Why Am I Seeing the ‘Java Virtual Machine Launcher: A Java Exception Has Occurred’ Error?
Have you ever been deep into a coding project, only to be abruptly halted by the ominous message: “Java Virtual Machine Launcher: A Java Exception Has Occurred”? If you’re a developer or even a casual user of Java applications, this frustrating notification can feel like a roadblock, leaving you puzzled and searching for answers. Understanding the underlying causes of this error is essential not only for troubleshooting but also for enhancing your overall Java experience. In this article, we will explore the common reasons behind this exception, its implications, and how you can effectively resolve it to get back on track.
Java, known for its versatility and portability, relies heavily on the Java Virtual Machine (JVM) to execute applications. When an exception occurs within this environment, it signifies that something has gone awry, whether due to misconfigurations, compatibility issues, or other runtime problems. The JVM serves as a crucial bridge between your code and the underlying operating system, and when it encounters an obstacle, it can lead to the dreaded error message that disrupts your workflow.
Navigating the complexities of Java exceptions can be daunting, especially for those new to the language or its ecosystem. However, by gaining a deeper understanding of the JVM’s architecture and the common pitfalls that lead to these exceptions
Common Causes of Java Exceptions
Java exceptions can occur due to various reasons, often linked to the underlying application code or the configuration of the Java environment. Understanding these causes is crucial for effective troubleshooting. Here are some of the most frequent culprits:
- Incorrect Java Version: Running an application on an incompatible Java version can lead to exceptions. Ensure the application is designed for the version of Java currently in use.
- Classpath Issues: Missing or incorrectly set classpaths can prevent Java from locating necessary libraries or classes, resulting in exceptions.
- Corrupted Installation: A corrupted Java installation can lead to unexpected errors. Reinstalling Java may resolve these issues.
- Insufficient Memory: Java applications require adequate memory to function properly. Lack of memory can lead to `OutOfMemoryError`.
- Faulty Code: Syntax errors, logical errors, or unhandled exceptions in the application code itself can trigger runtime exceptions.
Troubleshooting Steps
When encountering a Java exception, following a systematic approach can help identify and resolve the issue effectively. Here are essential steps to consider:
- Check Java Version: Verify that the correct version of Java is being used for the application.
- Review Classpath: Ensure that all required libraries and dependencies are correctly included in the classpath.
- Inspect Error Messages: Pay close attention to the error messages provided by the Java Virtual Machine (JVM). They often contain valuable information regarding the nature of the exception.
- Update or Reinstall Java: If issues persist, consider updating or reinstalling Java to ensure a clean installation.
- Examine Code: Review the application code to identify any potential bugs or unhandled exceptions.
- Monitor System Resources: Check the system’s memory and CPU usage to ensure the application has sufficient resources.
Java Exception Types and Their Meanings
Java exceptions are categorized into two main types: checked exceptions and unchecked exceptions. Understanding these types aids in effective debugging.
Exception Type | Description |
---|---|
Checked Exceptions | Exceptions that must be either caught or declared in the method signature. Examples include IOException and SQLException. |
Unchecked Exceptions | Exceptions that do not need to be explicitly handled or declared. These include RuntimeException and its subclasses, such as NullPointerException and ArrayIndexOutOfBoundsException. |
Best Practices for Avoiding Java Exceptions
To minimize the risk of encountering Java exceptions, developers should adopt best practices in coding and application design:
- Use Try-Catch Blocks: Implement try-catch blocks to handle exceptions gracefully and maintain application stability.
- Validate Input: Always validate user inputs to prevent unexpected exceptions due to invalid data.
- Keep Dependencies Updated: Regularly update libraries and dependencies to their latest versions to mitigate compatibility issues.
- Implement Logging: Utilize logging frameworks to capture exception details, which aids in debugging and improves maintainability.
- Conduct Code Reviews: Regular code reviews can help identify potential issues early in the development process.
By adhering to these practices, developers can create more robust Java applications that are less prone to exceptions and easier to maintain.
Understanding the Java Exception
When encountering the message “A Java Exception has occurred” from the Java Virtual Machine (JVM) launcher, it indicates that an unexpected error has interrupted the execution of a Java application. This error can stem from various causes, and understanding these can aid in troubleshooting.
Common Causes of Java Exceptions
Several factors can contribute to Java exceptions, including:
- Corrupted installation: A damaged or incomplete Java installation can lead to exceptions during runtime.
- Incompatible software: Running Java applications on incompatible operating systems or versions can trigger exceptions.
- Insufficient memory: The application may require more memory than is available, resulting in an OutOfMemoryError.
- Classpath issues: Incorrectly defined classpaths may prevent the JVM from finding necessary classes and resources.
- Code errors: Bugs in the application code itself can lead to runtime exceptions.
