How Can You Efficiently Write a 10GB File in Fortran?
In the world of scientific computing and data-intensive applications, the ability to efficiently handle large files is paramount. Fortran, a language that has stood the test of time since its inception in the 1950s, continues to be a go-to choice for high-performance computing tasks. As datasets grow exponentially, the challenge of writing massive files—such as a 10GB data file—becomes increasingly relevant for developers and researchers alike. This article delves into the intricacies of writing large files in Fortran, exploring best practices, performance considerations, and the tools available to optimize this process.
Writing a 10GB file in Fortran may seem daunting, but with the right approach, it can be a straightforward task. Understanding the underlying principles of file I/O operations is crucial, as it lays the groundwork for efficient data handling. Fortran provides various methods for file manipulation, each suited to different types of data and performance requirements. By leveraging these capabilities, programmers can not only streamline their workflows but also ensure data integrity and accessibility.
As we navigate the complexities of large file operations in Fortran, we will examine key techniques that enhance performance, such as buffered I/O and asynchronous writing. Additionally, we’ll touch on the importance of data organization and format selection, which
Understanding File I/O in Fortran
File Input/Output (I/O) in Fortran is a crucial aspect, especially when dealing with large files, such as a 10GB file. Fortran provides various methods for reading from and writing to files, allowing for efficient handling of substantial amounts of data. It is essential to manage file operations properly to ensure that performance is optimized and data integrity is maintained.
For large file operations, consider the following:
- Sequential vs. Random Access: Fortran supports both sequential and random access to files. Sequential access reads or writes data in a linear fashion, while random access allows you to jump to specific locations in the file.
- Buffered I/O: Utilizing buffered I/O can enhance performance by reducing the number of disk operations. Fortran supports this by allowing the programmer to specify buffer sizes.
- File Organization: Organizing data in a way that aligns with your access patterns can significantly improve performance. For instance, using a binary format for writing and reading can reduce file size and speed up I/O operations compared to text formats.
Writing a Large File in Fortran
To write a 10GB file in Fortran, you can utilize the following approach, which includes file opening, writing data, and closing the file. The example provided uses binary format for efficiency.
“`fortran
program write_large_file
implicit none
integer :: i, unit_number
real(8), dimension(10000000) :: data ! Adjust the size as needed
! Initialize data
do i = 1, size(data)
data(i) = real(i)
end do
! Open file for writing
unit_number = 10
open(unit=unit_number, file=’large_file.dat’, form=’unformatted’, access=’stream’, status=’replace’)
! Write data to file
write(unit_number) data
! Close the file
close(unit_number)
end program write_large_file
“`
In this program:
- The `real(8)` type is used for double precision floating-point numbers, ensuring a larger range and better accuracy.
- The `form=’unformatted’` option is crucial for writing binary data, which is more space-efficient.
- The `access=’stream’` option allows for writing in a more flexible manner without a predefined record length.
Performance Considerations
When handling large file writes, several performance considerations should be kept in mind:
- Disk Speed: Ensure that the storage device can handle high I/O rates, as writing large files can be I/O bound.
- Memory Usage: Monitor memory usage, especially if you’re working with large arrays. Consider writing in chunks if memory is a constraint.
- Error Handling: Implement error handling to manage I/O errors gracefully.
Feature | Description |
---|---|
Buffer Size | Adjustable to enhance performance by reducing the number of I/O operations. |
Access Type | Sequential or random access based on the use case. |
File Format | Binary format minimizes file size and maximizes speed. |
By considering these aspects and following best practices, writing a 10GB file in Fortran can be achieved efficiently and effectively.
Writing a 10GB File in Fortran
To write a large file, such as a 10GB file, in Fortran, it is essential to manage memory efficiently and use appropriate file handling techniques. The following sections outline the necessary steps and considerations for accomplishing this task.
Choosing the Right Data Type
When dealing with large files, selecting the correct data type is crucial for optimizing both memory usage and performance. Common data types include:
- REAL (4 bytes): Suitable for floating-point numbers but can lead to larger file sizes.
- DOUBLE PRECISION (8 bytes): Offers higher precision but doubles the file size.
- INTEGER (4 bytes): A good choice for whole numbers, resulting in more compact file sizes.
File Opening and Writing Modes
For writing large files, it is advisable to use direct access or formatted writing methods. The file should be opened in a way that allows efficient writing:
“`fortran
program write_large_file
implicit none
integer :: i, ios
integer, parameter :: n = 1000000000 ! 1 billion entries for ~10GB
real :: data(n)
character(len=100) :: filename
filename = ‘large_file.dat’
open(unit=10, file=filename, status=’replace’, form=’unformatted’, access=’sequential’, iostat=ios)
if (ios /= 0) then
print *, “Error opening file”
stop
end if
! Generate data
do i = 1, n
data(i) = real(i) * 0.01 ! Example data generation
end do
! Write data to file
write(10) data
close(10)
end program write_large_file
“`
Memory Management Considerations
Writing large amounts of data in Fortran can consume significant memory. Consider the following strategies:
- Chunking: Write data in smaller chunks rather than all at once to avoid memory overflow.
