What is .items() in Python and How Can You Use It Effectively?
In the world of Python programming, understanding the nuances of data structures is crucial for efficient coding and data manipulation. Among these structures, dictionaries stand out for their versatility and ease of use. One of the key features that enhances the functionality of dictionaries is the `.items()` method. This seemingly simple tool holds the power to unlock a wealth of information about the key-value pairs stored within a dictionary, making it an essential component for any Python developer’s toolkit. Whether you’re a novice coder or a seasoned programmer, grasping the significance of `.items()` can elevate your coding skills and streamline your data handling processes.
The `.items()` method is a built-in function that returns a view object displaying a list of a dictionary’s key-value pairs as tuples. This allows developers to iterate over the dictionary efficiently, providing a straightforward way to access both keys and their corresponding values simultaneously. By leveraging this method, programmers can simplify their code and enhance readability, which is particularly beneficial when working with complex datasets or performing operations that require both keys and values.
Additionally, the use of `.items()` extends beyond mere iteration. It plays a vital role in various data manipulation tasks, such as filtering, transforming, and aggregating data. Understanding how to effectively utilize this method can lead to more elegant solutions and improved performance
Understanding .items() in Python
The `.items()` method is a built-in function available for dictionary objects in Python. It provides a convenient way to access the key-value pairs stored within a dictionary. When called, it returns a view object that displays a list of a dictionary’s key-value tuple pairs. This functionality is particularly useful when you need to iterate over the dictionary or when you want to perform operations that involve both keys and values.
How .items() Works
When you invoke the `.items()` method on a dictionary, it does not create a new list of tuples; rather, it provides a dynamic view that reflects changes in the dictionary. The syntax for using `.items()` is straightforward:
“`python
dictionary.items()
“`
Here, `dictionary` is the dictionary object you are working with. The method returns a view object that can be converted into a list if needed.
Example of Using .items()
Consider the following example to illustrate how `.items()` can be utilized:
“`python
sample_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
for key, value in sample_dict.items():
print(f”Key: {key}, Value: {value}”)
“`
In this example, the output will be:
“`
Key: a, Value: 1
Key: b, Value: 2
Key: c, Value: 3
“`
Benefits of Using .items()
- Efficient Iteration: The `.items()` method allows for efficient iteration over key-value pairs without needing to access keys and values separately.
- Dynamic View: Since it provides a view, any modifications made to the dictionary will be reflected in the output of `.items()`.
- Ease of Use: The method simplifies the code needed to process both keys and values simultaneously.
Table: Comparison of Dictionary Methods
Method | Description | Returns |
---|---|---|
.keys() | Returns a view of all the keys in the dictionary. | View object of keys. |
.values() | Returns a view of all the values in the dictionary. | View object of values. |
.items() | Returns a view of the dictionary’s key-value pairs. | View object of tuples (key, value). |
Common Use Cases
The `.items()` method is particularly useful in scenarios such as:
- Data Processing: When parsing JSON or other nested data structures where you need to access both keys and values.
- Filtering: Creating filtered views of data based on specific criteria involving both keys and values.
- Aggregation: Summarizing or aggregating data by iterating through keys and values.
By employing the `.items()` method, programmers can write cleaner and more efficient code, enhancing both readability and maintainability.
Understanding `.items()` in Python
The `.items()` method is a built-in function associated with dictionaries in Python. It returns a view object that displays a list of a dictionary’s key-value pairs as tuples. This method is particularly useful for iterating over dictionaries in a more readable and structured manner.
Usage of `.items()`
To utilize the `.items()` method, you can invoke it on a dictionary object. The syntax is straightforward:
“`python
dictionary.items()
“`
Here, `dictionary` is the variable name of your dictionary. The method will return a view object that can be converted to a list or used directly in loops.
Example of `.items()`
Consider the following example:
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
Using .items() to iterate over the dictionary
for key, value in my_dict.items():
print(f’Key: {key}, Value: {value}’)
“`
Output:
“`
Key: a, Value: 1
Key: b, Value: 2
Key: c, Value: 3
“`
In this example, the `.items()` method allows for a clean and concise way to access both keys and values of the dictionary simultaneously.
