What is Scripting in Python? Exploring Its Purpose and Benefits
### Introduction
In the ever-evolving landscape of programming, Python has emerged as a powerhouse, captivating developers and enthusiasts alike with its simplicity and versatility. Among its many applications, scripting in Python stands out as a powerful tool that enables users to automate tasks, manipulate data, and streamline workflows with remarkable ease. Whether you’re a seasoned programmer or just dipping your toes into the world of coding, understanding scripting in Python can unlock a plethora of possibilities, making your projects more efficient and your coding experience more enjoyable.
Scripting in Python refers to the practice of writing small programs, or scripts, that perform specific tasks. Unlike traditional software development, which often involves building large applications from the ground up, scripting allows for quick solutions to everyday problems. From automating repetitive tasks to processing data and even web scraping, Python scripts can be tailored to fit a wide range of needs. This flexibility, combined with Python’s readable syntax, makes it an ideal choice for both beginners and experienced developers looking to enhance their productivity.
As we delve deeper into the world of Python scripting, we will explore its fundamental concepts, practical applications, and the tools that can help you harness its full potential. Whether you’re looking to automate mundane tasks or create sophisticated applications, understanding the nuances of scripting in Python will empower you to take
Understanding Scripting in Python
Scripting in Python refers to the process of writing short programs or scripts that automate tasks or manipulate data. Unlike traditional programming, which often involves developing large software applications, scripting is generally focused on quick solutions and automation of repetitive tasks. Python, with its clear syntax and dynamic typing, is particularly well-suited for this purpose.
Python scripts can be used for a variety of tasks, including:
- Automating mundane tasks (e.g., file manipulation, data entry)
- Web scraping to collect data from websites
- Data analysis and visualization
- Writing simple games or applications
- Server-side scripting for web applications
One of the defining features of scripting in Python is its ease of use, allowing for rapid development and testing. Python scripts are usually run in a command-line interface or through an integrated development environment (IDE), making it accessible for both beginners and experienced developers.
Key Features of Python Scripting
Several characteristics make Python an attractive choice for scripting:
- Readability: Python syntax is clean and easy to read, which helps developers understand and maintain scripts.
- Extensive Libraries: Python has a rich ecosystem of libraries and frameworks that simplify complex tasks. Libraries like `os`, `sys`, and `requests` provide built-in functionality for various operations.
- Cross-Platform Compatibility: Python scripts can run on any operating system with a Python interpreter, making them versatile for different environments.
- Interpreted Language: Python is an interpreted language, meaning that scripts are executed line-by-line. This allows for easier debugging and interactive coding sessions.
Common Applications of Python Scripting
Python scripting is widely used in various domains. Here are some common applications:
Application Area | Description | Popular Libraries |
---|---|---|
Web Development | Server-side scripting for dynamic web content. | Django, Flask |
Data Analysis | Processing and analyzing large datasets. | Pandas, NumPy |
Automation | Automating repetitive tasks, such as file management. | os, shutil |
Web Scraping | Extracting data from websites. | BeautifulSoup, Scrapy |
Game Development | Creating simple games or simulations. | Pygame |
These applications highlight Python’s versatility as a scripting language, catering to various professional and personal needs. With its extensive community support and continuous development, Python remains a top choice for developers looking to harness the power of scripting.
Getting Started with Python Scripting
To begin scripting in Python, one can follow these steps:
- Install Python: Download and install the latest version of Python from the official website.
- Choose an IDE: Select an integrated development environment such as PyCharm, VS Code, or Jupyter Notebook.
- Write a Script: Start by writing a simple script. For example, a script that prints “Hello, World!” can be created as follows:
python
print(“Hello, World!”)
- Run the Script: Execute the script using the command line or the IDE’s run feature.
- Iterate and Improve: Modify and expand the script based on your requirements, utilizing Python libraries as needed.
By following these steps, anyone can start leveraging Python for scripting tasks effectively.
Understanding Scripting in Python
Scripting in Python refers to the process of writing small programs, often called scripts, that automate tasks or perform specific functions. Python’s simplicity and readability make it an excellent choice for scripting, allowing developers to write code quickly and efficiently.
Characteristics of Python Scripting
Python scripts are typically characterized by the following features:
- Interpreted Language: Python scripts are executed by an interpreter, which means they can be run directly without the need for a separate compilation step.
- High-Level Language: Python abstracts many complex details, allowing developers to focus on logic and functionality rather than low-level programming concerns.
- Dynamic Typing: Variables in Python are dynamically typed, which simplifies code writing and reduces verbosity.
- Extensive Libraries: Python’s rich ecosystem of libraries and frameworks enables developers to perform a wide range of tasks with minimal code.
Common Uses of Python Scripting
Python scripting is utilized in various domains and for numerous purposes, including:
- Automation: Automating repetitive tasks such as file manipulation, data entry, and report generation.
