Why Do We Use While Loops in JavaScript: Understanding Their Importance and Application?
### Introduction
In the world of programming, efficiency and control are paramount, and JavaScript offers a plethora of tools to achieve both. Among these tools, the while loop stands out as a fundamental construct that allows developers to execute a block of code repeatedly based on a specified condition. But why do we use while loops in JavaScript? This question opens the door to understanding not just the mechanics of looping, but also the broader principles of flow control and problem-solving in coding. As we delve deeper into this topic, we will explore the scenarios where while loops shine, their syntax, and the unique advantages they offer in crafting dynamic and responsive applications.
While loops are essential for scenarios where the number of iterations is not known ahead of time. This flexibility makes them particularly useful in situations that require continuous checks, such as processing user input or iterating through data until a certain condition is met. Unlike for loops, which are often used when the number of iterations is predetermined, while loops can adapt to varying conditions, making them a powerful tool for developers looking to create robust and efficient code.
Moreover, understanding the use of while loops can enhance your overall programming skill set. They encourage a deeper comprehension of logical conditions and control flow, which are critical in building complex applications. By mastering while loops
Understanding the While Loop
The while loop in JavaScript is a fundamental construct that enables the execution of a block of code repeatedly as long as a specified condition remains true. This makes it particularly useful in scenarios where the number of iterations is not known in advance.
When to Use While Loops
While loops are ideal in situations where:
- The number of iterations is uncertain or depends on dynamic conditions.
- Continuous checks are required to ensure that certain criteria are met before proceeding.
- Simplifying code for scenarios where a condition must be evaluated before each iteration.
For instance, while processing user input or waiting for a specific event to occur, while loops maintain flexibility and control.
Syntax of a While Loop
The syntax for a while loop is straightforward:
javascript
while (condition) {
// code to be executed
}
- condition: A boolean expression that is evaluated before each iteration. If it evaluates to true, the loop continues; if , the loop terminates.
Example of a While Loop
Consider the following example where we print numbers from 1 to 5 using a while loop:
javascript
let number = 1;
while (number <= 5) {
console.log(number);
number++;
}
In this case, the loop will continue executing until the value of `number` exceeds 5.
Comparison with Other Loops
While loops can often be compared with other loop constructs like for loops and do-while loops. Here’s a brief comparison:
Loop Type | Condition Check | Use Case |
---|---|---|
While Loop | Before each iteration | When the number of iterations is unknown |
For Loop | Before each iteration | When the number of iterations is known |
Do-While Loop | After each iteration | When the code must run at least once |
Common Pitfalls
While loops can lead to infinite loops if the condition never evaluates to . This typically occurs due to:
- Missing or incorrect updates to the loop variable.
- Logic errors in the condition.
To prevent these pitfalls, ensure that:
- The condition will eventually become .
- The loop variable is updated correctly within the loop.
By understanding these principles and applying while loops appropriately, developers can create efficient and effective JavaScript applications.
Purpose of While Loops in JavaScript
While loops are fundamental control structures in JavaScript that allow for repeated execution of a block of code as long as a specified condition evaluates to true. They provide a way to perform tasks that require iteration, especially when the number of iterations is not known beforehand.
Scenarios for Using While Loops
While loops are particularly useful in various programming scenarios:
- Dynamic Iteration: When the number of iterations is dependent on user input or external data, while loops can adapt accordingly.
- Event Handling: Continuously checking for a condition, such as waiting for user actions or responses, can be efficiently managed with while loops.
- Data Processing: Processing items in a collection until a certain condition is met, for instance, reading from a stream until the end is reached.
Syntax of While Loops
The basic structure of a while loop in JavaScript is as follows:
javascript
while (condition) {
// code block to be executed
}
- condition: A boolean expression that is evaluated before each iteration.
- code block: The statements that are executed as long as the condition remains true.
Example of a While Loop
Consider an example where we want to print numbers from 1 to 5:
javascript
let number = 1;
while (number <= 5) {
console.log(number);
number++;
}
In this example, the loop continues until the variable `number` exceeds 5, demonstrating how a while loop operates under a dynamic condition.
Advantages of While Loops
While loops come with several advantages:
- Flexibility: They can handle situations where the number of iterations is not predetermined.
- Simplicity: The syntax is straightforward and easy to understand, making it accessible for beginners.
- Control: Programmers have fine-grained control over the loop’s execution, allowing for complex conditions.
