managing Case Sensitivity
To effectively manage case sensitivity in Python, consider the following best practices:
- Be Consistent: Choose a naming convention and apply it uniformly throughout your code.
- Avoid Single Character Names: This can lead to confusion and make it difficult to track variable usage.
- Use Descriptive Names: Descriptive identifiers reduce ambiguity and enhance understanding of the code’s purpose.
By implementing these practices, developers can minimize the potential for errors related to case sensitivity while improving the overall quality of their code.
Understanding Case Sensitivity in Python
In Python, case sensitivity refers to the distinction between uppercase and lowercase letters in variable names, function names, class names, and other identifiers. This characteristic is crucial for the language’s syntax and semantics.
- Identifiers: Python treats identifiers with different cases as distinct entities. For example, `variable`, `Variable`, and `VARIABLE` would be recognized as three separate identifiers.
- Keywords: Python’s keywords are case-sensitive and must be written in lowercase. For instance, `if`, `else`, and `while` must be used exactly as defined.
Common Practices for Naming Conventions
To maintain code readability and avoid confusion, Python developers follow specific naming conventions based on case styles. Here are the most common:
Case Style | Description | Example |
---|---|---|
Snake Case | Words are separated by underscores, all lowercase. | `my_variable_name` |
Camel Case | The first letter of each word is capitalized except for the first word. | `myVariableName` |
Pascal Case | Similar to Camel Case but starts with a capital letter. | `MyVariableName` |
Upper Case | All letters are uppercase, typically used for constants. | `MY_CONSTANT` |
Examples of Case Sensitivity
To illustrate case sensitivity, consider the following examples:
“`python
Variable names
name = “Alice”
Name = “Bob”
print(name) Outputs: Alice
print(Name) Outputs: Bob
Function names
def greet():
return “Hello”
def Greet():
return “Hi”
print(greet()) Outputs: Hello
print(Greet()) Outputs: Hi
“`
In this example, the variables `name` and `Name`, as well as the functions `greet()` and `Greet()`, are treated as entirely different due to their casing.
Impact on Code Maintenance
The case sensitivity in Python impacts code maintenance significantly. Developers must be careful to use consistent casing to avoid errors. Here are some considerations:
- Readability: Following consistent casing conventions improves code readability, making it easier for others (and yourself) to understand the code later.
- Avoiding Errors: Mixing cases can lead to runtime errors. For example, referencing a variable as `myVariable` when it is defined as `myvariable` will cause a `NameError`.
- Refactoring: When refactoring code, maintaining consistent naming conventions is vital. Tools and IDEs often help by providing features to rename identifiers while ensuring case consistency.
Conclusion on Case Sensitivity
Understanding and utilizing case sensitivity properly is essential for writing clear, maintainable, and error-free Python code. Adhering to established naming conventions enhances collaboration and minimizes potential mistakes in code interpretation.
Understanding Case Sensitivity in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, the concept of ‘case’ refers to the distinction between uppercase and lowercase letters in variable names, function names, and other identifiers. This case sensitivity means that ‘Variable’, ‘VARIABLE’, and ‘variable’ are recognized as three distinct identifiers, which can lead to errors if not managed carefully.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Understanding case in Python is crucial for maintaining code readability and preventing bugs. Developers must consistently use the same case for identifiers throughout their codebase to ensure that functions and variables are properly recognized and utilized.”
Sarah Thompson (Python Educator, Code Academy). “When teaching Python, I emphasize the importance of case sensitivity to beginners. It is a fundamental aspect of the language that affects how code is interpreted. Misunderstanding this can lead to confusion and frustration, especially for those transitioning from case-insensitive languages.”
Frequently Asked Questions (FAQs)
What is case in Python?
Case in Python refers to the distinction between uppercase and lowercase letters in identifiers, keywords, and string literals. Python is case-sensitive, meaning that variables named `Variable` and `variable` would be treated as different entities.
What are the different types of case conventions in Python?
Common case conventions in Python include:
- Snake_case: All letters are lowercase, with words separated by underscores (e.g., `my_variable`).
- CamelCase: Each word starts with an uppercase letter, with no spaces (e.g., `MyClass`).
- kebab-case: Words are separated by hyphens (not typically used in Python identifiers).
- UPPERCASE: All letters are uppercase, often used for constants (e.g., `MAX_VALUE`).
Why is case sensitivity important in Python?
Case sensitivity is crucial in Python to avoid ambiguity and enhance code readability. It allows developers to create distinct identifiers that can coexist without conflict, thereby promoting better coding practices.
How does case affect string comparisons in Python?
In Python, string comparisons are case-sensitive. For instance, the strings `hello` and `Hello` are considered different. To perform case-insensitive comparisons, functions like `str.lower()` or `str.upper()` can be utilized to normalize the case before comparison.
Can I use mixed case in variable names in Python?
Yes, mixed case can be used in variable names, but it is advisable to follow established naming conventions for readability. For example, using `myVariable` (CamelCase) or `my_variable` (snake_case) enhances clarity and maintains consistency within the codebase.
Are there any restrictions on case usage in Python identifiers?
Yes, identifiers must start with a letter (a-z, A-Z) or an underscore (_), followed by letters, digits (0-9), or underscores. Identifiers cannot begin with a digit, and while they can be of any length, they should avoid using Python reserved keywords.
In Python, the term “case” can refer to several concepts, primarily focusing on the way text is formatted, particularly regarding the capitalization of letters. The most common types of case in programming and text manipulation include lower case, upper case, title case, and sentence case. Understanding these cases is essential for tasks such as string manipulation, data normalization, and ensuring consistency in user input or output.
Lower case refers to all letters being in small letters, while upper case consists of all letters in capital form. Title case capitalizes the first letter of each word, making it useful for formatting titles or headings. Sentence case capitalizes the first letter of the first word in a sentence, which is important for proper grammar in textual outputs. Python provides built-in string methods such as .lower(), .upper(), .title(), and .capitalize() to facilitate these transformations easily.
Moreover, case sensitivity is a critical aspect of Python programming. Variable names and function names in Python are case-sensitive, meaning that ‘Variable’, ‘variable’, and ‘VARIABLE’ would be treated as distinct identifiers. This characteristic can lead to errors if not properly managed, emphasizing the need for careful naming conventions and consistency throughout the codebase.
In summary
Author Profile
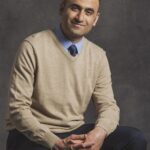
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?