Troubleshooting Steps
When a Java exception occurs, follow these troubleshooting steps:
- Check Java Installation:
- Verify that the latest version of Java is installed.
- Reinstall Java to ensure all components are intact.
- Examine Application Logs:
- Look for detailed error messages in the logs generated by the application.
- These logs often provide insights into the specific nature of the exception.
- Review System Requirements:
- Ensure the application meets the necessary system requirements, including CPU, RAM, and OS version.
- Adjust Java Options:
- Modify JVM options, such as increasing the heap size with `-Xmx` and `-Xms` parameters.
- Example:
“`bash
java -Xms512m -Xmx1024m -jar yourApplication.jar
“`
- Check Classpath:
- Ensure that the classpath is set correctly and includes all required libraries and dependencies.
Common Java Exceptions and Their Solutions
Exception Type | Description | Suggested Solution |
---|---|---|
NullPointerException | Attempted to use an object reference that is null. | Check for null references before use. |
ArrayIndexOutOfBoundsException | Accessing an array with an invalid index. | Validate indices before accessing arrays. |
ClassNotFoundException | The specified class could not be found. | Check classpath and ensure the class is available. |
OutOfMemoryError | The JVM has run out of memory. | Increase heap size or optimize memory usage. |
Preventive Measures
To minimize the occurrence of Java exceptions, consider implementing the following strategies:
- Regular Updates: Keep Java and all related libraries up to date to benefit from fixes and improvements.
- Code Reviews: Conduct thorough code reviews to identify potential bugs early in development.
- Monitoring Tools: Utilize monitoring tools to track application performance and catch exceptions proactively.
- Unit Testing: Implement unit tests to cover critical paths and catch exceptions during development.
By understanding the nature of Java exceptions, common causes, and effective troubleshooting methods, users can address issues promptly and maintain the smooth operation of Java applications.
Understanding Java Virtual Machine Launcher Exceptions
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “The error message ‘A Java exception has occurred’ typically indicates that there is an issue with the Java application or the environment it is running in. It is crucial to check the application’s logs for specific stack traces that can provide insights into the root cause of the exception.”
Michael Chen (Java Performance Analyst, CodeCraft Solutions). “When encountering a Java exception during the launch of a Java application, developers should first verify their Java Runtime Environment (JRE) version. Incompatibilities between the application and the JRE can often lead to such exceptions, so ensuring alignment is essential.”
Sarah Patel (Software Engineer, Open Source Projects). “It is advisable to run the Java application with increased verbosity by using the ‘-verbose’ flag. This can help in identifying the exact point of failure and the nature of the exception, allowing for a more targeted debugging approach.”
Frequently Asked Questions (FAQs)
What does “A Java Exception has occurred” mean?
This message indicates that an error occurred during the execution of a Java application, often due to issues such as missing files, incorrect configurations, or incompatible Java versions.
What are common causes of the Java Virtual Machine Launcher error?
Common causes include corrupted Java installation, incompatible Java versions, insufficient system resources, or issues with the application’s code itself.
How can I troubleshoot the Java Virtual Machine Launcher error?
To troubleshoot, ensure that Java is properly installed, check for updates, verify the application’s compatibility with your Java version, and review the system’s environment variables.
Is it necessary to reinstall Java to fix this error?
Reinstalling Java can resolve the issue if the installation is corrupted. However, it is advisable to first try other troubleshooting steps before resorting to reinstallation.
Can I run Java applications without the Java Virtual Machine?
No, the Java Virtual Machine (JVM) is essential for executing Java applications, as it provides the environment in which the code runs.
What steps can I take if the error persists after troubleshooting?
If the error persists, consider checking the application’s documentation for specific requirements, seeking support from the developer, or reviewing online forums for similar issues and solutions.
The Java Virtual Machine (JVM) Launcher is a crucial component in the Java programming ecosystem, responsible for starting Java applications by executing Java bytecode. However, users often encounter the error message “A Java Exception has occurred,” which indicates that an issue has arisen during the execution of a Java application. This error can stem from various sources, including configuration problems, compatibility issues, or bugs within the application itself. Understanding the root cause of this error is essential for effective troubleshooting and resolution.
Common causes of the “A Java Exception has occurred” error include incorrect Java installation, insufficient memory allocation, and conflicts with other software or system settings. Users may also experience this error when attempting to run applications that require a specific version of Java that is not installed on their system. Therefore, ensuring that the correct version of the Java Runtime Environment (JRE) or Java Development Kit (JDK) is installed and properly configured is vital for smooth application performance.
To resolve this error, users should first verify their Java installation and check for any updates or necessary configurations. Additionally, reviewing the application’s documentation for specific requirements can provide insights into potential compatibility issues. If the problem persists, users may need to consult online forums or support resources for further assistance.
Author Profile
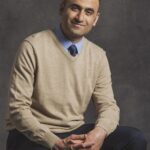
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?