What is Sequencing in Python and Why is it Important?
In the world of programming, the order in which we execute tasks can significantly influence the outcome of our code. This concept, known as sequencing, is a fundamental principle in Python that shapes the way we write and understand our programs. Whether you’re a novice coder or a seasoned developer, grasping the nuances of sequencing will empower you to create more efficient and logical scripts. As we delve into the intricacies of sequencing in Python, you’ll discover how this essential concept not only enhances code readability but also ensures that your programs run smoothly and effectively.
Sequencing in Python refers to the linear execution of statements, where each line of code is processed in the order it appears. This straightforward approach is crucial for establishing a clear flow of operations, allowing developers to construct logical sequences that lead to desired outcomes. By understanding how Python handles sequencing, you can better manage the control of your program, ensuring that each command is executed at the right time and in the right context.
Moreover, the concept of sequencing lays the groundwork for more advanced programming constructs, such as conditionals and loops. These elements build upon the foundation of sequential execution, enabling programmers to introduce complexity and interactivity into their applications. As we explore the various aspects of sequencing in Python, you will gain insights into how this
Understanding Sequencing in Python
Sequencing in Python refers to the ordered arrangement of elements in data structures, allowing operations to be performed in a specific order. Python supports several built-in sequence types, including lists, tuples, and strings. These data structures are fundamental for managing collections of data effectively.
Types of Sequences
Python provides several types of sequences, each with unique characteristics and use cases:
- Lists: Mutable sequences that can contain items of different types. Lists are created using square brackets `[]` and allow for modifications such as adding, removing, or changing elements.
- Tuples: Immutable sequences that are defined using parentheses `()`. Once a tuple is created, its elements cannot be modified, making it useful for fixed collections of items.
- Strings: Immutable sequences of characters. Strings are defined using single or double quotes and support various operations, including slicing and concatenation.
Key Characteristics of Sequences
- Indexing: Each element in a sequence can be accessed using an index, which starts at 0 for the first element. Negative indexing is also supported, allowing access from the end of the sequence.
- Slicing: Sequences can be sliced to extract a subset of elements. The syntax for slicing is `sequence[start:stop:step]`, where `start` is the index of the first element, `stop` is the index where the slice ends, and `step` defines the increment.
- Concatenation and Repetition: Sequences can be concatenated using the `+` operator and repeated using the `*` operator. This allows for flexible manipulation of data collections.
Sequence Type | Mutability | Syntax | Common Methods |
---|---|---|---|
List | Mutable | list = [1, 2, 3] | append(), remove(), pop() |
Tuple | Immutable | tuple = (1, 2, 3) | count(), index() |
String | Immutable | string = “hello” | upper(), lower(), split() |
Working with Sequences
To illustrate how to work with sequences, consider the following examples:
- Creating a List:
“`python
my_list = [1, 2, 3, 4]
“`
- Accessing Elements:
“`python
first_element = my_list[0] Returns 1
“`
- Slicing a List:
“`python
sub_list = my_list[1:3] Returns [2, 3]
“`
- Concatenating Lists:
“`python
new_list = my_list + [5, 6] Returns [1, 2, 3, 4, 5, 6]
“`
- Iterating Over a Sequence:
“`python
for item in my_list:
print(item)
“`
By mastering the concept of sequencing in Python, developers can leverage the power of ordered collections to create efficient and readable code.
Understanding Sequencing in Python
Sequencing in Python refers to the arrangement of elements in a specific order. It is a fundamental concept that underpins various data types and structures used in programming. In Python, sequences are iterable collections that allow you to access, modify, and manipulate data elements in an organized manner.
Types of Sequences
Python primarily supports several built-in sequence types:
- Lists: Mutable sequences that can store mixed data types.
- Tuples: Immutable sequences that can also hold mixed data types.
- Strings: Immutable sequences of characters.
- Ranges: Immutable sequences of numbers, primarily used for looping.
Sequence Type | Mutability | Example |
---|---|---|
List | Mutable | `[1, 2, 3, ‘a’, ‘b’]` |
Tuple | Immutable | `(1, 2, 3, ‘a’, ‘b’)` |
String | Immutable | `”Hello, World!”` |
Range | Immutable | `range(0, 10)` |
Accessing Elements in Sequences
Elements in sequences can be accessed using indexing. Python uses zero-based indexing, meaning the first element is at index 0.
