Why Do We Use If/Else Statements in JavaScript: What Makes Them Essential?
### Introduction
In the dynamic world of programming, decision-making is a fundamental concept that drives the functionality of applications. Among the various tools at a developer’s disposal, the `if/else` statement in JavaScript stands out as a powerful mechanism for controlling the flow of code execution. Whether you’re building a simple webpage or a complex web application, understanding how to effectively use `if/else` statements can significantly enhance your ability to create interactive and responsive user experiences. But why do we rely on these statements so heavily?
At its core, the `if/else` statement allows developers to introduce conditional logic into their code, enabling programs to respond differently based on varying inputs or states. This capability is essential for creating dynamic applications that can adapt to user actions or external data. By evaluating conditions and executing specific blocks of code accordingly, developers can ensure that their applications behave in a logical and predictable manner, which is crucial for user satisfaction and functionality.
Moreover, the versatility of `if/else` statements extends beyond simple binary choices. They can be nested, combined with logical operators, and even integrated with other control structures to create complex decision-making pathways. This flexibility empowers developers to implement sophisticated algorithms and workflows, making `if/else` statements an indispensable part of JavaScript programming
Understanding If/Else Statements
If/else statements are fundamental control structures in JavaScript that allow developers to execute different blocks of code based on specified conditions. This conditional logic enables dynamic behavior in applications, making them responsive to user inputs, data changes, and other runtime conditions.
When an if/else statement is evaluated, the JavaScript engine checks the condition specified in the if clause. If the condition evaluates to true, the code block within the if statement is executed. If it evaluates to , the code block within the else statement (if present) is executed. This branching logic is crucial for creating applications that can handle various scenarios and outcomes.
Syntax of If/Else Statements
The basic syntax of an if/else statement in JavaScript is as follows:
javascript
if (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is
}
In addition to the basic structure, developers can also implement multiple conditions using else if statements:
javascript
if (condition1) {
// code if condition1 is true
} else if (condition2) {
// code if condition2 is true
} else {
// code if neither condition1 nor condition2 is true
}
Benefits of Using If/Else Statements
The advantages of using if/else statements include:
- Decision Making: They allow the program to choose different paths based on conditions, making the code dynamic.
- Control Flow: They help manage the execution flow of the program based on logical conditions.
- Readability: The structure improves code readability by clearly outlining decision points.
Condition | Action Taken |
---|---|
Condition is true | Execute the code in the if block |
Condition is | Execute the code in the else block (if present) |
Common Use Cases
If/else statements are widely used in various scenarios, including but not limited to:
- User Authentication: Checking if a user has entered the correct credentials before granting access.
- Form Validation: Validating user inputs in forms to ensure data integrity.
- Feature Toggles: Enabling or disabling features based on user roles or preferences.
By leveraging if/else statements effectively, developers can create applications that are not only functional but also intuitive and user-friendly.
Purpose of If/Else Statements
If/else statements are fundamental control structures in JavaScript that enable developers to direct the flow of execution based on specific conditions. Their main purposes include:
- Decision Making: They allow programs to execute different blocks of code based on whether a condition evaluates to true or .
- Conditional Logic: Complex logic can be implemented by nesting multiple if/else statements, creating a robust decision-making framework.
- Error Handling: They facilitate error checking and handling, enabling developers to manage unexpected inputs or conditions effectively.
Syntax of If/Else Statements
The basic syntax of if/else statements in JavaScript is straightforward:
javascript
if (condition) {
// code to execute if condition is true
} else {
// code to execute if condition is
}
Multiple conditions can be checked using `else if`:
javascript
if (condition1) {
// code for condition1
} else if (condition2) {
// code for condition2
} else {
// code if none of the conditions are true
}
Use Cases of If/Else Statements
The flexibility of if/else statements allows them to be used in various scenarios, such as:
- User Input Validation: Ensuring that user inputs meet certain criteria before processing.
- Feature Toggles: Enabling or disabling features based on user roles or configurations.
- Dynamic Content Display: Showing or hiding elements on a web page based on user actions or state.
