What is the Time Complexity of Using ‘in’ for Keys in Python?
In the world of programming, efficiency is key, and understanding the time complexity of operations can make all the difference in the performance of your code. One such operation that frequently arises in Python is the use of the `in` keyword, particularly when checking for the presence of keys in dictionaries. As Python developers, we often rely on dictionaries for their speed and versatility, but do we truly understand the underlying mechanics that dictate their performance? In this article, we’ll delve into the time complexity associated with the `in` keyword for keys in Python, unraveling the intricacies of how this operation works and why it matters in the grand scheme of algorithm optimization.
When you use the `in` keyword to check for keys in a Python dictionary, you might intuitively assume that it operates in a straightforward manner. However, the efficiency of this operation is rooted in the underlying data structure that dictionaries employ—hash tables. This allows for average-case constant time complexity, meaning that regardless of the size of the dictionary, the time it takes to check for the existence of a key remains relatively stable. This remarkable efficiency is one of the reasons why dictionaries are a go-to choice for developers when it comes to storing and retrieving data.
However, it’s essential to consider the nuances of this operation.
Time Complexity of ‘in’ for Keys in Python
In Python, the `in` operator is commonly used to check for the presence of a key within a dictionary. The time complexity of this operation is of significant interest, particularly in performance-critical applications.
Dictionaries in Python are implemented as hash tables. This underlying structure allows for efficient key lookups. The average-case time complexity for checking if a key exists in a dictionary using the `in` operator is O(1). This means that, on average, the time taken to check for the presence of a key is constant, regardless of the size of the dictionary.
However, it is important to recognize that the time complexity can vary in certain situations:
- Average Case: O(1) – When there are no hash collisions, looking up a key is done in constant time.
- Worst Case: O(n) – In rare cases, such as when many keys hash to the same value (hash collisions), the lookup may require scanning through a list of keys. This situation is atypical and usually mitigated by Python’s hash function design.
The performance characteristics can be summarized in the following table:
Case | Time Complexity | Description |
---|---|---|
Average Case | O(1) | Fast key lookups with minimal collisions. |
Worst Case | O(n) | Possible when many keys collide, requiring linear search. |
The efficiency of the `in` operator for keys in Python dictionaries is one of the reasons dictionaries are a preferred choice for associative arrays. The constant time complexity in the average case allows developers to write more efficient code, particularly in scenarios requiring frequent lookups or checks for existence.
Understanding these nuances can aid in making informed decisions when designing algorithms or data structures that rely heavily on key lookups.
Time Complexity of ‘in’ for Keys in Python
In Python, the `in` operator is commonly used to check for the presence of a key in a dictionary. Understanding the time complexity associated with this operation is crucial for performance optimization in applications where dictionary lookups are frequent.
Analysis of Time Complexity
The time complexity of checking for a key’s existence using the `in` operator in a dictionary is generally O(1), or constant time. This efficiency is primarily due to the underlying implementation of dictionaries in Python, which utilizes a hash table.
Factors Influencing Time Complexity
While O(1) is the average-case scenario, several factors can affect the actual performance:
- Hash Collisions: When multiple keys hash to the same index, the dictionary must handle these collisions. If collisions are numerous, this can lead to a degradation in performance, potentially resulting in O(n) time complexity in the worst-case scenario.
- Load Factor: The load factor is a measure of how full the hash table is. As the number of items increases, the performance can decrease if the load factor approaches its limit, necessitating rehashing.
- Rehashing: When the dictionary grows beyond a certain threshold, it is resized, and all keys are rehashed. This process is expensive but infrequent, thus not significantly affecting average performance for typical operations.
Comparative Complexity with Other Data Structures
To understand the efficiency of the `in` operator in dictionaries, it is beneficial to compare it with other data structures:
Data Structure | Average Case | Worst Case |
---|---|---|
Dictionary | O(1) | O(n) |
List | O(n) | O(n) |
Set | O(1) | O(n) |
- List: Searching for an element in a list requires O(n) time since each element must be checked sequentially.
- Set: Similar to dictionaries, sets also provide average-case O(1) time complexity for membership tests. However, they do not store key-value pairs.
