What Does It Mean for an Object to Be Mutable in Python?
In the world of programming, understanding how data structures behave is crucial for writing efficient and effective code. Among the many languages available, Python stands out for its simplicity and versatility, yet it also introduces some nuanced concepts that can trip up even seasoned developers. One such concept is mutability. Have you ever wondered what it means for an object to be mutable in Python? This fundamental characteristic not only influences how data is stored and manipulated but also impacts performance, memory usage, and even the logic of your programs.
At its core, mutability refers to an object’s ability to be changed after it has been created. In Python, this concept is pivotal, as it distinguishes between mutable and immutable types. Mutable objects, such as lists and dictionaries, can be altered in place, allowing for dynamic changes during program execution. On the other hand, immutable objects, like tuples and strings, cannot be modified once they are created, leading to different considerations when it comes to data handling and manipulation.
Understanding mutability is essential for any Python programmer, as it influences how you approach problem-solving and data management. By grasping the implications of mutable and immutable types, you can write more robust code, avoid common pitfalls, and leverage Python’s capabilities to their fullest. In the sections that follow, we
Understanding Mutable Types
In Python, mutable types are those that allow modification after their creation. This means that you can change, add, or remove elements from these data structures without creating a new object. Mutable types are crucial for certain programming tasks, especially when you need to maintain state or modify collections dynamically.
Common mutable types in Python include:
- Lists: Ordered collections that can contain items of different types.
- Dictionaries: Key-value pairs that allow for dynamic insertion and deletion of items.
- Sets: Unordered collections of unique elements that support mathematical set operations.
Characteristics of Mutable Objects
Mutable objects have several key characteristics:
- In-place Modification: You can change the contents without affecting the identity of the object.
- Dynamic Sizing: Many mutable types can grow or shrink in size as elements are added or removed.
- Shared References: If a mutable object is passed to a function or assigned to a new variable, any changes made to the object will reflect across all references.
Examples of Mutable Types
To illustrate the properties of mutable types, consider the following examples with lists and dictionaries:
“`python
Example with a list
my_list = [1, 2, 3]
my_list.append(4) Adding an item
print(my_list) Output: [1, 2, 3, 4]
Example with a dictionary
my_dict = {‘a’: 1, ‘b’: 2}
my_dict[‘c’] = 3 Adding a new key-value pair
print(my_dict) Output: {‘a’: 1, ‘b’: 2, ‘c’: 3}
“`
Mutable vs Immutable Types
Understanding the difference between mutable and immutable types is vital. Immutable types, such as strings, tuples, and frozensets, do not allow modification after creation. This distinction can impact performance and behavior in Python programs.
Type | Mutable | Example |
---|---|---|
List | Yes | [1, 2, 3] |
Dictionary | Yes | {‘a’: 1, ‘b’: 2} |
Set | Yes | {1, 2, 3} |
String | No | “hello” |
Tuple | No | (1, 2, 3) |
Performance Considerations
Mutable types can lead to performance benefits when dealing with large datasets, as they avoid the overhead of creating new objects for every modification. However, caution must be exercised to avoid unintended side effects due to shared references.
When using mutable objects, consider:
- Cloning: If you need to work on a copy without affecting the original, ensure you create a deep copy using the `copy` module.
- Thread Safety: Mutable objects may not be thread-safe, requiring synchronization mechanisms when accessed from multiple threads.
By understanding the properties and behaviors of mutable types, you can leverage their flexibility while avoiding common pitfalls associated with mutable objects in Python.
Understanding Mutability in Python
In Python, mutability refers to the ability of an object to be changed after it is created. This characteristic significantly influences how data structures behave and interact within the language.
Mutable vs. Immutable Types
Python categorizes its data types into two primary groups: mutable and immutable.
Mutable Types:
- Lists: Collections of items that can be modified. Items can be added, removed, or changed.
- Dictionaries: Key-value pairs that allow changes to both keys and values.
- Sets: Unordered collections of unique items that can be altered.
Immutable Types:
- Tuples: Fixed collections of items that cannot be changed once created.
- Strings: Sequences of characters that do not allow modification of individual characters.
- Frozensets: Immutable versions of sets, which cannot be altered after creation.
Implications of Mutability
The distinction between mutable and immutable types has significant implications in programming:
- Performance: Mutability can lead to performance improvements due to the ability to modify existing objects rather than creating new ones.
