What Is Parsing in Python? Understanding Its Importance and Applications
### Introduction
In the world of programming, the ability to interpret and manipulate data is a cornerstone skill that underpins countless applications. Among the various languages available, Python stands out for its simplicity and versatility, making it a favorite among developers. One of the essential processes in Python that enables this data manipulation is parsing. But what exactly does parsing entail, and why is it so crucial in the realm of programming? In this article, we will delve into the intricacies of parsing in Python, exploring its significance, methods, and applications that can elevate your coding projects to new heights.
Parsing in Python refers to the process of analyzing a string of symbols, either in natural language or in programming code, to extract meaningful information from it. This process is vital for transforming unstructured data into a format that can be easily understood and utilized by programs. Whether you’re dealing with JSON files, XML documents, or even simple text, parsing allows you to break down complex data into manageable components, facilitating tasks such as data validation, extraction, and transformation.
The beauty of parsing in Python lies in its rich ecosystem of libraries and tools designed to streamline this process. From built-in modules like `json` and `csv` to third-party libraries such as `BeautifulSoup` for HTML parsing, Python offers
Understanding Parsing in Python
Parsing in Python refers to the process of analyzing a string of symbols, either in natural language or programming language, and converting it into a more manageable structure. This is essential in various applications such as data processing, text analysis, and programming language interpreters. In Python, parsing can be accomplished using various libraries and techniques, each suited to different types of data.
Types of Parsing
There are several types of parsing techniques commonly utilized in Python, including:
- Syntactic Parsing: This involves breaking down a string into its grammatical components. It is often used in compilers and interpreters.
- Semantic Parsing: This is the process of converting a parsed string into a representation that can be understood and manipulated programmatically.
- Data Parsing: This type is focused on converting data formats, such as JSON, XML, or CSV, into Python objects.
Common Libraries for Parsing
Python provides a rich ecosystem of libraries that facilitate parsing. Some of the most widely used libraries include:
- `re`: The built-in regular expression library, which allows for pattern matching and manipulation of strings.
- `json`: This library is used to parse JSON data, enabling the conversion of JSON strings into Python dictionaries.
- `xml.etree.ElementTree`: A module for parsing and creating XML documents.
- `BeautifulSoup`: A library for parsing HTML and XML documents, particularly useful for web scraping.
- `pyparsing`: A library that provides a toolkit for defining grammars to parse complex text formats.
Basic Example of JSON Parsing
To illustrate parsing in Python, here is a basic example of how to parse JSON data:
python
import json
# Sample JSON string
json_data = ‘{“name”: “John”, “age”: 30, “city”: “New York”}’
# Parsing JSON string into a Python dictionary
parsed_data = json.loads(json_data)
print(parsed_data[‘name’]) # Output: John
Table of Parsing Libraries
Library | Purpose | Usage Example |
---|---|---|
re | Regular expression operations | re.findall(pattern, string) |
json | Parsing JSON data | json.loads(json_string) |
xml.etree.ElementTree | Parsing XML documents | ET.parse(file).getroot() |
BeautifulSoup | HTML and XML parsing | BeautifulSoup(html, ‘html.parser’) |
pyparsing | Defining grammars for text parsing | ParserElement.parseString(text) |
Parsing Techniques
Parsing is a fundamental aspect of data manipulation in Python, allowing developers to work with various data formats efficiently. Understanding the different types of parsing and the libraries available can greatly enhance productivity when handling complex data scenarios.
Understanding Parsing in Python
Parsing in Python refers to the process of analyzing a string of symbols, either in natural language or computer languages, and extracting meaningful information from it. This is crucial in various applications, including data processing, language translation, and web scraping.
Types of Parsing
There are several types of parsing techniques used in Python, including:
- Syntactic Parsing: Analyzes the structure of code or text based on grammar rules. Commonly used in compilers and interpreters.
- Semantic Parsing: Goes beyond structure to understand the meaning or intent behind the text, often employed in natural language processing.
- Lexical Parsing: Breaks down text into tokens, which are the smallest units of meaning, such as words or symbols.
Libraries for Parsing in Python
Python offers several libraries that facilitate parsing tasks. Some of the most popular include:
Library | Purpose |
---|---|
`BeautifulSoup` | Parsing HTML and XML documents. |
`lxml` | Fast and efficient parsing of XML and HTML. |
`json` | Parsing JSON data structures. |
`re` | Regular expressions for complex pattern matching. |
`argparse` | Parsing command-line arguments. |
Parsing HTML with BeautifulSoup
BeautifulSoup is a powerful library for parsing HTML and XML documents. It creates parse trees from page source codes, allowing easy navigation and modification.
