How Can You Effectively Use the WHERE Clause with a CASE Statement in SQL?
In the world of SQL, crafting precise queries is essential for extracting meaningful insights from vast datasets. One powerful tool at your disposal is the combination of the WHERE clause and the CASE statement, which together allow for dynamic filtering based on specific conditions. Whether you’re analyzing sales data, customer interactions, or any other relational information, mastering this technique can significantly enhance your querying capabilities and improve the accuracy of your results.
The WHERE clause serves as a fundamental component of SQL queries, enabling users to specify criteria that filter records returned from a database. When combined with the CASE statement, which allows for conditional logic within your queries, you can create sophisticated filters that adapt based on varying conditions. This synergy not only simplifies complex queries but also enhances readability and maintainability, making it easier for others (and yourself) to understand the logic behind your data retrieval.
As we delve deeper into the intricacies of using a WHERE clause with a CASE statement, you’ll discover practical applications, best practices, and examples that illustrate how this powerful combination can be leveraged to meet diverse data analysis needs. Whether you’re a seasoned SQL developer or a newcomer eager to elevate your skills, understanding this concept will undoubtedly empower you to write more effective and dynamic SQL queries.
Using CASE Statements in WHERE Clauses
In SQL, the `CASE` statement allows for conditional logic within queries, and it can be effectively used within a `WHERE` clause to filter records based on complex conditions. This adds flexibility and enhances the power of SQL queries, enabling you to include multiple conditions that depend on various column values.
The syntax for incorporating a `CASE` statement within a `WHERE` clause generally follows this structure:
“`sql
SELECT column1, column2
FROM table_name
WHERE condition1
AND (CASE
WHEN condition2 THEN result1
WHEN condition3 THEN result2
ELSE result3
END) = some_value;
“`
Example of a CASE Statement in a WHERE Clause
Consider a scenario where you have a sales table that records the sales amount and sales type. You want to filter results based on specific criteria that depend on the sales type.
“`sql
SELECT sales_id, sales_amount, sales_type
FROM sales
WHERE (CASE
WHEN sales_type = ‘Retail’ AND sales_amount > 100 THEN ‘High’
WHEN sales_type = ‘Wholesale’ AND sales_amount > 500 THEN ‘High’
ELSE ‘Low’
END) = ‘High’;
“`
In this example, the `WHERE` clause evaluates the `CASE` statement, determining whether the sales record qualifies as ‘High’ based on the `sales_type` and `sales_amount`.
Advantages of Using CASE in WHERE Clauses
- Dynamic Filtering: Allows for more complex filtering conditions based on different criteria.
- Improved Readability: Simplifies the logic within the query, making it easier to understand.
- Reduced Complexity: Minimizes the need for multiple `OR` conditions by consolidating logic into a single statement.
Example Table for Reference
Here is a simple illustration of how different sales types might relate to sales amounts:
Sales Type | Sales Amount | Classification |
---|---|---|
Retail | 150 | High |
Wholesale | 600 | High |
Retail | 80 | Low |
Wholesale | 300 | Low |
Best Practices
When using `CASE` statements in `WHERE` clauses, consider the following best practices:
- Limit Complexity: Keep the `CASE` statement straightforward to maintain performance and clarity.
- Test Conditions: Always test the individual conditions to ensure they return the expected results.
- Use Aliases: If the query is complex, consider using aliases for better readability.
By leveraging `CASE` statements within `WHERE` clauses, you can create more powerful and dynamic SQL queries that can adapt to various data conditions effectively.
Understanding the WHERE Clause with CASE Statement
The `WHERE` clause in SQL is essential for filtering records based on specified conditions. Integrating a `CASE` statement within a `WHERE` clause allows for more dynamic and conditional filtering. The `CASE` statement evaluates conditions and returns a value based on which condition is met.
Syntax of WHERE Clause with CASE Statement
The basic syntax of using a `CASE` statement within a `WHERE` clause can be described as follows:
“`sql
SELECT column1, column2, …
FROM table_name
WHERE condition1
AND (CASE
WHEN condition2 THEN result1
WHEN condition3 THEN result2
ELSE result3
END) = desired_value;
“`
This structure enables the SQL query to filter results based on multiple conditions evaluated within the `CASE` statement.
Examples of WHERE Clause with CASE Statement
Here are some practical examples to illustrate how to implement a `CASE` statement within a `WHERE` clause.
Example 1: Conditional Filtering Based on Status
“`sql
SELECT employee_id, employee_name, status
FROM employees
WHERE department_id = 5
AND (CASE
WHEN status = ‘active’ THEN 1
WHEN status = ‘inactive’ THEN 0
ELSE -1
END) >= 0;
“`
In this example, the query retrieves employees from department 5 whose status is either ‘active’ or ‘inactive’.