Potential Pitfalls of While Loops
While loops can lead to issues if not used carefully:
- Infinite Loops: If the condition never becomes , the loop will run indefinitely, leading to performance issues.
- Complex Conditions: Complicated conditions can make it difficult to understand the loop’s behavior, which can introduce bugs.
Best Practices for Using While Loops
To ensure effective use of while loops, consider the following best practices:
- Initialize Variables: Ensure that any variables used in the condition are properly initialized before the loop starts.
- Update Conditions: Always modify the condition within the loop to avoid infinite loops.
- Test Conditions: Use simple, clear conditions to improve readability and maintainability.
Comparative Analysis with Other Loop Constructs
Feature | While Loop | For Loop | Do…While Loop |
---|---|---|---|
Iteration Control | Condition checked before execution | Initialization, condition, and increment defined in one line | Condition checked after execution |
Use Case | Unknown number of iterations | Known number of iterations | At least one execution required |
Readability | Can be less readable for complex conditions | Generally more concise for counting | Clear for scenarios needing at least one execution |
By understanding the purpose and functionality of while loops, developers can leverage their capabilities effectively in JavaScript programming.
Understanding the Importance of While Loops in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “While loops in JavaScript are essential for scenarios where the number of iterations is not known beforehand. They allow developers to execute a block of code repeatedly as long as a specified condition remains true, making them ideal for tasks such as processing user input or iterating through data until a certain state is reached.”
Michael Chen (Lead JavaScript Developer, CodeCraft Solutions). “The flexibility of while loops is one of their greatest strengths. Unlike for loops, which require a predetermined number of iterations, while loops can adapt to varying conditions, enabling dynamic programming approaches that can respond to real-time data changes or user interactions.”
Sarah Patel (JavaScript Educator, Web Development Academy). “While loops are a fundamental concept in JavaScript that help beginners grasp the principles of control flow and iteration. Understanding how to implement while loops effectively lays the groundwork for more complex programming constructs and enhances problem-solving skills in coding.”
Frequently Asked Questions (FAQs)
Why do we use while loops in JavaScript?
While loops are used in JavaScript to execute a block of code repeatedly as long as a specified condition evaluates to true. This allows for dynamic and flexible iteration based on real-time conditions.
How does a while loop differ from a for loop in JavaScript?
A while loop continues until its condition is , making it suitable for situations where the number of iterations is not predetermined. In contrast, a for loop is typically used when the number of iterations is known beforehand.
What is the syntax of a while loop in JavaScript?
The syntax of a while loop consists of the `while` keyword followed by a condition in parentheses, and a block of code in curly braces. For example: `while (condition) { // code to execute }`.
Can a while loop be used to create an infinite loop?
Yes, a while loop can create an infinite loop if the condition always evaluates to true and there is no break statement or exit condition within the loop. This can lead to performance issues if not handled properly.
What are common use cases for while loops in JavaScript?
Common use cases for while loops include reading data until a certain condition is met, implementing user-driven processes, and performing tasks where the number of iterations is not known in advance, such as waiting for user input.
How can we prevent an infinite loop when using a while loop?
To prevent an infinite loop, ensure that the loop’s condition will eventually evaluate to by modifying variables within the loop. Additionally, implementing a break statement can provide a controlled exit from the loop.
While loops are an essential construct in JavaScript that allow developers to execute a block of code repeatedly as long as a specified condition evaluates to true. This capability is particularly useful for scenarios where the number of iterations is not known in advance, enabling dynamic control over the flow of execution. By utilizing while loops, programmers can effectively manage tasks such as iterating through data structures, processing user input, or implementing algorithms that require repeated actions until a certain state is achieved.
One of the primary advantages of while loops is their flexibility. Unlike for loops, which are often used when the number of iterations is predetermined, while loops provide the freedom to continue looping based on conditions that may change during execution. This makes them ideal for situations where the loop’s termination depends on external factors, such as user interactions or the results of computations that evolve over time.
Moreover, while loops contribute to cleaner and more maintainable code. By encapsulating repetitive logic within a while loop, developers can avoid code duplication and enhance readability. This encapsulation not only simplifies debugging but also allows for easier modifications in the future, as changes to the loop’s logic can be made in one central location rather than throughout the codebase.
while loops
Author Profile
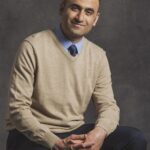
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?