What Does the Double Slash in Python Mean?
In the world of Python programming, clarity and precision are paramount, especially when it comes to understanding syntax and operators. Among the various symbols and operators that Python offers, the double slash (`//`) often raises questions for both novice and seasoned programmers alike. This seemingly simple operator holds significant importance in the realm of arithmetic operations, particularly when it comes to division. But what exactly does it do, and how can it enhance your coding experience? In this article, we will delve into the nuances of the double slash in Python, unraveling its purpose and applications to empower you in your programming journey.
At its core, the double slash operator is a powerful tool that performs floor division, a concept that might be unfamiliar to those accustomed to traditional division. Unlike standard division, which can yield floating-point results, floor division rounds down the result to the nearest whole number. This characteristic makes the double slash particularly useful in scenarios where integer results are essential, such as in algorithms that require counting or indexing. Understanding how and when to use this operator can streamline your code and improve its efficiency.
Moreover, the double slash operator is not just a mathematical tool; it also plays a crucial role in various programming contexts, from data manipulation to algorithm design. As we explore its functionalities, we will uncover practical examples
Understanding Double Slash in Python
In Python, the double slash operator (`//`) is used for floor division. This operator divides two numbers and returns the largest integer less than or equal to the quotient. This can be particularly useful when dealing with integer division where a non-integer result is not desired.
For example:
- `7 // 2` results in `3`, because `3.5` is floored to `3`.
- `8 // 4` results in `2`, as the division is exact.
- `-3 // 2` results in `-2`, demonstrating that floor division rounds towards negative infinity.
The double slash operator can be applied to both integers and floating-point numbers. It is essential to note that when both operands are floating-point numbers, the result will still be a float:
- `7.0 // 2` yields `3.0`
- `7 // 2.0` yields `3.0`
Comparison with Single Slash
To better understand the distinction, it’s beneficial to compare the double slash (`//`) with the single slash (`/`), which performs standard division and returns a float:
Operator | Example | Result |
---|---|---|
`/` | `7 / 2` | `3.5` |
`//` | `7 // 2` | `3` |
`//` | `-3 // 2` | `-2` |
`/` | `-3 / 2` | `-1.5` |
This table clearly illustrates how the behavior of each operator differs, especially in handling negative numbers and fractional results.
Practical Applications
The double slash operator is commonly used in scenarios where integer results are necessary, such as:
- Indexing: When calculating the middle index of a list or array, you want to ensure the result is an integer.
- Pagination: In applications that require dividing data into pages, floor division can help determine how many items fit on a page.
- Mathematical computations: When implementing algorithms that require integer results, such as in graphics programming or certain simulations.
By leveraging the double slash operator, developers can ensure that their calculations yield the expected integer results without needing to manually handle rounding or type conversion.
Understanding Double Slash in Python
The double slash (`//`) operator in Python is primarily utilized for floor division. Floor division divides two numbers and rounds down the result to the nearest whole number. This operator is particularly useful when one needs to ensure that the result of a division is an integer, discarding any fractional part.
Usage of Double Slash
The syntax for using the double slash operator is straightforward:
“`python
result = a // b
“`
Here, `a` and `b` are the operands. The division is performed, and the result is floored to the nearest integer.
Examples of Double Slash
- Basic Example:
“`python
print(5 // 2) Output: 2
print(5.0 // 2) Output: 2.0
“`
- Negative Numbers:
The behavior of floor division with negative numbers is noteworthy:
“`python
print(-5 // 2) Output: -3
print(-5.0 // 2) Output: -3.0
“`
In the above examples, notice how the result is always rounded down towards the more negative integer.
Comparison with Regular Division
To illustrate the difference between regular division (`/`) and floor division (`//`), consider the following table:
Operation | Example | Result |
---|---|---|
Regular | `5 / 2` | `2.5` |
Floor | `5 // 2` | `2` |
Regular | `-5 / 2` | `-2.5` |
Floor | `-5 // 2` | `-3` |
Regular division returns a floating-point number, while floor division always yields an integer result, effectively rounding down.
Type of Result
The type of the result from floor division depends on the types of the operands:
- If both operands are integers, the result is an integer.
- If either operand is a float, the result is a float.
This behavior can be summarized as follows:
Operand Types | Result Type |
---|---|
`int` & `int` | `int` |
`float` & `float` | `float` |
`int` & `float` | `float` |
`float` & `int` | `float` |
Practical Applications
The double slash operator is commonly employed in various scenarios, including but not limited to:
- Indexing: When determining the index for data structures.
- Pagination: Calculating the number of pages needed when displaying items.
- Game Development: For grid calculations, ensuring player or object positions align correctly.
Utilizing floor division can enhance code clarity and correctness in these contexts, ensuring predictable outcomes.
Understanding the Significance of Double Slash in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). The double slash operator in Python, represented as `//`, is known as the floor division operator. It performs division and returns the largest integer less than or equal to the result, effectively discarding any fractional component. This is particularly useful in scenarios where integer results are necessary, such as in algorithms that require whole number outputs.
Michael Chen (Lead Software Engineer, CodeCraft Solutions). The double slash operator is essential for developers working with data types that require precision in integer calculations. Unlike the regular division operator, which can lead to floating-point results, the double slash ensures that the output remains an integer, making it a vital tool in applications that rely on discrete values, such as indexing or counting items.
Sarah Thompson (Python Educator, LearnPython.org). Understanding the double slash operator is crucial for beginners in Python programming. It not only simplifies the process of obtaining integer results but also helps in grasping the concept of floor division, which can be a common requirement in mathematical computations. Teaching this operator early on can enhance a learner’s ability to handle numerical data effectively.
Frequently Asked Questions (FAQs)
What is double slash in Python?
The double slash (`//`) in Python is the operator for floor division. It divides two numbers and returns the largest integer less than or equal to the result.
How does double slash differ from single slash?
The single slash (`/`) performs standard division and returns a float, while the double slash (`//`) performs floor division and returns an integer.
Can double slash be used with negative numbers?
Yes, double slash can be used with negative numbers. It still performs floor division, which means it rounds down towards negative infinity.
What is the output of 5 // 2 in Python?
The output of `5 // 2` is `2`, as it returns the largest integer less than or equal to the division result.
Is double slash applicable to both integers and floats?
Yes, double slash can be used with both integers and floats. The result will be an integer if both operands are integers, and a float if at least one operand is a float.
How can I convert the result of double slash to a float?
You can convert the result of double slash to a float by using the `float()` function. For example, `float(5 // 2)` will return `2.0`.
The double slash in Python, represented as `//`, is known as the floor division operator. It is used to divide two numbers and return the largest integer less than or equal to the quotient. This operator is particularly useful when one needs to perform division while discarding any fractional part, thereby simplifying the result to an integer value. For example, using `5 // 2` would yield `2`, as it rounds down the result of the division.
Another important aspect of the double slash is its behavior with negative numbers. When using floor division with negative operands, the result is still rounded down towards negative infinity. For instance, `-5 // 2` results in `-3`, as it moves to the next lower integer. This characteristic distinguishes floor division from standard division, which may lead to confusion if not properly understood.
In summary, the double slash in Python serves a critical function in mathematical operations, particularly in scenarios where integer results are required. Understanding its behavior with both positive and negative numbers is essential for accurate calculations. Developers and programmers should leverage this operator to ensure their code handles division appropriately, especially when working with integer values.
Author Profile
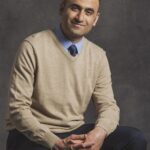
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?