- Hashability: Only immutable objects can be hashed, which means they can serve as keys in dictionaries. Mutable objects cannot be hashed and thus cannot be used as dictionary keys.
Type | Mutable/Immutable | Example |
---|---|---|
List | Mutable | `[1, 2, 3]` |
Dictionary | Mutable | `{‘key’: ‘value’}` |
Set | Mutable | `{1, 2, 3}` |
Tuple | Immutable | `(1, 2, 3)` |
String | Immutable | `”hello”` |
Frozenset | Immutable | `frozenset([1, 2, 3])` |
Examples of Mutable Operations
To illustrate mutability, consider the following examples with lists and dictionaries:
List Operations:
“`python
my_list = [1, 2, 3]
my_list.append(4) Adding an element
my_list[0] = 10 Modifying an element
“`
Dictionary Operations:
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict[‘c’] = 3 Adding a new key-value pair
my_dict[‘a’] = 10 Updating an existing value
“`
Conclusion on Mutability
Understanding the concept of mutability in Python is crucial for effective programming. It influences data manipulation strategies, memory management, and the overall design of data structures within applications. Mastering when to use mutable versus immutable types can lead to more efficient and effective coding practices.
Understanding Mutability in Python: Expert Insights
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, mutability refers to the ability of an object to be changed after it has been created. Mutable objects, such as lists and dictionaries, allow for modifications like adding or removing elements, which can significantly impact performance and memory management in larger applications.”
Michael Chen (Software Engineer, CodeMaster Solutions). “Understanding which data types are mutable is crucial for effective programming in Python. Mutable types, like sets and lists, can lead to unintended side effects if not handled carefully, especially when passed as arguments to functions. This can result in bugs that are difficult to trace.”
Sarah Johnson (Data Scientist, Analytics Hub). “When working with mutable objects in Python, it is essential to consider how they interact with immutable types, such as tuples and strings. This knowledge not only aids in writing cleaner code but also enhances the efficiency of data manipulation tasks, especially in data-heavy applications.”
Frequently Asked Questions (FAQs)
What is mutable in Python?
Mutable refers to objects in Python that can be changed or modified after their creation. Examples include lists, dictionaries, and sets.
What are some examples of mutable objects in Python?
Common examples of mutable objects are lists, dictionaries, sets, and byte arrays. These objects allow for modifications such as adding, removing, or changing elements.
How do mutable objects differ from immutable objects in Python?
Mutable objects can be altered without creating a new object, while immutable objects, such as tuples and strings, cannot be changed once created. Any modification to an immutable object results in the creation of a new object.
Can mutable objects lead to unexpected behavior in Python?
Yes, mutable objects can lead to unexpected behavior, particularly when they are shared between functions or passed as arguments. Changes made to the object in one location can affect its state elsewhere in the program.
How can I check if an object is mutable in Python?
You can determine if an object is mutable by attempting to modify it. If the operation succeeds without raising an error, the object is mutable. Alternatively, you can refer to Python documentation for specific object types.
Are there any performance considerations when using mutable objects?
Yes, mutable objects can have performance implications, especially in memory management and copying. Modifications to large mutable objects can lead to increased memory usage and slower performance compared to immutable objects.
In Python, mutability refers to the ability of an object to be changed after its creation. Mutable objects can be modified in place, allowing for alterations to their content without needing to create a new object. Common examples of mutable types in Python include lists, dictionaries, and sets. These data structures allow for operations such as adding, removing, or changing elements, which distinguishes them from immutable types like tuples and strings that cannot be altered once created.
Understanding the concept of mutability is crucial for effective programming in Python. It influences how data is stored, passed around, and manipulated within a program. For instance, when mutable objects are passed to functions, any modifications made within the function will affect the original object, which can lead to unintended side effects if not managed carefully. In contrast, immutable objects maintain their state, providing a level of safety against accidental changes.
Key takeaways include the importance of choosing the appropriate data type based on the requirements of mutability. Developers should consider whether they need to modify an object after its creation and select mutable or immutable types accordingly. Additionally, understanding mutability can enhance performance and memory management in Python applications, as mutable objects can be more efficient in certain scenarios due to their ability to be modified without
Author Profile
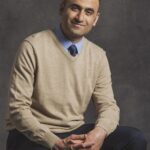
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?