Example of parsing HTML using BeautifulSoup:
python
from bs4 import BeautifulSoup
html_doc = “
Hello World!
”
soup = BeautifulSoup(html_doc, ‘html.parser’)
# Extracting title
title = soup.title.string
# Extracting paragraph text
paragraph = soup.find(‘p’, class_=’text’).get_text()
print(title) # Output: Test
print(paragraph) # Output: Hello World!
Parsing JSON Data
Python’s built-in `json` library allows for easy parsing of JSON data, which is common in web APIs.
Example of parsing JSON:
python
import json
json_data = ‘{“name”: “John”, “age”: 30, “city”: “New York”}’
data = json.loads(json_data)
print(data[‘name’]) # Output: John
print(data[‘age’]) # Output: 30
Regular Expressions for Parsing
The `re` module in Python provides tools for parsing and manipulating strings using regular expressions. This is particularly useful for tasks like data validation, extraction, and transformation.
Example of using regular expressions:
python
import re
text = “The price is $100.”
match = re.search(r’\$\d+’, text)
if match:
price = match.group()
print(price) # Output: $100
Parsing in Python is a fundamental skill that enables developers to manipulate and analyze data efficiently. Utilizing libraries like BeautifulSoup, `json`, and `re` empowers programmers to handle a wide range of data formats and structures seamlessly.
Understanding Parsing in Python: Insights from Experts
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). Parsing in Python is a crucial process that involves analyzing a string of symbols, either in natural language or computer languages. It allows developers to convert data into a format that is easier to manipulate and analyze, making it essential for data processing tasks.
Michael Chen (Software Engineer, CodeCraft Solutions). The parsing capabilities in Python are extensive, thanks to libraries like `json`, `xml.etree.ElementTree`, and `BeautifulSoup`. These tools enable developers to efficiently extract and transform data from various formats, which is vital for web scraping and API interactions.
Sarah Patel (Lead Developer, DataOps Group). In Python, parsing is not just about reading data; it is about understanding its structure and semantics. This understanding is fundamental for building robust applications that rely on accurate data interpretation, especially in fields like machine learning and artificial intelligence.
Frequently Asked Questions (FAQs)
What is parsing in Python?
Parsing in Python refers to the process of analyzing a string of symbols, either in natural language or in programming code, to extract meaningful information or transform it into a more usable format. This often involves breaking down the input into its constituent parts for further processing.
Why is parsing important in programming?
Parsing is crucial in programming as it enables the interpretation of data structures, such as JSON, XML, or even user input. It allows programs to understand and manipulate data effectively, facilitating tasks like data validation, transformation, and extraction.
What libraries are commonly used for parsing in Python?
Common libraries for parsing in Python include `json` for JSON data, `xml.etree.ElementTree` for XML, `BeautifulSoup` for HTML parsing, and `re` for regular expressions. Each library serves specific parsing needs based on the data format.
How does Python handle errors during parsing?
Python raises exceptions when parsing errors occur, such as `ValueError` for incorrect data types or `SyntaxError` for malformed code. Developers can handle these exceptions using try-except blocks to ensure graceful error management.
Can parsing be done on large datasets in Python?
Yes, Python can efficiently parse large datasets using libraries like `pandas` for structured data or `Dask` for parallel computing. These libraries provide optimized methods for reading and processing large volumes of data without consuming excessive memory.
What are some common applications of parsing in Python?
Common applications of parsing in Python include web scraping, data extraction from APIs, configuration file processing, natural language processing, and transforming data formats for analysis or storage.
Parsing in Python refers to the process of analyzing a string of data or text and converting it into a structured format that is easier to work with. This is often necessary when dealing with various data formats, such as JSON, XML, HTML, or even simple text files. Python provides several libraries and tools, such as `json`, `xml.etree.ElementTree`, and `BeautifulSoup`, which facilitate the parsing of different data types and formats efficiently.
One of the key insights regarding parsing in Python is the importance of choosing the right library or method based on the data format being processed. For instance, `json` is ideal for handling JSON data, while `BeautifulSoup` excels in parsing HTML and XML documents. Understanding the specific requirements and characteristics of the data can significantly streamline the parsing process and enhance performance.
Moreover, parsing is not only about extracting data but also about transforming it into a usable format. This transformation might involve cleaning the data, filtering out unnecessary information, or converting it into a different structure, such as a list or a dictionary. Mastering these techniques is essential for effective data manipulation and analysis in Python.
parsing is a fundamental skill in Python programming that enables developers to handle and
Author Profile
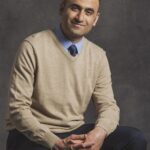
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?