What Symbol Do You Use in Python to Create a Comment?
In the world of programming, clarity and communication are paramount. As developers craft intricate lines of code, the need to annotate and explain their thought processes becomes essential. Enter the humble comment—an invaluable tool that allows programmers to leave notes, clarify intentions, and enhance the readability of their code. In Python, a language celebrated for its simplicity and elegance, comments play a crucial role in making code more understandable for both the original author and others who may encounter it later. But what exactly is the symbol that enables this functionality in Python?
Comments in Python serve as a means to document code without affecting its execution. They allow developers to include explanations, reminders, or even temporary notes that can be easily ignored by the interpreter. This practice not only aids in personal understanding but also fosters collaboration among teams, ensuring that everyone is on the same page. As we delve deeper into the mechanics of Python comments, we will explore how they can be effectively utilized to enhance your coding experience.
Understanding the syntax and purpose of comments is foundational for anyone looking to master Python. Whether you are a novice programmer or a seasoned developer, knowing how to create and manage comments can significantly improve your code’s maintainability and clarity. So, let’s uncover the symbol that unlocks this essential aspect of Python
Commenting in Python
In Python, comments are crucial for enhancing code readability and maintainability. They allow developers to explain their code, provide context, or temporarily disable code without removing it. The primary symbol used to create a comment in Python is the hash symbol (“).
When a hash symbol precedes text, Python ignores everything on that line after the “. This allows developers to include annotations or notes directly within the code. Here are some key points regarding comments in Python:
- Single-line comments: These comments start with the “ symbol and extend to the end of the line. Example:
“`python
This is a single-line comment
print(“Hello, World!”) This prints a message
“`
- Multi-line comments: Although Python does not have a specific syntax for multi-line comments, developers often use triple quotes (`”’` or `”””`). However, this method is technically a multi-line string and not a comment per se. Example:
“`python
“””
This is a multi-line comment
that spans multiple lines.
“””
print(“Hello, World!”)
“`
- Docstrings: These are a type of comment used to describe a function, class, or module. They are defined using triple quotes and are accessible via the `__doc__` attribute. Example:
“`python
def example_function():
“””This function does nothing.”””
pass
“`
Best Practices for Commenting
Effective commenting practices can significantly enhance the quality of the code. Here are some best practices to consider:
- Use comments to explain why something is done, not what is done, as the code should be self-explanatory.
- Keep comments up-to-date to reflect any changes in the code.
- Avoid obvious comments that do not add value or clarity.
- Use consistent formatting for comments to maintain a uniform style across the codebase.
Comment Type | Symbol | Description |
---|---|---|
Single-line comment | Used for brief explanations or notes on a single line. | |
Multi-line comment | ”’ or “”” | Used to comment out blocks of text, typically for descriptions. |
Docstring | ”’ or “”” | Specifically for documenting functions, classes, or modules. |
By adhering to these commenting conventions and best practices, programmers can create more understandable and maintainable code, facilitating collaboration and future modifications.
Commenting in Python
In Python, comments are used to provide explanations or annotations within the code, making it easier to understand for both the original author and other developers. The primary symbol used to create comments in Python is the hash or pound sign (“).
Types of Comments
Python supports two main types of comments:
- Single-line Comments:
- These comments are initiated with the “ symbol. Everything following this symbol on the same line is treated as a comment and will not be executed by the Python interpreter.
- Example:
“`python
This is a single-line comment
print(“Hello, World!”) This comment is after a line of code
“`
- Multi-line Comments:
- While Python does not have a specific syntax for multi-line comments, developers often use triple quotes (`”’` or `”””`) to achieve this effect. These strings are not assigned to a variable, hence they are ignored by the interpreter.
- Example:
“`python
“””
This is a multi-line comment.
It can span multiple lines.
“””
print(“Hello, World!”)
“`
Best Practices for Commenting
Effective commenting is crucial for maintaining code readability and clarity. Consider the following best practices:
- Be Concise:
- Comments should be clear and to the point. Avoid unnecessary verbosity.
- Explain Why, Not What:
- Focus on the reasoning behind complex logic rather than stating what the code does, as the code itself should be self-explanatory.
- Update Comments:
- Ensure that comments are updated alongside code changes to prevent them from becoming misleading.
- Avoid Obvious Comments:
- Do not comment on trivial lines of code where the purpose is obvious, such as simple print statements.
Examples of Comments
The following table illustrates various examples of comments in Python:
Comment Type | Example Code | Explanation |
---|---|---|
Single-line | `Initialize counter` | This comment describes the purpose of the following code. |
After Code | `total += 1 Increment total` | This comment clarifies what the operation is doing. |
Multi-line | `””” This function computes the sum. “””` | A description of the function’s purpose. |
By adhering to these commenting conventions, developers can create more maintainable and understandable code, facilitating collaboration and future code enhancements.
Understanding Comments in Python Programming
Dr. Emily Carter (Senior Software Engineer, CodeMaster Inc.). “In Python, the symbol used to create a comment is the hash symbol (). This allows developers to annotate their code, making it easier to understand and maintain.”
James Liu (Lead Python Developer, Tech Innovations). “The use of the hash symbol () for comments in Python is crucial for effective code documentation. It enables programmers to leave notes without affecting the execution of the code.”
Maria Gonzalez (Computer Science Educator, Future Coders Academy). “Understanding that the hash symbol () is used for comments in Python is fundamental for beginners. It helps them learn to communicate their thought processes within the code.”
Frequently Asked Questions (FAQs)
Which symbol is used in Python to create a comment?
The hash symbol “ is used in Python to create a comment. Any text following this symbol on the same line is ignored by the interpreter.
Can comments be placed on the same line as code in Python?
Yes, comments can be placed on the same line as code by using the “ symbol. Everything after the “ will be treated as a comment.
Are there multi-line comments in Python?
Python does not have a specific multi-line comment symbol. However, multi-line strings enclosed in triple quotes `”’` or `”””` can be used as comments, although they are technically string literals.
What is the purpose of comments in Python?
Comments are used to explain code, making it easier to understand for others or for the original author when revisiting the code later. They do not affect the execution of the program.
Can comments be used to temporarily disable code in Python?
Yes, comments can be used to temporarily disable code by placing the “ symbol before the code line. This prevents the line from executing while retaining it in the source code.
Is there a specific style guide for writing comments in Python?
Yes, the PEP 8 style guide recommends writing comments that are clear and concise. It advises using full sentences and proper capitalization for readability.
In Python, comments are created using the hash symbol (). This symbol indicates that the text following it on the same line is a comment and will not be executed as part of the program. Comments are essential in programming as they allow developers to annotate their code, making it easier to understand for themselves and others who may read it later. They can explain the purpose of a code block, provide context for complex logic, or serve as reminders for future modifications.
There are two primary types of comments in Python: single-line comments and multi-line comments. Single-line comments begin with the hash symbol and continue to the end of the line. For multi-line comments, developers can use triple quotes (”’ or “””) to create a block of text that is treated as a comment. This flexibility allows for clear documentation of code, which is a best practice in software development.
In summary, the hash symbol () is the key character used in Python to create comments. Understanding how to effectively use comments enhances code readability and maintainability, which are critical components of professional programming. By incorporating comments thoughtfully, developers can improve collaboration and reduce the learning curve for future code reviewers.
Author Profile
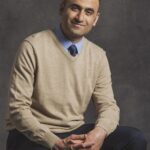
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?