What Is an Identifier in Python and Why Is It Important?
In the world of programming, the ability to name and identify elements within your code is crucial for clarity and functionality. This is where the concept of identifiers comes into play, especially in a versatile language like Python. Whether you’re a novice coder or a seasoned developer, understanding what identifiers are and how they function can significantly enhance your coding experience. From variables and functions to classes and modules, identifiers serve as the building blocks that allow you to communicate your intentions to the Python interpreter and other programmers alike.
Identifiers in Python are essentially names that you give to various entities in your code, enabling you to reference them easily. They are fundamental to writing clear and effective code, as they help convey the purpose of different components within your program. In Python, identifiers must adhere to specific rules and conventions, which not only ensure that your code runs smoothly but also improve its readability and maintainability.
As we delve deeper into the topic, we will explore the characteristics of valid identifiers, the significance of naming conventions, and common pitfalls to avoid. By the end of this article, you’ll have a solid understanding of how to effectively use identifiers in Python, empowering you to write cleaner, more efficient code.
Understanding Identifiers in Python
Identifiers in Python are names used to identify variables, functions, classes, modules, and other objects. They serve as a means to reference these entities within the code. Understanding the rules and conventions surrounding identifiers is crucial for writing clean and effective Python code.
Rules for Naming Identifiers
When creating identifiers in Python, several rules must be followed:
- Character Set: Identifiers can consist of letters (both uppercase and lowercase), digits (0-9), and underscores (_). However, they must begin with a letter or an underscore, not a digit.
- Case Sensitivity: Python is case-sensitive, meaning that `myVariable`, `MyVariable`, and `MYVARIABLE` are considered three distinct identifiers.
- Reserved Keywords: Identifiers cannot be the same as Python’s reserved keywords (e.g., `if`, `else`, `for`, `while`, `def`, `class`, etc.). Using a reserved keyword as an identifier will result in a syntax error.
Here is a summary of the identifier rules:
Rule | Description |
---|---|
Start Character | Must start with a letter or underscore |
Allowed Characters | Letters, digits, and underscores |
Case Sensitivity | Identifiers are case-sensitive |
Keywords | Cannot use reserved keywords as identifiers |
Naming Conventions
While Python allows a wide range of identifier names, following naming conventions can greatly enhance code readability. Here are some common conventions:
- Snake Case: Use lowercase letters and underscores to separate words (e.g., `my_variable`).
- Camel Case: Capitalize the first letter of each word except the first (e.g., `myVariable`).
- Pascal Case: Capitalize the first letter of each word, including the first (e.g., `MyVariable`).
In addition to these styles, it’s important to choose descriptive names that convey the purpose of the identifier. This practice helps others (and yourself) understand the code better.
Examples of Identifiers
Here are some examples of valid and invalid identifiers in Python:
- Valid Identifiers:
- `my_variable`
- `data123`
- `_privateVariable`
- `calculateArea`
- Invalid Identifiers:
- `2ndValue` (starts with a digit)
- `my-variable` (contains a hyphen)
- `class` (reserved keyword)
By adhering to the rules and conventions outlined above, you can create identifiers that contribute to clear and maintainable Python code.
Definition of an Identifier in Python
An identifier in Python is a name used to identify a variable, function, class, module, or any other object. It serves as a label that allows programmers to reference these entities within their code. Identifiers are essential for creating readable and maintainable code.
Rules for Naming Identifiers
Identifiers must adhere to specific rules:
- Characters Allowed: Identifiers can consist of letters (both uppercase and lowercase), digits (0-9), and underscores (_).
- Starting Character: An identifier must begin with a letter or an underscore; it cannot start with a digit.
- Case Sensitivity: Identifiers are case-sensitive, meaning `myVariable` and `myvariable` are considered different identifiers.
- No Special Characters: Special characters such as @, , $, %, etc., are not permitted in identifiers.
- Length: There is no explicit limit on the length of identifiers, but it is advisable to keep them concise and meaningful.
Reserved Keywords
Certain words in Python are reserved keywords and cannot be used as identifiers. These keywords have predefined meanings in the language. Here is a list of some common reserved keywords:
Keyword | Description |
---|---|
“ | Boolean value indicating |
`None` | Represents a null value |
`True` | Boolean value indicating true |
`and` | Logical AND operator |
`as` | Used for creating aliases |
`assert` | Used for debugging purposes |
`class` | Defines a new class |
`def` | Defines a new function |
`if` | Conditional statement |
`import` | Imports a module |
`return` | Exits a function and returns a value |
Best Practices for Choosing Identifiers
Selecting appropriate identifiers is crucial for code clarity. Here are some best practices:
- Descriptive Names: Use meaningful names that convey the purpose of the variable or function. For example, use `calculate_area` instead of `ca`.
