What Is the Prototype in JavaScript and Why Is It Important?
### Introduction
In the dynamic world of JavaScript, understanding the concept of prototypes is crucial for mastering the language’s object-oriented features. Prototypes serve as a foundational element that empowers developers to create efficient, reusable code, enabling them to build complex applications with ease. Whether you’re a seasoned programmer or just starting your journey into JavaScript, grasping the intricacies of prototypes can significantly enhance your coding prowess and deepen your understanding of how JavaScript operates under the hood.
At its core, a prototype in JavaScript is an object that is associated with every function and object by default, allowing for the inheritance of properties and methods. This mechanism forms the backbone of JavaScript’s prototype-based inheritance, offering a unique alternative to classical inheritance found in other programming languages. By leveraging prototypes, developers can extend the functionality of existing objects and create new ones that inherit characteristics from their predecessors, fostering a more organized and modular approach to coding.
As we delve deeper into the topic, we will explore how prototypes work, their role in the JavaScript object model, and the practical implications of using prototypes in your projects. From understanding the prototype chain to implementing custom prototypes, this article will equip you with the knowledge needed to harness the full potential of prototypes in JavaScript, setting the stage for more advanced programming
Understanding the Prototype
In JavaScript, every object has an internal property called `[[Prototype]]`, which is utilized for inheritance. This mechanism allows an object to inherit properties and methods from another object. The prototype is essentially a reference to another object, enabling JavaScript to implement a form of inheritance known as prototype-based inheritance.
When you attempt to access a property on an object, JavaScript first checks if the property exists on that object. If it does not, JavaScript looks up the prototype chain, which is a series of linked prototypes, until it either finds the property or reaches the end of the chain (the `null` prototype).
How Prototypes Work
The prototype of an object can be accessed via the `__proto__` property or by using the `Object.getPrototypeOf()` method. Every function in JavaScript has a prototype property that is used when the function is used as a constructor with the `new` keyword. This prototype property allows instances of the constructor to inherit properties and methods defined on the prototype.
Key aspects of prototypes include:
- Prototype Chain: A series of linked prototypes that JavaScript checks to resolve properties.
- Constructor Functions: Functions that create objects and define their prototype.
- Inheritance: Allows one object to access the properties and methods of another.
Creating and Using Prototypes
Creating a prototype can be done using constructor functions or the `class` syntax introduced in ES6. Here’s an example using a constructor function:
javascript
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ‘ makes a noise.’);
};
const dog = new Animal(‘Dog’);
dog.speak(); // Outputs: Dog makes a noise.
In this example, `speak` is a method defined on `Animal.prototype`, allowing all instances of `Animal` to use this method.
Prototype vs. Instance Properties
It’s crucial to differentiate between properties defined on an instance and properties defined on a prototype. Instance properties are unique to each object, while prototype properties are shared among all instances.
Property Type | Description |
---|---|
Instance Property | Defined directly on the object instance. |
Prototype Property | Defined on the prototype and shared among all instances. |
Benefits of Using Prototypes
Utilizing prototypes offers several advantages:
- Memory Efficiency: Shared methods reduce memory usage since they exist in one place.
- Inheritance: Easily extend functionality by adding new methods to the prototype.
- Dynamic Behavior: Prototypes can be modified at runtime, allowing for flexible code changes.
understanding prototypes is fundamental for mastering object-oriented programming in JavaScript. The prototype system provides a powerful means to facilitate inheritance and share behavior among objects efficiently.
Understanding the Prototype in JavaScript
The prototype in JavaScript is a fundamental concept that underpins its object-oriented nature. Every JavaScript object has an internal property called `[[Prototype]]`, which references another object. This creates a prototype chain, enabling inheritance.
How Prototypes Work
When attempting to access a property or method on an object, JavaScript first checks if the property exists on the object itself. If it does not, JavaScript looks up the prototype chain until it finds the property or reaches the end of the chain (i.e., `null`).
- Object Creation: When an object is created using a constructor function, the new object’s prototype is set to the constructor’s prototype property.
- Prototype Chain: If a property is not found on the object, JavaScript checks the object’s prototype, then the prototype’s prototype, and so on.
Creating and Modifying Prototypes
You can create and modify prototypes in the following ways:
- Using Constructor Functions:
javascript
function Person(name) {
this.name = name;
}
Person.prototype.greet = function() {
console.log(`Hello, my name is ${this.name}`);
};
const alice = new Person(‘Alice’);
alice.greet(); // Output: Hello, my name is Alice
- Directly Modifying the Prototype:
javascript
Person.prototype.age = 30;
console.log(alice.age); // Output: 30
Prototype Inheritance
Prototype inheritance allows one object to inherit properties and methods from another. This is achieved through the prototype chain.
