What Is Parse in Python? Understanding Its Role and Importance
In the world of programming, the ability to interpret and manipulate data is paramount, and Python offers a robust set of tools to accomplish just that. One of the fundamental concepts that every Python developer should grasp is parsing. Whether you’re working with strings, files, or complex data structures, understanding how to parse information effectively can streamline your workflow and enhance your application’s functionality. In this article, we will delve into the intricacies of parsing in Python, exploring its significance, various techniques, and practical applications.
Parsing in Python refers to the process of analyzing a string or data structure to extract meaningful information or convert it into a more usable format. This is particularly useful when dealing with data formats such as JSON, XML, or even plain text, where the raw data often needs to be transformed into a structured form for further manipulation. By mastering parsing techniques, developers can efficiently handle data input and output, enabling them to create applications that are not only functional but also user-friendly.
Moreover, Python provides an array of libraries and built-in functions designed to simplify the parsing process. From the powerful `json` module for handling JSON data to the `xml.etree.ElementTree` for XML parsing, these tools empower developers to tackle a variety of data formats with ease. As we explore the different aspects of
Understanding Parsing in Python
Parsing in Python refers to the process of analyzing a string of symbols, either in natural language or computer languages, and converting it into a format that is understandable by the program. This is a crucial step in data processing, allowing for the manipulation and analysis of structured data. Python provides various libraries and tools that facilitate parsing tasks, making it easier for developers to extract meaningful information from complex data formats.
Common Parsing Libraries
Python offers several libraries that are widely used for parsing different data formats:
- json: For parsing JSON (JavaScript Object Notation) data, which is commonly used in web APIs.
- xml.etree.ElementTree: For parsing XML (eXtensible Markup Language) documents.
- csv: For handling Comma-Separated Values (CSV) files, which are often used for tabular data.
- Beautiful Soup: For parsing HTML and XML documents, particularly useful for web scraping.
- re: The regular expression module for parsing strings based on patterns.
Each library is tailored for specific data formats, providing functions that make it easier to read, manipulate, and write data.
Example of JSON Parsing
To illustrate parsing in Python, consider the following example using the `json` library. The code snippet demonstrates how to parse a JSON string into a Python dictionary.
“`python
import json
Sample JSON string
json_string = ‘{“name”: “Alice”, “age”: 30, “city”: “New York”}’
Parsing the JSON string
data = json.loads(json_string)
Accessing parsed data
print(data[‘name’]) Output: Alice
print(data[‘age’]) Output: 30
“`
In this example, the `json.loads()` function is utilized to convert the JSON string into a Python dictionary, allowing for easy access to the values.
Parsing Structured Text with Regular Expressions
Regular expressions (regex) are a powerful way to parse structured text data. They allow users to define search patterns to extract specific information from strings. Here’s a simple example:
“`python
import re
Sample text
text = “Contact: [email protected]”
Regular expression to extract email
pattern = r'[\w\.-]+@[\w\.-]+’
Searching for the email
match = re.search(pattern, text)
if match:
print(match.group()) Output: [email protected]
“`
This code uses the `re` module to find an email address in a given string. The regex pattern specifies the structure of the email, demonstrating how flexible and effective regex can be for parsing tasks.
Table: Comparison of Parsing Libraries
Library | Data Format | Use Case |
---|---|---|
json | JSON | Web APIs, Configuration Files |
xml.etree.ElementTree | XML | Data Interchange, Configuration |
csv | CSV | Tabular Data, Spreadsheets |
Beautiful Soup | HTML/XML | Web Scraping |
re | Strings | Pattern Matching, Text Processing |
This table summarizes the key parsing libraries available in Python, their associated data formats, and typical use cases, providing a quick reference for developers.
Understanding Parsing in Python
Parsing in Python refers to the process of analyzing and converting input data into a format that a program can easily manipulate or understand. This can involve breaking down strings, files, or other data types into more manageable components, enabling the extraction of meaningful information.
Common Parsing Techniques
Python offers various libraries and methods for parsing different types of data:
- String Parsing: Utilizing built-in string methods like `.split()`, `.join()`, and regular expressions for manipulating and extracting information from strings.
- File Parsing: Reading and interpreting structured data from files, such as CSV, JSON, XML, and HTML, using libraries like `csv`, `json`, `xml.etree.ElementTree`, and `BeautifulSoup`.
- Command-Line Argument Parsing: Managing command-line inputs with libraries such as `argparse`, which allows for easy handling of user inputs.
Example: Parsing JSON Data
JSON (JavaScript Object Notation) is a popular data interchange format. Python’s `json` module makes it straightforward to parse JSON data.
“`python
import json
Sample JSON string
json_string = ‘{“name”: “Alice”, “age”: 30, “city”: “New York”}’
Parsing JSON string into a Python dictionary
parsed_data = json.loads(json_string)
Accessing data
print(parsed_data[‘name’]) Output: Alice
“`
Regular Expressions for Advanced Parsing
Regular expressions (regex) are powerful tools for pattern matching and parsing complex strings. Python provides the `re` module for this purpose.
