How Can You Programmatically Add Custom Attributes to WooCommerce Products?
In the ever-evolving world of e-commerce, customization is key to standing out from the competition. For WooCommerce users, the ability to add custom attributes to products programmatically can unlock a plethora of possibilities, enhancing the shopping experience and tailoring products to meet specific customer needs. Whether you’re a developer looking to streamline product management or a store owner eager to enrich your product offerings, understanding how to implement custom attributes can significantly elevate your WooCommerce store’s functionality.
Adding custom attributes programmatically allows for greater flexibility and control over your product data. This capability is particularly beneficial for businesses that have unique requirements or want to implement complex product variations. By leveraging WooCommerce’s built-in hooks and functions, you can automate the process of assigning attributes to products, ensuring consistency and saving valuable time. This approach not only simplifies inventory management but also enhances the customer experience by providing detailed product information.
As we delve deeper into the intricacies of adding custom attributes, we’ll explore the essential steps, best practices, and potential pitfalls to avoid. Whether you’re looking to enhance product descriptions, filter options, or improve searchability, mastering this technique will empower you to create a more dynamic and user-friendly online store. Get ready to transform your WooCommerce experience and take your product offerings to the next level
Understanding Custom Attributes in WooCommerce
In WooCommerce, custom attributes allow you to add extra product information that can help enhance product details and improve user experience. These attributes can represent specific characteristics of products, such as size, color, or any other relevant feature. Adding these attributes programmatically can save time, especially when dealing with multiple products.
Adding Custom Attributes Programmatically
To add custom attributes to a product programmatically in WooCommerce, you can use the `update_post_meta` function. This function allows you to update the metadata of a product post. Below is a step-by-step guide to achieve this.
Code Example
The following code snippet demonstrates how to add a custom attribute to a product:
“`php
// Function to add custom attributes
function add_custom_product_attribute($product_id) {
// Check if the product ID is valid
if (!$product_id) return;
// Define your custom attribute
$custom_attributes = array(
‘custom_attribute_name’ => array(
‘name’ => ‘Custom Attribute’,
‘value’ => ‘Attribute Value’,
‘position’ => 1,
‘is_visible’ => 1,
‘is_variation’ => 0,
‘is_taxonomy’ => 0
)
);
// Set the custom attributes
wp_set_object_terms($product_id, ‘custom_attribute_name’, ‘product_attribute’);
update_post_meta($product_id, ‘_product_attributes’, $custom_attributes);
}
// Example usage
add_custom_product_attribute(123); // Replace 123 with your actual product ID
“`
This code does the following:
- Checks if the provided product ID is valid.
- Defines the custom attribute with its properties, including name, value, visibility, and whether it’s a variation or taxonomy.
- Uses `wp_set_object_terms` to set the attribute terms, and `update_post_meta` to store the attribute metadata.
Attributes Properties Explained
When defining custom attributes, it’s essential to understand the properties you can set:
Property | Description |
---|---|
name | The name of the custom attribute as it will appear on the product page. |
value | The value of the custom attribute, which can be a single value or a list. |
position | The display order of the attribute on the product page. |
is_visible | Determines whether the attribute is visible on the product page (1 for yes, 0 for no). |
is_variation | Indicates if the attribute can be used for variations (1 for yes, 0 for no). |
is_taxonomy | Specifies if the attribute is a taxonomy (1 for yes, 0 for no). |
Best Practices
When adding custom attributes programmatically, consider the following best practices:
- Use Unique Names: Ensure attribute names are unique to prevent conflicts with existing attributes.
- Test Your Code: Always test your code on a staging environment before deploying it to a live site.
- Maintain Readability: Keep your code organized and commented for easier maintenance.
- Batch Processing: If you are adding attributes to multiple products, consider creating a loop to handle batch processing efficiently.
By following these guidelines, you can effectively manage product attributes in WooCommerce, enhancing your store’s functionality and user experience.
Programmatically Adding Custom Attributes to WooCommerce Products
To add custom attributes to a WooCommerce product programmatically, you can utilize the WooCommerce functions available in the WordPress framework. This process generally involves creating or updating an attribute and then assigning it to a product. Below is a detailed guide on how to achieve this.
Creating a Custom Attribute
Before assigning an attribute to a product, it must first be created. The following code snippet demonstrates how to create a custom attribute:
“`php
function create_custom_product_attribute() {
// Define the attribute name and settings
$attribute_name = ‘Custom Attribute’;
$attribute_slug = sanitize_title($attribute_name);
// Check if the attribute already exists
if (!taxonomy_exists(‘pa_’ . $attribute_slug)) {
// Create the attribute
$args = array(
‘slug’ => $attribute_slug,
‘name’ => $attribute_name,
‘type’ => ‘select’,
‘order_by’ => ‘menu_order’,
‘has_archives’ => ,
);
// Add the attribute
wc_create_attribute($args);
}
}
add_action(‘init’, ‘create_custom_product_attribute’);
“`
This code checks if the attribute already exists and creates it if not, ensuring no duplicates are created.