- List Example:
“`python
my_list = [10, 20, 30]
print(my_list[0]) Output: 10
“`
- Tuple Example:
“`python
my_tuple = (10, 20, 30)
print(my_tuple[1]) Output: 20
“`
- String Example:
“`python
my_string = “Hello”
print(my_string[4]) Output: ‘o’
“`
Common Operations on Sequences
Python provides a variety of operations that can be performed on sequences:
- Slicing: Extracting a portion of the sequence.
- Example: `my_list[1:3]` retrieves the elements from index 1 to 2.
- Concatenation: Merging two sequences.
- Example: `[1, 2] + [3, 4]` results in `[1, 2, 3, 4]`.
- Repetition: Repeating a sequence multiple times.
- Example: `[1] * 3` produces `[1, 1, 1]`.
- Membership Testing: Checking if an element exists in a sequence.
- Example: `3 in [1, 2, 3]` evaluates to `True`.
Looping Through Sequences
You can iterate over elements in a sequence using loops, such as `for` loops:
“`python
for element in my_list:
print(element)
“`
This will output each element in `my_list`, demonstrating how to access elements sequentially.
Conclusion on Sequencing
Understanding and utilizing sequences effectively is crucial for efficient programming in Python. Their versatile nature allows for various operations, making them an essential component in data manipulation and algorithm development.
Understanding Sequencing in Python: Perspectives from Experts
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Sequencing in Python refers to the ordered arrangement of elements within data structures such as lists, tuples, and strings. This concept is fundamental in programming, as it allows developers to access and manipulate data in a predictable manner.”
Michael Chen (Python Developer Advocate, CodeMaster Labs). “In Python, sequencing is not just about data structures; it also encompasses the flow of execution in scripts. Understanding how sequences work enables programmers to write more efficient and readable code, particularly when using loops and conditionals.”
Lisa Patel (Software Engineer, Data Solutions Group). “The concept of sequencing in Python is pivotal for data analysis and manipulation. It allows for operations such as slicing and indexing, which are essential for extracting specific data points from larger datasets.”
Frequently Asked Questions (FAQs)
What is sequencing in Python?
Sequencing in Python refers to the arrangement of elements in a specific order, typically within data structures such as lists, tuples, and strings. It allows for the organization and manipulation of data in a linear format.
How does sequencing differ from other data structures in Python?
Sequencing specifically involves ordered collections, meaning the position of each element is significant. In contrast, other data structures like sets and dictionaries do not maintain order, focusing instead on unique elements or key-value pairs.
What are common examples of sequence types in Python?
Common sequence types in Python include lists, tuples, and strings. Each of these types allows for indexing, slicing, and iteration, providing various methods for data manipulation.
Can you modify elements in a sequence in Python?
Yes, elements in mutable sequences like lists can be modified, added, or removed. However, immutable sequences like tuples and strings do not allow for direct modification; instead, new sequences must be created.
What are some built-in functions related to sequencing in Python?
Python offers several built-in functions for sequences, including `len()` to determine the length, `max()` and `min()` to find the largest and smallest elements, and `sorted()` to return a sorted version of the sequence.
How does indexing work in Python sequences?
Indexing in Python sequences allows access to individual elements using their position, starting from zero. Negative indexing is also supported, allowing access to elements from the end of the sequence.
Sequencing in Python refers to the arrangement and organization of data elements in a specific order. This concept is fundamental to Python programming, as it allows developers to manipulate and access data efficiently. Python provides several built-in data types that support sequencing, including lists, tuples, and strings. Each of these data types allows for the storage of multiple items in a single variable, making it easier to manage collections of data.
One of the key features of sequencing in Python is the ability to access elements using indexing. Python uses zero-based indexing, which means that the first element in a sequence is accessed with index 0. This feature enables programmers to retrieve, modify, and iterate over elements in a straightforward manner. Additionally, Python supports various operations on sequences, such as slicing, concatenation, and repetition, which enhance the flexibility and functionality of data manipulation.
Furthermore, understanding the properties of different sequence types is crucial for effective programming in Python. For example, lists are mutable, allowing for changes to be made after their creation, while tuples are immutable, providing a stable structure for data that should not change. Strings, while also immutable, offer a rich set of methods for text manipulation. Recognizing these distinctions helps developers choose the appropriate data type
Author Profile
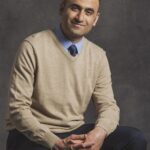
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?