What Does ‘Does Not Equal’ Mean in Python?
In the world of programming, understanding how to compare values is fundamental to writing effective code. Among the various comparison operators available in Python, the concept of “does not equal” plays a crucial role in decision-making processes within your scripts. Whether you’re filtering data, validating user input, or controlling the flow of your program, knowing how to correctly implement this operator can significantly enhance your coding proficiency. This article will delve into the intricacies of the “does not equal” operator in Python, equipping you with the knowledge to use it confidently in your projects.
At the heart of Python’s comparison operators lies the ability to evaluate whether two values are unequal. This operator, often represented in a unique syntax, allows developers to create conditions that dictate the behavior of their programs. Understanding how to leverage this operator not only aids in constructing logical expressions but also helps in debugging and refining code. As we explore its functionality, we will also touch upon common use cases and scenarios where the “does not equal” operator shines.
Moreover, grasping the nuances of this operator can lead to more efficient coding practices. By mastering how to implement and combine it with other logical operators, you can build more complex conditions that enhance the interactivity and responsiveness of your applications. Join us as we unravel the mysteries of
Understanding the Not Equal Operator
In Python, the “not equal” operator is represented by `!=`. This operator is used to compare two values or expressions, returning `True` if they are not equal and “ if they are equal. It is a fundamental part of conditional statements, allowing for effective decision-making within a program.
Usage of the Not Equal Operator
The `!=` operator can be utilized with various data types, including integers, floats, strings, and even complex data structures like lists and dictionaries. Below are some examples demonstrating its application:
- Numerical Comparison:
“`python
a = 5
b = 3
print(a != b) Output: True
“`
- String Comparison:
“`python
str1 = “Hello”
str2 = “World”
print(str1 != str2) Output: True
“`
- List Comparison:
“`python
list1 = [1, 2, 3]
list2 = [1, 2, 3]
print(list1 != list2) Output:
“`
Boolean Context of the Not Equal Operator
When using the not equal operator within conditional statements, it can help control the flow of the program. Here’s how it integrates into an `if` statement:
“`python
value = 10
if value != 5:
print(“Value is not equal to 5.”) This will be printed.
“`
Comparison with Other Operators
The `!=` operator is one of several comparison operators in Python. Here’s a comparison of the key operators:
Operator | Description |
---|---|
== | Equal to |
!= | Not equal to |
> | Greater than |
< | Less than |
>= | Greater than or equal to |
<= | Less than or equal to |
Common Pitfalls
While the `!=` operator is straightforward, developers may encounter some common pitfalls:
- Type Comparison: Comparing different data types can lead to unexpected results.
“`python
print(1 != “1”) Output: True (integer vs. string)
“`
- Floating-Point Precision: Due to the nature of floating-point arithmetic, comparisons may yield unexpected results.
“`python
a = 0.1 + 0.2
print(a != 0.3) Output: True (due to precision issues)
“`
Understanding these aspects of the not equal operator in Python can greatly enhance a programmer’s ability to write effective and efficient code.
Understanding ‘Not Equal’ in Python
In Python, the concept of “not equal” is represented by the `!=` operator. This operator is essential for comparing two values to determine if they are distinct. When using `!=`, the expression evaluates to `True` if the values are not equal and “ if they are equal.
Usage of the ‘!=’ Operator
The `!=` operator can be used with various data types, including:
- Integers
- Floats
- Strings
- Lists
- Dictionaries
- Tuples
Here are some examples demonstrating its use:
“`python
Integer Comparison
a = 5
b = 10
print(a != b) Output: True
Float Comparison
x = 3.14
y = 3.14
print(x != y) Output:
String Comparison
str1 = “hello”
str2 = “world”
print(str1 != str2) Output: True
List Comparison
list1 = [1, 2, 3]
list2 = [1, 2, 3]
print(list1 != list2) Output:
Dictionary Comparison
dict1 = {‘key’: ‘value’}
dict2 = {‘key’: ‘different_value’}
print(dict1 != dict2) Output: True
“`
Comparison with Other Operators
In Python, comparisons can be made using several operators, including:
Operator | Description | Example |
---|---|---|
`==` | Equal to | `a == b` |
`!=` | Not equal to | `a != b` |
`>` | Greater than | `a > b` |
`<` | Less than | `a < b` |
`>=` | Greater than or equal to | `a >= b` |
`<=` | Less than or equal to | `a <= b` |
While `==` checks for equality, `!=` specifically checks for inequality. This distinction is crucial for conditional statements and loops.
