How Can I Check if Another Plugin is Active in WordPress?
In the vibrant world of WordPress development, plugins serve as the lifeblood of functionality and customization. With thousands of options available, each plugin brings its own unique features and capabilities to the table. However, as developers and site owners dive into the realm of plugin integration, a common challenge arises: how can one determine if another plugin is active? This seemingly simple question is crucial for ensuring compatibility, avoiding conflicts, and optimizing performance. In this article, we’ll explore the methods and best practices for checking the status of plugins within your WordPress environment, empowering you to create seamless and efficient websites.
Understanding whether a plugin is active can save developers from potential headaches down the line. For instance, if you’re building a new feature that relies on another plugin’s functionality, knowing its status can help you avoid errors and improve user experience. Additionally, this knowledge allows for conditional loading of scripts and styles, ensuring that your site remains lightweight and fast. As we delve deeper into the topic, we will uncover various techniques to check for active plugins, including built-in WordPress functions and custom code snippets.
Moreover, the implications of checking plugin status extend beyond mere functionality. It plays a significant role in maintaining site security and stability. Conflicts between plugins can lead to unexpected behavior or crashes,
Checking if Another Plugin is Active in WordPress
To determine if another plugin is active in your WordPress environment, you can utilize the built-in function `is_plugin_active()`. This function is part of the WordPress core and can be used within your own plugin or theme’s code to check the status of another plugin.
Using `is_plugin_active()` Function
The `is_plugin_active()` function checks if a specified plugin is currently active. It requires the plugin’s path relative to the plugins directory. Here’s the syntax for using this function:
“`php
is_plugin_active( ‘plugin-folder/plugin-file.php’ );
“`
To effectively use this function, ensure you include the necessary file in your code:
“`php
include_once( ABSPATH . ‘wp-admin/includes/plugin.php’ );
“`
Once you have included the file, you can check for any plugin’s activation status. For example, if you want to check if the WooCommerce plugin is active, you would do the following:
“`php
if ( is_plugin_active( ‘woocommerce/woocommerce.php’ ) ) {
// WooCommerce is active
}
“`
Checking for Multiple Plugins
In some cases, you may need to check for multiple plugins. You can easily create an array of plugin paths and loop through them to verify their activation status.
“`php
$plugins_to_check = array(
‘woocommerce/woocommerce.php’,
‘yoast-seo/wp-seo.php’,
);
foreach ( $plugins_to_check as $plugin ) {
if ( is_plugin_active( $plugin ) ) {
// The plugin is active
}
}
“`
Alternative Method: Using `get_option()`
Another method involves checking the options table in the database for specific plugin activation states. This is less common but can be useful in certain scenarios where you want to check for custom settings related to plugins.
“`php
$active_plugins = get_option( ‘active_plugins’ );
if ( in_array( ‘woocommerce/woocommerce.php’, $active_plugins ) ) {
// WooCommerce is active
}
“`
Common Use Cases
Checking for the activation of other plugins can be essential for:
- Compatibility Checks: Ensuring that your plugin operates correctly with others.
- Feature Dependencies: Activating certain features only if required plugins are present.
- Error Handling: Providing user notifications when essential plugins are not active.
Best Practices
- Always include the plugin check within function hooks like `plugins_loaded` to ensure that all plugins are fully loaded before running your checks.
- Avoid hardcoding plugin paths; instead, consider using constants or options to manage them dynamically.
- Use `is_plugin_active_for_network()` for multisite installations to check if a plugin is active across the network.
Example Table of Common Plugins
Plugin Name | Plugin Path | Active Status Check |
---|---|---|
WooCommerce | woocommerce/woocommerce.php | is_plugin_active( ‘woocommerce/woocommerce.php’ ) |
Yoast SEO | yoast-seo/wp-seo.php | is_plugin_active( ‘yoast-seo/wp-seo.php’ ) |
Contact Form 7 | contact-form-7/wp-contact-form-7.php | is_plugin_active( ‘contact-form-7/wp-contact-form-7.php’ ) |
Checking for Active Plugins in WordPress
To determine if another plugin is active in WordPress, you can utilize the built-in functions provided by WordPress. The primary function to check for active plugins is `is_plugin_active()`, which is part of the `plugin.php` file.
Using `is_plugin_active()` Function
This function allows you to check if a specific plugin is active by passing the plugin’s main file path. Here’s how to use it:
- Include the Plugin File: First, make sure you include the `plugin.php` file in your custom code or theme functions:
“`php
include_once( ABSPATH . ‘wp-admin/includes/plugin.php’ );
“`
- Check Plugin Status: Use the `is_plugin_active()` function to check if the desired plugin is active:
“`php
if ( is_plugin_active( ‘plugin-directory/plugin-file.php’ ) ) {
// The plugin is active.
}
“`
Replace `’plugin-directory/plugin-file.php’` with the actual path of the plugin you want to check.