Example 2: Using CASE for Dynamic Date Filtering
“`sql
SELECT order_id, order_date, customer_id
FROM orders
WHERE (CASE
WHEN order_status = ‘shipped’ THEN order_date
WHEN order_status = ‘pending’ THEN CURRENT_DATE
ELSE NULL
END) IS NOT NULL;
“`
This query filters orders based on their status. If the order is ‘shipped’, it checks the order date; if ‘pending’, it compares against the current date.
Advantages of Using CASE in WHERE Clauses
Utilizing a `CASE` statement within a `WHERE` clause provides several benefits:
- Enhanced Readability: It makes complex conditions easier to read and understand.
- Dynamic Filtering: It allows for flexible queries based on varying conditions without needing multiple `WHERE` clauses.
- Compact Code: Reduces the need for multiple logical operators and simplifies query construction.
Best Practices for Implementing WHERE Clause with CASE Statement
To optimize the use of `CASE` statements in `WHERE` clauses, consider the following best practices:
- Limit Complexity: Avoid excessive nesting of `CASE` statements to maintain clarity.
- Use Descriptive Aliases: When applicable, use aliases for easier reference in larger queries.
- Test Queries Thoroughly: Validate the logic by testing with various datasets to ensure expected results.
Using the `WHERE` clause with a `CASE` statement can significantly enhance the flexibility and power of your SQL queries, allowing for more tailored data retrieval based on complex conditions.
Expert Insights on Using WHERE Clause with CASE Statement in SQL
Dr. Emily Chen (Database Architect, Tech Solutions Inc.). “The use of a CASE statement within a WHERE clause can significantly enhance query flexibility by allowing conditional filtering. This approach is particularly useful when you need to apply different criteria based on varying conditions, thereby streamlining complex queries.”
Michael Thompson (Senior SQL Developer, Data Insights LLC). “Incorporating a CASE statement in the WHERE clause is an advanced technique that can optimize performance in certain scenarios. It allows developers to avoid multiple queries and instead condense logic into a single statement, which can lead to improved execution times.”
Sarah Patel (Business Intelligence Analyst, Analytics Corp). “Using a CASE statement within a WHERE clause can be particularly beneficial for data analysis tasks. It enables analysts to create dynamic filters that adapt based on the data context, facilitating more nuanced insights and reporting capabilities.”
Frequently Asked Questions (FAQs)
What is a WHERE clause in SQL?
The WHERE clause in SQL is used to filter records that meet specific conditions. It restricts the results returned by a query based on the criteria defined.
What is a CASE statement in SQL?
The CASE statement in SQL is a conditional expression that allows for if-then-else logic within SQL queries. It evaluates conditions and returns a specified value when the first condition is met.
How can I use a CASE statement within a WHERE clause?
You can use a CASE statement within a WHERE clause to dynamically determine the filtering criteria based on specific conditions. For example, you can evaluate a column’s value and apply different filters based on that evaluation.
Can you provide an example of a WHERE clause with a CASE statement?
Certainly. An example would be:
“`sql
SELECT * FROM Employees
WHERE Department = CASE
WHEN JobTitle = ‘Manager’ THEN ‘Sales’
WHEN JobTitle = ‘Developer’ THEN ‘IT’
ELSE ‘HR’
END;
“`
This query filters employees based on their job title.
Are there performance considerations when using CASE in a WHERE clause?
Yes, using a CASE statement in a WHERE clause can affect performance, especially with large datasets. It may lead to slower query execution if the conditions are complex or if indexes cannot be utilized effectively.
Can I use multiple CASE statements in a single WHERE clause?
Yes, you can use multiple CASE statements in a single WHERE clause. However, ensure that the logic remains clear and manageable to avoid confusion and maintain query performance.
The use of a WHERE clause with a CASE statement in SQL allows for conditional filtering of query results based on specific criteria. This technique enhances the flexibility of SQL queries, enabling developers to apply complex logic directly within the filtering process. By incorporating the CASE statement, users can evaluate multiple conditions and return different values, which can then be utilized to refine the dataset returned by the query.
One of the key advantages of using a CASE statement within a WHERE clause is the ability to handle various scenarios without the need for multiple queries or UNION operations. This approach simplifies the SQL code, making it more readable and maintainable. Furthermore, it allows for dynamic filtering based on the results of the CASE evaluation, which can be particularly useful in reports or dashboards where conditions may change based on user input or other variables.
In summary, combining a WHERE clause with a CASE statement is a powerful technique in SQL that enhances query capabilities. It provides a streamlined method for implementing conditional logic, thereby improving both the efficiency and clarity of SQL statements. As such, mastering this approach can significantly benefit SQL practitioners in crafting sophisticated queries that meet complex business requirements.
Author Profile
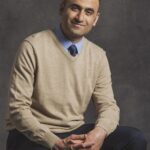
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?