Why Am I Seeing the ’12: Error: SOAP Struct Not Initialized’ Message?
In the world of software development, encountering errors is a common hurdle that can test even the most seasoned programmers. Among these, the enigmatic message `12: error: soap struct not initialized?` can leave developers scratching their heads, particularly those working with SOAP (Simple Object Access Protocol) web services. This error often signifies an underlying issue with the initialization of structures within the SOAP framework, and understanding it is crucial for efficient debugging and application performance. In this article, we will delve into the intricacies of this error, explore its common causes, and provide insights on how to effectively resolve it, ensuring your web services run smoothly and reliably.
The `soap struct not initialized` error typically arises when a SOAP client or server attempts to utilize a data structure that has not been properly set up. This can occur for a variety of reasons, such as missing configuration settings, improper instantiation of objects, or even issues with the underlying libraries used for SOAP communication. As developers navigate the complexities of web services, recognizing the signs of this error and understanding its implications becomes essential for maintaining robust and functional applications.
In our exploration, we will outline the typical scenarios that lead to this error, discuss best practices for initializing SOAP structures, and highlight troubleshooting techniques that can save developers valuable time
Error Diagnosis
The error message `12: error: soap struct not initialized?` typically arises in scenarios involving SOAP (Simple Object Access Protocol) web services. This error indicates that a data structure intended for SOAP communication has not been properly initialized before use.
Common causes for this error include:
- Missing initialization code for the SOAP data structure.
- Incorrect usage of the SOAP library or framework.
- Programming logic errors that prevent the structure from being set up correctly.
To effectively address this issue, it is essential to verify that all required fields in the SOAP struct are initialized before making a SOAP call.
SOAP Struct Initialization
Initialization of SOAP structs usually requires specific attention to detail. Each struct should be populated with valid values to ensure that the SOAP request is formatted correctly and can be processed by the server.
Here’s a typical approach to initializing a SOAP struct:
- Define the Struct: Ensure the struct is defined in accordance with the specifications of the SOAP service.
- Allocate Memory: Depending on the programming language being used, make sure to allocate memory if necessary.
- Set Default Values: Assign default values to all fields within the struct.
- Error Handling: Implement checks to confirm that the struct is not null before proceeding with the SOAP call.
Step | Action |
---|---|
1 | Define the struct as per the WSDL (Web Services Description Language). |
2 | Use appropriate methods to allocate memory (if required by the programming environment). |
3 | Initialize all fields in the struct with valid data. |
4 | Implement error checking to confirm the struct is properly initialized. |
Debugging Techniques
When encountering this error, several debugging techniques can help identify the underlying issue:
- Print Debugging: Output the state of the struct before making the SOAP call to ensure all fields are populated.
- Use Logging: Implement logging throughout the code to capture the flow of execution and state of variables.
- Step-through Debugging: Utilize debugging tools to step through the code line by line, allowing for a close examination of when the struct is initialized.
Best Practices
To avoid encountering this error in the future, consider the following best practices:
- Consistent Initialization: Always initialize structs immediately after defining them.
- Documentation: Keep documentation updated regarding the expected structure and data types for SOAP requests.
- Unit Testing: Regularly test the SOAP integration with unit tests that validate the initialization of structs.
By applying these strategies, developers can reduce the likelihood of encountering the `soap struct not initialized` error, ensuring smoother interactions with SOAP web services.
Understanding the Error
The error message `12: error: soap struct not initialized?` typically arises when working with SOAP (Simple Object Access Protocol) in programming environments. This error indicates that a structure expected by the SOAP library has not been properly initialized before being used.
Common Causes of the Error
Several factors can lead to the occurrence of this error:
- Uninitialized Variables: The most frequent cause is the failure to initialize a structure that is intended to be sent or received via SOAP.
- Incorrect Data Types: Using the wrong data type for a SOAP struct can lead to initialization issues.
- Library Version Mismatches: Sometimes, using incompatible versions of the SOAP library can result in unexpected behavior.
- Memory Management Issues: Improper handling of memory allocation and deallocation can cause structures to become uninitialized.
How to Diagnose the Problem
To effectively diagnose the issue, consider the following steps:
- Check Initialization: Ensure that all SOAP structs are explicitly initialized before use.
- Review Code for Typos: Look for typographical errors that may prevent proper struct initialization.
- Examine Data Types: Verify that the types of the variables match those expected by the SOAP methods.
- Debugging Tools: Utilize debugging tools to step through the code and identify where the struct fails to initialize.
- Consult Documentation: Refer to the documentation of the SOAP library being used to understand the required initialization process.
