How Can I Write JSON to a File?
In today’s data-driven world, the ability to efficiently store and manipulate information is paramount. As developers and data enthusiasts increasingly turn to JSON (JavaScript Object Notation) for its lightweight and human-readable format, the question arises: how do you effectively write JSON to a file? Whether you’re working on a web application, a data processing script, or simply looking to save structured data for later use, mastering the art of writing JSON to a file is an essential skill that can streamline your workflow and enhance your projects.
Writing JSON to a file is not just about saving data; it’s about ensuring that your information is organized, accessible, and easy to share. This process involves converting your data structures into a format that can be easily written to disk, allowing for seamless retrieval and manipulation later on. With various programming languages offering built-in support for JSON, the methods to accomplish this task can vary, but the underlying principles remain consistent across platforms.
As you delve deeper into this topic, you’ll discover the nuances of file handling, the importance of proper formatting, and the best practices for ensuring data integrity. Whether you’re a seasoned programmer or just starting your coding journey, understanding how to write JSON to a file will empower you to manage your data more effectively and open up new possibilities for your projects.
Understanding JSON File Structure
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. A typical JSON file is structured as a collection of key-value pairs, which can represent complex data structures.
- Objects: Enclosed in curly braces `{}`, containing key-value pairs.
- Arrays: Enclosed in square brackets `[]`, containing a list of values.
- Values: Can be strings, numbers, objects, arrays, booleans, or null.
Example of a JSON structure:
“`json
{
“name”: “John Doe”,
“age”: 30,
“isEmployed”: true,
“skills”: [“JavaScript”, “Python”, “Java”],
“address”: {
“street”: “123 Main St”,
“city”: “Anytown”
}
}
“`
Writing JSON to a File
Writing JSON data to a file involves converting the data into a JSON string format and then saving it to a specified file path. This process varies slightly depending on the programming language being used. Below are examples using Python, JavaScript (Node.js), and Java.
Python Example
In Python, the `json` module provides methods to work with JSON data easily. To write JSON to a file, follow these steps:
- Import the `json` module.
- Create a Python dictionary (or list).
- Open a file in write mode.
- Use `json.dump()` to write the data.
“`python
import json
data = {
“name”: “John Doe”,
“age”: 30,
“isEmployed”: True,
“skills”: [“JavaScript”, “Python”, “Java”],
“address”: {
“street”: “123 Main St”,
“city”: “Anytown”
}
}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
“`
JavaScript (Node.js) Example
In Node.js, you can use the built-in `fs` module along with `JSON.stringify()` to write JSON data to a file:
“`javascript
const fs = require(‘fs’);
const data = {
name: “John Doe”,
age: 30,
isEmployed: true,
skills: [“JavaScript”, “Python”, “Java”],
address: {
street: “123 Main St”,
city: “Anytown”
}
};
fs.writeFileSync(‘data.json’, JSON.stringify(data, null, 2));
“`
Java Example
In Java, libraries like `org.json` or `Gson` make it straightforward to convert objects to JSON and write them to a file. The following example uses Gson:
“`java
import com.google.gson.Gson;
import java.io.FileWriter;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Gson gson = new Gson();
Data data = new Data(“John Doe”, 30, true, new String[]{“JavaScript”, “Python”, “Java”}, new Address(“123 Main St”, “Anytown”));
try (FileWriter writer = new FileWriter(“data.json”)) {
gson.toJson(data, writer);
} catch (IOException e) {
e.printStackTrace();
}
}
}
class Data {
String name;
int age;
boolean isEmployed;
String[] skills;
Address address;
// Constructor, getters, setters omitted for brevity
}
class Address {
String street;
String city;
// Constructor, getters, setters omitted for brevity
}
“`
Common Considerations
When writing JSON data to a file, consider the following:
- File Permissions: Ensure the program has permission to write to the target directory.
- Error Handling: Implement error handling to manage exceptions, such as IO errors.
- Data Validation: Validate your data to ensure it conforms to the expected JSON format.
Language | Library/Function | Code Snippet |
---|---|---|
Python | json.dump() | json.dump(data, json_file) |
Node.js | fs.writeFileSync() | fs.writeFileSync('data.json', JSON.stringify(data, null, 2)) |
Java | Gson.toJson() | gson.toJson(data, writer) |
Writing JSON to a File in Python
To write JSON data to a file in Python, the `json` module provides a straightforward approach. This module enables serialization of Python objects into JSON format and handles file operations seamlessly. Below are the steps and code examples for writing JSON to a file.
Step-by-Step Process
- Import the JSON Module: Ensure you have imported the `json` module in your Python script.
“`python
import json
“`
- Prepare Your Data: Create the data you want to write. This data can be in the form of dictionaries, lists, or any data structure that is serializable.