Assigning the Custom Attribute to a Product
Once the custom attribute has been created, it can be assigned to a specific product. The following code shows how to add the attribute to a product programmatically:
“`php
function add_custom_attribute_to_product($product_id) {
// Define the attribute values
$attribute_slug = ‘custom-attribute’;
$attribute_value = ‘Value 1’;
// Prepare the attribute array
$attribute = array(
‘name’ => ‘Custom Attribute’,
‘value’ => $attribute_value,
‘position’ => 0,
‘is_visible’ => 1,
‘is_variation’ => 0,
‘is_filter’ => 0,
);
// Get the existing attributes
$product = wc_get_product($product_id);
$attributes = $product->get_attributes();
// Add the custom attribute to the attributes array
$attributes[$attribute_slug] = $attribute;
// Set the new attributes back to the product
$product->set_attributes($attributes);
$product->save();
}
“`
This function takes a product ID and assigns the custom attribute to the specified product.
Using Hooks for Dynamic Product Updates
To dynamically add attributes when products are created or updated, you can utilize WooCommerce hooks. Here’s how you can incorporate the attribute addition into the product creation process:
“`php
function add_attribute_on_product_creation($product_id) {
add_custom_attribute_to_product($product_id);
}
add_action(‘woocommerce_new_product’, ‘add_attribute_on_product_creation’);
“`
This hook ensures that each time a new product is created, the custom attribute is added automatically.
Best Practices
When adding custom attributes programmatically, consider the following best practices:
- Sanitize Input: Always sanitize attribute names and values to prevent security vulnerabilities.
- Check for Duplicates: Before creating attributes, ensure they do not already exist to avoid conflicts.
- Version Control: Keep track of changes to your code using version control systems like Git.
- Testing: Test your code in a staging environment before deploying to a live site.
By following the above steps, you can effectively add custom attributes to WooCommerce products programmatically. This process enhances your product management capabilities and allows for a more tailored shopping experience.
Expert Insights on Programmatically Adding Custom Attributes in WooCommerce
Jessica Lane (Senior WooCommerce Developer, E-Commerce Innovations). “Adding custom attributes programmatically in WooCommerce can significantly enhance product management. Utilizing hooks such as ‘woocommerce_product_options_attributes’ allows developers to integrate additional attributes seamlessly, improving both the user experience and backend organization.”
Mark Thompson (E-Commerce Consultant, Digital Commerce Solutions). “For developers looking to add custom attributes to products in WooCommerce, it is essential to understand the product data structure. Implementing the ‘update_post_meta’ function effectively allows for dynamic attribute assignment, which can be tailored to specific product types or categories, ensuring flexibility in product offerings.”
Linda Garcia (WooCommerce Specialist, Online Store Experts). “When programmatically adding custom attributes, one must consider the implications on product filtering and search functionality. Leveraging the ‘woocommerce_attribute_label’ filter can enhance how these attributes are displayed to customers, ultimately improving the shopping experience and conversion rates.”
Frequently Asked Questions (FAQs)
How can I add a custom attribute to a WooCommerce product programmatically?
You can add a custom attribute to a WooCommerce product programmatically by using the `update_post_meta` function in your theme’s `functions.php` file or a custom plugin. Use the product ID, attribute name, and attribute value to set the meta data.
What code snippet is required to add a custom attribute to a product?
You can use the following code snippet:
“`php
function add_custom_attribute($product_id) {
$attribute = array(
‘name’ => ‘Custom Attribute’,
‘value’ => ‘Custom Value’,
‘position’ => 0,
‘is_visible’ => 1,
‘is_variation’ => 0,
‘is_taxonomy’ => 0
);
update_post_meta($product_id, ‘_product_attributes’, $attribute);
}
add_action(‘woocommerce_product_after_variable_attributes’, ‘add_custom_attribute’, 10, 1);
“`
Can I add multiple custom attributes to a WooCommerce product?
Yes, you can add multiple custom attributes by creating an array of attributes and looping through them to update the product meta data. Each attribute should be defined with its own properties.
Will adding custom attributes affect the product’s SEO?
Adding custom attributes can enhance SEO by providing additional context about the product. Ensure that the attributes are relevant and include keywords that potential customers may search for.
How can I display custom attributes on the product page?
To display custom attributes on the product page, you can modify the WooCommerce template files or use hooks such as `woocommerce_single_product_summary` to output the custom attributes in the desired location.
Is it possible to remove a custom attribute from a WooCommerce product programmatically?
Yes, you can remove a custom attribute by using the `delete_post_meta` function. Specify the product ID and the meta key associated with the custom attribute to remove it from the product.
adding custom attributes to WooCommerce products programmatically is a valuable technique for enhancing product data management and improving user experience. By utilizing WooCommerce’s built-in functions and hooks, developers can seamlessly integrate custom attributes into their products without the need for manual input through the admin interface. This process not only saves time but also ensures consistency across product listings.
Key takeaways from the discussion include the importance of understanding the WooCommerce data structure and the specific functions available for product manipulation. Utilizing functions such as `update_post_meta()` and `wc_add_attribute()` allows for precise control over product attributes. Additionally, leveraging action hooks can automate the process, making it easier to apply custom attributes in bulk or under specific conditions.
Overall, programmatically adding custom attributes to WooCommerce products is a powerful tool for developers looking to enhance their eCommerce sites. By following best practices and utilizing the appropriate WooCommerce functions, developers can create a more dynamic and tailored shopping experience for customers, ultimately leading to increased sales and customer satisfaction.
Author Profile
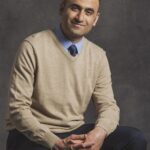
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?