Common Use Cases
The `!=` operator is frequently used in various programming scenarios, such as:
- Conditional Statements: To execute certain blocks of code based on inequality.
“`python
if user_input != “exit”:
print(“Continue working…”)
“`
- Loops: To iterate until a condition is met.
“`python
while response != “yes”:
response = input(“Do you want to continue? “)
“`
- Filtering Data: To filter out elements that do not match a specific criterion.
“`python
numbers = [1, 2, 3, 4, 5]
filtered_numbers = [num for num in numbers if num != 3]
“`
Conclusion of Usage
Understanding the `!=` operator is fundamental for effective programming in Python. It enables developers to create dynamic and responsive applications by allowing for straightforward comparisons between variables and data structures.
Understanding the Concept of Inequality in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, the operator ‘!=’ is used to determine if two values are not equal. This operator is fundamental in control flow statements, allowing developers to implement logic based on differing conditions.”
Michael Chen (Python Developer Advocate, CodeMaster). “The ‘!=’ operator in Python is not just a simple comparison; it also respects the data types of the values being compared. For instance, comparing a string with an integer will yield a ‘True’ result, indicating they are not equal, which is crucial for type safety in programming.”
Lisa Patel (Data Scientist, AI Analytics Group). “Understanding how ‘!=’ works in Python is essential for data manipulation and analysis. It allows for filtering datasets effectively, ensuring that only the relevant data points are processed based on specific criteria.”
Frequently Asked Questions (FAQs)
What does “does not equal” mean in Python?
In Python, “does not equal” is represented by the operator `!=`, which is used to compare two values or expressions, returning `True` if they are not equal and “ otherwise.
How is the “does not equal” operator used in conditional statements?
The `!=` operator can be used in conditional statements, such as `if` statements, to execute a block of code when two values are not equal. For example: `if a != b: print(“a is not equal to b”)`.
Can “does not equal” be used with different data types in Python?
Yes, the `!=` operator can compare values of different data types. Python will attempt to convert the values to a common type for comparison, but it may lead to unexpected results or a “ return if the types are fundamentally incompatible.
What is the difference between “does not equal” and “not equal” in Python?
There is no difference; “does not equal” and “not equal” refer to the same operator `!=` in Python. Both terms are used interchangeably to describe the operation of comparing two values for inequality.
Are there any alternative ways to check for inequality in Python?
Yes, besides using `!=`, you can also use the `is not` keyword to check if two variables do not refer to the same object in memory. However, this is not the same as checking for value inequality.
What happens if I compare two objects with “does not equal” that are of different types?
When comparing two objects of different types using `!=`, Python will return `True` if the values are not considered equal. For example, `1 != “1”` will return `True`, as an integer and a string are not equal.
In Python, the concept of inequality is represented by the operator `!=`, which is used to determine whether two values are not equal to each other. This operator can be applied to various data types, including integers, strings, lists, and more. When using `!=`, Python evaluates the operands on either side of the operator and returns a Boolean value: `True` if the values are not equal and “ if they are equal. This functionality is essential for control flow in programming, allowing developers to implement conditional logic based on the comparison of values.
It is important to note that Python’s `!=` operator is versatile and can handle comparisons between different data types. However, when comparing values of different types, Python follows specific rules that may lead to unexpected results. For instance, comparing a string to an integer will always yield `True`, as Python considers them inherently different. Thus, understanding the behavior of the `!=` operator in various contexts is crucial for writing effective and error-free code.
In summary, the `!=` operator is a fundamental component of Python’s syntax for expressing inequality. It plays a critical role in decision-making processes within programs. Developers should be aware of its behavior across different data types to avoid
Author Profile
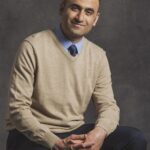
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?