Why Does My Tool Keep Falling Down in Lua? Unraveling the Mystery!
If you’ve ever found yourself immersed in the world of Lua programming, you may have encountered a perplexing problem: your tool, whether it’s a game object, a UI element, or a physics simulation, just won’t stay upright. This seemingly simple issue can lead to frustration, especially when you’re trying to create a seamless experience for users. Understanding why your tool falls down in Lua is not just about fixing a bug; it’s about grasping the underlying principles of physics, object manipulation, and the Lua environment itself. In this article, we will delve into the common reasons behind this phenomenon and equip you with the knowledge to keep your creations standing tall.
As you navigate the intricacies of Lua, it’s essential to recognize that the behavior of your tools is influenced by a myriad of factors, including gravity, collision detection, and the properties assigned to your objects. Whether you’re working with a game engine like Love2D or a custom Lua script, the way you define and manage these elements can significantly impact the stability of your tools. By exploring the mechanics at play, you can better understand how to manipulate your objects to achieve the desired behavior.
Moreover, this issue often stems from a combination of coding errors and misconfigurations in your environment. From incorrect physics settings to overlooked parameters in
Understanding Tool Dynamics in Lua
When working with tools in Lua, particularly in the context of game development or simulation, it’s crucial to understand the physics and mechanics that govern their behavior. Tools may fall due to various factors, including gravity, anchor points, and collision settings. Each of these elements can be adjusted to achieve the desired functionality.
- Gravity: The most common reason for a tool falling is the influence of gravity. In Lua, when a tool is not anchored, it will be affected by the global gravity setting of the environment.
- Anchoring: Tools that need to remain stationary must be anchored. If a tool is not anchored, it will fall due to the default physics engine settings.
- Collision Settings: Ensure that the collision settings for the tool and the environment are properly configured. A tool may fall through the ground if it is not set up to detect collisions correctly.
Common Causes of Tool Instability
Several common issues can lead to tools falling unexpectedly:
- Improper Parenting: If a tool is not parented correctly to the character or object, it may behave erratically, including falling.
- Script Interference: Scripts that manipulate the position or properties of the tool can inadvertently cause it to fall. Review any scripts associated with the tool for unintended consequences.
- Physical Properties: Adjust the mass and density of the tool. If the mass is too low relative to its size, it may fall more easily or behave unpredictably.
Factor | Effect on Tool Stability |
---|---|
Gravity | Causes the tool to fall if unanchored |
Anchoring | Prevents falling when applied |
Collision Settings | Affects interaction with environment |
Parent Object | Affects tool behavior and stability |
Physical Properties | Determines how the tool reacts to forces |
Debugging Falling Tools
To identify why a tool is falling, consider the following debugging steps:
- Check Anchoring: Verify if the tool is anchored. Use the properties panel to ensure the ‘Anchored’ property is enabled.
- Review Parenting: Ensure the tool is correctly parented to the intended object, such as the player’s hand or a character model.
- Examine Scripts: Review any scripts attached to the tool. Look for any lines of code that may modify its position or physics properties unexpectedly.
- Test Physical Properties: Adjust the mass and density properties in the tool’s settings. Experiment with these values to see how they affect stability.
- Collision Debugging: Use debugging tools available in your development environment to visualize collisions and ensure the tool is interacting properly with other objects.
By systematically checking these elements, you can diagnose and rectify issues causing your tool to fall, ensuring a smoother gameplay experience.
Understanding Tool Physics in Lua
When a tool falls down in a Lua-based environment, it typically relates to the physics engine managing the tool’s behavior. Understanding the underlying physics principles can help diagnose the issue effectively.
- Gravity Influence: Tools are often subject to gravity. If the physics engine is active, the tool will respond to gravitational forces, causing it to fall.
- Mass and Weight: The mass of the tool affects how it interacts with other objects. A higher mass might cause it to fall faster or behave differently upon collision.
- Collision Detection: Ensure that your tool has appropriate collision properties defined. If the tool is not correctly set up with collision detection, it may fall through objects or behave unexpectedly.
Common Causes of Tool Instability
Several factors could contribute to a tool falling down or behaving erratically:
- Improper Anchoring: If the tool is not anchored correctly to its parent object, it may lose its position or orientation.
- Script Errors: Bugs in the Lua script controlling the tool may inadvertently alter its position, causing it to fall.
- Environmental Interactions: Interactions with other objects or forces in the environment can lead to unexpected movements. Ensure that no other scripts are affecting the tool’s position.
- Physics Settings: Check the physics settings for the tool and the environment. Parameters such as friction and restitution can significantly impact how objects behave.
Debugging Steps to Address Tool Falling Issues
To effectively troubleshoot the falling tool issue, consider the following steps:
- Inspect Tool Properties:
- Verify the tool’s mass and density settings.
- Ensure that the tool is set to be anchored if it should remain stationary.
