How Can You Retrieve the Parent Post of a WordPress Attachment by ID?
When working with WordPress, managing media attachments effectively can significantly enhance your site’s functionality and user experience. One common scenario that developers and site owners encounter is the need to retrieve the parent post of an attachment using its ID. Understanding how to navigate this relationship between attachments and their parent posts is crucial, especially when building custom themes or plugins that require dynamic content handling. In this article, we will explore the methods and best practices for fetching the parent post associated with an attachment, empowering you to leverage WordPress’s robust media management capabilities.
In WordPress, every media file uploaded to the media library is treated as an attachment post type, which means it has its own unique ID. However, these attachments are often linked to a parent post, such as a page or a post where the media is utilized. Knowing how to retrieve this parent post by using the attachment ID can streamline your content management process, enabling you to display related information or create more cohesive layouts. This relationship not only enhances the organization of your media but also allows for more dynamic content presentation.
Throughout this article, we will delve into the various methods available for fetching the parent post of an attachment by its ID. We’ll discuss built-in WordPress functions, code snippets, and practical examples to illustrate how you can implement this functionality
Retrieving the Parent Post of an Attachment
To obtain the parent post of an attachment in WordPress, you can use the built-in function `get_post()`. This function allows you to fetch a post object by its ID, which can be particularly useful for attachments that are linked to specific posts. Here’s how you can achieve this:
- **Get Attachment ID**: First, you need the ID of the attachment you are interested in.
- **Fetch the Attachment Post**: Use the `get_post()` function to retrieve the attachment.
- **Access Parent ID**: The attachment object contains a `post_parent` property that holds the ID of the parent post.
Here is a code snippet that illustrates this process:
“`php
$attachment_id = 123; // Replace with your attachment ID
$attachment = get_post($attachment_id);
if ($attachment) {
$parent_id = $attachment->post_parent;
$parent_post = get_post($parent_id);
if ($parent_post) {
echo ‘Parent Post Title: ‘ . $parent_post->post_title;
} else {
echo ‘No parent post found.’;
}
} else {
echo ‘No attachment found.’;
}
“`
This code will display the title of the parent post associated with the specified attachment.
Understanding Attachment Metadata
When dealing with attachments in WordPress, it’s essential to recognize the metadata associated with each attachment. The `wp_get_attachment_metadata()` function can be used to retrieve metadata such as file type, dimensions, and more.
Here’s a table summarizing key metadata properties for attachments:
Property | Description |
---|---|
file | Path to the file relative to the uploads directory. |
width | Width of the image in pixels. |
height | Height of the image in pixels. |
image_meta | Array containing additional image metadata such as camera settings. |
By examining this metadata, developers can gain insights into how attachments are utilized within their WordPress site and make decisions accordingly.
Using Custom Queries to Retrieve Parent Posts
In more complex scenarios, you may want to perform custom queries to retrieve parent posts based on specific criteria. The `WP_Query` class can be leveraged for this purpose. Below is an example of how to query for parent posts of a specific attachment type:
“`php
$args = array(
‘post_type’ => ‘attachment’,
‘post__in’ => array(123), // Replace with your attachment ID
‘posts_per_page’ => 1,
‘post_status’ => ‘any’
);
$query = new WP_Query($args);
if ($query->have_posts()) {
while ($query->have_posts()) {
$query->the_post();
$parent_id = get_post_field(‘post_parent’, get_the_ID());
$parent_post = get_post($parent_id);
if ($parent_post) {
echo ‘Parent Post Title: ‘ . $parent_post->post_title;
}
}
wp_reset_postdata();
} else {
echo ‘No attachments found.’;
}
“`
This approach allows for more flexibility and customization when retrieving parent posts associated with attachments, particularly when dealing with multiple attachments or specific conditions.
Retrieving the Parent Post of an Attachment in WordPress
To get the parent post of an attachment using its ID in WordPress, you can utilize the built-in WordPress functions. The `get_post()` function allows you to access the attachment’s details, including its parent post ID.
Using `get_post()` Function
The `get_post()` function retrieves the post object for a given post ID. For attachments, you can extract the parent ID directly from this object.