Example Code Snippet
Here is a complete example that checks if the WooCommerce plugin is active:
“`php
include_once( ABSPATH . ‘wp-admin/includes/plugin.php’ );
if ( is_plugin_active( ‘woocommerce/woocommerce.php’ ) ) {
echo ‘WooCommerce is active!’;
} else {
echo ‘WooCommerce is not active.’;
}
“`
Checking Multiple Plugins
You may want to check the status of multiple plugins at once. This can be achieved using a simple array and a loop:
“`php
$plugins = array(
‘woocommerce/woocommerce.php’,
‘contact-form-7/wp-contact-form-7.php’,
‘yoast-seo/wp-seo.php’
);
foreach ( $plugins as $plugin ) {
if ( is_plugin_active( $plugin ) ) {
echo “$plugin is active.
“;
} else {
echo “$plugin is not active.
“;
}
}
“`
Using `get_option()` for Site-Wide Checks
Another way to check if a plugin is active is by using the `get_option()` function to see if its settings exist. This method is less reliable than `is_plugin_active()`, as it depends on the specific plugin’s implementation.
“`php
if ( get_option( ‘woocommerce_enable’ ) !== ) {
echo ‘WooCommerce is likely active.’;
}
“`
Considerations for Custom Themes and Plugins
When developing custom themes or plugins, consider the following best practices:
- Dependency Management: Clearly specify dependencies in your plugin’s documentation.
- Fail Gracefully: If a required plugin is not active, provide users with guidance on how to activate it.
- Performance: Avoid excessive checks for active plugins, as this can lead to performance issues.
Using `is_plugin_active()` is the most straightforward and reliable method to check if another plugin is active in WordPress. Incorporating this functionality into your code ensures compatibility and better user experience within your themes or plugins.
Evaluating Plugin Interactions in WordPress
Jessica Lin (WordPress Developer, WP Innovations). “To check if another plugin is active in WordPress, developers can utilize the `is_plugin_active()` function. This function allows for straightforward verification of a plugin’s status, ensuring compatibility and preventing conflicts during development.”
Mark Thompson (Senior Software Engineer, Plugin Solutions Inc.). “It’s crucial to incorporate checks for active plugins within your code to avoid unexpected behavior. Using `function_exists()` in conjunction with `is_plugin_active()` can provide a robust way to ensure that dependencies are met before executing specific functionalities.”
Linda Garcia (Technical Writer, WordPress Expert Blog). “Understanding how to check for active plugins is essential for maintaining a healthy WordPress environment. By leveraging the `get_option()` function alongside plugin checks, developers can create dynamic features that adapt based on the plugins currently active on a site.”
Frequently Asked Questions (FAQs)
How can I check if a specific plugin is active in WordPress?
You can check if a specific plugin is active by using the `is_plugin_active()` function within your theme or plugin code. This function requires the plugin’s main file path relative to the `plugins` directory.
What is the correct way to use the `is_plugin_active()` function?
To use `is_plugin_active()`, include the following code: `include_once( ABSPATH . ‘wp-admin/includes/plugin.php’ );` followed by `if ( is_plugin_active( ‘plugin-directory/plugin-file.php’ ) ) { … }`. Replace `plugin-directory/plugin-file.php` with the actual path of the plugin.
Can I check for multiple plugins at once?
Yes, you can check for multiple plugins by using multiple `is_plugin_active()` calls within a single conditional statement or by creating an array of plugins and iterating through it.
What should I do if `is_plugin_active()` returns ?
If `is_plugin_active()` returns , it indicates that the specified plugin is either not installed or not activated. Ensure the plugin is correctly installed and activated in the WordPress admin panel.
Is there a way to check for active plugins without coding?
Yes, you can check for active plugins through the WordPress admin dashboard. Navigate to the “Plugins” section, where you will see a list of all installed plugins along with their activation status.
What if I want to check for a plugin’s activation status in a shortcode?
You can use the `is_plugin_active()` function within a shortcode by defining the shortcode function and including the necessary code to check the plugin’s status. Ensure to add the shortcode using `add_shortcode()` in your theme or plugin.
In WordPress development, checking if another plugin is active is a crucial task for ensuring compatibility and functionality within a site. Developers often need to verify the presence of specific plugins to either extend their features or prevent conflicts. This can be accomplished using the `is_plugin_active()` function, which is part of the WordPress core. By utilizing this function, developers can conditionally execute code based on whether a particular plugin is activated, thus enhancing the overall user experience.
Moreover, it is essential to ensure that the necessary checks are performed before calling functions or features that depend on another plugin. This practice not only prevents errors but also contributes to a more robust and reliable WordPress environment. Developers should also consider using the `function_exists()` function as an additional measure to verify that specific functions from the other plugin are available, further safeguarding against potential issues.
In summary, checking for active plugins in WordPress is a fundamental practice that aids in maintaining compatibility and functionality across different components of a website. By leveraging built-in functions like `is_plugin_active()` and `function_exists()`, developers can create more resilient and user-friendly WordPress applications. This approach not only enhances the site’s performance but also fosters a smoother integration of various plugins, ultimately leading to
Author Profile
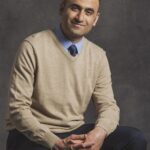
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?