Characteristics of `.items()`
- Returns a view object: The result of `.items()` is a view that reflects any changes made to the dictionary. If the dictionary is updated, the view will reflect these changes dynamically.
- Iterable: The view object returned by `.items()` can be iterated over, making it easy to loop through the key-value pairs.
- Memory Efficient: Unlike creating a list of tuples, the view object is a lightweight representation that does not consume additional memory for storing the tuples.
Common Use Cases
The `.items()` method is frequently used in various programming scenarios, including:
- Data Processing: When working with configurations or settings stored in dictionaries.
- Merging Dictionaries: Facilitating the combination of multiple dictionaries by iterating over key-value pairs.
- Data Analysis: In data science applications, `.items()` can be employed to analyze frequency distributions or other statistical data.
Comparison with Other Dictionary Methods
Method | Description |
---|---|
`.keys()` | Returns a view object of all keys in the dictionary. |
`.values()` | Returns a view object of all values in the dictionary. |
`.items()` | Returns a view object of key-value pairs (tuples). |
Each of these methods serves distinct purposes, and choosing the appropriate one depends on whether you need keys, values, or both.
Conclusion on `.items()`
The `.items()` method is an essential tool for effective dictionary manipulation in Python, providing a clear and efficient means to access and process key-value pairs. Understanding its functionality is crucial for any Python programmer looking to leverage dictionary data structures effectively.
Understanding the Use of .items() in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The .items() method in Python is essential for iterating over key-value pairs in dictionaries. It provides a clear and efficient way to access both the keys and values simultaneously, which is particularly useful in data manipulation and analysis.”
Mark Thompson (Python Developer, Data Solutions Group). “Using the .items() method enhances readability and performance when working with dictionaries. It allows developers to unpack dictionary items directly in loops, making the code cleaner and more Pythonic.”
Linda Zhao (Data Scientist, AI Research Lab). “In data science applications, the .items() method is invaluable for transforming and aggregating data. It enables quick access to both keys and values, facilitating efficient data processing and analysis workflows.”
Frequently Asked Questions (FAQs)
What is .items() in Python?
The `.items()` method in Python is used with dictionaries to return a view object that displays a list of a dictionary’s key-value tuple pairs.
How do you use .items() in a for loop?
You can iterate over a dictionary using `.items()` in a for loop by unpacking the key-value pairs. For example: `for key, value in my_dict.items():` allows access to both the key and its corresponding value.
What is the return type of .items()?
The `.items()` method returns a view object, specifically a `dict_items` object, which reflects the dictionary’s items and updates dynamically as the dictionary changes.
Can .items() be used with other data types besides dictionaries?
No, the `.items()` method is specific to dictionary objects in Python. Other data types do not have this method.
How does .items() improve code readability?
Using `.items()` enhances code readability by clearly indicating that both keys and values are being accessed, which makes the intent of the code more explicit compared to accessing keys and values separately.
Is .items() available in Python 2.x?
In Python 2.x, `.items()` is available but returns a list of tuples instead of a view object. In Python 3.x, it returns a view object that is more memory efficient.
The `.items()` method in Python is a versatile function primarily used with dictionaries. It returns a view object that displays a list of a dictionary’s key-value pairs as tuples. This method is particularly useful for iterating over the elements of a dictionary, allowing developers to access both keys and values simultaneously in a clean and efficient manner.
One of the significant advantages of using `.items()` is its ability to facilitate operations that require both keys and values. For example, when processing data stored in a dictionary, developers can easily unpack the key-value pairs within loops, making the code more readable and maintainable. Additionally, the view object returned by `.items()` reflects changes made to the dictionary, ensuring that the most current data is always available during iteration.
Moreover, the `.items()` method can enhance performance in certain scenarios. Since it returns a view rather than a list, it consumes less memory and allows for more efficient iteration, especially with large dictionaries. This efficiency is crucial in applications where performance is a key consideration, such as data analysis or real-time processing.
In summary, the `.items()` method is an essential tool in Python for working with dictionaries. It simplifies the process of accessing key-value pairs, improves code clarity
Author Profile
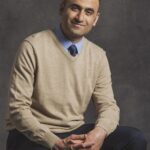
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?