“`php
$attachment_id = 123; // Replace with your attachment ID
$attachment = get_post($attachment_id);
if ($attachment) {
$parent_id = $attachment->post_parent; // This gives you the parent post ID
echo ‘Parent Post ID: ‘ . $parent_id;
} else {
echo ‘No attachment found.’;
}
“`
Understanding the Attachment Structure
In WordPress, every attachment is a post of type ‘attachment’, and it has a `post_parent` attribute that points to the parent post. Here’s a breakdown of key attributes:
Attribute | Description |
---|---|
`ID` | Unique identifier for the attachment |
`post_parent` | ID of the parent post |
`post_type` | Type of the post (should be ‘attachment’) |
`post_title` | Title of the attachment |
`guid` | URL of the attachment |
Fetching Parent Post Details
Once you have the parent post ID, you can retrieve additional details about the parent post using the same `get_post()` function:
“`php
if ($parent_id) {
$parent_post = get_post($parent_id);
if ($parent_post) {
echo ‘Parent Post Title: ‘ . $parent_post->post_title;
echo ‘Parent Post URL: ‘ . get_permalink($parent_post->ID);
} else {
echo ‘No parent post found.’;
}
}
“`
Common Use Cases
Understanding how to get the parent post of an attachment can be beneficial in various scenarios:
- Display Related Content: Show a list of attachments related to their parent post.
- Custom Queries: Use the parent post information to create custom queries that filter attachments by parent.
- Integration with Plugins: Enhance functionality in plugins that rely on attachments and their parent posts.
Performance Considerations
When working with multiple attachments, consider the following to optimize performance:
- Batch Processing: Use a loop to fetch multiple attachments and their parent posts in one go.
- Caching: Implement caching mechanisms to reduce database queries if the same data is accessed frequently.
- Check Existence: Always verify the existence of posts before attempting to use their properties to avoid errors.
Utilizing these methods efficiently allows for a robust handling of attachments and their relationships to parent posts within WordPress.
Expert Insights on Retrieving Attachment Parent Posts in WordPress
Dr. Emily Carter (WordPress Developer and Author, WP Insights). “To retrieve the parent post of an attachment in WordPress using its ID, you can utilize the `get_post()` function. This function allows you to access the attachment’s metadata, including its parent post ID, which is essential for linking media to its respective content.”
Michael Thompson (Senior WordPress Engineer, CodeCraft). “When working with attachments in WordPress, it is crucial to remember that the parent post ID is stored in the `post_parent` field of the attachment’s post object. Using `wp_get_attachment_metadata()` can also provide additional context about the attachment, enhancing your ability to manage media effectively.”
Jessica Lee (WordPress Consultant and Educator, Digital Academy). “For developers looking to streamline their workflow, creating a custom function that utilizes `get_post()` to fetch the parent post by attachment ID can save time. This approach not only improves efficiency but also ensures that you are adhering to best practices in WordPress development.”
Frequently Asked Questions (FAQs)
How can I get the parent post ID of an attachment in WordPress?
You can retrieve the parent post ID of an attachment using the `get_post_field()` function with the attachment’s ID and the ‘post_parent’ field. For example: `$parent_id = get_post_field(‘post_parent’, $attachment_id);`.
What function retrieves the attachment’s parent post in WordPress?
The function `get_post()` can be used to retrieve the entire post object of the parent by passing the attachment’s parent ID. This can be done as follows: `$parent_post = get_post($parent_id);`.
Is it possible to get the parent post details from an attachment ID?
Yes, you can obtain the parent post details by first fetching the parent post ID and then using `get_post()` to get the complete post object, which includes the title, content, and other metadata.
Can I use a custom query to find the parent post of an attachment?
While it is possible to use a custom query, it is generally more efficient to use built-in WordPress functions like `get_post_field()` and `get_post()` to ensure compatibility and performance.
What if the attachment has no parent post?
If the attachment has no parent post, the `post_parent` field will return 0. You can check for this condition to handle cases where the attachment is not associated with any parent post.
Are there any performance considerations when retrieving parent posts for attachments?
Using built-in functions is generally optimized for performance. However, if you are processing a large number of attachments, consider caching results or limiting queries to improve efficiency.
In WordPress, retrieving the parent post of an attachment by its ID is a common requirement for developers and content managers. Attachments in WordPress, such as images or documents, are treated as a specific post type, and each attachment is linked to a parent post. By utilizing built-in WordPress functions, one can easily fetch the parent post associated with a given attachment ID, allowing for better content management and display.
To achieve this, the `get_post()` function can be employed, which retrieves the post object for the attachment. Once the attachment post object is obtained, the parent post ID can be accessed via the `post_parent` property. Subsequently, another call to `get_post()` with the parent post ID will yield the details of the parent post, enabling developers to access its title, content, and other relevant metadata.
Understanding how to navigate the relationship between attachments and their parent posts is crucial for effective content organization in WordPress. This knowledge not only enhances the functionality of themes and plugins but also improves the user experience by ensuring that related content is easily accessible. By mastering these techniques, developers can create more dynamic and interconnected WordPress sites.
Author Profile
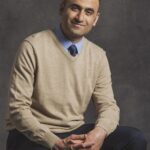
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?