Why Isn’t My Variable Declaring in Python? Common Issues and Solutions Explained
### Why Isn’t My Variable Declaring in Python?
Have you ever found yourself staring at a Python script, scratching your head in confusion as to why your variable just won’t declare? You’re not alone. Many aspiring programmers encounter this frustrating issue, often leading to hours of debugging and head-scratching. Understanding the intricacies of variable declaration in Python is crucial for anyone looking to master the language, and it’s a common stumbling block for both beginners and seasoned developers alike. In this article, we’ll dive into the potential pitfalls that can prevent your variables from declaring properly, offering insights and solutions to help you navigate these challenges with confidence.
At its core, variable declaration in Python should be straightforward, but several factors can complicate the process. From syntax errors to scope issues, there are numerous reasons why a variable might not behave as expected. Additionally, the dynamic nature of Python can lead to misunderstandings about how and when variables are created and accessed. By exploring these common scenarios, we can uncover the underlying principles that govern variable declaration and assignment in Python.
As we delve deeper into this topic, we’ll examine the most frequent mistakes that programmers make when declaring variables, as well as best practices to ensure your code runs smoothly. Whether you’re dealing with simple typos or more
Common Reasons for Variable Declaration Issues
When encountering problems with variable declaration in Python, several common issues may arise. Understanding these can help streamline your debugging process.
- Syntax Errors: Python requires specific syntax for declaring variables. Forgetting to use the assignment operator (`=`) or misplacing colons can lead to errors.
- Variable Scope: Variables declared within a function are local to that function. Attempting to access them outside their scope will result in a `NameError`.
- Uninitialized Variables: If a variable is referenced before it has been assigned a value, Python will raise an error. Always ensure that a variable is initialized before use.
Scope and Lifetime of Variables
The concept of scope is crucial in understanding variable declaration in Python. Variables can be declared in different scopes, which determines their accessibility.
Scope Type | Description | Example |
---|---|---|
Local | Defined within a function. Accessible only inside it. | `def func(): x = 10` |
Global | Defined outside all functions. Accessible throughout the module. | `x = 10; def func(): print(x)` |
Nonlocal | Refers to variables in the nearest enclosing scope that is not global. | `def outer(): x = 10; def inner(): nonlocal x` |
Understanding these scopes can prevent common pitfalls when working with variables.
Using the Correct Syntax
Proper syntax is essential for declaring variables correctly. Python’s dynamic typing means you do not need to declare a variable’s type explicitly, but you must follow the correct syntax rules.
- Declaration: Use the assignment operator to declare a variable, e.g., `my_var = 5`.
- Naming Conventions: Variable names should start with a letter or underscore and can include letters, numbers, and underscores. Avoid starting with a number or using special characters.
Debugging Variable Declaration Issues
When troubleshooting variable declaration problems, consider employing the following strategies:
- Check for Typos: Ensure that variable names are spelled consistently throughout your code.
- Print Statements: Use `print()` to display variable values at various points in your code to track changes and identify where things may be going wrong.
- Use an IDE: Integrated Development Environments (IDEs) often provide syntax highlighting and error detection, which can help catch issues before executing the code.
By systematically addressing these areas, you can often pinpoint the source of variable declaration issues effectively.
Common Reasons for Variable Declaration Issues
When encountering issues with variable declaration in Python, several common pitfalls may be responsible. Understanding these can help in diagnosing the problem effectively.
- Syntax Errors: A misspelling or incorrect punctuation can prevent a variable from being declared.
- Indentation Problems: Python relies on indentation to define code blocks. Improper indentation can lead to confusion about variable scope.
- Scope Issues: Variables declared within a function are not accessible outside of that function unless explicitly returned or declared as global.
- Overwriting Built-in Names: Using names that are identical to Python’s built-in functions or types (e.g., `list`, `str`) can lead to unexpected behavior.
Example Scenarios
Scenario | Problem Description | Solution |
---|---|---|
Misspelling a variable name | `varible = 10` instead of `variable = 10` | Correct the spelling. |
Indentation error | Variables declared inside an indented block are ignored | Ensure proper indentation. |
Variable scope | Declaring `x` in a function and trying to access it outside | Use `global x` or return the variable. |
Overriding built-in names | `list = [1, 2, 3]` makes `list()` unusable | Rename the variable to avoid conflicts. |
Debugging Techniques
To troubleshoot variable declaration issues effectively, consider the following techniques:
- Print Statements: Use `print()` to display variable values and track their scope.