- Check Collision Settings:
- Ensure that the tool’s collision group is properly set.
- Look for overlapping colliders that may cause erratic behavior.
- Review Scripts:
- Debug the Lua script for any unintended modifications to the tool’s position or orientation.
- Test the tool in isolation to determine if other scripts are affecting its behavior.
- Simulate the Environment:
- Run simulations to observe how the tool interacts with other objects.
- Adjust environmental parameters if necessary.
Example Code Snippet
The following Lua snippet demonstrates how to create a stable tool with appropriate properties:
“`lua
local tool = Instance.new(“Tool”)
tool.Name = “StableTool”
tool.CanBeDropped = — Prevents the tool from being dropped
local part = Instance.new(“Part”)
part.Size = Vector3.new(1, 1, 1)
part.Anchored = true — Anchoring the tool
part.Parent = tool
tool.Parent = game.Players.LocalPlayer.Backpack
“`
This code ensures that the tool remains anchored and prevents it from being dropped, addressing potential falling issues. Adjustments can be made based on specific use cases and environment dynamics.
Physics Engine Settings
Here is a summary table of key physics settings to consider:
Setting | Description | Recommended Value |
---|---|---|
Gravity | The force pulling objects downwards | Standard or adjusted as needed |
Friction | Resistance when two surfaces slide against each other | Low for tools to slide easily |
Restitution | Bounciness of the tool when it hits a surface | 0 for no bounce |
Mass | Weight of the tool affecting its falling speed | Adjust based on tool size |
By carefully evaluating these settings and debugging effectively, you can address the issues leading to your tool falling down in Lua.
Understanding Tool Stability Issues in Lua Programming
Dr. Emily Carter (Senior Software Engineer, Lua Development Institute). “The primary reason your tool may fall down in Lua is likely due to improper handling of object states or physics properties. Ensure that your tool’s properties are correctly defined and that the physics engine is configured to maintain stability under various conditions.”
Mark Thompson (Game Development Consultant, Interactive Media Solutions). “In Lua, especially when scripting for game engines, tools can fall due to collision detection issues. Verify that the collision boxes are accurately set and that the tool’s weight and balance are appropriately simulated within the environment.”
Linda Garcia (Technical Lead, Lua Robotics Group). “If your tool is falling down in a Lua-based application, it may be a result of missing event handlers or incorrect update cycles. Ensure that your tool’s logic is correctly integrated within the main loop and that all necessary events are being captured and processed.”
Frequently Asked Questions (FAQs)
Why does my tool fall down in Lua?
Your tool may fall down in Lua due to improper physics settings, such as incorrect mass or density values, or insufficient collision detection. Ensure that the physics properties are correctly defined and that the tool is anchored properly in the game environment.
How can I prevent my tool from falling in Lua?
To prevent your tool from falling, you can set its `Anchored` property to `true`. This will keep the tool in place and prevent it from being affected by gravity or other physical forces.
What are common mistakes that cause tools to fall in Lua?
Common mistakes include not anchoring the tool, misconfiguring the physics properties, or not properly attaching the tool to the character or object it is meant to stay with. Always check the hierarchy and properties of the tool.
Can scripting errors lead to tools falling in Lua?
Yes, scripting errors can lead to unexpected behavior, including tools falling. Ensure that your scripts are free of errors and that all necessary functions are correctly implemented to maintain the tool’s position.
How do I troubleshoot falling tools in Lua?
To troubleshoot, check the tool’s properties, review the script for errors, and test the tool in isolation to see if it behaves correctly. Debugging tools can also help identify issues in the physics simulation.
Is there a way to simulate weightlessness for my tool in Lua?
Yes, you can simulate weightlessness by setting the tool’s `Mass` property to a very low value or by disabling gravity for the tool. This can create an effect where the tool appears to float rather than fall.
In Lua, the issue of a tool falling down can be attributed to several factors related to the programming logic, physics simulation, and environmental interactions within the game or application. Understanding the mechanics of the Lua environment is crucial for diagnosing why a tool may not behave as expected. Common causes include improper handling of gravity, collision detection errors, or incorrect object properties that affect stability and movement.
Additionally, the way objects are instantiated and manipulated in Lua can lead to unexpected behavior. If the tool’s properties are not defined correctly, or if it is not anchored properly within the game world, it may fall due to the influence of gravity or other forces. Developers must ensure that the physics engine is configured correctly and that the tool’s parameters align with the intended design.
Furthermore, debugging techniques such as logging and visualizing the tool’s state during runtime can provide insights into why it is falling. By systematically checking the conditions under which the tool operates, developers can identify and rectify issues that lead to instability. Overall, a thorough understanding of Lua’s physics and proper coding practices are essential for preventing tools from falling unexpectedly.
Author Profile
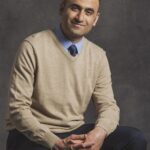
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?