What is a NameError in Python and How Can You Fix It?
If you’ve ever dabbled in Python programming, you may have encountered the dreaded `NameError`. This seemingly innocuous error can bring your code to a screeching halt, leaving you scratching your head and wondering what went wrong. Understanding `NameError` is crucial for any aspiring Python developer, as it not only highlights the importance of variable management but also serves as a gateway to mastering debugging techniques. In this article, we will unravel the mystery behind `NameError`, explore its common causes, and equip you with the tools to resolve it effectively.
Overview
At its core, a `NameError` in Python occurs when the interpreter encounters a name that it doesn’t recognize. This can happen for various reasons, such as using a variable that hasn’t been defined, misspelling a variable name, or attempting to access a function that hasn’t been imported. Each of these scenarios can lead to confusion, especially for beginners who are still getting accustomed to the syntax and structure of Python.
Understanding the nuances of `NameError` is essential for improving your coding skills. By learning how to identify and fix this error, you’ll not only enhance your problem-solving abilities but also gain a deeper appreciation for Python’s dynamic nature. As we delve deeper into this topic, we will
Understanding NameError in Python
In Python, a `NameError` occurs when the interpreter encounters a name that is not defined in the current scope. This error typically arises when a variable or function is referenced before it has been assigned a value or declared. It indicates that the Python interpreter cannot find the name you are trying to use.
The most common scenarios that lead to a `NameError` include:
- Misspelling the name of a variable or function.
- Attempting to use a variable that has not been initialized.
- Using a function that has not been defined yet.
- Referring to variables in a different scope where they are not accessible.
Common Causes of NameError
To further illustrate the causes of `NameError`, consider the following examples:
- Misspelled Variable Name
“`python
my_variable = 10
print(my_varible) NameError: name ‘my_varible’ is not defined
“`
- Using a Variable Before Declaration
“`python
print(my_variable) NameError: name ‘my_variable’ is not defined
my_variable = 10
“`
- Function
“`python
my_function() NameError: name ‘my_function’ is not defined
def my_function():
print(“Hello, World!”)
“`
- Scope Issues
“`python
def my_function():
local_var = 5
print(local_var) NameError: name ‘local_var’ is not defined
“`
How to Resolve NameError
Resolving a `NameError` involves identifying the specific cause of the error and correcting it. Here are some strategies:
- Check Spelling: Always verify the spelling of variable and function names.
- Initialize Variables: Ensure that variables are initialized before they are used.
- Define Functions Before Use: Always define functions before calling them in your code.
- Scope Awareness: Be mindful of the scope of variables, especially in functions and classes.
Here’s a summary of how to address `NameError` effectively:
Cause | Solution |
---|---|
Misspelled Name | Correct the spelling |
Variable Not Initialized | Initialize the variable before usage |
Function Not Defined | Define the function before calling it |
Scope Issue | Ensure the variable is accessible in the required scope |
By following these guidelines, developers can effectively troubleshoot and resolve `NameError` occurrences, leading to a smoother coding experience.
Understanding NameError in Python
NameError is a specific type of exception in Python that occurs when the code references a variable or function name that has not been defined in the current scope. This exception serves as a signal to the developer that there is an issue with the naming of variables or functions within the code.
Common Causes of NameError
Several situations can lead to a NameError:
- Variables: Attempting to use a variable before it is assigned a value.
- Misspelling: Typos in variable or function names that are defined elsewhere in the code.
- Scope Issues: Accessing a variable that is outside the current scope, such as trying to access a local variable from an outer function.
- Import Errors: Failing to import a module correctly or referencing a function or variable from a module that hasn’t been imported.
- Deleted Variables: Trying to access a variable that has been deleted or not yet initialized.
Examples of NameError
Below are some examples illustrating common scenarios that lead to NameError.