- Type Checking: Use `type()` to verify the variable type, ensuring it matches your expectations.
- Python’s Built-in Functions: Functions like `dir()` can show available variables, helping identify scope problems.
Best Practices for Variable Declaration
To minimize issues with variable declaration, adhere to these best practices:
- Use Descriptive Names: Choose meaningful variable names that convey the purpose of the variable.
- Follow Naming Conventions: Stick to conventions such as snake_case for variables to improve readability.
- Avoid Shadowing Built-ins: Refrain from using names that clash with Python’s built-in functions and types.
- Limit Scope: Declare variables in the narrowest scope necessary to avoid unintended access.
Variable Declaration Issues
By understanding the common issues that can arise with variable declarations in Python and employing effective debugging techniques, developers can ensure their code functions as intended. Adopting best practices further strengthens code reliability and maintainability.
Understanding Variable Declaration Issues in Python
Dr. Emily Carter (Senior Software Engineer, Code Solutions Inc.). Variables in Python may not declare as expected due to scope issues. If a variable is defined within a function, it cannot be accessed outside that function unless explicitly returned or declared as global. This is a common oversight among new Python developers.
Michael Chen (Python Instructor, Tech Academy). Another frequent reason for variable declaration failure is syntax errors. A missing equal sign or incorrect indentation can cause the interpreter to throw an error, leading to confusion about whether the variable was declared at all.
Lisa Patel (Lead Developer, NextGen Software). Additionally, it’s important to remember that Python is dynamically typed. If a variable is assigned a value of a different type later in the code, it can lead to unexpected behavior. Ensuring consistent variable usage is crucial for successful declaration and assignment.
Frequently Asked Questions (FAQs)
Why isn’t my variable declaring in Python?
Variable declaration issues in Python often arise from syntax errors, improper indentation, or using reserved keywords. Ensure that you are using the correct assignment operator (`=`) and that the variable name adheres to Python’s naming conventions.
What are common mistakes that prevent variable declaration?
Common mistakes include forgetting to assign a value to the variable, using invalid characters in the variable name, or attempting to declare a variable inside a function without the proper scope. Ensure that your variable names start with a letter or underscore and do not contain spaces.
Can I declare a variable without assigning a value?
In Python, you cannot declare a variable without assigning a value to it. Unlike some other programming languages, Python requires that a variable be initialized before it can be used.
How does indentation affect variable declaration?
Indentation in Python defines the scope of variables. If a variable is declared within an indented block (like a function or loop), it will not be accessible outside that block. Ensure that your variable is declared in the appropriate scope.
What should I do if my variable seems to be declared but still causes an error?
If a variable appears to be declared but causes errors, check for typos, ensure that it is not being used before assignment, and verify that it is not shadowed by another variable with the same name in a different scope.
Are there any tools to help diagnose variable declaration issues?
Yes, using an Integrated Development Environment (IDE) or code editor with syntax highlighting and linting features can help identify variable declaration issues. Tools like PyLint or Flake8 can provide insights and suggestions for correcting errors in your code.
In Python, variable declaration issues can arise from various factors, including syntax errors, scope limitations, and naming conflicts. Understanding how Python handles variable declaration is crucial for troubleshooting. Unlike some programming languages, Python does not require explicit variable declaration; instead, variables are created when they are first assigned a value. However, if a variable is referenced before it has been assigned, a NameError will occur, indicating that the variable is not defined.
Another common issue is related to variable scope. Variables defined within a function are local to that function and cannot be accessed outside of it. This can lead to confusion if a variable is expected to be available in a broader context. Additionally, naming conflicts can arise when a variable name shadows a built-in function or another variable, leading to unexpected behavior. It is essential to use unique and descriptive variable names to avoid such conflicts.
To effectively address variable declaration issues in Python, developers should carefully review their code for syntax errors and ensure that variables are defined before they are used. Utilizing tools such as linters can help identify potential problems early in the development process. Moreover, understanding the scope of variables and adhering to best practices in naming conventions can significantly reduce the likelihood of encountering these issues.
Author Profile
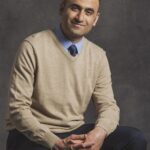
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?