- Basic Functions:
- `re.match()`: Checks for a match only at the beginning of the string.
- `re.search()`: Searches the entire string for a match.
- `re.findall()`: Returns all occurrences of the pattern in a string.
“`python
import re
text = “Contact us at [email protected] or [email protected]”
emails = re.findall(r'[\w\.-]+@[\w\.-]+’, text)
print(emails) Output: [‘[email protected]’, ‘[email protected]’]
“`
Parsing CSV Files
CSV (Comma-Separated Values) files are widely used for data storage. Python’s `csv` module simplifies reading and writing CSV files.
Function | Description |
---|---|
`csv.reader()` | Reads a CSV file and returns a reader object. |
`csv.writer()` | Writes data to a CSV file. |
“`python
import csv
Reading a CSV file
with open(‘data.csv’, newline=”) as csvfile:
reader = csv.reader(csvfile)
for row in reader:
print(row)
Writing to a CSV file
with open(‘output.csv’, ‘w’, newline=”) as csvfile:
writer = csv.writer(csvfile)
writer.writerow([‘name’, ‘age’])
writer.writerow([‘Bob’, 25])
“`
Using BeautifulSoup for HTML Parsing
BeautifulSoup is a library used for parsing HTML and XML documents. It provides Pythonic idioms for iterating, searching, and modifying the parse tree.
“`python
from bs4 import BeautifulSoup
html_doc = “
”
soup = BeautifulSoup(html_doc, ‘html.parser’)
Extracting the title
print(soup.title.string) Output: Test
“`
Parsing in Python is a versatile skill that enables developers to work with various types of data effectively. By leveraging built-in libraries and modules, one can efficiently extract, manipulate, and analyze data to suit specific needs.
Understanding Parsing in Python: Expert Insights
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). Parsing in Python refers to the process of analyzing a string of symbols, either in natural language or in programming languages, to extract meaningful information. It is a fundamental skill for data manipulation, allowing developers to convert data formats and extract relevant data from text.
Michael Chen (Lead Software Engineer, CodeCraft Solutions). In Python, parsing is often achieved using libraries such as `json` for JSON data, `xml.etree.ElementTree` for XML, and `BeautifulSoup` for HTML. These tools simplify the extraction of structured data from unstructured sources, making it easier for developers to work with various data formats.
Lisa Patel (Technical Writer, Python Programming Journal). Understanding how to parse data in Python is crucial for any aspiring programmer. It allows for the manipulation and transformation of data, which is essential in applications ranging from web scraping to data analysis. Mastery of parsing techniques can significantly enhance a developer’s ability to handle real-world data challenges.
Frequently Asked Questions (FAQs)
What is parse in Python?
Parsing in Python refers to the process of analyzing a string of symbols, either in natural language or computer languages, to extract meaningful data or convert it into a structured format. This often involves breaking down the input into components that can be easily manipulated.
What libraries are commonly used for parsing in Python?
Common libraries for parsing in Python include `json` for JSON data, `xml.etree.ElementTree` for XML data, and `BeautifulSoup` for HTML parsing. Additionally, `argparse` is used for parsing command-line arguments.
How do you parse JSON data in Python?
To parse JSON data in Python, you can use the `json` library. You can load a JSON string into a Python dictionary using `json.loads()` or read from a JSON file using `json.load()`.
What is the difference between parsing and serialization in Python?
Parsing is the process of interpreting and converting data into a usable format, while serialization is the process of converting an object into a format that can be easily stored or transmitted, such as converting a Python object into a JSON string.
Can you parse command-line arguments in Python?
Yes, Python provides the `argparse` module, which allows you to define and parse command-line arguments easily. This module helps in creating user-friendly command-line interfaces.
What is the role of regular expressions in parsing?
Regular expressions (regex) are powerful tools for parsing strings in Python. They allow for pattern matching and extraction of specific substrings from text, making it easier to validate and manipulate data based on defined patterns.
In Python, parsing refers to the process of analyzing a string of symbols, either in natural language or computer languages, to extract meaningful information. This process is crucial when dealing with data formats such as JSON, XML, or even simple text files. Python offers various libraries and built-in functions that facilitate parsing, making it easier for developers to manipulate and extract data efficiently.
Key libraries for parsing in Python include `json` for JSON data, `xml.etree.ElementTree` for XML parsing, and `re` for regular expressions. Each of these tools provides specific functionalities that cater to different parsing needs. Understanding how to utilize these libraries effectively can significantly enhance a programmer’s ability to handle diverse data formats and improve overall data processing workflows.
Moreover, parsing is not limited to structured data. It also plays a vital role in natural language processing (NLP), where it helps in understanding and interpreting human language. By leveraging parsing techniques, developers can build applications that can analyze text, extract sentiments, and even generate responses based on user input.
In summary, mastering parsing in Python is essential for any developer working with data. It enables the extraction of valuable insights from various data formats, thus enhancing the capability to build robust applications
Author Profile
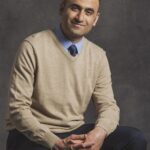
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?