“`python
Example 1: Using an variable
print(x) This will raise NameError: name ‘x’ is not defined
Example 2: Misspelling a variable name
my_variable = 10
print(my_varible) This will raise NameError: name ‘my_varible’ is not defined
Example 3: Scope issues
def my_function():
y = 5
my_function()
print(y) This will raise NameError: name ‘y’ is not defined
Example 4: Import errors
import math
print(math.sqrt(16)) Correct usage
print(math.squrt(16)) This will raise NameError: name ‘squrt’ is not defined
“`
How to Handle NameError
When encountering a NameError, several strategies can be employed to resolve the issue:
- Check Variable Definitions: Ensure that all variables are defined before they are used.
- Review Spelling: Double-check the spelling of variable and function names.
- Scope Awareness: Understand the scope of variables, ensuring that you are accessing them within the correct context.
- Import Statements: Verify that all necessary modules are imported and that the imported names are correctly referenced.
- Use Try-Except Blocks: Implement error handling to manage NameErrors gracefully.
“`python
try:
print(x)
except NameError as e:
print(f”An error occurred: {e}”)
“`
Best Practices to Avoid NameError
To minimize the chances of encountering a NameError, consider the following best practices:
- Consistent Naming Conventions: Use clear and consistent naming for variables and functions.
- Commenting and Documentation: Document your code to keep track of variable usage and scope.
- Use of IDEs: Leverage Integrated Development Environments (IDEs) that provide syntax highlighting and error detection.
- Code Reviews: Engage in code reviews to catch potential naming issues early in the development process.
By adhering to these practices, developers can significantly reduce the occurrence of NameErrors in their Python code.
Understanding NameError in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A NameError in Python occurs when the interpreter encounters a name that it does not recognize. This typically happens when a variable is referenced before it has been assigned a value or if there is a typo in the variable name.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “To effectively troubleshoot a NameError, developers should check for common mistakes such as misspellings, incorrect variable scopes, and ensuring that all necessary imports are correctly executed before usage.”
Sarah Johnson (Python Educator, LearnPython.org). “Understanding the context in which a NameError arises is crucial for new programmers. It not only helps in debugging but also reinforces the importance of variable management and naming conventions in coding practices.”
Frequently Asked Questions (FAQs)
What is a NameError in Python?
A NameError in Python occurs when the interpreter encounters a name that is not defined in the current scope. This typically happens when a variable or function is referenced before it has been assigned a value or declared.
What causes a NameError in Python?
Common causes of a NameError include misspelling variable names, using variables that have not been initialized, or attempting to access variables from a different scope where they are not defined.
How can I fix a NameError in my Python code?
To fix a NameError, ensure that all variable names are spelled correctly, check that variables are initialized before use, and verify that the variable is accessible in the current scope.
Can a NameError occur with built-in functions?
Yes, a NameError can occur with built-in functions if they are misspelled or if the function is not imported correctly. For example, calling `printt(“Hello”)` instead of `print(“Hello”)` will raise a NameError.
Are there any tools to help identify NameErrors in Python?
Yes, integrated development environments (IDEs) like PyCharm or code linters such as Pylint can help identify potential NameErrors by providing real-time feedback and highlighting names in the code.
What is the difference between NameError and UnboundLocalError?
A NameError indicates that a variable is not defined in the current scope, while an UnboundLocalError occurs when a local variable is referenced before it has been assigned a value within a function.
A NameError in Python occurs when the interpreter encounters a name that it does not recognize. This typically happens when a variable or function is referenced before it has been defined or if there is a typo in the name. For instance, if you attempt to print a variable that has not been assigned a value, Python will raise a NameError, indicating that the name is not defined in the current scope. Understanding the context of variable scope and initialization is crucial to avoiding such errors.
Key takeaways include the importance of defining all variables and functions before use, as well as ensuring that names are spelled correctly. Additionally, being mindful of the scope of variables—whether they are local or global—can help prevent NameErrors. Utilizing tools like linters or IDEs with error-checking capabilities can also assist in identifying potential NameErrors before runtime.
In summary, a NameError is a common exception in Python that signifies an issue with names. By adhering to best practices in variable declaration and scope management, developers can significantly reduce the occurrence of this error in their code. Continuous learning and attention to detail are essential for effective programming in Python.
Author Profile
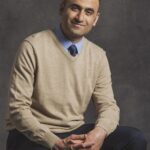
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?