Which of the Following Methods Outputs Data in a Python Program?
In the world of programming, the ability to effectively communicate information is paramount. For Python developers, understanding how to output data is not just a fundamental skill—it’s the gateway to creating interactive, user-friendly applications. Whether you’re developing a simple script or a complex software solution, knowing the various methods available for outputting data can significantly enhance the functionality and user experience of your program. In this article, we will explore the different ways to display data in Python, unraveling the tools and techniques that can help you convey your program’s results with clarity and precision.
Outputting data in Python encompasses a range of methods, each suited to different contexts and requirements. From the straightforward `print()` function to more advanced techniques like logging and formatting, Python offers a versatile toolkit for developers. Understanding these options not only helps in debugging and testing but also plays a crucial role in user interaction, making your applications more engaging and informative.
As we delve deeper into the various output methods, we will examine their unique features, advantages, and best use cases. Whether you are a beginner just starting your coding journey or an experienced developer looking to refine your skills, this exploration will equip you with the knowledge to choose the right output method for your specific needs. Prepare to elevate your Python programming by mastering the art of
Standard Output in Python
In Python, the most common way to output data is through the `print()` function. This function sends data to the standard output device, which is usually the console. It can accept multiple arguments, which allows for versatile formatting and output.
- Basic Usage:
“`python
print(“Hello, World!”)
“`
- Multiple Arguments: You can print multiple items by separating them with commas.
“`python
name = “Alice”
age = 30
print(“Name:”, name, “Age:”, age)
“`
- String Formatting: You can format strings using f-strings (Python 3.6 and later) for more readable code.
“`python
print(f”Name: {name}, Age: {age}”)
“`
Output to Files
Aside from the console, Python can also output data to files. This is done using the built-in `open()` function, which allows you to create a file object. The `write()` method can then be used to write data into the file.
- Writing to a Text File:
“`python
with open(‘output.txt’, ‘w’) as file:
file.write(“Hello, World!”)
“`
- Appending to a File: Use the mode ‘a’ to append data.
“`python
with open(‘output.txt’, ‘a’) as file:
file.write(“\nAppending this line.”)
“`
Logging Output
For more sophisticated output needs, especially for debugging or tracking application behavior, Python provides the `logging` module. This module allows you to log messages at different severity levels, including debug, info, warning, error, and critical.
- Basic Logging Setup:
“`python
import logging
logging.basicConfig(level=logging.INFO)
logging.info(“This is an informational message.”)
“`
- Log Levels:
Level | Description |
---|---|
DEBUG | Detailed information for debugging |
INFO | General information |
WARNING | Indicates a potential problem |
ERROR | Error events that might still allow the program to continue |
CRITICAL | Serious errors that prevent the program from continuing |
Graphical Output
For applications requiring graphical output, libraries such as Matplotlib can be utilized. This library enables the creation of charts, graphs, and other visual representations of data.
- Simple Plot Example:
“`python
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [10, 20, 25, 30]
plt.plot(x, y)
plt.title(‘Sample Plot’)
plt.xlabel(‘X-axis’)
plt.ylabel(‘Y-axis’)
plt.show()
“`
Python provides a variety of methods for outputting data, whether to the console, files, logs, or through graphical representations, allowing developers to select the most appropriate method for their specific needs.
Common Methods to Output Data in Python
In Python, there are several methods to output data, each serving different purposes depending on the context of the program. Below are the primary methods used for outputting data:
Print Function
The most straightforward way to output data in Python is using the built-in `print()` function. This method is commonly used for displaying information to the console.
- Syntax: `print(value, …, sep=’ ‘, end=’\n’, file=sys.stdout, flush=)`
Key parameters include:
- `value`: The data to be printed (can be multiple values).
- `sep`: String inserted between values (default is a space).
- `end`: String appended after the last value (default is a newline).
- `file`: Specifies the output stream (default is standard output).
- `flush`: Controls whether to flush the output buffer.
Example:
“`python
print(“Hello, World!”)
print(“Value:”, 42, sep=’ ‘)
“`
Formatted Output
For more control over how data is displayed, formatted output can be achieved using various methods such as f-strings, the `format()` method, or the older `%` operator.
- F-strings (Python 3.6 and later):
“`python
name = “Alice”
age = 30
print(f”{name} is {age} years old.”)
