What Is the ‘end’ Parameter in Python and How Does It Work?
In the world of programming, every language has its unique quirks and features that set it apart. Python, known for its simplicity and readability, is no exception. Among its many built-in functionalities, the concept of “end” plays a pivotal role in how we interact with output and manage data presentation. Whether you’re a seasoned developer or a newcomer to the coding realm, understanding the nuances of “end” in Python can enhance your coding experience and streamline your output processes. This article delves into what “end” signifies in Python, exploring its applications and implications in various programming scenarios.
When we talk about “end” in Python, we are primarily referring to a parameter that modifies the behavior of the print function. This seemingly simple feature can significantly impact how data is displayed on the console. By default, the print function concludes its output with a newline character, but the “end” parameter allows developers to customize this behavior, enabling them to create more dynamic and user-friendly outputs. This flexibility is particularly useful in scenarios where formatting and presentation are key, such as in data visualization or user interfaces.
Moreover, the concept of “end” extends beyond just the print function. It invites programmers to think creatively about how they present information and interact with users. As we explore
Understanding the `end` Parameter in Python
In Python, the `end` parameter is primarily used in the `print()` function to determine what is printed at the end of the output. By default, the `print()` function adds a newline character (`\n`) after each call, which means that subsequent print statements will appear on a new line. However, this behavior can be modified using the `end` parameter to suit various formatting needs.
The syntax of the `print()` function with the `end` parameter is as follows:
“`python
print(value, …, sep=’ ‘, end=’\n’, file=None, flush=)
“`
Where:
- `value`: The value(s) to be printed.
- `sep`: A string inserted between values, defaulting to a space.
- `end`: A string appended after the last value, defaulting to a newline.
- `file`: An optional file-like object (default is the current sys.stdout).
- `flush`: A boolean specifying whether to forcibly flush the stream.
Examples of Using `end`
Here are some practical examples of how the `end` parameter can be utilized:
- Default Behavior:
“`python
print(“Hello”)
print(“World”)
“`
Output:
“`
Hello
World
“`
- Custom End Character:
“`python
print(“Hello”, end=”, “)
print(“World”)
“`
Output:
“`
Hello, World
“`
- No Space or Newline:
“`python
for i in range(5):
print(i, end=’ ‘)
“`
Output:
“`
0 1 2 3 4
“`
Common Use Cases
The `end` parameter is useful in various scenarios, including:
- Creating formatted output, such as generating a single line of output from multiple print statements.
- Building progress indicators in loops where you might want to update the same line rather than printing new lines.
Practical Table of `end` Parameter Usages
Use Case | Example Code | Output |
---|---|---|
Default Newline | print("First Line") |
First Line Second Line |
Comma Separation | print("A", end=", ") |
A, B |
Single Line Output | for i in range(3): |
0 1 2 |
Updating Progress | for i in range(5): |
Progress: 80% |
Utilizing the `end` parameter effectively can enhance the readability and presentation of output in Python scripts, allowing for more control over how data is displayed to the user.
Understanding the `end` Parameter in Python
In Python, the `end` parameter is primarily associated with the `print()` function. By default, the `print()` function appends a newline character (`\n`) after printing its output. The `end` parameter allows you to specify what should be printed at the end of the output, providing more control over formatting and output behavior.
Default Behavior of `print()`
The default behavior of the `print()` function can be observed as follows:
“`python
print(“Hello”)
print(“World”)
“`
Output:
“`
Hello
World
“`
In this example, each call to `print()` ends with a newline, resulting in each string appearing on a separate line.
Using the `end` Parameter
The `end` parameter can be customized to change what follows the printed output. For instance:
“`python
print(“Hello”, end=”, “)
print(“World”)
“`
Output:
“`
Hello, World
“`
Here, the first `print()` call uses `end=”, “` to add a comma and a space instead of a newline, resulting in both strings appearing on the same line.
Common Use Cases for the `end` Parameter
The `end` parameter can be useful in various scenarios:
- Creating Custom Delimiters:
“`python
print(“Item 1″, end=” | “)
print(“Item 2″, end=” | “)
print(“Item 3”)
“`
Output:
“`
Item 1 | Item 2 | Item 3
“`
- Progress Indicators:
“`python
for i in range(5):
print(f”Progress: {i * 20}%”, end=”\r”)
“`
This example shows how to overwrite the same line in the terminal.
