Why Do We Use While Loops in Python? Exploring Their Importance and Use Cases
In the world of programming, efficiency and control are paramount, and Python provides a versatile tool to achieve both: the while loop. Imagine a scenario where you need to perform a task repeatedly until a certain condition is met—this is where while loops shine. They allow developers to create dynamic and flexible code that can adapt to varying input and conditions, making them an essential component of any programmer’s toolkit. Whether you’re automating a process, managing user input, or implementing complex algorithms, understanding the power of while loops can significantly enhance your coding prowess.
While loops in Python are designed to execute a block of code as long as a specified condition remains true. This feature is particularly useful for scenarios where the number of iterations isn’t predetermined, allowing for greater adaptability in your programs. For instance, you might use a while loop to keep prompting a user for input until they provide a valid response or to continuously check for changes in a data stream. The ability to control the flow of execution based on real-time conditions makes while loops a powerful construct for both novice and experienced programmers alike.
Moreover, while loops foster a deeper understanding of logical conditions and control structures in programming. By mastering their use, developers can create more efficient algorithms and streamline their code, reducing redundancy and improving readability.
Understanding the Purpose of While Loops in Python
While loops in Python serve a critical function in programming, enabling developers to execute a block of code repeatedly as long as a specified condition remains true. This mechanism is particularly useful for scenarios where the number of iterations is not predetermined, allowing for greater flexibility in coding.
The primary reasons for utilizing while loops include:
- Dynamic Iteration: Unlike for loops, which iterate over a fixed sequence, while loops continue until a condition changes, making them ideal for tasks that require indefinite repetition.
- Condition-Based Execution: They enable execution based on conditions that may change during runtime, such as user input or variable states.
- Simplifying Complex Logic: They can simplify complex algorithms that require repeated checks of conditions, enhancing code readability and maintainability.
Structure and Syntax of While Loops
The basic syntax of a while loop in Python is straightforward:
“`python
while condition:
code block
“`
In this structure, the loop will continue executing the code block as long as the `condition` evaluates to `True`. If the condition is “, the loop terminates, and control moves to the next statement following the loop.
Here is a simple example:
“`python
count = 0
while count < 5:
print(count)
count += 1
```
This code will print numbers from 0 to 4. Once `count` reaches 5, the condition evaluates to ``, and the loop exits.
Use Cases for While Loops
While loops are particularly effective in various scenarios, including:
- User Input Validation: Continuously prompting the user until valid input is received.
- Game Loops: Running game logic until a certain game state is reached, such as a win or loss condition.
- Data Processing: Iterating over datasets where the total size may not be known beforehand.
Comparison of While Loops and For Loops
While loops and for loops serve different purposes, and understanding their differences can help in deciding which to use in specific situations. The following table outlines key distinctions:
Feature | While Loop | For Loop |
---|---|---|
Iteration Count | Indeterminate | Determinate |
Use Case | Condition-based repetition | Iterating over a sequence or collection |
Control Structure | Requires manual condition update | Automatically iterates over elements |
Understanding these differences is crucial for selecting the appropriate loop based on the specific requirements of a task.
while loops are a powerful feature in Python that can enhance the flexibility and dynamism of your code. They are particularly useful in situations where the iteration count is not known in advance, enabling developers to write more adaptable and efficient programs.
Purpose of While Loops in Python
While loops are fundamental constructs in Python that allow for repeated execution of a block of code as long as a specified condition remains true. They are particularly useful in scenarios where the number of iterations is not predetermined.
Key Features of While Loops
- Condition-Driven Execution: The loop continues executing as long as the condition evaluates to true.
- Dynamic Iteration Control: The number of iterations can vary based on real-time conditions or user input.
- Early Termination: The loop can be exited prematurely using the `break` statement.
Common Use Cases for While Loops
While loops are employed in various programming situations, including:
- User Input Validation: Repeatedly prompt the user until valid input is received.
- Game Loops: Maintain an active game state until a certain condition (like a win or lose state) occurs.
- Data Processing: Iterate over data until all relevant data is processed or a specific condition is met.
Syntax of a While Loop
The basic structure of a while loop in Python is as follows:
“`python
while condition:
Block of code to be executed
“`
Example
“`python
count = 0
while count < 5:
print(count)
count += 1
```
This loop will output numbers 0 to 4, incrementing the `count` variable until the condition `count < 5` is no longer true.