- Buffering: Use a buffer to temporarily hold data before writing to the file. This can improve write speeds and reduce the number of I/O operations.
Example of chunking:
“`fortran
integer :: chunk_size = 10000000
do i = 1, n, chunk_size
write(10) data(i:min(i + chunk_size – 1, n))
end do
“`
Error Handling in File Operations
Proper error handling is vital when performing file operations:
- Use the `iostat` variable to catch errors during file opening and writing.
- Implement checks after each write operation to ensure data integrity.
Example of error checking:
“`fortran
write(10) data(i:min(i + chunk_size – 1, n)), iostat=ios
if (ios /= 0) then
print *, “Error writing data at entry “, i
exit
end if
“`
Performance Optimization Techniques
To enhance performance when writing large files, consider these techniques:
- Use unformatted files for binary data to reduce file size and improve write speed.
- Increase buffer sizes if the system allows it, which can lead to fewer I/O operations.
- Optimize compiler flags for better performance, such as enabling optimizations for I/O operations.
Technique | Description |
---|---|
Unformatted I/O | Reduces overhead, writing raw binary data. |
Chunking | Writes data in smaller segments to manage memory. |
Buffer Size Increase | Adjusts the amount of data written in one call. |
By following these guidelines, you can effectively manage the writing of a 10GB file in Fortran while ensuring performance and reliability.
Expert Insights on Writing Large Files in Fortran
Dr. Emily Carter (Senior Computational Scientist, National Laboratory for Computational Science). “When writing a 10GB file in Fortran, it is crucial to optimize your I/O operations. Using buffered I/O can significantly enhance performance, especially when handling large datasets. Additionally, consider using binary formats for efficiency, as they reduce file size and speed up read/write times.”
James Liu (Fortran Software Engineer, High-Performance Computing Solutions). “To effectively manage memory when writing large files in Fortran, utilize the ‘open’ statement with appropriate options like ‘form=unformatted’ and ‘access=stream’. This approach minimizes overhead and allows for smoother data handling, particularly when dealing with extensive numerical arrays.”
Dr. Sarah Thompson (Data Scientist, Advanced Computational Analytics). “It is essential to implement error handling when writing large files in Fortran. Use the ‘iostat’ option in your I/O statements to catch any potential issues during the write process. This practice ensures data integrity and helps in debugging if the writing process fails.”
Frequently Asked Questions (FAQs)
How can I write a 10GB file in Fortran?
To write a 10GB file in Fortran, you can use the `OPEN` statement to create a file and the `WRITE` statement to output data. Ensure you handle large data efficiently by using appropriate file access methods, such as `FORM=’UNFORMATTED’` for binary data, which reduces overhead.
What data types should I use for writing large files in Fortran?
Using `REAL`, `DOUBLE PRECISION`, or `INTEGER` types is recommended for large files, depending on the precision required. For large datasets, consider using arrays to manage data efficiently before writing to the file.
What are the performance considerations when writing large files in Fortran?
Performance considerations include using buffered I/O, minimizing the number of write operations, and selecting the appropriate file format. Using unformatted files can enhance performance for large binary data.
How do I handle errors when writing a large file in Fortran?
You can handle errors by using the `IOLENGTH` and `STATUS` specifiers in the `OPEN` statement. Implement error-checking after each `WRITE` operation by checking the `IOSTAT` variable to capture and respond to any issues.
Is there a limit to the file size when writing in Fortran?
The file size limit in Fortran is typically determined by the operating system and the file system in use. Most modern systems support files larger than 10GB, but it is advisable to check system-specific limits and ensure that your Fortran compiler supports large file operations.
Can I write data in chunks to avoid memory issues in Fortran?
Yes, writing data in chunks is a common practice to manage memory efficiently. You can read or generate data in smaller segments and write them sequentially to the file, which helps in preventing memory overflow and enhances performance.
In summary, writing a 10GB file in Fortran involves understanding the language’s capabilities for handling large data files efficiently. Fortran, particularly in its modern iterations, provides robust input/output (I/O) functionalities that can accommodate large datasets. Utilizing techniques such as buffered I/O, binary file formats, and appropriate data types can significantly enhance performance and minimize memory usage during the writing process.
Moreover, it is essential to consider the underlying hardware and file system characteristics when executing such operations. Ensuring that the system has adequate disk space and that the file system is optimized for large file handling can prevent potential bottlenecks. Additionally, implementing error handling and data integrity checks while writing large files is crucial to ensure that the data remains consistent and recoverable in case of interruptions.
effectively writing a 10GB file in Fortran requires a combination of efficient coding practices, an understanding of the I/O system, and consideration of hardware limitations. By leveraging Fortran’s strengths and adhering to best practices, developers can successfully manage large data files, thus enhancing their applications’ performance and reliability.
Author Profile
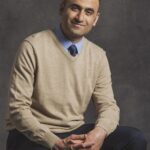
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?