“`python
data = {
“name”: “John Doe”,
“age”: 30,
“is_student”: ,
“courses”: [“Math”, “Science”],
“address”: {
“street”: “123 Main St”,
“city”: “Anytown”
}
}
“`
- Open a File for Writing: Use the `open()` function to create or open a file in write mode. This file will store the JSON data.
“`python
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
“`
- Use `json.dump()`: The `json.dump()` function writes the data to the file in JSON format. This method takes two arguments: the data to write and the file object.
Example Code
Here is a complete example that combines all the above steps:
“`python
import json
data = {
“name”: “Jane Doe”,
“age”: 28,
“is_student”: True,
“courses”: [“English”, “History”],
“address”: {
“street”: “456 Elm St”,
“city”: “Othertown”
}
}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file, indent=4) Using indent for pretty printing
“`
Additional Options
When using `json.dump()`, there are several optional parameters that can enhance the output:
- indent: Specify the number of spaces to use for indentation, which makes the JSON file more readable.
- sort_keys: If set to `True`, the output will have its keys sorted.
Example with additional options:
“`python
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file, indent=4, sort_keys=True)
“`
Handling Exceptions
When writing to a file, it is essential to handle potential exceptions such as file access issues. The following example demonstrates basic exception handling:
“`python
try:
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file, indent=4)
except IOError as e:
print(f”An error occurred while writing to the file: {e}”)
“`
Writing JSON to a file in Python is a simple yet powerful process that allows for easy data serialization. By following the outlined steps and utilizing the features of the `json` module, developers can efficiently manage data storage in JSON format.
Expert Insights on Writing JSON to a File
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Writing JSON to a file is a fundamental skill for data management and integration. It is essential to ensure that the data structure is properly formatted, as any discrepancies can lead to errors in data processing later on.”
Michael Thompson (Software Engineer, CodeMaster Solutions). “When writing JSON to a file, it is crucial to utilize the appropriate libraries and methods specific to the programming language in use. For instance, in Python, the ‘json’ module provides straightforward functions that simplify this process, ensuring data integrity and ease of access.”
Linda Xu (Technical Writer, Developer’s Digest). “Documentation is key when writing JSON to a file. Clear comments and explanations within the code can greatly assist other developers in understanding the structure and purpose of the JSON data, thereby enhancing collaboration and reducing the likelihood of errors.”
Frequently Asked Questions (FAQs)
How can I write JSON data to a file in Python?
To write JSON data to a file in Python, use the `json` module. First, convert your data to a JSON string using `json.dumps()` or directly write it using `json.dump()`. For example:
“`python
import json
data = {“name”: “John”, “age”: 30}
with open(‘data.json’, ‘w’) as json_file:
json.dump(data, json_file)
“`
What is the difference between json.dump() and json.dumps()?
`json.dump()` writes JSON data directly to a file-like object, while `json.dumps()` converts the data into a JSON string. Use `json.dump()` for file operations and `json.dumps()` when you need the JSON string for other purposes.
Can I write pretty-printed JSON to a file?
Yes, you can write pretty-printed JSON by using the `indent` parameter in `json.dump()`. For example:
“`python
json.dump(data, json_file, indent=4)
“`
This will format the JSON with indentation for better readability.
What file extension should I use for JSON files?
The standard file extension for JSON files is `.json`. This convention helps identify the file format and ensures compatibility with various applications and libraries.
Is it safe to overwrite an existing JSON file when writing?
Overwriting an existing JSON file can lead to data loss. It is advisable to back up the original file or use a temporary file during the write operation, renaming it only after the write is successful.
What should I do if I encounter a JSONEncodeError while writing to a file?
A `JSONEncodeError` typically occurs when the data contains non-serializable types. Ensure that all data structures are composed of JSON-compatible types, such as dictionaries, lists, strings, numbers, and booleans. Use custom serialization methods if necessary.
Writing JSON to a file is a straightforward process that involves converting a JSON object into a string format and then saving it to a file system. This task is commonly performed in various programming languages, each offering built-in libraries or modules that facilitate the serialization of data into JSON format. The process generally includes creating or opening a file, writing the JSON string to that file, and ensuring that the file is properly closed to prevent data loss or corruption.
One of the critical aspects of writing JSON to a file is understanding the structure of JSON itself. JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. It is essential to ensure that the data being written adheres to the JSON syntax, which includes proper use of braces, brackets, and quotation marks. Failure to follow these conventions can lead to errors when attempting to read the file later.
Additionally, error handling is an important consideration when writing JSON to a file. It is advisable to implement mechanisms to catch and manage exceptions that may arise during file operations, such as issues related to file permissions or disk space. This not only enhances the robustness of the application but also improves the user experience
Author Profile
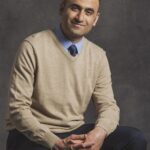
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?