- Formatted Output:
“`python
print(“Loading”, end=””)
for _ in range(3):
print(“.”, end=””, flush=True)
print(” Done!”)
“`
Output (with a slight delay):
“`
Loading… Done!
“`
Additional Notes on the `end` Parameter
- Type: The `end` parameter accepts a string. If an invalid type is provided, it will raise a `TypeError`.
- Flushing Output: Combining `end` with the `flush` parameter can help control when output appears, particularly in loops.
- Compatibility: The `end` parameter is available in Python 3.x. In Python 2.x, `print` is a statement rather than a function, so it does not support the `end` parameter directly.
Comparison of `end` with Other Print Parameters
Parameter | Purpose | Default Value |
---|---|---|
`sep` | Specifies the separator between multiple items | `’ ‘` (space) |
`end` | Specifies what to print at the end of the output | `’\n’` (newline) |
`file` | Specifies the file where the output is sent | `sys.stdout` |
`flush` | Determines whether to flush the output buffer immediately | “ |
The `end` parameter enhances the flexibility of the `print()` function, allowing for tailored output suitable for diverse applications.
Understanding the Role of `end` in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). The `end` parameter in Python’s print function is crucial for controlling how output is terminated. By default, print adds a newline character at the end of its output. However, using the `end` parameter allows developers to customize this behavior, enabling the creation of more complex output formats and improving user experience in console applications.
Michael Chen (Lead Software Engineer, CodeCraft Solutions). The flexibility of the `end` parameter in Python’s print function cannot be overstated. It allows for seamless integration of outputs without unnecessary line breaks, which is particularly useful in scenarios where multiple outputs need to be displayed on the same line. This feature enhances the readability of the output and provides a more polished interface for users.
Sarah Johnson (Python Educator, LearnPython.org). Understanding the `end` parameter is essential for beginners in Python programming. It serves as an excellent to the concept of function parameters and how they can modify the behavior of built-in functions. By experimenting with different values for `end`, learners can gain a deeper appreciation for Python’s versatility and its approach to output formatting.
Frequently Asked Questions (FAQs)
What is `end` in Python?
The `end` parameter in Python is used in the `print()` function to specify what should be printed at the end of the output. By default, it is set to a newline character (`\n`), but it can be changed to any string.
How can I change the default `end` value in Python?
You can change the default `end` value by passing a different string to the `end` parameter in the `print()` function. For example, `print(“Hello”, end=”, “)` will output “Hello, ” without moving to a new line.
Can I use `end` with multiple print statements?
Yes, you can use the `end` parameter with multiple print statements to control how the outputs are separated. For instance, `print(“Hello”, end=”, “)` followed by `print(“World!”)` will result in “Hello, World!” on the same line.
What happens if I set `end` to an empty string?
If you set `end` to an empty string (`end=””`), the next output will be printed immediately after the current output without any space or newline in between.
Is the `end` parameter specific to the `print()` function?
Yes, the `end` parameter is specific to the `print()` function in Python. It is not a general feature of the language but rather a customizable aspect of how output is formatted in print statements.
Can I use special characters with the `end` parameter?
Yes, you can use special characters with the `end` parameter. For example, you can use `end=”\t”` for a tab space or `end=”\n\n”` for two new lines, allowing for flexible formatting of output.
In Python, the term “end” is primarily associated with the `print()` function, where it serves as a parameter that determines what is printed at the end of the output. By default, the `print()` function adds a newline character (`\n`) after printing the specified content. However, the `end` parameter allows developers to customize this behavior by specifying a different string or character to be appended instead of the newline. This feature enhances the flexibility of output formatting in Python, enabling more control over how data is presented to users.
Another context in which “end” appears is in the realm of control flow statements, particularly in loops and conditional statements. While Python does not explicitly use the keyword “end” to denote the termination of these structures, the concept of an endpoint is inherent in the way Python manages indentation and code blocks. Each block of code is clearly defined by its indentation level, which eliminates the need for explicit end markers, making the code cleaner and more readable.
In summary, the concept of “end” in Python is multifaceted, primarily relating to output formatting through the `print()` function and the structural integrity of code blocks. Understanding how to manipulate the `end` parameter can significantly improve the presentation of
Author Profile
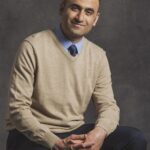
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?