“`
- `format()` method:
“`python
print(“{} is {} years old.”.format(name, age))
“`
- Percent formatting:
“`python
print(“%s is %d years old.” % (name, age))
“`
Writing to Files
Outputting data can also involve writing to files. This is done using the `open()` function along with `write()` or `writelines()` methods.
Example:
“`python
with open(‘output.txt’, ‘w’) as f:
f.write(“Hello, World!\n”)
f.writelines([“Line 1\n”, “Line 2\n”])
“`
Logging Module
For applications that require tracking events or debugging information, the `logging` module provides a flexible framework for emitting log messages.
- Basic setup:
“`python
import logging
logging.basicConfig(level=logging.INFO)
logging.info(“This is an info message.”)
“`
Log levels include:
- `DEBUG`
- `INFO`
- `WARNING`
- `ERROR`
- `CRITICAL`
Outputting Data in GUI Applications
In graphical user interface (GUI) applications, data output is managed through widgets. For instance, in Tkinter, you can use labels or text boxes to display information.
Example using Tkinter:
“`python
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text=”Hello, GUI!”)
label.pack()
root.mainloop()
“`
Using Jupyter Notebooks
In Jupyter notebooks, the last expression in a cell is automatically outputted, which is useful for interactive data exploration. To display variables or results explicitly, you can use the `display()` function from the IPython library.
Example:
“`python
from IPython.display import display
data = {“A”: 1, “B”: 2}
display(data)
“`
Understanding these various output methods allows for effective communication of information within Python programs, whether for debugging, user interaction, or data logging. Each method has its specific use cases, and choosing the appropriate one enhances the clarity and functionality of the code.
Understanding Data Output in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). In Python programming, the primary functions responsible for outputting data are `print()` and `return`. The `print()` function displays information to the console, while `return` sends data back from a function to the caller, making both essential for effective data handling.
Michael Tran (Lead Software Engineer, CodeCraft Solutions). When discussing data output in Python, it is crucial to recognize that not only built-in functions like `print()` are used, but also file handling methods such as `write()` in file objects. These methods allow programmers to direct output to various destinations, enhancing the versatility of data presentation.
Jessica Liu (Data Scientist, Analytics Hub). In the context of data output, Python also supports libraries like Matplotlib and Pandas, which provide advanced capabilities for visualizing and exporting data. These tools are invaluable for presenting data insights in a more digestible format beyond simple console output.
Frequently Asked Questions (FAQs)
Which of the following outputs data in a Python program?
The primary function used to output data in a Python program is `print()`. It displays the specified message or variables to the console.
Can you use other methods to output data in Python?
Yes, besides `print()`, you can use file handling methods to write output to files, such as `write()` for file objects. Additionally, libraries like `logging` can also output data to various destinations.
What is the difference between print() and logging in Python?
`print()` is used for simple output to the console, while `logging` provides a more robust framework for tracking events, errors, and warnings, allowing for different log levels and output formats.
Are there any built-in functions for formatted output in Python?
Yes, Python provides several ways for formatted output, including f-strings (formatted string literals), the `format()` method, and the `%` operator for string formatting.
Can you output data to a graphical user interface (GUI) in Python?
Yes, libraries like Tkinter, PyQt, and Kivy allow you to create GUIs where you can output data through various widgets such as labels, text boxes, and message boxes.
Is it possible to redirect output in Python?
Yes, you can redirect output by changing the `sys.stdout` to a different file or stream, allowing you to capture printed output to a file or another output stream instead of the console.
In Python programming, various methods and functions are utilized to output data effectively. The most common way to display information is through the use of the `print()` function, which allows developers to send data to the standard output, typically the console. This function can handle multiple data types, including strings, integers, and lists, making it versatile for various programming scenarios.
Additionally, Python offers other output methods such as logging, writing to files, and utilizing graphical user interfaces (GUIs). The `logging` module provides a way to output data with different severity levels, which is particularly useful for debugging and monitoring applications. Writing to files allows for data persistence, enabling programmers to save output for later analysis or record-keeping. GUIs can also be employed to present data visually, enhancing user interaction and experience.
Overall, understanding the different ways to output data in Python is crucial for effective programming. Each method serves specific purposes and can be selected based on the context of the application being developed. Mastery of these output techniques can significantly improve the quality and usability of Python programs.
Author Profile
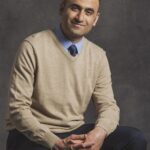
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?