- Web Development: Creating server-side scripts for web applications using frameworks like Flask and Django.
- Data Analysis: Writing scripts to process and analyze data using libraries like Pandas and NumPy.
- System Administration: Managing system tasks through scripts that interact with the operating system.
- Testing: Developing automated tests for applications, ensuring code quality and performance.
Basic Syntax of Python Scripts
Understanding the basic syntax of Python is essential for effective scripting. Here are some fundamental components:
– **Comments**: Begin with `#`, allowing for annotations in the code.
– **Variables**: No need to declare types explicitly; simply assign a value:
python
name = “John”
age = 30
– **Control Structures**:
– **If Statements**:
python
if age >= 18:
print(“Adult”)
- Loops:
python
for i in range(5):
print(i)
Creating and Running a Python Script
To create and run a Python script, follow these steps:
- Write the Script:
- Use any text editor to write your Python code.
- Save the file with a `.py` extension, for example, `script.py`.
- Run the Script:
- Open a terminal or command prompt.
- Navigate to the directory containing your script.
- Execute the script using:
bash
python script.py
Best Practices for Python Scripting
Adopting best practices enhances the quality and maintainability of Python scripts:
- Use Meaningful Variable Names: Choose descriptive names for better readability.
- Modularize Code: Break down complex scripts into functions or modules.
- Handle Exceptions: Use try-except blocks to manage errors gracefully.
- Write Documentation: Include docstrings and comments to explain the code’s purpose and functionality.
- Maintain Consistent Formatting: Follow PEP 8 guidelines for code style to improve clarity.
Scripting in Python leverages its powerful features and extensive libraries, making it an invaluable tool for developers across various fields. By mastering Python scripting, one can significantly enhance productivity and efficiency in numerous tasks.
Understanding Scripting in Python: Perspectives from Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “Scripting in Python is a powerful way to automate tasks and streamline workflows. It allows developers to write concise code that can manipulate data, interact with APIs, and automate repetitive processes, making it an essential skill in the modern programming landscape.”
Michael Tran (Lead Data Scientist, Data Insights LLC). “Python scripting is particularly valuable in data analysis and machine learning. Its extensive libraries and frameworks enable data scientists to write scripts that can quickly process large datasets, perform statistical analyses, and visualize results, thus enhancing productivity and efficiency.”
Jessica Liu (Educational Technology Specialist, FutureLearn Academy). “In the realm of education, Python scripting serves as an excellent introduction to programming concepts. It provides learners with a hands-on approach to coding, where they can see immediate results from their scripts, fostering engagement and deeper understanding of computational thinking.”
Frequently Asked Questions (FAQs)
What is scripting in Python?
Scripting in Python refers to writing small programs or scripts that automate tasks or perform specific functions. These scripts are typically executed directly by the Python interpreter without the need for compilation.
What are the advantages of using Python for scripting?
Python offers several advantages for scripting, including its simplicity and readability, extensive libraries, cross-platform compatibility, and strong community support, which facilitates rapid development and debugging.
How does Python scripting differ from programming?
Python scripting is generally focused on automating tasks and handling smaller, simpler programs, whereas programming encompasses a broader scope, including the development of larger, more complex applications and systems.
Can Python scripts be used for web development?
Yes, Python scripts can be utilized in web development. Frameworks like Flask and Django allow developers to create web applications efficiently by using Python scripts to handle backend logic and server interactions.
What types of tasks can be automated with Python scripting?
Python scripting can automate a wide range of tasks, including file manipulation, data processing, web scraping, system administration tasks, and automating repetitive workflows in various applications.
Is Python a suitable language for beginners in scripting?
Yes, Python is highly suitable for beginners due to its straightforward syntax and extensive documentation. It allows newcomers to quickly grasp scripting concepts and start building functional scripts with minimal learning curve.
Scripting in Python refers to the process of writing small programs, often called scripts, to automate tasks or perform specific functions. Python’s simplicity and readability make it an ideal language for scripting, allowing developers to write code quickly and efficiently. Scripting can involve various applications, such as web scraping, data manipulation, system automation, and more, showcasing Python’s versatility in handling different tasks with ease.
One of the key advantages of using Python for scripting is its extensive standard library and the availability of numerous third-party modules. This rich ecosystem enables developers to leverage pre-built functions and tools, significantly reducing the time and effort required to write scripts from scratch. Furthermore, Python’s interactive shell allows for rapid prototyping and testing, making it easier for developers to experiment with code snippets and iterate on their solutions.
scripting in Python serves as a powerful tool for automating repetitive tasks and streamlining workflows. Its user-friendly syntax, combined with a robust set of libraries, empowers both beginners and experienced programmers to create effective scripts. As Python continues to grow in popularity, its role in scripting will likely expand, further solidifying its position as a go-to language for automation and task management.
Author Profile
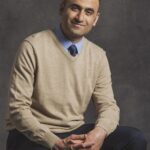
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?