- Consistent Naming Conventions: Follow a consistent naming convention throughout your code. Common conventions include:
- snake_case: Use underscores between words (e.g., `my_variable`).
- camelCase: Capitalize the first letter of each word except the first (e.g., `myVariable`).
- Avoid Single Character Names: Except for counters or iterators in small scopes, avoid using single-letter identifiers.
- Use of Prefixes/Suffixes: For specific types of identifiers, consider using prefixes or suffixes (e.g., `is_` for boolean variables like `is_active`).
Examples of Valid and Invalid Identifiers
To illustrate the rules and practices, here are examples of valid and invalid identifiers:
Valid Identifiers | Invalid Identifiers |
---|---|
`my_variable` | `2ndVariable` |
`name` | `my-variable` |
`_temp` | `class` |
`MAX_SIZE` | `my variable` |
`getData()` | `get@Data` |
Identifying and adhering to these conventions helps ensure that Python code is both functional and easily understandable to others.
Understanding Identifiers in Python: Expert Insights
Dr. Emily Carter (Computer Science Professor, Tech University). “In Python, an identifier is a name used to identify a variable, function, class, or module. It is crucial to follow naming conventions, as identifiers must begin with a letter or an underscore and can be followed by letters, digits, or underscores.”
James Liu (Senior Software Engineer, Code Innovations). “Identifiers in Python are case-sensitive, meaning ‘Variable’ and ‘variable’ would be considered two distinct identifiers. This sensitivity can lead to subtle bugs if not managed carefully, especially in larger codebases.”
Sarah Thompson (Python Developer Advocate, Open Source Community). “Choosing meaningful identifiers is essential for code readability and maintainability. Good identifiers convey the purpose of the variable or function, making it easier for others to understand the code’s intent.”
Frequently Asked Questions (FAQs)
What is an identifier in Python?
An identifier in Python is a name used to identify a variable, function, class, module, or other objects. It is a user-defined name that helps in distinguishing one entity from another in the code.
What are the rules for naming identifiers in Python?
Identifiers in Python must start with a letter (a-z, A-Z) or an underscore (_), followed by letters, digits (0-9), or underscores. They cannot contain spaces or special characters and are case-sensitive.
Can identifiers in Python start with a digit?
No, identifiers cannot start with a digit. They must begin with a letter or an underscore to be considered valid.
Are there any reserved keywords that cannot be used as identifiers in Python?
Yes, Python has reserved keywords such as `if`, `else`, `while`, `for`, `class`, and `def`, among others. These keywords have special meanings in the language and cannot be used as identifiers.
What is the maximum length of an identifier in Python?
While Python does not impose a specific limit on the length of identifiers, it is recommended to keep them reasonably short for readability. However, they can technically be as long as the implementation allows.
Can identifiers in Python contain special characters?
No, identifiers cannot contain special characters other than the underscore (_). Special characters like @, , $, %, etc., are not permitted in identifiers.
In Python, an identifier is a name used to identify a variable, function, class, module, or other objects. Identifiers are essential for writing clear and understandable code, as they allow programmers to refer to their data and functions meaningfully. Python identifiers must follow specific rules: they can consist of letters, digits, and underscores, but must begin with a letter or an underscore. Additionally, Python is case-sensitive, meaning that ‘Variable’ and ‘variable’ would be considered different identifiers.
It is crucial to avoid using reserved words or keywords in Python as identifiers, as these have predefined meanings in the language. Good practices for naming identifiers include using descriptive names that convey the purpose of the variable or function, adhering to naming conventions such as snake_case for variables and functions, and CamelCase for class names. This practice enhances code readability and maintainability, making it easier for developers to understand and collaborate on projects.
In summary, understanding identifiers in Python is fundamental for effective programming. By following the rules and best practices for naming identifiers, developers can create code that is not only functional but also clear and easy to navigate. This attention to detail contributes significantly to the overall quality of the code and facilitates better teamwork and project management.
Author Profile
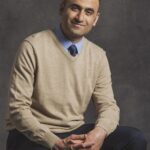
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?