What is the ‘try’ Statement in Python and How Does It Work?
In the world of programming, handling errors and exceptions is a critical skill that can make or break the reliability of your code. Python, a language celebrated for its simplicity and readability, offers a powerful mechanism to gracefully manage these unexpected situations: the `try` statement. Whether you’re a seasoned developer or a newcomer to coding, understanding how to effectively use `try` in Python can enhance your programming toolkit, allowing you to write more robust and fault-tolerant applications.
At its core, the `try` statement in Python serves as a protective barrier around code that may potentially raise exceptions. By encapsulating risky operations within a `try` block, developers can anticipate and handle errors without crashing their programs. This not only improves user experience but also aids in debugging and maintaining code integrity. Alongside the `try` statement, Python provides complementary structures, such as `except`, `else`, and `finally`, which together create a comprehensive error-handling framework.
As we delve deeper into the mechanics of `try` in Python, we will explore its syntax, the various ways to handle exceptions, and best practices for implementing error management in your code. Understanding these concepts will empower you to write cleaner, more efficient Python programs that can withstand the unpredictability of real-world applications. Join
Understanding the Try Block
The `try` block in Python is a crucial component of exception handling. It allows developers to write code that can gracefully handle errors and exceptions, which are runtime issues that may occur during the execution of a program. By using the `try` block, you can prevent your program from crashing and provide alternatives or informative messages when an error arises.
When you place code within a `try` block, Python will attempt to execute it. If an exception occurs, the flow of control is transferred to the corresponding `except` block, where you can define how to respond to the error. This mechanism not only enhances the robustness of your code but also improves user experience by managing unexpected situations effectively.
Syntax of Try-Except
The basic syntax of a `try` block followed by an `except` block is as follows:
“`python
try:
Code that may cause an exception
except SomeException:
Code that runs if the exception occurs
“`
You can also have multiple `except` blocks to handle different types of exceptions:
“`python
try:
Code that may cause an exception
except TypeError:
Handle TypeError
except ValueError:
Handle ValueError
“`
Additionally, you can use a generic `except` to catch all exceptions:
“`python
try:
Code that may cause an exception
except Exception as e:
Handle any exception and optionally log the error
“`
Finally and Else Clauses
In addition to `try` and `except`, Python allows the use of `finally` and `else` clauses to provide further control over exception handling.
- Finally: The code within the `finally` block will always execute, regardless of whether an exception occurred or not. This is useful for cleaning up resources, such as closing files or releasing network connections.
- Else: The code within the `else` block will execute if the code in the `try` block does not raise an exception. This is beneficial for executing code that should run only when no errors occur.
The complete structure can be illustrated as follows:
“`python
try:
Code that may cause an exception
except SomeException:
Handle the exception
else:
Code that runs if no exception occurs
finally:
Code that always runs
“`
Common Exceptions
Understanding common exceptions can help in anticipating potential issues in your code. Here are some frequently encountered exceptions in Python:
Exception Type | Description |
---|---|
TypeError | Raised when an operation or function is applied to an object of inappropriate type. |
ValueError | Raised when a function receives an argument of the right type but inappropriate value. |
IndexError | Raised when trying to access an element from a list using an invalid index. |
KeyError | Raised when trying to access a dictionary with a key that doesn’t exist. |
Using `try` blocks effectively allows developers to identify and manage errors systematically, leading to cleaner, more maintainable code.
Understanding the Try Block in Python
In Python, the `try` block is a fundamental component of exception handling. It allows programmers to write code that can gracefully handle potential errors without crashing the program. The `try` block is used to wrap code that might generate an exception, allowing the developer to manage errors more effectively.
Structure of Try-Except Blocks
A typical structure of a try-except block includes the following components:
“`python
try:
Code that may raise an exception
except ExceptionType:
Code that runs if the exception occurs
“`
- `try`: This block contains the code that may raise an exception.
- `except`: This block executes if an exception occurs in the try block. You can specify the type of exception to catch.
Examples of Try-Except Usage
Here are some practical examples illustrating the use of try-except blocks:
“`python
Example 1: Handling division by zero
try:
result = 10 / 0
except ZeroDivisionError:
print(“Cannot divide by zero.”)
Example 2: Handling file operations
try:
with open(“nonexistent_file.txt”) as file:
content = file.read()
except FileNotFoundError:
print(“File not found.”)