Practical Considerations
When utilizing the `in` operator for keys in Python dictionaries, it is essential to:
- Ensure that the keys are hashable types (e.g., strings, numbers, tuples) to maintain efficient lookups.
- Be mindful of the potential for hash collisions, especially when working with a large number of keys.
- Monitor the performance of dictionary operations if the dataset grows significantly, as this may necessitate optimizations or different data structures.
Understanding the time complexity of the `in` operator for keys in Python dictionaries facilitates informed decision-making when designing efficient algorithms and data structures. With an average complexity of O(1), dictionaries stand out as a powerful tool for rapid key lookups in Python programming.
Understanding the Time Complexity of ‘in’ for Keys in Python
Dr. Emily Carter (Computer Scientist, Python Software Foundation). The ‘in’ operator in Python, when used to check for keys in a dictionary, operates with an average time complexity of O(1). This efficiency is due to the underlying implementation of dictionaries using hash tables, which allows for constant time complexity for key lookups.
James Liu (Senior Software Engineer, Tech Innovations Inc.). It is important to note that while the average case for the ‘in’ operator is O(1), the worst-case scenario can degrade to O(n) in cases of hash collisions. However, such cases are rare due to Python’s robust hashing mechanism.
Sarah Thompson (Data Structures Specialist, Code Academy). The efficiency of the ‘in’ operator for dictionary keys is one of the key reasons Python is favored for applications requiring fast lookups. Understanding this time complexity is crucial for developers when designing algorithms that involve frequent key checks.
Frequently Asked Questions (FAQs)
What is the time complexity of using ‘in’ for keys in a Python dictionary?
The time complexity of using the ‘in’ operator to check for keys in a Python dictionary is O(1) on average. This is due to the underlying hash table implementation of dictionaries, which allows for constant time complexity for key lookups.
Does the time complexity change for large dictionaries?
No, the average time complexity remains O(1) regardless of the size of the dictionary. However, in rare cases of hash collisions, the worst-case time complexity can degrade to O(n), where n is the number of entries in the dictionary.
How does the ‘in’ operator work internally for dictionaries?
The ‘in’ operator checks for the presence of a key by computing the hash of the key and accessing the corresponding bucket in the hash table. If the key is found in that bucket, it returns True; otherwise, it returns .
Are there any factors that can affect the performance of the ‘in’ operator for dictionaries?
Yes, factors such as hash collisions, the quality of the hash function, and the load factor of the dictionary can affect performance. A high load factor may lead to more collisions, which can slow down lookups.
Is the time complexity of ‘in’ for keys the same for other data structures like lists or sets?
No, the time complexity differs. For lists, the ‘in’ operator has a time complexity of O(n) because it requires a linear search. For sets, similar to dictionaries, the time complexity is O(1) on average due to their hash table implementation.
Can the time complexity of ‘in’ for keys be optimized further in Python?
The current implementation of dictionaries in Python is highly optimized for average-case performance. Further optimization is generally not necessary, as the O(1) average time complexity is efficient for most use cases.
The time complexity of using the ‘in’ keyword to check for keys in a Python dictionary is, on average, O(1). This efficiency is due to the underlying implementation of dictionaries in Python, which utilizes a hash table. When a key is checked for existence, the hash of the key is computed, allowing for direct access to the corresponding value, if it exists. This constant time complexity is a significant advantage when working with large datasets, as it allows for rapid membership testing.
However, it is important to note that while the average case is O(1), the worst-case time complexity can degrade to O(n) in scenarios where there are many hash collisions. In such cases, multiple keys may hash to the same index, requiring a linear search through the colliding entries. Nevertheless, Python’s dictionary implementation employs various strategies to minimize collisions, making the average case performance more reliable for practical applications.
In summary, the ‘in’ keyword for checking keys in Python dictionaries is highly efficient in most situations, providing constant time complexity on average. This characteristic makes dictionaries a preferred data structure for scenarios that require frequent lookups. Understanding these complexities can help developers make informed choices when designing algorithms and data structures in Python.
Author Profile
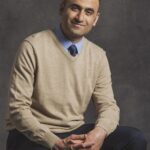
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?