Advantages of Using While Loops
- Flexibility: They allow for complex iteration control based on dynamic conditions.
- Simplicity: Easy to read and understand, especially for simple conditions.
- Efficiency in Certain Scenarios: They can be more efficient than other loops for certain tasks, such as when the number of iterations is not known beforehand.
Disadvantages and Considerations
While loops can lead to several issues if not used correctly, such as:
- Infinite Loops: If the condition never becomes , the loop will execute indefinitely.
- Complexity: They can become difficult to read and maintain when the condition is complex or involves multiple variables.
Example of an Infinite Loop
“`python
while True:
Code that never breaks the loop
“`
This loop will run forever unless explicitly terminated.
Best Practices
To utilize while loops effectively, consider the following best practices:
- Ensure that the loop has a clear exit condition.
- Use `break` and `continue` statements judiciously to control flow.
- Limit the complexity of the loop condition for better readability.
- Always test loops to prevent unintended infinite executions.
By adhering to these guidelines, developers can harness the power of while loops while maintaining code quality and integrity.
The Importance of While Loops in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “While loops are fundamental in Python because they allow for repeated execution of a block of code as long as a specified condition remains true. This is particularly useful for scenarios where the number of iterations is not known in advance, such as processing user input or iterating through data until a certain condition is met.”
Michael Chen (Lead Data Scientist, Data Insights Group). “The versatility of while loops in Python makes them invaluable for data manipulation tasks. They enable developers to create dynamic algorithms that can adapt to varying datasets, ensuring that operations continue until all necessary data has been processed, which is essential in data analysis and machine learning workflows.”
Sarah Patel (Python Instructor, Code Academy). “In educational settings, while loops serve as an excellent teaching tool for beginners. They illustrate the concept of iteration and condition checking in programming, helping students understand how to control the flow of their code effectively. This foundational knowledge is crucial for more advanced programming concepts.”
Frequently Asked Questions (FAQs)
Why do we use while loops in Python?
While loops are used in Python to execute a block of code repeatedly as long as a specified condition remains true. This allows for dynamic iteration where the number of iterations is not predetermined.
What is the syntax of a while loop in Python?
The syntax of a while loop in Python is:
“`python
while condition:
code block to execute
“`
The loop continues to execute the code block until the condition evaluates to .
How does a while loop differ from a for loop?
A while loop continues until a condition is no longer met, while a for loop iterates over a sequence (like a list or range) for a fixed number of iterations. This makes while loops more suitable for scenarios where the number of iterations is unknown beforehand.
What are some common use cases for while loops?
Common use cases for while loops include reading data until EOF, waiting for user input until a valid response is received, and implementing game loops where the game continues until a specific condition is met.
What can cause an infinite loop in a while loop?
An infinite loop can occur if the condition in the while loop never evaluates to . This often happens when the loop’s internal state is not updated correctly, preventing the condition from changing.
How can you exit a while loop prematurely?
You can exit a while loop prematurely using the `break` statement. This immediately terminates the loop, allowing the program to continue with the next statement following the loop.
While loops in Python are a fundamental control flow structure that allows for the repeated execution of a block of code as long as a specified condition remains true. This feature is particularly useful for scenarios where the number of iterations is not predetermined, enabling developers to create flexible and dynamic programs. By utilizing while loops, programmers can efficiently handle tasks such as data processing, user input validation, and iterative calculations without the need for explicit counter variables.
One of the significant advantages of while loops is their ability to facilitate continuous execution until a specific condition is met. This characteristic is particularly beneficial in situations where the termination condition may depend on user input or external factors, making while loops an essential tool for interactive applications. Additionally, while loops can simplify code readability by reducing the need for complex indexing or counter management, allowing developers to focus on the logic of their programs.
In summary, while loops are an integral part of Python programming that provide a powerful mechanism for iteration. They enhance the flexibility of code execution and improve the handling of dynamic conditions. By understanding and effectively employing while loops, programmers can create more efficient and user-friendly applications that respond appropriately to varying inputs and conditions.
Author Profile
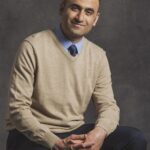
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?