- Example:
javascript
function Employee(name, position) {
Person.call(this, name);
this.position = position;
}
Employee.prototype = Object.create(Person.prototype);
Employee.prototype.constructor = Employee;
const bob = new Employee(‘Bob’, ‘Developer’);
bob.greet(); // Output: Hello, my name is Bob
Prototype vs. Object Properties
Understanding the distinction between properties directly on an object and those inherited from its prototype is crucial.
Aspect | Object Property | Prototype Property |
---|---|---|
Location | Defined directly on the object | Defined on the constructor’s prototype |
Access | Directly accessible | Accessible via prototype chain |
Overriding | Overrides prototype property | Can be overridden by object property |
Common Use Cases for Prototypes
- Method Sharing: Define methods on the prototype to allow all instances to share the same method, saving memory.
- Inheritance: Create new objects that inherit from existing objects using prototypes.
- Dynamic Property Addition: Add properties or methods at runtime to enhance functionality without modifying existing instances.
Prototypes
The prototype system in JavaScript provides a powerful mechanism for inheritance and method sharing, enabling developers to create efficient and organized code structures. Understanding prototypes is essential for mastering JavaScript’s object-oriented features.
Understanding Prototypes in JavaScript: Expert Insights
Dr. Emily Carter (Senior JavaScript Developer, Tech Innovations Inc.). Prototypes in JavaScript serve as a fundamental mechanism for inheritance and property sharing. They allow objects to inherit properties and methods from other objects, which is essential for creating efficient and reusable code.
Michael Chen (Software Architect, CodeCraft Solutions). The prototype chain is a core concept in JavaScript that enables dynamic property resolution. Understanding how prototypes work is crucial for mastering object-oriented programming in JavaScript, as it influences how methods and properties are accessed and overridden.
Sarah Thompson (JavaScript Educator, LearnCode Academy). In JavaScript, every function has a prototype property, which is used to attach methods and properties that can be shared by all instances of that function. This prototype-based inheritance model is what sets JavaScript apart from classical inheritance languages, making it unique and powerful.
Frequently Asked Questions (FAQs)
What is prototype in JavaScript?
The prototype in JavaScript is an object that is associated with every function and object by default. It allows for inheritance and the sharing of properties and methods among instances of objects.
How does prototypal inheritance work in JavaScript?
Prototypal inheritance allows objects to inherit properties and methods from other objects through the prototype chain. When a property or method is not found on an object, JavaScript looks up the prototype chain until it finds the property or reaches the end of the chain.
Can you modify the prototype of built-in objects in JavaScript?
Yes, you can modify the prototype of built-in objects such as Array, Object, and Function. However, doing so is generally discouraged as it can lead to unexpected behavior and conflicts with other code.
What is the difference between __proto__ and prototype?
`__proto__` is an accessor property that points to the prototype of an object, while `prototype` is a property of functions that defines the prototype object for instances created by that function. `__proto__` is used for instance objects, whereas `prototype` is used for constructor functions.
How can you create a new object with a specific prototype?
You can create a new object with a specific prototype using `Object.create()`. This method allows you to specify an existing object as the prototype for the new object, enabling prototypal inheritance.
What are the implications of using prototypes in JavaScript?
Using prototypes allows for memory efficiency and code reuse, as methods and properties are shared among instances rather than duplicated. However, it can also introduce complexity and potential performance issues if not managed properly.
The concept of prototypes in JavaScript is a fundamental aspect of its object-oriented programming model. In JavaScript, every object has a prototype, which is another object from which it can inherit properties and methods. This prototype-based inheritance allows for the creation of more complex objects while maintaining efficient memory usage, as shared methods and properties are stored in the prototype rather than in each individual object instance.
Understanding prototypes is crucial for effective JavaScript programming. Developers can extend built-in objects or create custom prototypes to enhance functionality and ensure code reusability. The prototype chain is a powerful mechanism that enables dynamic property and method resolution, allowing objects to access properties and methods from their prototype and, by extension, from the prototypes of their prototypes, forming a chain of inheritance.
Key takeaways include the importance of the `__proto__` property, which allows access to an object’s prototype, and the `Object.create()` method, which facilitates the creation of new objects with a specified prototype. Additionally, understanding how to manipulate prototypes can lead to more efficient code and a deeper comprehension of JavaScript’s behavior, especially in relation to inheritance and object creation.
Author Profile
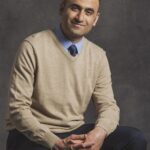
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?