“`
Multiple Except Blocks
You can have multiple `except` blocks to handle different types of exceptions:
“`python
try:
value = int(input(“Enter a number: “))
result = 10 / value
except ValueError:
print(“Invalid input; please enter a number.”)
except ZeroDivisionError:
print(“Cannot divide by zero.”)
“`
This structure allows for more granular control over different error types, improving the robustness of the code.
Finally Clause
A `finally` block can be added to ensure that specific code runs regardless of whether an exception occurred:
“`python
try:
file = open(“data.txt”, “r”)
data = file.read()
except FileNotFoundError:
print(“File not found.”)
finally:
file.close() Ensures the file is closed whether or not an exception occurred
“`
This is particularly useful for resource management, such as closing files or releasing resources.
Else Clause
An `else` clause can be included after all `except` blocks to execute code when no exceptions are raised:
“`python
try:
result = 10 / 2
except ZeroDivisionError:
print(“Cannot divide by zero.”)
else:
print(“Result is:”, result) Executes only if no exceptions occur
“`
Best Practices
When using try-except blocks, consider the following best practices:
- Catch specific exceptions: Always catch specific exceptions instead of using a general `except Exception`, as this can obscure other unrelated errors.
- Keep try blocks small: Limit the amount of code in the try block to only what may raise an exception, making debugging easier.
- Use finally for cleanup: Always use the `finally` block for cleanup activities, such as closing files or releasing resources.
The `try` block in Python is essential for writing robust applications that can handle errors gracefully. By utilizing try-except structures, along with `finally` and `else` clauses, developers can create more resilient code that enhances user experience and application stability.
Understanding the `try` Statement in Python: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The `try` statement in Python is essential for handling exceptions gracefully. It allows developers to write robust code that can anticipate and manage errors without crashing the program, thus improving user experience.”
Michael Chen (Python Developer and Educator, Code Academy). “Using the `try` block is a fundamental practice in Python programming. It helps encapsulate code that may raise exceptions, enabling the use of `except` clauses to define how to respond to specific errors, which ultimately leads to cleaner and more maintainable code.”
Sarah Johnson (Lead Data Scientist, Data Insights Corp.). “In data processing applications, the `try` statement is invaluable. It allows data scientists to handle unexpected data formats or missing values without halting the entire analysis, ensuring that insights can still be derived from available data.”
Frequently Asked Questions (FAQs)
What is try in Python?
The `try` statement in Python is used to catch and handle exceptions. It allows you to test a block of code for errors and define a response to those errors without crashing the program.
How does the try-except block work?
A `try-except` block consists of a `try` clause followed by one or more `except` clauses. The code inside the `try` block is executed, and if an exception occurs, the control is passed to the first matching `except` block.
Can I use multiple except blocks with a single try?
Yes, you can have multiple `except` blocks following a single `try` block. This allows you to handle different types of exceptions in specific ways, providing tailored error handling for various scenarios.
What is the purpose of the finally clause in a try block?
The `finally` clause is used to define a block of code that will execute regardless of whether an exception was raised or not. It is typically used for cleanup actions, such as closing files or releasing resources.
What is the difference between try-except and try-finally?
The `try-except` block is used to handle exceptions, while the `try-finally` block ensures that the code in the `finally` section runs after the `try` block, regardless of whether an exception occurred. They serve different purposes in error handling and resource management.
Can I use else with try-except in Python?
Yes, you can use an `else` clause with a `try-except` block. The code inside the `else` block will execute if the `try` block does not raise any exceptions, allowing you to separate normal execution from error handling.
The `try` statement in Python is a fundamental construct used for exception handling, allowing developers to manage errors gracefully and maintain the flow of execution in their programs. By encapsulating code that may raise exceptions within a `try` block, developers can specify how to respond to various error conditions without crashing the program. This is particularly useful in scenarios where user input or external resources may lead to unpredictable behavior.
When an exception occurs within the `try` block, the control is transferred to the corresponding `except` block, where specific actions can be taken to handle the error. This mechanism not only aids in debugging but also enhances user experience by providing meaningful feedback instead of abrupt termination of the program. Additionally, the `finally` block can be employed to execute cleanup actions, ensuring that resources are released appropriately regardless of whether an exception occurred.
Key takeaways from the discussion on the `try` statement include the importance of robust error handling in software development. By leveraging `try`, `except`, and `finally`, developers can create more resilient applications that can cope with unexpected situations. Furthermore, understanding how to effectively use these constructs is essential for writing clean, maintainable, and user-friendly code in Python.
Author Profile
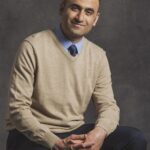
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?