Examples of If/Else Statements
Here are some practical examples illustrating the use of if/else statements:
- **Basic User Age Validation**:
javascript
let age = 18;
if (age >= 18) {
console.log(“You are eligible to vote.”);
} else {
console.log(“You are not eligible to vote.”);
}
- **Temperature Check**:
javascript
let temperature = 30;
if (temperature > 25) {
console.log(“It’s a hot day.”);
} else if (temperature <= 25 && temperature >= 15) {
console.log(“It’s a pleasant day.”);
} else {
console.log(“It’s a cold day.”);
}
Best Practices
To ensure effective use of if/else statements, consider the following best practices:
- Keep Conditions Simple: Complex conditions can reduce readability. Break them into smaller functions when necessary.
- Avoid Deep Nesting: Limit the depth of nested if/else statements to maintain clarity. Use switch statements for multiple conditions when appropriate.
- Use Descriptive Conditionals: Use clear and descriptive variable names to enhance the code’s readability and maintainability.
Performance Considerations
While if/else statements are efficient for decision-making, there are considerations to keep in mind:
- Short-Circuit Evaluation: JavaScript evaluates conditions in order and stops at the first true condition, which can optimize performance in some cases.
- Complex Conditions: Overly complex boolean expressions can lead to slower evaluations. Simplifying these can improve performance.
- Profiling: Use profiling tools to assess if/else performance in critical sections of code, especially within loops.
If/Else Statement Usage
Understanding the utility and structure of if/else statements is essential for effective JavaScript programming. They serve as a crucial tool for implementing logic that responds to varying conditions, thereby enhancing the interactivity and functionality of applications.
The Importance of If/Else Statements in JavaScript Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “If/else statements are fundamental to controlling the flow of execution in JavaScript. They allow developers to implement decision-making logic, enabling programs to respond dynamically to different conditions and user inputs.”
Michael Chen (JavaScript Educator, Code Academy). “Using if/else statements is crucial for writing clear and maintainable code. They provide a structured way to handle multiple scenarios, making it easier for developers to understand and manage the logic of their applications.”
Sarah Thompson (Lead Frontend Developer, Creative Solutions Inc). “In JavaScript, if/else statements are essential for implementing business logic. They enable conditional rendering in web applications, allowing developers to create interactive and responsive user experiences.”
Frequently Asked Questions (FAQs)
Why do we use if/else statements in JavaScript?
If/else statements allow developers to execute different blocks of code based on specific conditions, enabling dynamic decision-making in applications.
What is the syntax of an if/else statement in JavaScript?
The syntax consists of the `if` keyword followed by a condition in parentheses, and a block of code in curly braces. The `else` keyword can be used to define an alternative block of code that executes if the condition is .
Can if/else statements be nested in JavaScript?
Yes, if/else statements can be nested within each other, allowing for multiple layers of conditions to be evaluated sequentially.
What are the alternatives to if/else statements in JavaScript?
Alternatives include the ternary operator for simple conditions and switch statements for handling multiple cases based on a single expression.
How do if/else statements improve code readability?
They enhance readability by clearly outlining the logic flow, making it easier for developers to understand the conditions under which specific code executes.
Are there performance implications when using if/else statements?
While if/else statements are generally efficient, excessive nesting or complex conditions can lead to decreased performance. It’s important to structure conditions logically for optimal efficiency.
If/else statements in JavaScript serve as fundamental control structures that enable developers to implement conditional logic within their code. By allowing the execution of specific blocks of code based on whether a condition evaluates to true or , these statements facilitate decision-making processes in programming. This capability is essential for creating dynamic and responsive applications, as it enables the code to react differently under varying circumstances.
The use of if/else statements enhances the readability and maintainability of code by clearly outlining the logic flow. Developers can structure their code to handle various scenarios, which not only makes it easier to understand but also simplifies debugging and future modifications. Furthermore, these statements can be nested or combined with other logical operators, providing a robust framework for handling complex conditions and branching paths in the application logic.
In summary, if/else statements are indispensable in JavaScript for implementing conditional operations. They empower developers to create more interactive and user-friendly applications by allowing the code to adapt based on user input or other variables. Mastery of these control structures is crucial for anyone looking to excel in JavaScript programming, as they form the backbone of decision-making in code execution.
Author Profile
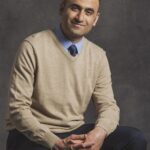
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?