Solutions and Best Practices
To resolve the error and prevent it from occurring in the future, follow these best practices:
- Initialize Structures: Always initialize your SOAP structs using appropriate constructors or initialization functions.
- Use Default Values: If applicable, set default values for the members of the struct to prevent behavior.
- Consistent Testing: Regularly test your SOAP implementations to catch potential issues early.
- Error Handling: Implement error handling to gracefully manage scenarios where initialization fails.
- Version Control: Keep your SOAP library versions consistent across your development and production environments.
Example Code Snippet
Here is a simple example demonstrating the proper initialization of a SOAP struct:
“`c
include
struct myStruct {
char *data;
};
void exampleFunction() {
struct myStruct myData;
// Properly initialize the struct
myData.data = NULL; // or allocate memory as needed
// Ensure that other SOAP related functions use this struct after initialization
soap_call_myFunction(soapInstance, NULL, NULL, &myData);
}
“`
Resources for Further Assistance
For additional support and resources, consider the following:
- Official Documentation: Review the documentation for the SOAP library you are using.
- Online Forums: Participate in community forums such as Stack Overflow for peer assistance.
- Example Repositories: Explore GitHub repositories that implement SOAP to see best practices in action.
- Technical Support: If all else fails, consider reaching out to technical support for the specific SOAP implementation.
Understanding SOAP Struct Initialization Errors
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ’12: error: soap struct not initialized’ message typically indicates that the SOAP struct has not been properly instantiated before use. This can lead to unexpected behavior in web service interactions, and developers should ensure that all necessary fields are initialized to avoid such errors.”
Mark Thompson (Lead Developer, Web Services Solutions). “In my experience, this error often arises when developers overlook the initialization of complex data types in their SOAP requests. It’s crucial to review the API documentation and ensure that all required parameters are set correctly to prevent this issue.”
Linda Zhang (Technical Architect, Cloud Computing Experts). “Properly initializing SOAP structs is essential for the integrity of data transmission. I recommend implementing thorough validation checks in your code to catch uninitialized structs early in the development process, thus enhancing overall application reliability.”
Frequently Asked Questions (FAQs)
What does the error “12: error: soap struct not initialized” indicate?
This error indicates that a SOAP structure has not been properly initialized before being used in a SOAP request or response. It typically occurs when the code attempts to access or manipulate a SOAP object that has not been allocated memory or set up correctly.
How can I resolve the “soap struct not initialized” error?
To resolve this error, ensure that all necessary SOAP structures are initialized before use. This includes calling the appropriate initialization functions for the SOAP client or server and verifying that all required fields are set.
What are common causes of the “soap struct not initialized” error?
Common causes include failing to call the initialization function for the SOAP context, missing required parameters in the SOAP struct, or attempting to use a struct that has been freed or not allocated.
Can this error occur in both client and server SOAP implementations?
Yes, this error can occur in both client and server implementations of SOAP. It is crucial for both sides to ensure that all SOAP structures are correctly initialized before making calls or processing responses.
What programming languages are commonly associated with this error?
This error is often encountered in programming languages that utilize SOAP libraries, such as C, C++, Python, and PHP. Each language may have its own specific initialization requirements for SOAP structures.
Are there any debugging tips for the “soap struct not initialized” error?
Debugging tips include checking for proper initialization calls in the code, using logging to trace the flow of execution, and validating that all necessary fields in the SOAP struct are populated before use. Additionally, reviewing documentation for the specific SOAP library can provide insights into proper usage.
The error message “12: error: soap struct not initialized” typically arises in the context of using the SOAP (Simple Object Access Protocol) library in programming, particularly when dealing with web services. This error indicates that a SOAP structure, which is essential for handling requests and responses, has not been properly initialized before it is used. The failure to initialize these structures can lead to unexpected behavior, crashes, or failure to communicate with the web service effectively.
To address this issue, developers should ensure that all necessary SOAP structures are initialized before invoking any operations. This includes allocating memory for the structures and setting their fields to appropriate default values. Additionally, it is crucial to check the documentation of the SOAP library being used, as different implementations may have specific initialization requirements or functions that need to be called prior to usage.
Furthermore, implementing thorough error handling and debugging practices can help identify the root cause of the error. Developers are encouraged to utilize logging mechanisms to capture the state of the application at various points, which can provide insights into where the initialization process may be failing. By following best practices for initializing SOAP structures and employing robust debugging techniques, developers can mitigate the occurrence of this error and enhance the reliability of their web service interactions.
Author Profile
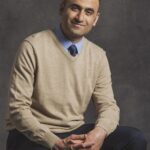
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?