What Is Indentation in Python and Why Is It So Important?
Understanding Indentation in Python
In Python, indentation is a crucial part of the syntax. Unlike many programming languages that use braces or keywords to define blocks of code, Python relies on whitespace to determine the structure and flow of the code. This feature makes Python code visually clean and easy to read, but it also requires developers to be meticulous about consistent indentation.
Importance of Indentation
The significance of indentation in Python can be summarized as follows:
- Block Definition: Indentation is used to define the body of loops, functions, and classes. Each block of code that follows a statement (like `if`, `for`, `while`, `def`, etc.) must be indented.
- Syntax Requirements: Failure to adhere to proper indentation will result in `IndentationError`, which interrupts program execution.
- Readability: Consistent indentation enhances code readability, allowing developers to quickly understand the structure and flow of the code.
How Indentation Works
Indentation in Python typically involves:
- Spaces vs. Tabs: Developers can use either spaces or tabs for indentation, but it is recommended to use spaces for consistency. The Python community typically advocates for using four spaces per indentation level.
- Mixed Indentation: Using a combination of tabs and spaces is discouraged, as it can lead to confusion and errors. Python 3 does not allow mixing the two and will raise an error if detected.
Indentation Type | Usage |
---|---|
Spaces | Four spaces are the standard practice in the Python community. |
Tabs | Can be used, but should be avoided to maintain consistency. |
Examples of Indentation
Here are a few examples to illustrate how indentation is applied in various contexts:
– **Function Definition**:
“`python
def greet(name):
print(“Hello, ” + name + “!”)
“`
– **Conditional Statement**:
“`python
if age >= 18:
print(“You are an adult.”)
else:
print(“You are a minor.”)
“`
- Loop Structure:
“`python
for i in range(5):
print(i)
if i % 2 == 0:
print(“Even number”)
“`
In these examples, notice how the code blocks are indented to show their relationship to the control structures they belong to.
Common Indentation Errors
Errors related to indentation can hinder development. Some common issues include:
- IndentationError: Raised when indentation levels are inconsistent.
- TabError: Raised when mixing tabs and spaces is detected.
To avoid these errors, adhere to the following best practices:
- Use a consistent number of spaces (preferably four) for each indentation level.
- Configure your text editor to insert spaces when the tab key is pressed.
- Regularly check your code for mixed indentation.
By following these guidelines, developers can leverage the power of indentation in Python effectively and avoid common pitfalls associated with code formatting.
Understanding Indentation in Python: Perspectives from Programming Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Indentation in Python is not merely a stylistic choice; it is a fundamental aspect of the language’s syntax that defines code blocks. Unlike many other programming languages that use braces or keywords, Python relies on whitespace to determine the grouping of statements, which can significantly enhance code readability and maintainability.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “The importance of indentation in Python cannot be overstated. It enforces a clean and consistent structure in code, which is crucial for both new and experienced developers. Proper indentation helps prevent logical errors that can arise from misaligned code blocks, making it essential for effective debugging and collaboration.”
Sarah Thompson (Python Educator and Author, LearnPythonNow). “For beginners, understanding indentation in Python is often one of the first hurdles. However, mastering this concept is vital as it directly affects how the program executes. Teaching students the significance of consistent indentation helps them appreciate Python’s design philosophy of simplicity and clarity.”
Frequently Asked Questions (FAQs)
What is indentation in Python?
Indentation in Python refers to the spaces or tabs used at the beginning of a line to define the structure and flow of the code. It is crucial for indicating blocks of code, such as those used in functions, loops, and conditionals.
Why is indentation important in Python?
Indentation is essential in Python because it determines the grouping of statements. Unlike many programming languages that use braces or keywords, Python relies on indentation to define the scope of loops, functions, and classes, thereby enhancing code readability and maintainability.
How does indentation affect code execution in Python?
Improper indentation can lead to syntax errors or unexpected behavior in code execution. Python will raise an `IndentationError` if the indentation is inconsistent, and incorrect indentation can change the logical flow of the program.
What are the common practices for indentation in Python?
Common practices include using four spaces per indentation level, avoiding mixing tabs and spaces, and maintaining consistent indentation throughout the code. Following these guidelines helps ensure code clarity and prevents errors.
Can I use tabs instead of spaces for indentation in Python?
While Python allows the use of tabs for indentation, it is recommended to use spaces for consistency and compatibility. The Python community widely adopts the PEP 8 style guide, which advocates for using four spaces per indentation level.
What happens if I mix tabs and spaces in Python indentation?
Mixing tabs and spaces can lead to `TabError`, causing Python to throw an error due to inconsistent indentation. It is advisable to choose one method and stick to it throughout your code to avoid such issues.
Indentation in Python is a fundamental aspect of the language’s syntax that dictates the structure and flow of the code. Unlike many programming languages that use braces or keywords to define blocks of code, Python relies solely on whitespace to determine the grouping of statements. This means that consistent indentation is crucial for the correct execution of Python programs, as it directly affects how the interpreter understands the relationships between different code blocks, such as loops, conditionals, and function definitions.
The significance of indentation in Python extends beyond mere aesthetics; it serves as a means of enhancing code readability and maintainability. By enforcing a clear and uniform structure, Python encourages developers to write clean and organized code. This practice not only aids in collaborative efforts but also facilitates debugging and future code modifications. As a result, understanding and adhering to proper indentation practices is essential for anyone working with Python.
In summary, indentation is not just a stylistic choice in Python; it is a critical component of the language’s design that influences both functionality and readability. Developers must be vigilant in maintaining consistent indentation to avoid syntax errors and ensure their code executes as intended. By embracing this aspect of Python, programmers can contribute to a more efficient and comprehensible coding environment.
Author Profile
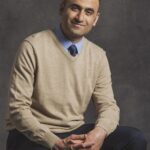
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?